assets文件夹资源的访问
assets文件夹里面的文件都是保持原始的文件格式,需要用AssetManager以字节流的形式读取文件。
1. 先在Activity里面调用getAssets() 来获取AssetManager引用。
2. 再用AssetManager的open(String fileName, int accessMode) 方法则指定读取的文件以及访问模式就能得到输入流InputStream。
3. 然后就是用已经open file 的inputStream读取文件,读取完成后记得inputStream.close() 。
4.调用AssetManager.close() 关闭AssetManager。
需要注意的是,来自Resources和Assets 中的文件只可以读取而不能进行写的操作
以下为从Raw文件中读取:
代码
1 public String getFromRaw(){ 2 try { 3 InputStreamReader inputReader = new InputStreamReader( getResources().openRawResource(R.raw.test1)); 4 BufferedReader bufReader = new BufferedReader(inputReader); 5 String line=""; 6 String Result=""; 7 while((line = bufReader.readLine()) != null) 8 Result += line; 9 return Result; 10 } catch (Exception e) { 11 e.printStackTrace(); 12 } 13 }
以下为直接从assets读取
代码
1 public String getFromAssets(String fileName){ 2 try { 3 InputStreamReader inputReader = new InputStreamReader( getResources().getAssets().open(fileName) ); 4 BufferedReader bufReader = new BufferedReader(inputReader); 5 String line=""; 6 String Result=""; 7 while((line = bufReader.readLine()) != null) 8 Result += line; 9 return Result; 10 } catch (Exception e) { 11 e.printStackTrace(); 12 } 13 }
当然如果你要得到内存流的话也可以直接返回内存流!<div "="">接下来,我们新建一个工程文件,命名为AssetsDemo。
然后建立一个布局文件,如下,很简单,无需我多介绍,大家一看就明白。
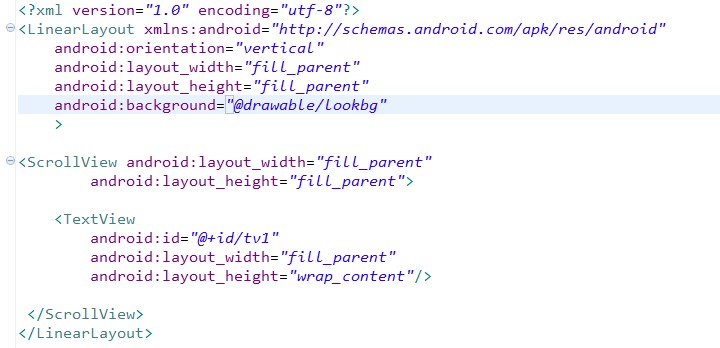
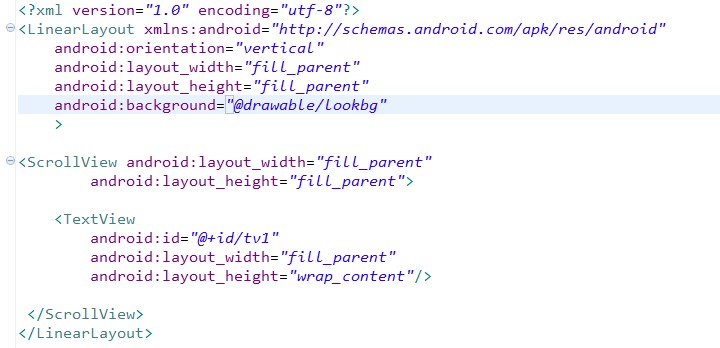
然后呢,我从网上找了段文字,存放在assets文件目录下,取名为health.txt 这就是今天我们要读取的文件啦。
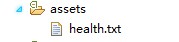
这个.txt文件,我们可以直接双击查看。如下所示。
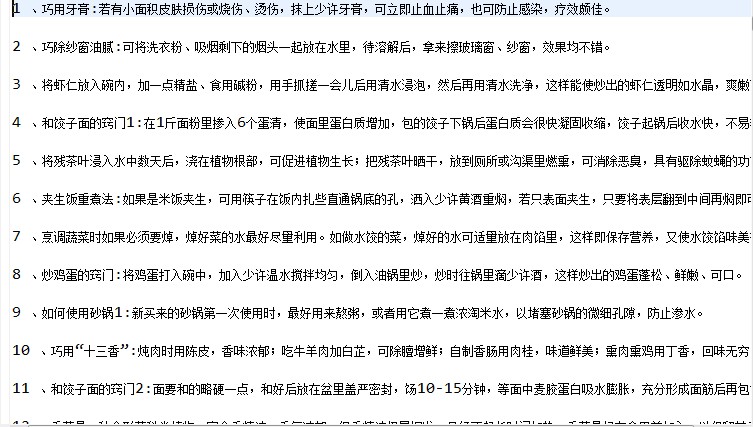
<div "="">
<div "="">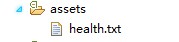
这个.txt文件,我们可以直接双击查看。如下所示。
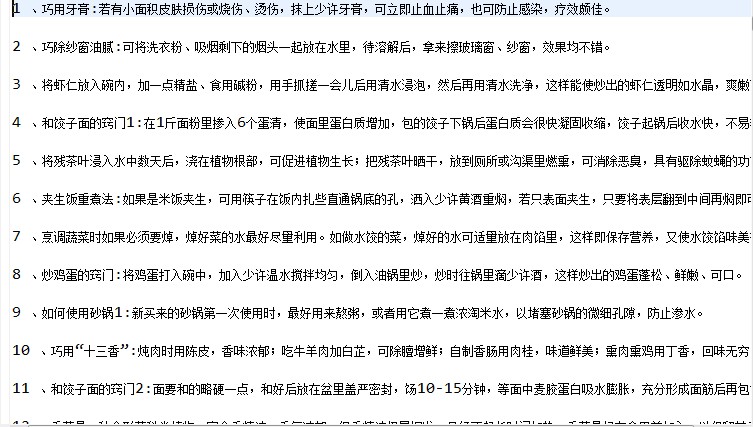
<div "="">接下来,就是今天的重头戏,Android读取文件的核心代码。就直接贴代码了。
<div "="">
1 package com.assets.cn; 2 import java.io.InputStream; 3 import org.apache.http.util.EncodingUtils; 4 import android.app.Activity; 5 import android.graphics.Color; 6 import android.os.Bundle; 7 import android.widget.TextView; 8 public class AssetsDemoActivity extends Activity { 9 public static final String ENCODING = "UTF-8"; 10 TextView tv1; 11 @Override 12 public void onCreate(Bundle savedInstanceState) { 13 super.onCreate(savedInstanceState); 14 setContentView(R.layout.main); 15 16 tv1 = (TextView)findViewById(R.id.tv1); 17 tv1.setTextColor(Color.BLACK); 18 tv1.setTextSize(25.0f); 19 tv1.setText(getFromAssets("health.txt")); 20 } 21 22 //从assets 文件夹中获取文件并读取数据 23 public String getFromAssets(String fileName){ 24 String result = ""; 25 try { 26 InputStream in = getResources().getAssets().open(fileName); 27 //获取文件的字节数 28 int lenght = in.available(); 29 //创建byte数组 30 byte[] buffer = new byte[lenght]; 31 //将文件中的数据读到byte数组中 32 in.read(buffer); 33 result = EncodingUtils.getString(buffer, ENCODING); 34 } catch (Exception e) { 35 e.printStackTrace(); 36 } 37 return result; 38 } 39 }
<div "="">这里是mainfest文件。<div "="">
1 <?xml version="1.0" encoding="utf-8"?> 2 <manifest xmlns:android="http://schemas.android.com/apk/res/android" 3 package="com.assets.cn" 4 android:versionCode="1" 5 android:versionName="1.0"> 6 <uses-sdk android:minSdkVersion="8" /> 7 8 <application android:icon="@drawable/icon" android:label="@string/app_name"> 9 <activity android:name=".AssetsDemoActivity" 10 android:label="@string/app_name"> 11 <intent-filter> 12 <action android:name="android.intent.action.MAIN" /> 13 <category android:name="android.intent.category.LAUNCHER" /> 14 </intent-filter> 15 </activity> 16 17 </application> 18 </manifest>
<div "="">最后,我们运行一下程序。