<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
<p>{{ message }}</p>
<table border="" cellspacing="" cellpadding="">
<tr>
<th>书籍名称</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</tr>
<tr v-for="(book, index) in books">
<td>{{book.name}}</td>
<td>{{book.price | showPrice}}</td>
<td>
<button type="button" @click="subClick(index)" v-bind:disabled="book.num <= 1">-</button>
{{book.num}}
<button type=" button" @click="addClick(index)">+</button>
</td>
<td>
<button type="button" @click="delClick(index)">移除</button>
</td>
</tr>
</table>
<p>总价格:{{totPrice | showPrice}}</p>
</div>
<script>
const app = new Vue({
el: '#app',
data: {
message: 'Hello Vue.js!',
books: [{
name: 'HTML/HTML5基础',
price: 15.5,
num: 1
},
{
name: '高健壮性CSS',
price: 16.5,
num: 1
},
{
name: '深入学习JS',
price: 17.5,
num: 1
},
]
},
methods: {
subClick(index) {
console.log("subClick " + index);
this.books[index].num--;
},
addClick(index) {
console.log("addClick " + index);
this.books[index].num++;
},
delClick(index) {
console.log("delClick " + index);
this.books.splice(index, 1);
},
},
// 过滤器
filters: {
showPrice(price) {
return '¥' + price.toFixed(2);
},
},
computed: {
totPrice() {
let total = 0;
for (let book of this.books) {
total += book.price * book.num;
}
return total;
}
}
})
</script>
</body>
</html>
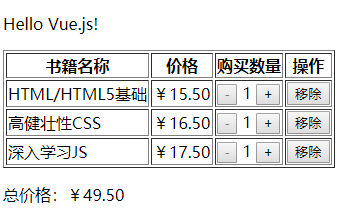