//因为不是类中所有代码都要被线程执行, 为了区分哪些代码需要被执行
//java提供了Thread类中的run()方法用来包含那些被线程执行的代码
public class SimpleThread extends Thread {
public SimpleThread(String name) { // 参数为线程名称
setName(name);
}
public void run() { // 覆盖run()方法
int i = 0;
while (i++ < 5) { // 循环5次
try {
System.out.println(getName() + "执行步骤" + i);
Thread.sleep(1000); // 休眠1秒
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
SimpleThread thread1 = new SimpleThread("线程1"); // 创建线程1
SimpleThread thread2 = new SimpleThread("线程2"); // 创建线程2
thread1.start(); // 启动线程1
thread2.start(); // 启动线程2
}
}
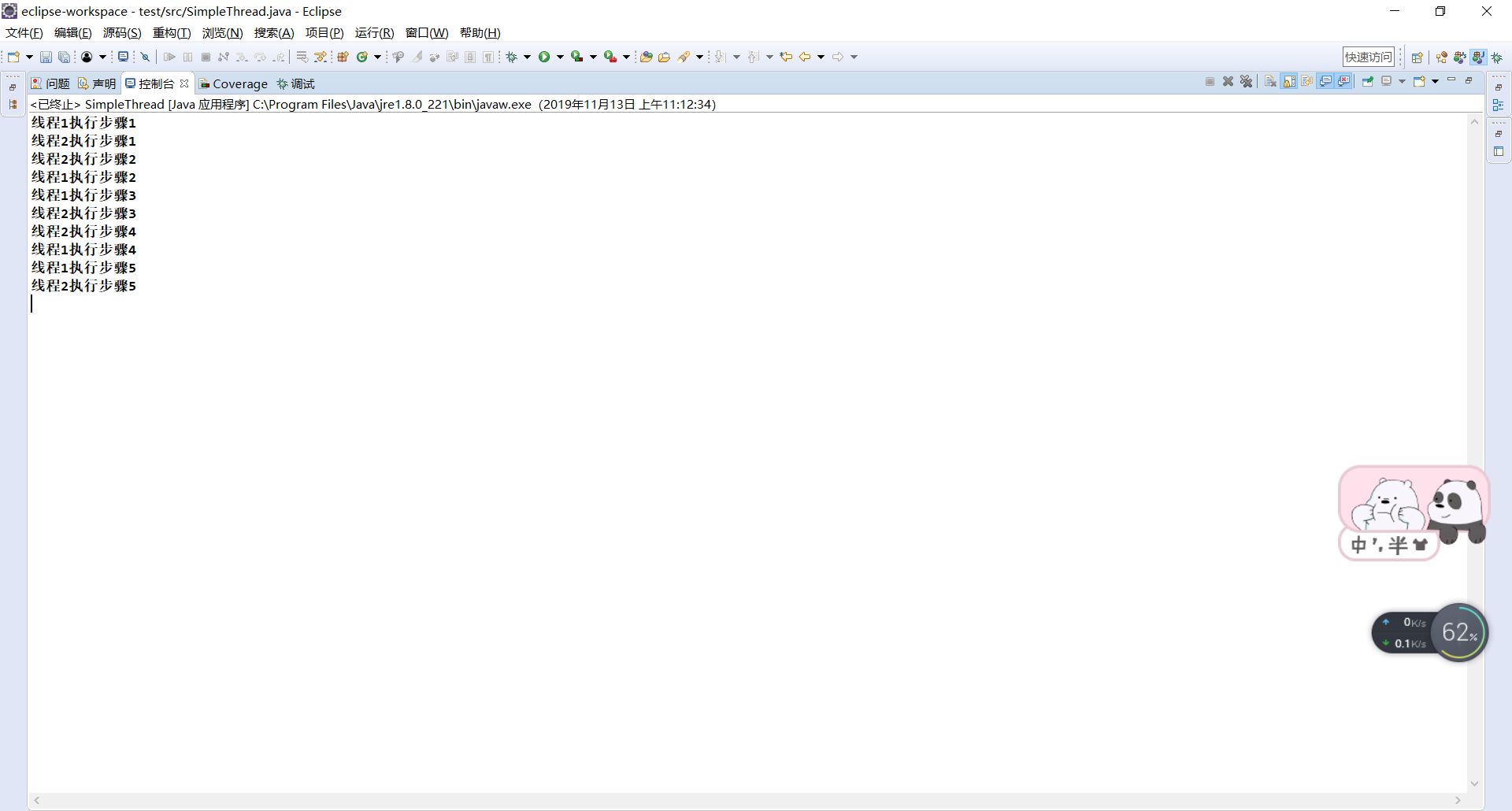
/*
* Runnable接口中只有一个run()方法,它非Thread类子类的类提供的一种激活方式。一个类实现Runnable接口后,并不代表
* 该类是一个“线程”类,不能直接运行,必须通过Thread实例才能创建并运行线程。
*/
public class SimpleRunnable implements Runnable {
public void run() { // 覆盖run()方法
int i = 15;
while (i-- >= 1) { // 循环15次
try {
System.out.print("*");
Thread.sleep(500);
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
//通过Thread实例运行线程
Thread thread1 = new Thread(new SimpleRunnable(),"线程1"); // 创建线程1
thread1.start(); // 启动线程1
}
}
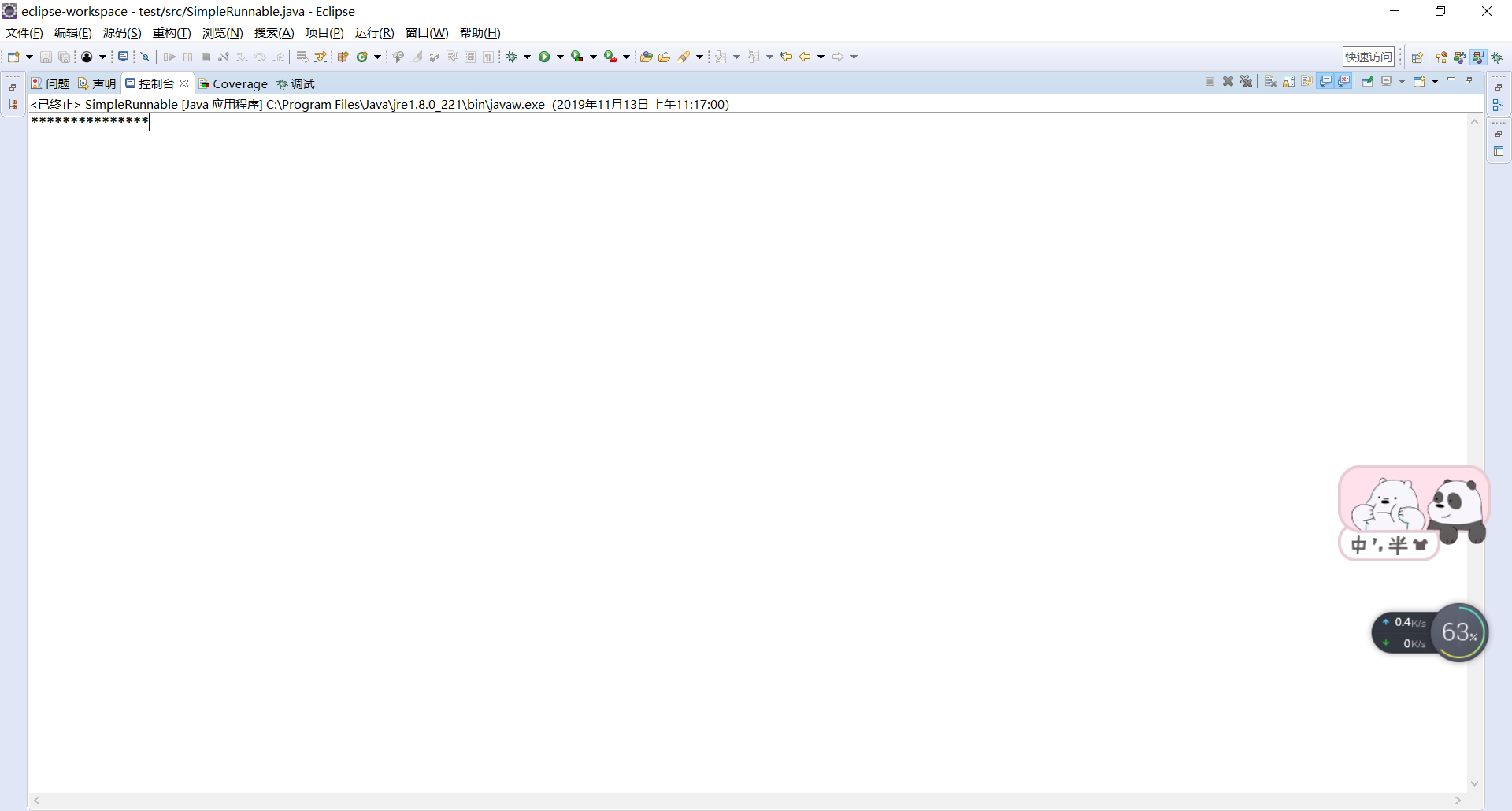
public class SyncThread extends Thread {
private char cha;
public SyncThread(char cha) { // 构造函数
this.cha = cha;
}
public void run() {
PrintClass.printch(cha); // 调用同步方法
System.out.println();
}
public static void main(String[] args) {
SyncThread t1 = new SyncThread('A'); // 创建线程A
SyncThread t2 = new SyncThread('B'); // 创建线程B
t1.start(); // 启动线程A
t2.start(); // 启动线程B
}
}
class PrintClass {
//同步方法的使用:在方法上加synchronized
public static synchronized void printch(char cha) { // 同步方法
for (int i = 0; i < 5; i++) {
try {
Thread.sleep(1000); // 打印一个字符休息1秒
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.print(cha);
}
}
}
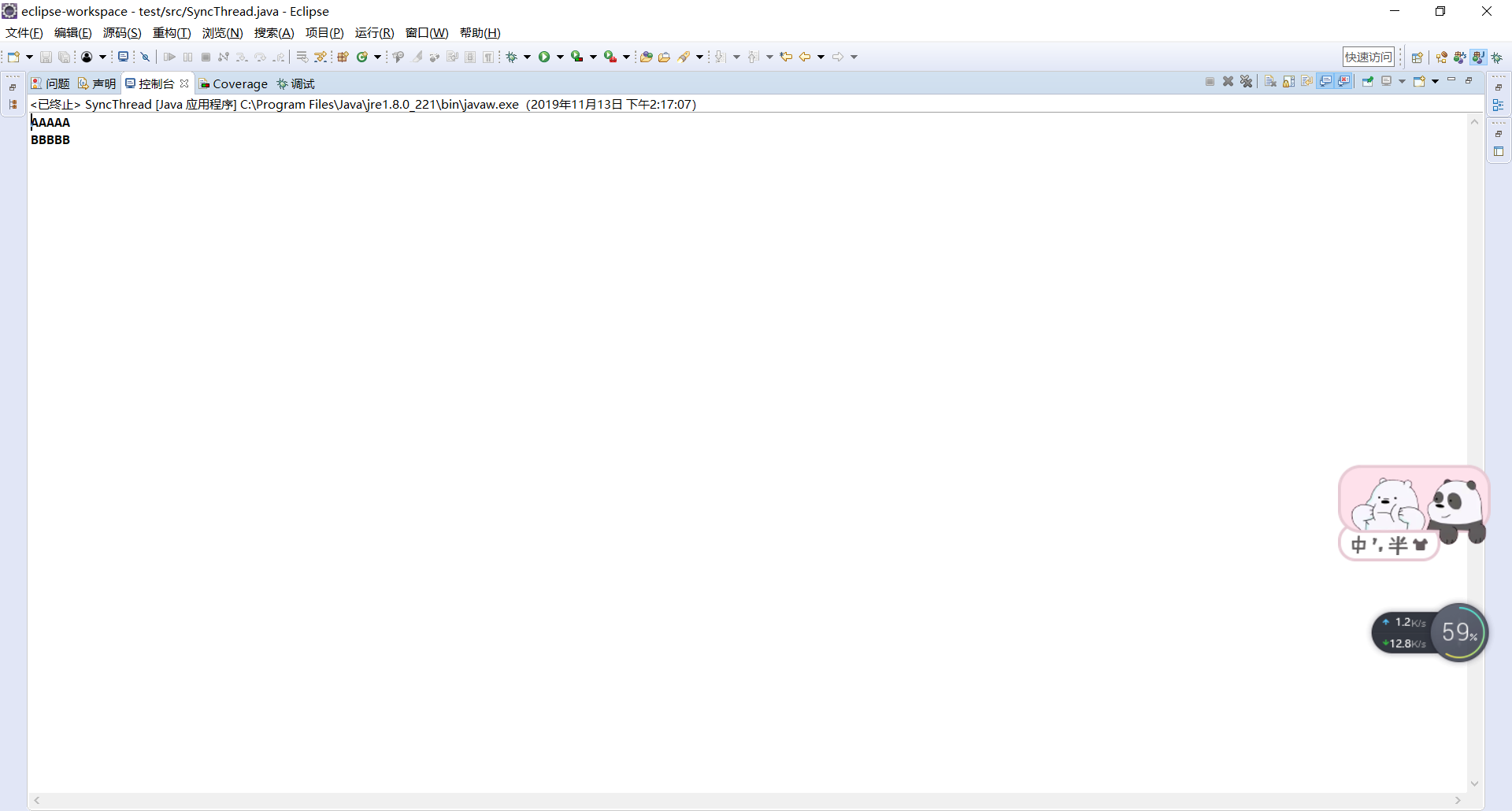
public class SyncThread extends Thread {
private String cha;
public SyncThread(String cha) { // 构造函数
this.cha = cha;
}
public void run() {
PrintClass.printch(cha); // 调用同步方法
}
public static void main(String[] args) {
SyncThread t1 = new SyncThread("线程A"); // 创建线程A
SyncThread t2 = new SyncThread("线程B"); // 创建线程B
t1.start(); // 启动线程A
t2.start(); // 启动线程B
}
}
class PrintClass {
static Object printer = new Object(); // 实例化Object对象
public static void printch(String cha) { // 同步方法
synchronized (printer) { // 同步代码块:同步代码块则在方法内部加
for (int i = 1; i < 5; i++) {
System.out.println(cha + " ");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
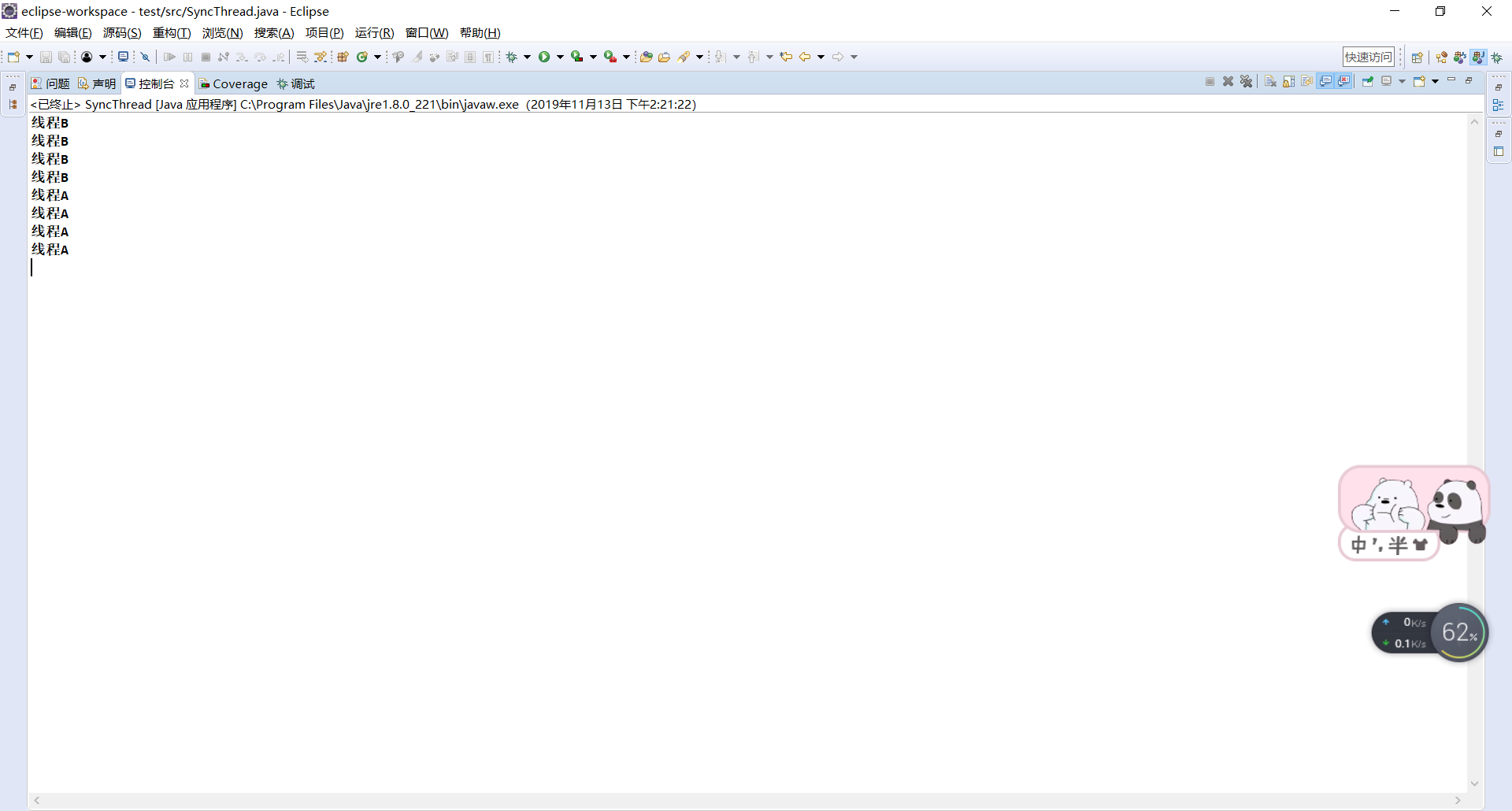
//进水
public class ThreadA extends Thread {
Water water;
public ThreadA(Water waterArg) {
water = waterArg;
}
public void run() {
System.out.println("开始进水.....");
for (int i = 1; i <= 5; i++) { // 循环5次
try {
Thread.sleep(1000); // 休眠1秒,模拟1分钟的时间
System.out.println(i + "分钟");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
water.setWater(true); // 设置水塘有水状态
System.out.println("进水完毕,水塘水满。");
synchronized (water) {
water.notify(); // 线程调用notify()方法
}
}
}
/*
notify()方法表示,
当前的线程已经放弃对资源的占有,
通知等待的线程来获得对资源的占有权,
但是只有一个线程能够从wait状态中恢复,
然后继续运行wait()后面的语句;
只会唤醒等待该锁的其中一个线程。
notifyAll()方法表示,
当前的线程已经放弃对资源的占有,
通知所有的等待线程从wait()方法后的语句开始运行;
唤醒等待该锁的所有线程。
*/
public class ThreadB extends Thread {
Water water;
public ThreadB(Water waterArg) {
water = waterArg;
}
public void run() {
System.out.println("启动排水");
if (water.isEmpty()) { // 如果水塘无水
synchronized (water) { // 同步代码块
try {
System.out.println("水塘无水,排水等待中.....");
water.wait(); // 使线程处于等待状态
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
System.out.println("开始排水.....");
for (int i = 5; i >= 1; i--) { // 循环5侧
try {
Thread.sleep(1000); // 休眠1秒,模拟1分钟
System.out.println(i + "分钟");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
water.setWater(false); // 设置水塘无水状态
System.out.println("排水完毕。");
}
}
public class Water {
boolean water = false; // 反应水塘状态的变量
public boolean isEmpty() { // 判断水塘是否无水的方法
return water ? false : true;
}
public void setWater(boolean haveWater) { // 更改水塘状态的方法
this.water = haveWater;
}
public static void main(String[] args) {
Water water=new Water(); // 创建水塘对象
ThreadA threadA = new ThreadA(water); // 创建进水线程
ThreadB threadB = new ThreadB(water); // 创建排水线程
threadB.start(); // 启动排水线程
threadA.start(); // 启动进水线程
}
}
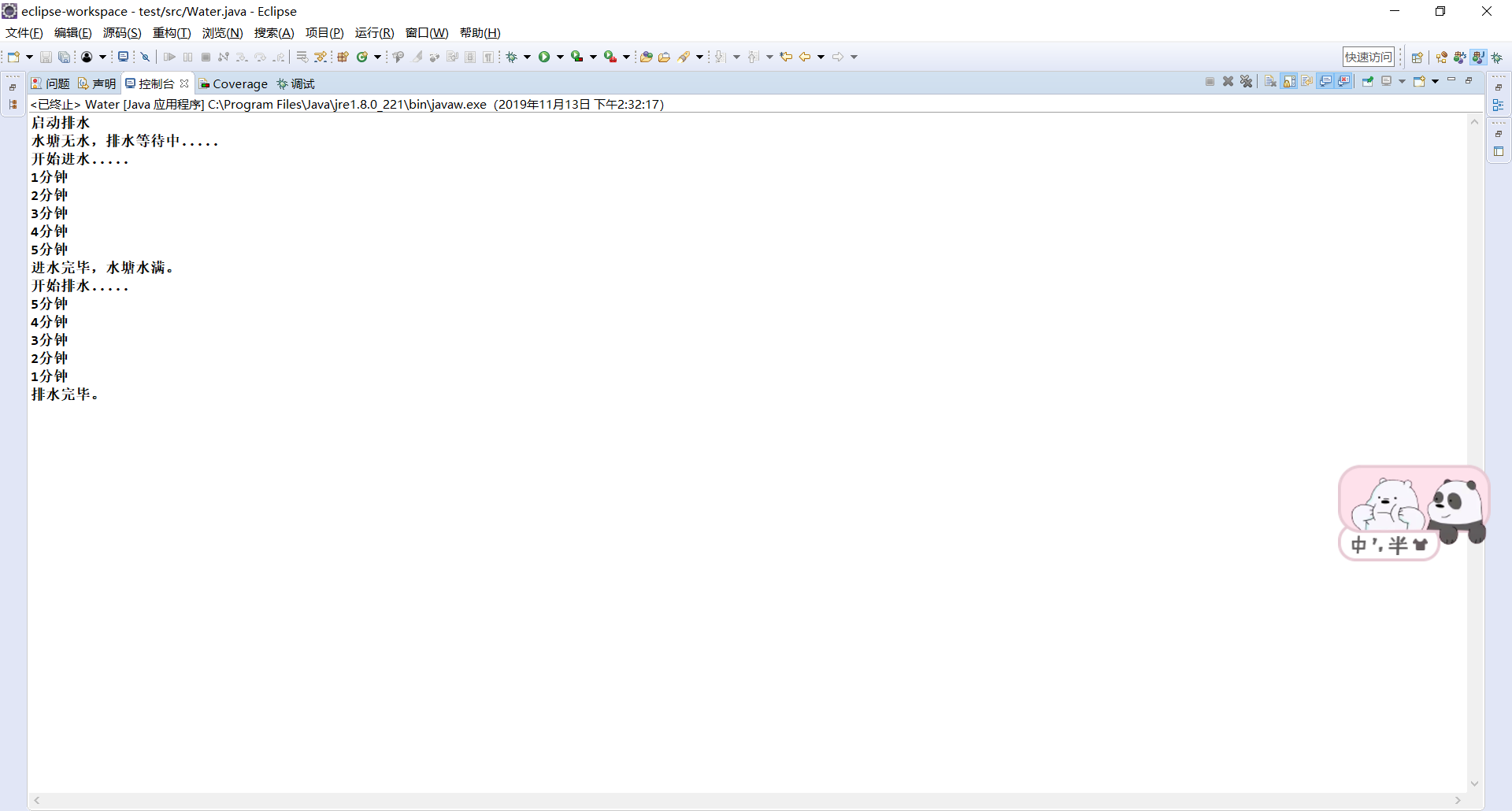
public class Consumer extends Thread {
private Share shared;//共享资源区
private int number;
public Consumer(Share s, int number) {
shared=s;
this.number=number;
}
public void run() {
int value = 0;
for (int i=0; i<10; i++) {
value=shared.get();//从共享区消费数字
System.out.println("消费者"+this.number+" 得到的数据为:"+value);
}
}
}
public class Producer extends Thread {
private Share shared;//共享资源区
private int number;
public Producer(Share s, int number) {
shared=s;
this.number=number;
}
public void run() {
for (int i=0; i<10; i++) {
shared.put(i);//生产数字放入共享区
System.out.println("生产者"+this.number+" 输出的数据为:"+i);
try {
sleep((int)(Math.random() * 100));//休眠随机时间
} catch (InterruptedException e) {}
}
}
}
//(1)
public class Share {
private int contents;
public int get(){//从共享区取数字
return contents;
}
public void put(int value){//放入共享区
contents=value;
}
}
public class PCTest {
public static void main(String[] args) {
Share s=new Share();
Producer p=new Producer(s,1);
Consumer c=new Consumer(s,1);
p.start();
c.start();
}
}
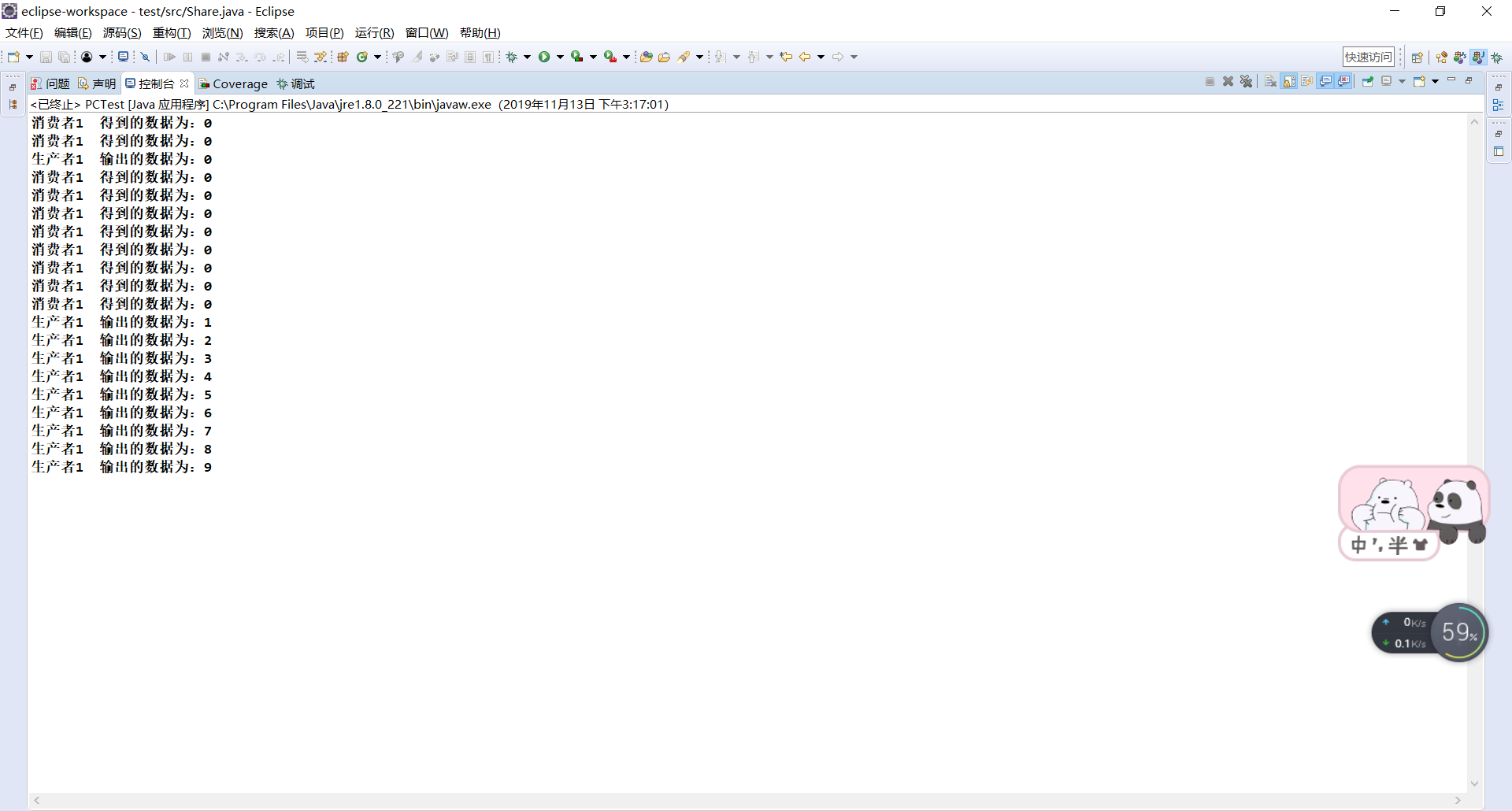
public class Share {
private int contents;
private boolean available = false;
//private int count=0;
//生产者
public synchronized int get() {
while (available==false) {
try {
Thread.sleep(1000);
wait();
} catch (InterruptedException e) {}
available=false;
notifyAll();
}
return contents;
}
//生产者输出
public synchronized void put(int value) {
while (available == true) {
try {
wait();
} catch (InterruptedException e) {
}
}
contents = value;
available =true;
notifyAll();
}
}
/*
*等待唤醒机制就是用于解决线程间通信的问题的,使用到的3个方法的含义如下:
wait:告诉当前线程放弃执行权,并放弃监视器(锁)并进入阻塞状态,直到其他线程持有获得执行权,并持有了相同的监视器(锁)并调用notify为止。
notify:唤醒持有同一个监视器(锁)中调用wait的第一个线程,被唤醒的线程是进入了可运行状态。等待cpu执行权。
notifyAll:唤醒持有同一监视器中调用wait的所有的线程。
*
*/
*
*/
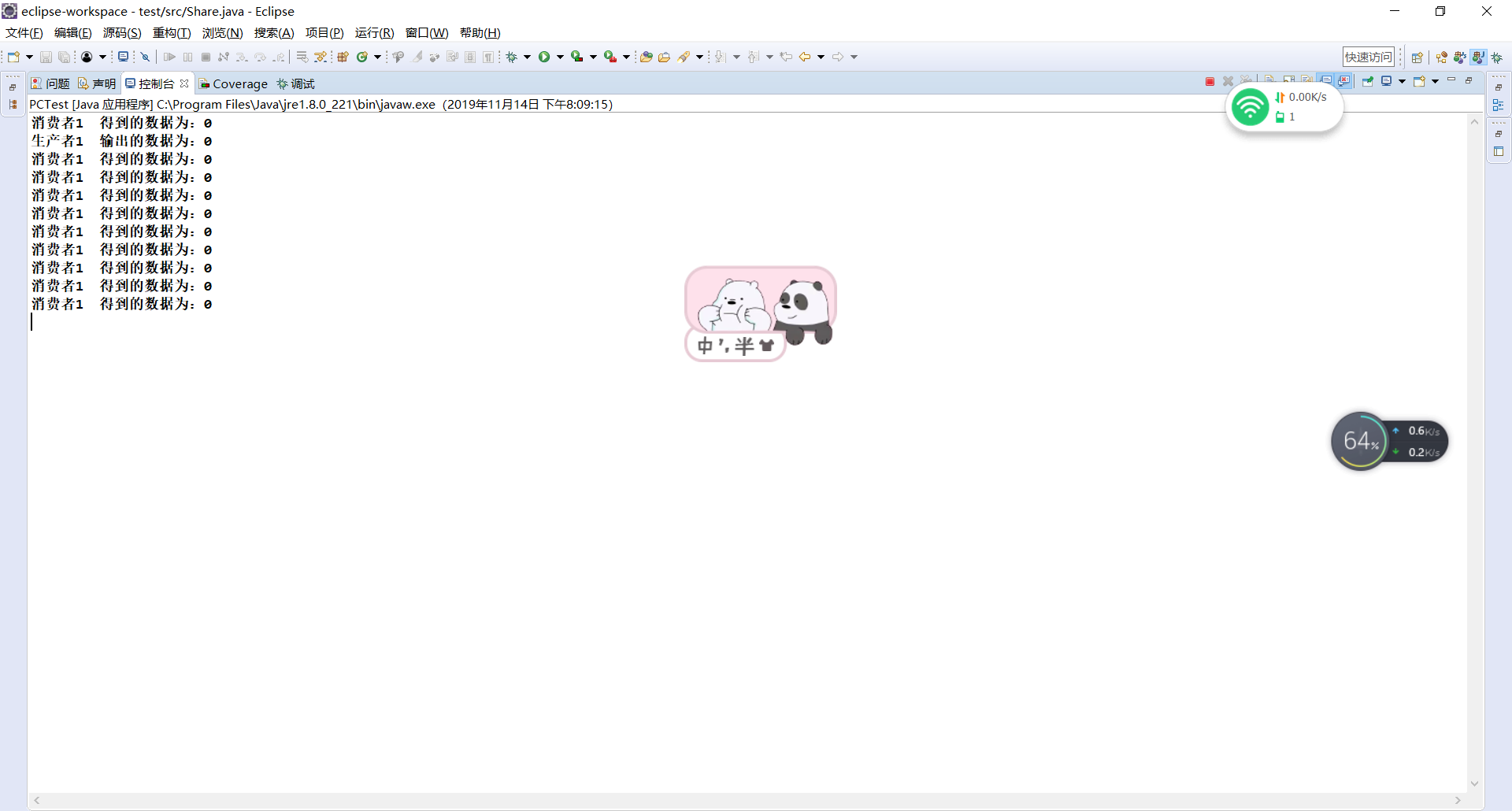