字节输出流OutputStream
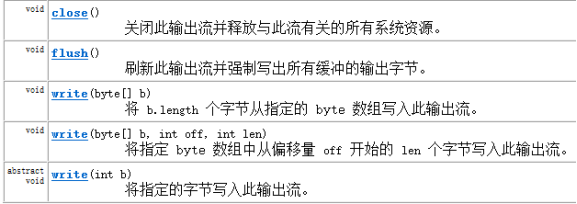
FileOutputStream类
构造方法

import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo01 {
public static void main(String[] args) throws IOException {
method1();
method2();
}
public static void method1() throws IOException{
//创建字节输出流对象
//如果该文件有则覆盖,如果没有则创建
FileOutputStream fos=new FileOutputStream("E:\java\demo.txt");
byte[] bytes={97,98,99,100};
fos.write(100);
fos.write(bytes, 1, 1);//数组存
fos.write("你好么".getBytes());//直接扔
//释放资源
fos.close();
}
//续写
public static void method2() throws IOException{
FileOutputStream fos=new FileOutputStream("E:\java\b.txt",true);
fos.write("
你好么".getBytes());
//释放资源
fos.close();
}
}
IO异常的处理
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo02 {
public static void main(String[] args) {
//创建字节输出流对象
FileOutputStream fos=null;
try{
fos=new FileOutputStream("E:\java");
fos.write("你好".getBytes());
}catch(IOException e){
e.printStackTrace();
//抛出运行异常终止程序
throw new RuntimeException();
}finally{
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
字节输入流InputStream
FileInputStream类
构造方法

方法

import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo03 {
public static void main(String[] args) throws IOException {
method1();
method2();
method3();
method4();
method5();
}
public static void method1() throws IOException{
//明确数据源
FileInputStream fos=new FileInputStream("E:\java\demo.txt");
//从文件中读取一个字节
int len1=fos.read();
System.out.println((char)len1);
len1=fos.read();
System.out.println((char)len1);
len1=fos.read();
System.out.println(len1);
fos.close();
}
//从文件读取内容
public static void method2() throws IOException{
FileInputStream fos=new FileInputStream("E:\java\demo.txt");
int len=0;
while((len=fos.read())!=-1){
System.out.println((char)len);
}
fos.close();
}
读取数据read(byte[])方法

//数组读取
public static void method3() throws IOException{
FileInputStream fos=new FileInputStream("E:\java\demo.txt");
byte[] bytes=new byte[2];
int len=fos.read(bytes);
System.out.println(new String(bytes));
System.out.println(len);
len=fos.read(bytes);
System.out.println(new String(bytes));
System.out.println(len);
fos.close();
}
public static void method4() throws IOException{
FileInputStream fos=new FileInputStream("E:\java\demo.txt");
byte[] bytes=new byte[2];
int len=0;
while((len=fos.read(bytes))!=-1){
System.out.println(new String(bytes,0,len));//不要后边多出来的 解码
}
}
复制文件
//文件复制
public static void method5() throws IOException{
FileInputStream fis=newFileInputStream("E:\java\demo.txt");
FileOutputStream fos=newFileOutputStream("E:\java\a.txt");
int len=0;
while((len=fis.read())!=-1){
fos.write(len);
}
fis.close();
fos.close();
}
}