一针见血的学习网址:
https://www.bilibili.com/video/av62405790?from=search&seid=15110123406808421495
Django 框架
关于网站框架的MVC结构
Django框架的MVC
Django框架的MVC流程
Django框架创建流程
Django框架目录的简单介绍
今天需要完成的任务
几个命令入门期间比较重要
命令行创建环境工程及应用
html中form表单
<form method="POST" action="/cal">
第一个数字<input type="number" name="valueA">
+
第二个数字<input type="number" name="valueB">
<input type="submit" value="提交计算">//submit点击提交按钮后页面会跳转到url对应名字为/cal中,会进入对应方法views.Cal中
用Pycharm中自带的数据库Sqlite
Download相关未下载内容,到当前项目中选择dbsqlite3文件,test测试连接是否可以跑通,successful 后进行数据库迁移操作:
1.创建迁移
(venv) F:scriptpy_scriptsmainproject>python manage.py makemigrations
2.执行迁移操作
(venv) F:scriptpy_scriptsmainproject>python manage.py migrate
刷新右侧数据库,关注“FirstWEB”表及其中字段
一个易错点
-----------------------------------------------------------------------------------------------------------------------------------------------------------
代码具体内容:
代码台式机路径:
mainproject
settings.py
1 """ 2 Django settings for mainproject project. 3 4 Generated by 'django-admin startproject' using Django 2.2.11. 5 6 For more information on this file, see 7 https://docs.djangoproject.com/en/2.2/topics/settings/ 8 9 For the full list of settings and their values, see 10 https://docs.djangoproject.com/en/2.2/ref/settings/ 11 """ 12 13 import os 14 15 # Build paths inside the project like this: os.path.join(BASE_DIR, ...) 16 BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__))) 17 18 19 # Quick-start development settings - unsuitable for production 20 # See https://docs.djangoproject.com/en/2.2/howto/deployment/checklist/ 21 22 # SECURITY WARNING: keep the secret key used in production secret! 23 SECRET_KEY = 'ho#q8-%k2zlqb($n&s^=lfa5!de66vb0kimp0k@(8*j8mj^eg^' 24 25 # SECURITY WARNING: don't run with debug turned on in production! 26 DEBUG = True 27 28 ALLOWED_HOSTS = [] 29 30 31 # Application definition 32 33 INSTALLED_APPS = [ 34 'django.contrib.admin', 35 'django.contrib.auth', 36 'django.contrib.contenttypes', 37 'django.contrib.sessions', 38 'django.contrib.messages', 39 'django.contrib.staticfiles', 40 'firstWEB', 41 ] 42 43 MIDDLEWARE = [ 44 'django.middleware.security.SecurityMiddleware', 45 'django.contrib.sessions.middleware.SessionMiddleware', 46 'django.middleware.common.CommonMiddleware', 47 'django.middleware.csrf.CsrfViewMiddleware', 48 'django.contrib.auth.middleware.AuthenticationMiddleware', 49 'django.contrib.messages.middleware.MessageMiddleware', 50 'django.middleware.clickjacking.XFrameOptionsMiddleware', 51 ] 52 53 ROOT_URLCONF = 'mainproject.urls' 54 55 TEMPLATES = [ 56 { 57 'BACKEND': 'django.template.backends.django.DjangoTemplates', 58 'DIRS': [os.path.join(BASE_DIR, 'templates')] 59 , 60 'APP_DIRS': True, 61 'OPTIONS': { 62 'context_processors': [ 63 'django.template.context_processors.debug', 64 'django.template.context_processors.request', 65 'django.contrib.auth.context_processors.auth', 66 'django.contrib.messages.context_processors.messages', 67 ], 68 }, 69 }, 70 ] 71 72 WSGI_APPLICATION = 'mainproject.wsgi.application' 73 74 75 # Database 76 # https://docs.djangoproject.com/en/2.2/ref/settings/#databases 77 78 DATABASES = { 79 'default': { 80 'ENGINE': 'django.db.backends.sqlite3', 81 'NAME': os.path.join(BASE_DIR, 'db.sqlite3'), 82 } 83 } 84 85 86 # Password validation 87 # https://docs.djangoproject.com/en/2.2/ref/settings/#auth-password-validators 88 89 AUTH_PASSWORD_VALIDATORS = [ 90 { 91 'NAME': 'django.contrib.auth.password_validation.UserAttributeSimilarityValidator', 92 }, 93 { 94 'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator', 95 }, 96 { 97 'NAME': 'django.contrib.auth.password_validation.CommonPasswordValidator', 98 }, 99 { 100 'NAME': 'django.contrib.auth.password_validation.NumericPasswordValidator', 101 }, 102 ] 103 104 105 # Internationalization 106 # https://docs.djangoproject.com/en/2.2/topics/i18n/ 107 108 LANGUAGE_CODE = 'zh-Hans' 109 110 TIME_ZONE = 'Asia/Shanghai' 111 112 USE_I18N = True 113 114 USE_L10N = True 115 116 USE_TZ = True 117 118 119 # Static files (CSS, JavaScript, Images) 120 # https://docs.djangoproject.com/en/2.2/howto/static-files/ 121 122 STATIC_URL = '/static/'
urls.py

1 from django.contrib import admin 2 from django.conf.urls import url 3 from firstWEB import views 4 urlpatterns = { 5 url('admin/', admin.site.urls), 6 url(r'^index/', views.index), 7 url(r'^calpage/', views.CalPage), 8 url(r'^cal', views.Cal), 9 url(r'^list', views.CalList), 10 url(r'^del', views.DelData) 11 }
应用firstWEB
models.py
1 from django.db import models 2 3 # Create your models here.models文件是对表的描述 4 5 class cal(models.Model): 6 value_a = models.CharField(max_length=10) 7 value_b = models.CharField(max_length=10) 8 result = models.CharField(max_length=10)
views.py
1 from __future__ import unicode_literals 2 from django.shortcuts import render 3 from django.http import HttpResponse 4 from firstWEB.models import cal 5 6 7 # Create your views here. 8 9 def index(request): 10 return render(request, 'index.html') 11 12 13 def CalPage(request): 14 return render(request, 'cal.html') 15 16 17 def Cal(request): 18 if request.POST:#如果是post访问才进入,避免如果本来是post才能访问到的html网址,通过get url链接访问会报错。 19 value_a = request.POST['valueA'] 20 value_b = request.POST['valueB'] 21 print(value_a, value_b) 22 result = int(value_a) + int(value_b) 23 # 把各个字段数据存储到数据库中 24 cal.objects.create(value_a=value_a, value_b=value_b, result=result) 25 return render(request, 'result.html', context={'data': result}) 26 else: 27 return HttpResponse('Please visit us with POST')#如果非post方式访问(可能是直接输入链接进行访问。如果是post访问时上一个页面post方式提交信息到此html页面的方式),报错。 28
直接访问http://127.0.0.1:8000/cal报错信息现象
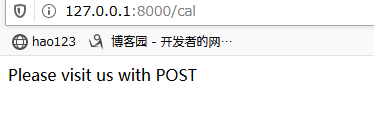
29 def CalList(request): 30 data = cal.objects.all() 31 # for data in data: 32 # print(data.value_a,data.value_b,data.result) 33 return render(request, 'list.html', context={'data': data}) 34 35 36 def DelData(request): 37 cal.objects.all().delete() 38 return HttpResponse('data Deleted')
templates--
index.html
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>My first Web from Django</title> 6 </head> 7 <body> 8 <h1 style="color:red">My first Django website</h1> 9 </body> 10 </html>
cal.html
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>计算页面</title> 6 </head> 7 <body> 8 <form method="POST" action="/cal"> 9 {% csrf_token %} 10 第一个数字<input type="number" name="valueA"> 11 + 12 第二个数字<input type="number" name="valueB"> 13 <input type="submit" value="提交计算"> 14 </form> 15 </body> 16 </html>
点击提交
result.html
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>结果页面</title> 6 </head> 7 <body> 8 <h1>计算结果是:</h1><h2>{{ data }}</h2> 9 </body> 10 </html>
list.html
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Cal LIST</title> 6 </head> 7 <body> 8 <table border="1"> 9 <thead> 10 <tr> 11 <th>valueA</th><th>valueB</th><th>result</th> 12 </tr> 13 </thead> 14 15 {% for data in data %} 16 <tr> 17 <td>{{ data.value_a }}</td><td>{{ data.value_b }}</td><td>{{ data.result }}</td> 18 </tr> 19 {% endfor %} 20 21 </table> 22 <form action="del" method="POST"> 23 {% csrf_token %} 24 <input type="submit" value="清库"> 25 </form> 26 </body> 27 </html>
访问:http://127.0.0.1:8000/list
点击“清库”
返回上一页,重新刷新浏览器