LeetCode Weekly Contest 18B
496. Next Greater Element I
- User Accepted: 680
- User Tried: 719
- Total Accepted: 691
- Total Submissions: 1128
- Difficulty: Easy
You are given two arrays (without duplicates) nums1
and nums2
where nums1
’s elements are subset of nums2
. Find all the next greater numbers for nums1
's elements in the corresponding places of nums2
.
The Next Greater Number of a number x in nums1
is the first greater number to its right in nums2
. If it does not exist, output -1 for this number.
Example 1:
Input: nums1 = [4,1,2], nums2 = [1,3,4,2]. Output: [-1,3,-1] Explanation: For number 4 in the first array, you cannot find the next greater number for it in the second array, so output -1. For number 1 in the first array, the next greater number for it in the second array is 3. For number 2 in the first array, there is no next greater number for it in the second array, so output -1.
Example 2:
Input: nums1 = [2,4], nums2 = [1,2,3,4]. Output: [3,-1] Explanation: For number 2 in the first array, the next greater number for it in the second array is 3. For number 4 in the first array, there is no next greater number for it in the second array, so output -1.
Note:
- All elements in
nums1
andnums2
are unique. - The length of both
nums1
andnums2
would not exceed 1000.
因为数据量小,所以 采用直接法。
class Solution { public: vector<int> nextGreaterElement(vector<int>& findNums, vector<int>& nums) { vector<int> ans; map<int, int> mp; int max_val; for(int i=nums.size()-1; i>=0; --i){ if(i == nums.size()-1){ mp[nums[i]] = -1; max_val = nums[i]; }else{ if(nums[i] >= max_val){ mp[nums[i]] = -1; max_val = nums[i]; }else{ for(int j=i+1; j<nums.size(); ++j){ if(nums[j] > nums[i]){ mp[nums[i]] = nums[j]; break; } } } } } for(int i=0; i<findNums.size(); ++i){ ans.push_back( mp[findNums[i]] ); } return ans; } };
506. Relative Ranks
- User Accepted: 654
- User Tried: 683
- Total Accepted: 696
- Total Submissions: 1210
- Difficulty: Easy
Given scores of N athletes, find their relative ranks and the people with the top three highest scores, who will be awarded medals: "Gold Medal", "Silver Medal" and "Bronze Medal".
Example 1:
Input: [5, 4, 3, 2, 1] Output: ["Gold Medal", "Silver Medal", "Bronze Medal", "4", "5"] Explanation: The first three athletes got the top three highest scores, so they got "Gold Medal", "Silver Medal" and "Bronze Medal".
For the left two athletes, you just need to output their relative ranks according to their scores.
Note:
- N is a positive integer and won't exceed 10,000.
- All the scores of athletes are guaranteed to be unique.
代码写得有点乱,逻辑也不好
class Solution { public: map<int, string> Generate(int n){ map<int, string> mp; mp[1] = "Gold Medal"; mp[2]="Silver Medal"; mp[3]="Bronze Medal"; for(int i=4; i<=n; ++i){ mp[i] = to_string(i); } return mp; } vector<string> findRelativeRanks(vector<int>& nums) { map<int, int> idx, idx2; int len = nums.size(); map<int, string> mp; mp = Generate(len); for(int i=0; i<len; ++i){ idx[ i+1 ] = nums[i]; } sort(nums.begin(), nums.end()); for(int i=0; i<len; ++i){ idx2[ nums[i] ] = len - i; } vector<string> ans; for(int i=1; i<=len; ++i){ ans.push_back( mp[ idx2[ idx[i] ] ] ); } return ans; } };
503. Next Greater Element II
- User Accepted: 505
- User Tried: 620
- Total Accepted: 514
- Total Submissions: 1256
- Difficulty: Medium
Given a circular array (the next element of the last element is the first element of the array), print the Next Greater Number for every element. The Next Greater Number of a number x is the first greater number to its traversing-order next in the array, which means you could search circularly to find its next greater number. If it doesn't exist, output -1 for this number.
Example 1:
Input: [1,2,1] Output: [2,-1,2] Explanation: The first 1's next greater number is 2;
The number 2 can't find next greater number;
The second 1's next greater number needs to search circularly, which is also 2.
Note: The length of given array won't exceed 10000.
得到了第一题的网友答案的提示,利用 stack + map 来完成
class Solution { public: vector<int> nextGreaterElements(vector<int>& nums) { vector<int> ans; int len = nums.size(); if(len == 0){ return ans; } int max_val = -0x3f3f3f3f; for(int i=0; i<len; ++i){ if(max_val < nums[i]){ max_val = nums[i]; } ans.push_back(-1); } stack<int> st; stack<int> idx; for(int i=0; i<len; ++i){ while(st.size()>0 && st.top() < nums[i]){ ans[ idx.top() ] = nums[i]; idx.pop(); st.pop(); } if(nums[i] == max_val){ ans[ i ] = -1; }else{ st.push( nums[i] ); idx.push(i); } } for(int i=0; i<len && nums[i] != max_val; ++i){ while( st.size()>0 && st.top() < nums[i]){ ans[ idx.top() ] = nums[i]; idx.pop(); st.pop(); } } while( st.size()>0 ){ ans[ idx.top() ] = max_val; idx.pop(); st.pop(); } return ans; } };
498. Diagonal Traverse
- User Accepted: 355
- User Tried: 418
- Total Accepted: 372
- Total Submissions: 845
- Difficulty: Medium
Given a matrix of M x N elements (M rows, N columns), return all elements of the matrix in diagonal order as shown in the below image.
Example:
Input: [ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ] Output: [1,2,4,7,5,3,6,8,9] Explanation:
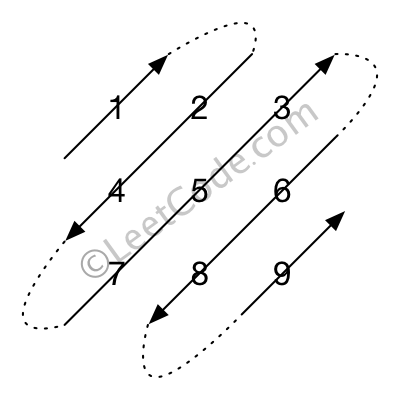
Note:
- The total number of elements of the given matrix will not exceed 10,000.