Description
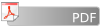
Little Valentine liked playing with binary trees very much. Her favorite game was constructing randomly looking binary trees with capital letters in the nodes.
This is an example of one of her creations:
D / / B E / / A C G / / F
To record her trees for future generations, she wrote down two strings for each tree: a preorder traversal (root, left subtree, right subtree) and an inorder traversal (left subtree, root, right subtree).
For the tree drawn above the preorder traversal is DBACEGF and the inorder traversal is ABCDEFG.
She thought that such a pair of strings would give enough information to reconstruct the tree later (but she never tried it).
Now, years later, looking again at the strings, she realized that reconstructing the trees was indeed possible, but only because she never had used the same letter twice in the same tree.
However, doing the reconstruction by hand, soon turned out to be tedious.
So now she asks you to write a program that does the job for her!
Input Specification
The input file will contain one or more test cases. Each test case consists of one line containing two strings preord and inord, representing the preorder traversal and inorder traversal of a binary tree. Both strings consist of unique capital letters. (Thus they are not longer than 26 characters.)Input is terminated by end of file.
Output Specification
For each test case, recover Valentine's binary tree and print one line containing the tree's postorder traversal (left subtree, right subtree, root).Sample Input
DBACEGF ABCDEFG BCAD CBAD
Sample Output
ACBFGED CDAB
思路:
题目将会告诉你一棵二叉树的前序和中序,求它的后序
前序:中,左,右
中序:左,中,右
后序:左,右,中
首先观察
DBACEGF ABCDEFG
由于前面一个是前序,所以正好可以由第一个元素确定这棵树的跟,然后在中序中查找到根的位置,正好又可以将中序遍历的树分为左子树和右子树,再对子树做类似的递归处理,就可以捋清一棵树了,注意建树的顺序为根左右。
最后是后序遍历,可以写成递归的形式,不过千万别忘了判断树是否到底了,不然程序会出错
#include<iostream> #include<cstdio> #include<cstring> using namespace std; struct node { char data; struct node*lchild; struct node*rchild; }; struct node *Build_tree(char *pre,char *in,int len) { if(len==0) return NULL; struct node*p; char *q; p=new (struct node); p->data=*pre; int k=0; for(q=in;q<in+len;q++) { if(*q==*pre) break; k++; } p->lchild=Build_tree(pre+1,in,k); //前半部分,所以传in,长度为k作为限制 p->rchild=Build_tree(pre+k+1,q+1,len-k-1); //后半部分,传q即可 return p; } void post_print(struct node *root) { if(root!=NULL) //是if!!!! { post_print(root->lchild); post_print(root->rchild); cout<<root->data; } } int main() { char a[100],b[100]; while(cin>>a>>b) { struct node*root; root=Build_tree(a,b,strlen(a)); post_print(root); cout<<endl; } return 0; }