本文不讨论Silverlight全屏模式的实现,有关实现这个,可以参考TerryLee的这篇文章,核心代码就是这行:
Application.Current.Host.Content.IsFullScreen = !Application.Current.Host.Content.IsFullScreen;
本文要讨论的是Silverlight的局部元素全屏,即Element部分全屏。我们在做Silverlight项目中有时候客户有这种需求:希望放大界面的局部,比如一个列表面板啥的,而不是整个界面全屏。如下图:
实现部分全屏
实现起来也是比较简单,主要思路是用Popup实现弹出全屏,把需要全屏的元素放到容器里并设置为屏幕大小,然后整体实现全屏;当用户退出全屏的时候需要把元素恢复回原位置。好,我们写一个ElementsFullScreenProvider类,实现一个UIElement的扩展方法即可。
主要实现类:ElementsFullScreenProvider

1 #region "Using Namespace" 2 3 using System; 4 5 using System.Windows; 6 7 using System.Windows.Controls; 8 9 using System.Windows.Controls.Primitives; 10 11 #endregion 12 13 namespace ElementFullScreen 14 15 { 16 17 /// <summary> 18 19 /// Make partial UI elements full screen 20 21 /// Provider features: 22 23 /// make UI element full screen 24 25 /// exit full screen and restore UI element 26 27 /// support UIElement.ToggleElementFullScreen -- extension methods 28 29 /// </summary> 30 31 public static class ElementsFullScreenProvider 32 33 { 34 35 #region Members 36 37 static readonly Popup PopupContainer; 38 39 static readonly Panel PopupContentContainer; 40 41 static ElementController _lastElementController; 42 43 #endregion 44 45 #region ElementController 46 47 class ElementController 48 49 { 50 51 double _height = double.NaN; 52 53 double _width = double.NaN; 54 55 int _lastPanelPosition; 56 57 bool _lastPopupIsOpen; 58 59 readonly DependencyObject _parent; 60 61 Thickness? _margin; 62 63 public UIElement Element { get; private set; } 64 65 public ElementController(UIElement element) 66 67 { 68 69 Element = element; 70 71 var elem = element as FrameworkElement; 72 73 if (elem != null && elem.Parent != null) 74 75 { 76 77 _parent = elem.Parent; 78 79 } 80 81 } 82 83 public void BringElementToFullScreen() 84 85 { 86 87 TryAction<frameworkelement>(Element, f => 88 89 { 90 91 _height = f.Height; 92 93 _width = f.Width; 94 95 f.Height = double.NaN; 96 97 f.Width = double.NaN; 98 99 }); 100 101 TryAction<control>(Element, f => 102 103 { 104 105 _margin = f.Margin; 106 107 f.Margin = new Thickness(0); 108 109 }); 110 111 if (_parent != null) 112 113 { 114 115 if (!TryAction<panel>(_parent, p => { _lastPanelPosition = p.Children.IndexOf(Element); p.Children.RemoveAt(_lastPanelPosition); })) 116 117 if (!TryAction<contentcontrol>(_parent, c => c.Content = null)) 118 119 if (!TryAction<usercontrol>(_parent, u => u.Content = null)) 120 121 TryAction<popup>(_parent, p => { _lastPopupIsOpen = p.IsOpen; p.Child = null; }); 122 123 } 124 125 } 126 127 public void ReturnElementFromFullScreen() 128 129 { 130 131 TryAction<frameworkelement>(Element, f => 132 133 { 134 135 f.Height = _height; 136 137 f.Width = _width; 138 139 }); 140 141 TryAction<control>(Element, f => 142 143 { 144 145 if (_margin.HasValue) 146 147 { 148 149 f.Margin = _margin.Value; 150 151 } 152 153 }); 154 155 if (_parent != null) 156 157 { 158 159 if (!TryAction<panel>(_parent, p => p.Children.Insert(_lastPanelPosition, Element))) 160 161 if (!TryAction<contentcontrol>(_parent, c => c.Content = Element)) 162 163 if (!TryAction<usercontrol>(_parent, u => u.Content = Element)) 164 165 TryAction<popup>(_parent, p => { p.Child = Element; p.IsOpen = _lastPopupIsOpen; }); 166 167 } 168 169 } 170 171 static bool TryAction<t>(object o, Action<t> action) 172 173 where T : class 174 175 { 176 177 T val = o as T; 178 179 if (val != null) 180 181 { 182 183 action(val); 184 185 return true; 186 187 } 188 189 return false; 190 191 } 192 193 } 194 195 #endregion 196 197 #region FullscreenElementID Attached Property 198 199 static readonly DependencyProperty FullscreenElementIDProperty = DependencyProperty.RegisterAttached( 200 201 "FullscreenElementID", typeof(Guid?), typeof(ElementsFullScreenProvider), new PropertyMetadata(null)); 202 203 static void SetFullscreenElementID(DependencyObject obj, Guid? value) 204 205 { 206 207 obj.SetValue(FullscreenElementIDProperty, value); 208 209 } 210 211 static Guid? GetFullscreenElementID(DependencyObject obj) 212 213 { 214 215 return (Guid?)obj.GetValue(FullscreenElementIDProperty); 216 217 } 218 219 #endregion 220 221 #region Initialization 222 223 /// <summary> 224 225 /// Initializes the <see cref="ElementsFullScreenProvider"> class. 226 227 /// </see></summary> 228 229 static ElementsFullScreenProvider() 230 231 { 232 233 PopupContentContainer = new Grid(); 234 235 PopupContainer = new Popup 236 237 { 238 239 Child = PopupContentContainer 240 241 }; 242 243 Application.Current.Host.Content.FullScreenChanged += delegate 244 245 { 246 247 if (_lastElementController == null) return; 248 249 if (!Application.Current.Host.Content.IsFullScreen) 250 251 { 252 253 ReturnElementFromFullScreen(); 254 255 } 256 257 else 258 259 { 260 261 UpdateContentSize(); 262 263 } 264 265 }; 266 267 } 268 269 #endregion 270 271 #region Public Methods 272 273 public static void BringElementToFullScreen(this UIElement element) 274 275 { 276 277 if (_lastElementController == null) 278 279 { 280 281 _lastElementController = new ElementController(element); 282 283 _lastElementController.BringElementToFullScreen(); 284 285 PopupContentContainer.Children.Add(element); 286 287 PopupContainer.IsOpen = true; 288 289 } 290 291 } 292 293 public static void ReturnElementFromFullScreen(this UIElement element) 294 295 { 296 297 ReturnElementFromFullScreen(); 298 299 } 300 301 public static void ReturnElementFromFullScreen() 302 303 { 304 305 if (_lastElementController != null) 306 307 { 308 309 PopupContentContainer.Children.Clear(); 310 311 _lastElementController.ReturnElementFromFullScreen(); 312 313 PopupContainer.IsOpen = false; 314 315 _lastElementController = null; 316 317 } 318 319 } 320 321 public static void ToggleElementFullScreen(this UIElement element) 322 323 { 324 325 bool newValue = !Application.Current.Host.Content.IsFullScreen; 326 327 bool toggle = false; 328 329 if (newValue) 330 331 { 332 333 if (_lastElementController == null) 334 335 { 336 337 element.BringElementToFullScreen(); 338 339 toggle = true; 340 341 } 342 343 } 344 345 else 346 347 { 348 349 if (_lastElementController != null && ReferenceEquals(element, _lastElementController.Element)) 350 351 { 352 353 element.ReturnElementFromFullScreen(); 354 355 toggle = true; 356 357 } 358 359 } 360 361 if (toggle) 362 363 { 364 365 ToggleFullScreen(); 366 367 } 368 369 } 370 371 public static void ToggleFullScreen() 372 373 { 374 375 Application.Current.Host.Content.IsFullScreen = !Application.Current.Host.Content.IsFullScreen; 376 377 } 378 379 #endregion 380 381 #region Private Method 382 383 private static void UpdateContentSize() 384 385 { 386 387 if (Application.Current != null && Application.Current.Host != null && Application.Current.Host.Content != null) 388 389 { 390 391 double height = Application.Current.Host.Content.ActualHeight; 392 393 double width = Application.Current.Host.Content.ActualWidth; 394 395 //if (Application.Current.Host.Settings.EnableAutoZoom) 396 397 //{ 398 399 // double zoomFactor = Application.Current.Host.Content.ZoomFactor; 400 401 // if (zoomFactor != 0.0) 402 403 // { 404 405 // height /= zoomFactor; 406 407 // width /= zoomFactor; 408 409 // } 410 411 //} 412 413 PopupContentContainer.Height = height; 414 415 PopupContentContainer.Width = width; 416 417 } 418 419 } 420 421 #endregion 422 423 } 424 425 }
调用的时候使用UIElement的扩展方法实现全屏:
1: private void button1_Click(object sender, RoutedEventArgs e)
2: {
3: SilverArea/*你希望全屏的元素*/.ToggleElementFullScreen();//调用UIElement的扩展方法
4: }
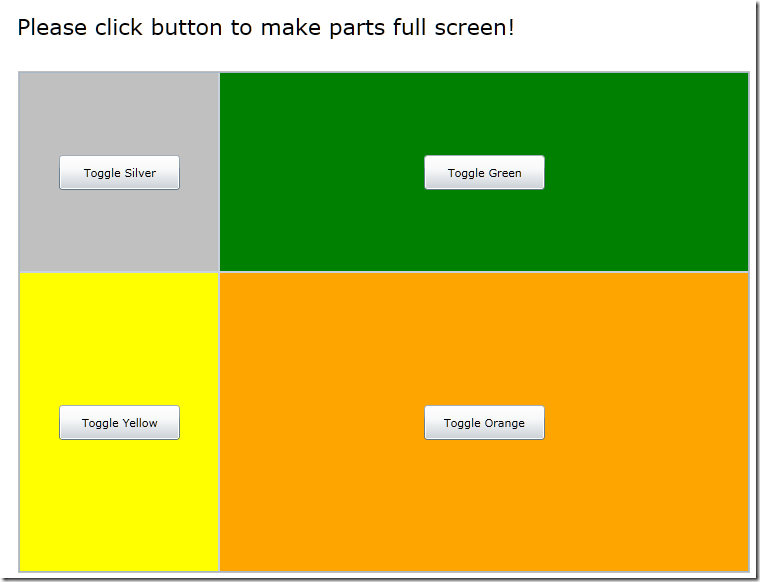
本文源码下载请点击此处。主要就一个类ElementsFullScreenProvider.cs,用起来就一个函数ToggleElementFullScreen(),简单之极。
说明:本文出处:http://www.cnblogs.com/Mainz/archive/2011/08/19/2145933.html