1、Python多条件判断:
多条件判断
if:
pass
else:
pass
循环
while for
i = 0
while i > 1:
print('hello')
else:
print('结束!')
i +=1
for i in range(5):
if i ==2:
print(2)
break
else:
print('正常循环结束后执行')
例子:
#登录程序,最多输入错误3次,输入账号密码,校验为空的情况,输入错误达到3次提示
#for循环
# import datetime
# today = datetime.date.today()
# for i in range(3):
# username = input('请输入账号:').strip()
# password = input('请输入密码:').strip()
# if username == 'wanghao' and password == '123456':
# welcome = '%s 欢迎登陆,今天的日期是:%s,程序结束 '%(username,today)
# print(welcome)
# break
# elif username == '' or password == '':
# print('账号密码不能为空!!!!!')
# else:
# print('账号密码错误!!!!')
# else:
# print('错误次数过多!!!!')
#while循环实现
import datetime
today = datetime.date.today()
count = 0
while count<3:
username = input('请输入账号:').strip()
password = input('请输入密码:').strip()
if username == 'wanghao' and password == '123456':
welcome = '%s 欢迎登陆,今天的日期是:%s,程序结束 '%(username,today)
print(welcome)
break
elif username == '' or password == '':
print('账号密码不能为空!!!!!')
else:
print('账号密码错误!!!!')
count +=1
else:
print('错误次数过多!!!!')
# Python数据类型:
# int 类型
# float 小数类型
# string 字符串
# True False 布尔类型
# stus='土匪,光比,渣渣辉,小黑,小白白,恩特'
#list列表增删改查
new_stus = ['土匪','光比','渣渣辉','小黑','小白白','恩特']
#列表、list、数组、array都指的是数组
#下标、索引、角标、编号
print(new_stus[2])
print(new_stus[-1])
#最前面一个元素的下标是0,最后面一个元素的下标是-1
查询:
cites = []
cites.append('北京') #append方法在列表末尾增加一个元素
cites.insert(0,'上海') #在指定的位置增加元素
cites.append('广州')
cites.append('杭州')
print(cites)
增
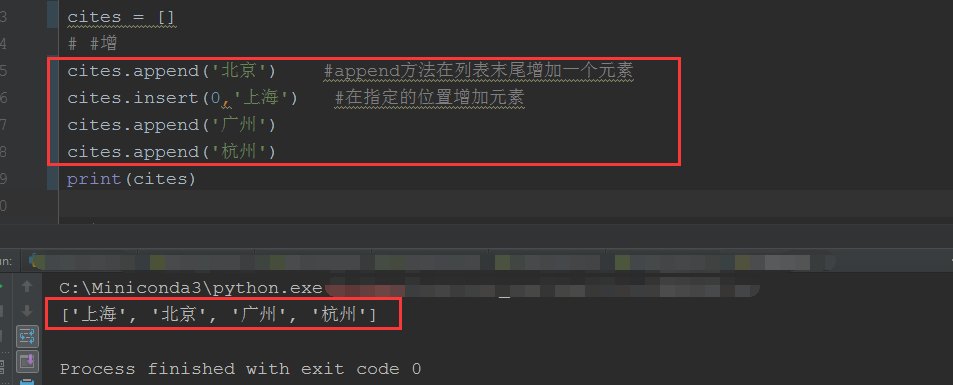
#删
cites = ['上海', '北京', '广州', '杭州']
cites.pop(-1) #删除指定位置的元素 -1删除最后一个元素
cites.remove('广州') #删除指定的元素 要传元素
# cites.clear() #清空list
# del cites[-1] #删除指定位置的元素
print(cites)
#改
cites = ['上海', '北京', '广州', '杭州']
print(cites)
cites[1] = '南京' #修改指定位置的元素,修改的时候如果指定元素的下标不存在会报错
print(cites)
#查
cites = ['上海', '北京', '广州', '杭州']
print(cites[0]) #指定位置的
print(cites.index('广州')) #获取元素的下标,如果找不到那个元素的话会报错
print(cites.count('广州')) #看元素在list里面出现了多少次
my_list = ['python','jmeter','postman','charles']
print(my_list.reverse()) #只是把这个list反转一下
print(my_list)
#排序
nums = [6,44,56,13,8,1,54356,231,567,3215,5673]
nums1 = ['s','e','f','g','h','b','n']
nums.sort() #排序,升序
# nums.sort(reverse=True) #排序,降序
nums1.sort()
nums1.sort(reverse=False) #排序,正序
# nums1.sort(reverse=True) #排序,倒序
print(nums)
print(nums1)
#合并、复制
# cities = ['哈尔滨','长春','吉林','黑龙江','沈阳']
# cities1 = ['武汉','厦门','澳门','香港','珠海']
# print(cities + cities1) #合并list
# print(cities * 3) #复制list N次
#多维数组取值
words = ['view','code','tools',['price','num',1995,['hello','usa','吃鸡']]]
print(words[3][3][2])
print(words[-1][2])