防止用户多次提交
ajax天性的异步,后台操作的特性使得程序在执行的时候,无法使用户了解当前正在等待的情况,所以用户往往会多次提交,为了避免由于重复提交造成的服务器损失以及用户体验降低,就有必要阻止用户多次提交同一个操作。
在ajax 中可以使用PageRequestManager对应所暴露出的 异步回送各个阶段的事件来判断是否重复提交,PageRequestManager正常时候的事件触发顺序如下:
1.用户点击UpdatePanel中的按钮,触发一个异步的操作
2.PageRequestManager对象触发initializeRequest事件。
3.PageRequestManager对象触发beginRequest事件
4.请求发送到服务器
5.客户端成功收到服务的相应。
6.PageRequestManager对象触发pageLoading事件。
7PageRequestManager对象触发pageLoaded事件。
8.Application对象触发load事件
9PageRequestManager对象触发endRequest时间。
示例:
aspx:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Multirequest.aspx.cs" Inherits="Multirequest" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>无标题页</title>
<script type="text/javascript">
var prm;
var btnsubmitid = '<%=Button1.ClientID %>'
function pageLoad() {
prm = Sys.WebForms.PageRequestManager.getInstance();
prm.add_initializeRequest(onInitializeRequest);
prm.add_pageLoaded(onPageLoaded);
}
function onInitializeRequest(sender,args)
{
if(prm.get_isInAsyncPostBack() && args.get_postBackElement().id==btnsubmitid)
{
args.set_cancel(true);
showWaitingInfo(true);
setTimeout("showWaitingInfo(false)",1500);
}
}
function showWaitingInfo(visible)
{
var id = $get("messagePanel");
id.style.display=visible?"block":"none";
}
function onPageLoaded(sender,args)
{
showWaitingInfo(false);
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ScriptManager ID="ScriptManager1" runat="server" />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<asp:Label ID="Label1" runat="server" Text="Name"></asp:Label> <asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
<br />
Msg:<asp:TextBox ID="TextBox2" runat="server"></asp:TextBox>
<asp:Button ID="Button1" runat="server" onclick="Button1_Click" Text="OK"
Width="55px" />
<br />
<br />
<asp:Label ID="Label2" runat="server" Text="Result:"></asp:Label>
<asp:Label ID="lblresult" runat="server"></asp:Label>
<div id="messagePanel" style="display:none;">
Still Processing,Please be patient.
</div>
</ContentTemplate>
</asp:UpdatePanel>
<br />
</div>
</form>
</body>
</html>
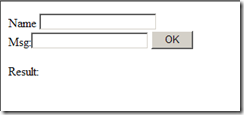
cs:
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
this.lblresult.Text = this.TextBox1.Text + " &&&&" + this.TextBox2.Text + "TIME:" + System.DateTime.Now.ToLocalTime();
System.Threading.Thread.Sleep(3000);
}
说明:
function pageLoad() {
//获取PageRequestManager对象
prm = Sys.WebForms.PageRequestManager.getInstance();
//设置事件触发
prm.add_initializeRequest(onInitializeRequest);
prm.add_pageLoaded(onPageLoaded);
}
function onInitializeRequest(sender,args)
{
//判断是否正在异步回送中,并且引发两次操作回送的按钮时同一个。
if(prm.get_isInAsyncPostBack() && args.get_postBackElement().id==btnsubmitid)
{
//取消本次的异步
args.set_cancel(true);
//显示信息提示用户
showWaitingInfo(true);
//1.5s后隐藏提示信息
setTimeout("showWaitingInfo(false)",1500);
}
}
停止正在执行的异步操作
可以使用PageRequestManager对象的abortPostBack()方法停止当前正在执行的异步操作。
相关code:
function btnCancel_onclick()
{
if(prm.get_isInAsyncPostBack())
{prm.abortPostBack();
}}
在执行异步回送时给用户提示
在PageRequestManager的onBeginRequest的时候,判断PageRequestManager的get_postBackElement().id是否为当前提交的按钮,如果是,则显示waiting的信息,并禁止使用页面等。
当onPageLoaded时,恢复页面。
在异步执行时的异常处理
处理PageRequestManager的onEndRequest事件,例如:
function onEndRequest(sender,args)
{
var error = args.get_error();
if(error)
{//显示异常。
$get(“messagePanel”).innerHTML = error.message';
//告知asp.net ajax Client framework error 已经处理。
args.set_errorHandled(true);
}
}