Given a string, find the length of the longest substring without repeating characters.
Example 1:
Input: "abcabcbb"
Output: 3
Explanation: The answer is "abc"
, with the length of 3.
Example 2:
Input: "bbbbb"
Output: 1
Explanation: The answer is "b"
, with the length of 1.
Example 3:
Input: "pwwkew" Output: 3 Explanation: The answer is"wke"
, with the length of 3. Note that the answer must be a substring,"pwke"
is a subsequence and not a substring.
定义两个辅助数组:
last:在串0到(i-1)中,i位置字符最近出现的位置;
res:存放以i位置字符结尾的最长互不重复的子串长度;
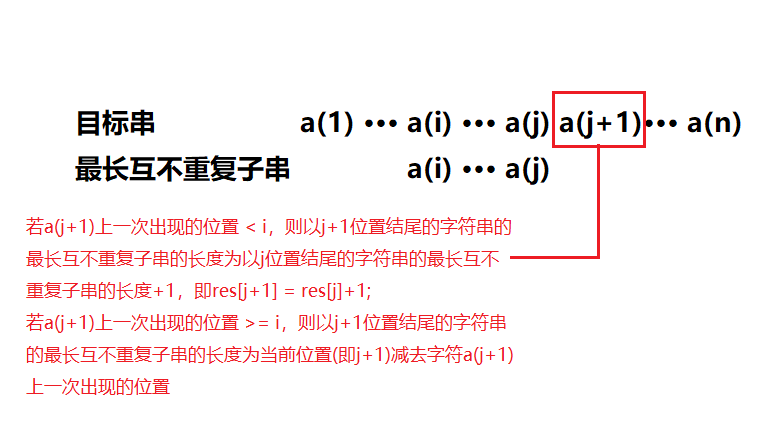
1 class Solution8 { 2 public int lengthOfLongestSubstring(String s) { 3 if (s.length() < 1) return 0; 4 if (s.length() == 1) return 1; 5 int[] last = new int[s.length()];//存放在串0到(i-1)中,i位置字符最近出现的位置 6 int[] res = new int[s.length()];//存放以i位置字符结尾的最长互不重复的子串长度 7 int max = 1; 8 Map<Character, Integer> map = new HashMap<>(); 9 for (int i = 0; i < s.length(); i++) { 10 Integer lastLoc = map.get(s.charAt(i)); 11 if (lastLoc != null) { 12 last[i] = lastLoc; 13 }else { 14 last[i] = -1; 15 } 16 map.put(s.charAt(i), i); 17 } 18 19 res[0] = 1; 20 for (int i = 1; i < s.length(); i++) { 21 if (last[i] < i - 1 - res[i-1]){ 22 res[i] = res[i-1] + 1; 23 }else { 24 res[i] = i - last[i]; 25 } 26 max = (max < res[i]) ? res[i] : max; 27 } 28 return max; 29 } 30 }