FreeMarker
JSP、Thymeleaf、FreeMarker
等模板引擎的区别 参考 https://www.cnblogs.com/ywb-articles/p/10627398.html
基础入门
环境搭建测试
搭建工程
- 导入pom依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
- 编写application.yml配置文件
server:
port: 8088
spring:
application:
name: freemarker-demo
freemarker:
cache: false
template-loader-path: classpath:/templates/
settings:
template_update_delay: 0 #检查模板更新延迟时间,设置为 0 表示立即检查,如果时间大于 0 会有缓存不方便进行模板测试
- 编写controller方法
@Controller
@RequestMapping("freemarker")
public class TestController {
@GetMapping("test1")
public String test01(ModelMap map){
map.addAttribute("name","zhangsan");
return "test01";
}
-----------
- 编写html页面
- 这里可以用
html
页面 因为暂时不会冲突 后面都将用ftlh
为后缀的格式 但是必须在配置文件中指定后缀 为.html
suffix: .html
- 这里可以用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>hello ${name}!</div>
</body>
</html>
- 测试
for的使用
- 编写一个实体类
student
@Data
@ToString
public class Student {
private String name;//姓名
private int age;//年龄
private Date birthday;//生日
private Float money;//钱包
private List<Student> friends;//朋友列表
private Student bestFriend;//最好的朋友
}
- 编写controller类
@GetMapping("test2")
public String test02(ModelMap map){
ArrayList<Student> students = new ArrayList<>();
Student student = new Student();
student.setAge(12);
student.setName("zhangsan");
student.setBirthday(new Date());
Student student1 = new Student();
student1.setAge(15);
student1.setName("lisi");
student1.setMoney(12.22F);
students.add(student);
students.add(student1);
map.addAttribute("list",students);
return "test02";
}
- 编写测试页面
test02.ftlh
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<table>
<tr>
<td>序号</td>
<td>姓名</td>
<td>年龄</td>
<td>金钱</td>
</tr>
<#list list as stu>
<tr>
<th>${stu_index+1}</th>
<th>${stu.name}</th>
<th>${stu.age}</th>
<#-- 当数值不存在的时候设置默认值 格式为 ${data!defaultData} -->
<th>${stu.money!12.2}</th>
<th>${kkk!'nihoa'}</th>
</tr>
</#list>
</table>
</body>
</html>
当某个值为null时页面会报错所以这里可以设置默认值 方法为 ${data!defaultData}
map的遍历
编写后端数据
@GetMapping("test3")
public String test(ModelMap map){
HashMap<String, Student> hashMap = new HashMap<>();
Student student = new Student();
student.setAge(12);
student.setName("zhangsan");
student.setBirthday(new Date());
Student student1 = new Student();
student1.setAge(15);
student1.setName("lisi");
student1.setMoney(12.22F);
student1.setBirthday(new Date());
hashMap.put("student",student);
hashMap.put("students",student1);
map.put("map",hashMap);
return "test03";
}
编写前端数据
<body>
输出stu1的学生信息</br>
姓名: ${map['student'].name}
年龄: ${map['student'].age}
<table border="1">
<tr>
<td>序号</td>
<td>姓名</td>
<td>年龄</td>
<td>金钱</td>
<td>时间</td>
</tr>
<#list map?keys as k>
<tr>
<th>${k_index+1}</th>
<th>${map[k].name}</th>
<th>${map[k].age/}</th>
<th>${map[k].money!12.2}</th>
<th>${map[k].birthday?string(('yyyy-MM-dd HH:mm:ss'))}</th>
<th>${map[k].birthday?date}</th>
<th>${map[k].birthday?datetime}</th>
<th>${map[k].birthday?time}</th>
</tr>
</#list>
</table>
</body>
-
这里
map?keys
获取map所有的key值 -
注意这里 birthday后端传过来的是date类型 所以要声明类型 或者进行转换
-
有三种方法:
-
?string(('yyyy-MM-dd HH:mm:ss'))
将date数据转换为该类型 -
?date
-
?datetime
-
?time
-
If指令
编写if后端
@GetMapping("test04")
public String test04(ModelMap map){
ArrayList<Student> list = new ArrayList<>();
Student student = new Student();
student.setAge(12);
student.setName("zhangsan");
student.setBirthday(new Date());
Student student1 = new Student();
student1.setAge(15);
student1.setName("lisi");
student1.setMoney(12.22F);
student1.setBirthday(new Date());
list.add(student);
list.add(student1);
map.addAttribute("list",list);
return "test04";
}
前端代码
<table border="1" cellspacing="0">
<tr>
<td>序号</td>
<td>姓名</td>
<td>年龄</td>
<td>金钱</td>
<td>时间</td>
</tr>
<#list list as l>
<tr>
<th>${l_index}</th>
<th <#if l.name=='zhangsan'>style="color: red" </#if>>${l.name}</th>
<th>${l.age}</th>
<#-- 非空判断 第一种方法 -->
<th ><#if l.money??>${l.money}</#if></th>
<#-- 第二种方法 -->
<#-- <th >${l.money!12.22}</th>-->
<th>${l.birthday?date}</th>
</tr>
</#list>
</table>
其他指令
运算符
算数运算符 FreeMarker表达式中完全支持算术运算,FreeMarker支持的算术运算符包括:+, - , * , / , % 2
、逻辑运算符 逻辑运算符有如下几个: 逻辑与:&& 逻辑或:|| 逻辑非:! 逻辑运算符只能作用于布尔值,否则将产生错误 3 、比较运算符 表达式中支持的比较运算符有如下几个: 1 =或者==:判断两个值是否相等. 2 !=:判断两个值是否不等. 3 >或者gt:判断左边值是否大于右边值 4 >=或者gte:判断左边值是否大于等于右边值 5 <或者lt:判断左边值是否小于右边值 6 <=或者lte:判断左边值是否小于等于右边值
注意: =和!=可以用于字符串,数值和日期来比较是否相等,但=和!=两边必须是相同类型的值,否则会产生错误,而且FreeMarker
是精确比较,"x","x ","X"是不等的.其它的运行符可以作用于数字和日期,但不能作用于字符串,大部分的时候,使用gt等字母运算符代替>会有更好的效果,因为 FreeMarker会把>解释成FTL标签的结束字符,当然,也可以使用括号来避免这种情况,如:<#if (x>y)>
<#-- 判断l.name是否等于张三 并输出 true,false ?c是以原来的方式输出 -->
<th>${(l.name=="zhangsan")?c}</th>
<#-- 将输出格式化为 yes , no 这里在FreeMarker 2.3.23 版本中已经弃用 使用 ?then("yes","no")
如果布尔值是true, 这会返回第一个参数(此处是:"yes"), 否则返回第二个参数(此处是:"no")。 请注意,返回值总是一个字符串;如果参数是数字,那么首先会转换成字符串。 也请注意,两个参数是评估过的,不管只有一个会被用到; 如果参数不仅仅是文字的话,这也许会有负面影响。
-->
<th>${(l.name=="zhangsan")?string("yes","no")}</th>
空值处理
1 、判断某变量是否存在使用 “??” 用法为:variable??
,如果该变量存在,返回true,否则返回false
例:为防止stus为空报错可以加上判断如下:
2 、缺失变量默认值使用 “!” 使用!要以指定一个默认值,当变量为空时显示默认值。
例: ${name!''}表示如果name为空显示空字符串。
前面已经讲过 这里不在详说
**如果是嵌套对象则建议使用()括起来。 ** ${(stu.bestFriend.name)!''}
表示,如果stu或bestFriend或name为空默认显示空字符串。
内建函数
更多函数请参考 官网 http://freemarker.foofun.cn/ref_builtins_string.html
内建函数语法格式 变量+?+函数名称
获取集合大小 : size
<table border="1" cellspacing="0">
<tr>
<td>size</td>
</tr>
<#list list as l>
<tr>
<th>${list?size}</th>
</tr>
</#list>
</table>
- 后端任意传入一个集合 list
日期格式化 : date、dateTime
- 这里前面已经演示过
- 有三种方法:
- `?string(('yyyy-MM-dd HH:mm:ss'))`将date数据转换为该类型
- `?date`
- `?datetime`
- `?time`
内建函数 c
map.put("point",10234561)
point
是数字型,使用${point}
会显示这个数字的值,不并每三位使用逗号分隔。如果不想显示为每三位分隔的数字,可以使用c函数将数字型转成字符串输出 ${point?c}
将json字符串转换为对象
其中用到了 assign
标签,assign的作用是定义一个变量。
<#assign text="{'bank':'工商银行','account':'123456'}"/>
<#assign data=text?eval />
<table border="1" cellspacing="0">
<tr>
<td>size</td>
</tr>
<#list list as l>
<tr>
<#-- <th>${list?size}</th>-->
<th>${data.bank}</th>
<th>${data.account}</th>
</tr>
</#list>
</table>
页面静态化测试
使用freemarker将页面生成html文件
- 创建测试类和模板
- 编写测试类
@SpringBootTest
@RunWith(SpringRunner.class)
class FreemarkerdemoApplicationTests {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Test
public void test01() throws IOException, TemplateException {
Configuration configuration = new Configuration(Configuration.getVersion());
String path = this.getClass().getResource("/").getPath();
logger.info(() -> path);
configuration.setDirectoryForTemplateLoading(new File(path+"/templates/"));
Template template = configuration.getTemplate("test03.ftlh");
//获取数据模型
Map map = getMap();
String content = FreeMarkerTemplateUtils.processTemplateIntoString(template,map);
logger.info(()->content);
}
//获取数据模型
public Map getMap(){
HashMap<String, Student> hashMap = new HashMap<>();
Student student = new Student();
student.setAge(12);
student.setName("zhangsan");
student.setBirthday(new Date());
Student student1 = new Student();
student1.setAge(15);
student1.setName("lisi");
student1.setMoney(12.22F);
student1.setBirthday(new Date());
hashMap.put("student",student);
hashMap.put("students",student1);
HashMap<String, Map> map = new HashMap<>();
map.put("map",hashMap);
return map;
}
}
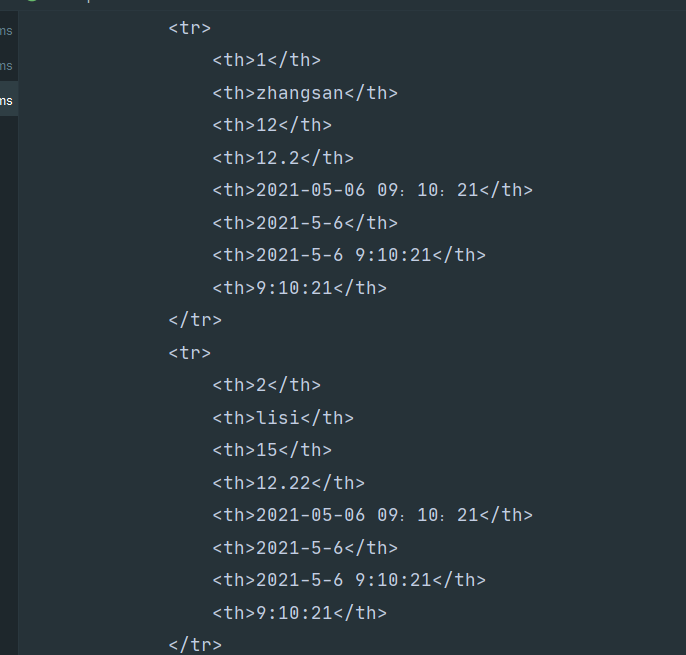
- 将输出内容导入到html文件中
@Test
public void test01() throws IOException, TemplateException {
Configuration configuration = new Configuration(Configuration.getVersion());
String path = this.getClass().getResource("/").getPath();
logger.info(() -> path);
configuration.setDirectoryForTemplateLoading(new File(path+"/templates/"));
Template template = configuration.getTemplate("test03.ftlh");
Map map = getMap();
String content = FreeMarkerTemplateUtils.processTemplateIntoString(template,map);
logger.info(()->content);
//两种方法
/*FileOutputStream fileOutputStream = new FileOutputStream(new File("D:/桌面/test1.html"));
fileOutputStream.write(content.getBytes());*/
/**
* 使用hutool工具类
* <dependency>
* <groupId>cn.hutool</groupId>
* <artifactId>hutool-all</artifactId>
* <version>5.6.3</version>
* </dependency>
*/
ByteArrayInputStream inputStream = IoUtil.toStream(content.getBytes());
BufferedOutputStream outputStream = FileUtil.getOutputStream(new File("D:/桌面/test1.html"));
IoUtil.copy(inputStream,outputStream);
inputStream.close();
outputStream.close();
}
使用模板字符串静态化
- 后续一般采用此方式实现静态化
/**
* 基于模板字符串生成模板文件
*/
@Test
public void test02() throws IOException, TemplateException {
Configuration configuration = new Configuration(Configuration.getVersion());
String templateString="" +
"<html>
" +
" <head></head>
" +
" <body>
" +
" 名称:${name}
" +
" </body>
" +
"</html>";
StringTemplateLoader templateLoader = new StringTemplateLoader();
templateLoader.putTemplate("template",templateString);
configuration.setTemplateLoader(templateLoader);
// 设置数据模型
HashMap<String,String> map = new HashMap<>();
map.put("name","zgrey");
Template template = configuration.getTemplate("template", "utf-8");
String content = FreeMarkerTemplateUtils.processTemplateIntoString(template, map);
ByteArrayInputStream inputStream = IoUtil.toStream(content.getBytes());
BufferedOutputStream outputStream = FileUtil.getOutputStream(new File("D:/桌面/test1.html"));
IoUtil.copy(inputStream,outputStream);
inputStream.close();
outputStream.close();
}