/* 在二元树中找出和为某一值的所有路径(树) 题目:输入一个整数和一棵二元树。 从树的根结点开始往下访问一直到叶结点所经过的所有结点形成一条路径。 打印出和与输入整数相等的所有路径。 例如 输入整数 22 和如下二元树 10 / 5 12 / 7 则打印出两条路径:10, 12 和 10, 5, 7 我们定义的二元查找树 节点的数据结构如下: #define Element int struct TreeNode { Element data; struct TreeNode *left; struct TreeNode *right; }; typedef struct TreeNode *Position; typedef Position BinarySearchTree; */
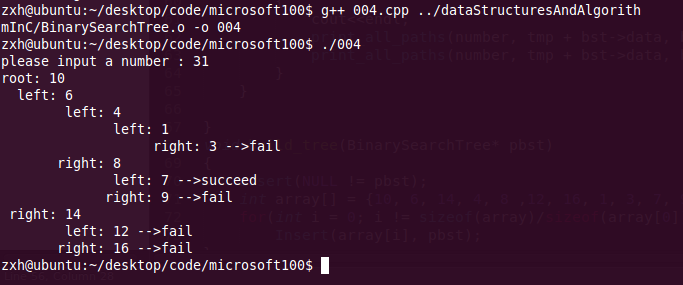
#include <iostream> #include <iomanip> #include <assert.h> #include "/home/zxh/desktop/code/dataStructuresAndAlgorithmInC/BinarySearchTree.h" using namespace std; void print_all_paths(int number, int tmp, BinarySearchTree bst, int depth, int ctrl)//ctrl:0=root 1=left 2=right { if(NULL == bst) return; cout<<setw(depth); if(0 == ctrl) cout<<"root: "; else if(1 == ctrl) cout<<"left: "; else if(2 == ctrl) cout<<"right: "; cout<<bst->data; if(NULL == bst->left && NULL == bst->right) { if(number == tmp + bst->data) cout<<" -->succeed"<<endl; else cout<<" -->fail"<<endl; return; } else // bst is not a leaf {
cout<<endl; print_all_paths(number, tmp + bst->data, bst->left, depth + 6, 1); print_all_paths(number, tmp + bst->data, bst->right, depth + 6, 2); } } void build_tree(BinarySearchTree* pbst) { assert(NULL != pbst); int array[] = {10, 6, 14, 4, 8 ,12, 16, 1, 3, 7, 9, 11, 20, 18, 17}; for(int i = 0; i != sizeof(array)/sizeof(array[0]) ; ++i) Insert(array[i], pbst); } int main(int argc, char const *argv[]) { BinarySearchTree bst = NULL; build_tree(&bst); if(NULL == bst)cout<<"NULL"<<endl; int n; cout<<"please input a number : "; cin>>n; print_all_paths(n, 0, bst, 2, 0); return 0; }