#while循环和 for 循环不同的另一种循环是 while 循环,while 循环不会迭代 list 或 tuple 的元素,而是根据表达式判
断循环是否结束
##while循环的用法
"""while 50<a<100:
print(a)
这里print(a)会报错a没有找到;while区别for这里的a需要明确赋值,判断赋值的取值范围,且并无50<a<100在while中的用户
"""
a=15 while a<100: print(a) """ while 判断条件: 执行语句 这里while会进入死循环中,Ctrl+c可终止循环"""
#a使用while循环实现输出2-3+4-5+6....+100的和
"""a=2
while a<=100:
count=a-(a+1)
a+=1
print(count)
这里不执行
"""
#a使用while循环实现输出2-3+4-5+6....+100的和 a=2 num=0 while a<=100: if a%2==0: num=num+a else: num=num-a a+=1 print(num) """ 奇数减,偶数增 """
"""也可以用递归来写
"""
def num(a):
if a==2:
return 2
return a-num(a-1)
num(100)
#使用while循环实现输出1,2,3,4,5,7,8,9,11,12 a=0 while a<=12: a+=1 if a==6or a==10: pass else: print(a)
#使用while循环输出100-50,从大到小,如100,99,98...,到50时候再从0循环输出到50,然后结束 #存在问题:
a=100 while a<=100: #注意这里的写法是a<=100而不是a=<100 小于等于 if a>=50: print(a) a-=1 else: print(a) a+=1 break
#这里执行到a=50就不执行了,当a=-1执行后a=49,执行else,print(a):49,break后即不执行了;去掉break会在49和50之间进入死循环
if判断语句不会循序执行,因此需要在while里面再添加,而且break用法有误
#使用while循环输出100-50,从大到小,如100,99,98...,到50时候再从0循环输出到50,然后结束
#正确写法1:
a=100
while a<=100:
if a>=50:
print(a)
a-=1
else:
a=0
while a<=50:
print(a)
a+=1
break#这里break终止循环 若删除则后面的break则死循环
#正确写法:2:
a = 100
while a >= 50:
print(a)
a -= 1
if a == 49:
a = 0
while a <= 50:
print(a)
a += 1
#使用while循环实现输出1-100内所有的奇数
#这个数不能被2整除,并能将判断结果打印出来,并且a能递增; 先判断循环条件,再判断结束条件
#错误用法:
a=1
while a<=100:
if a%2!=0:
print(a)
a+=1
#这里a+=1的位置错误,注意 if 条件判断:执行语句;
#这里自会打印1,之后的运算都会执行
如:
a=1
b=10
while a<=100:
if a%2!=0:
print(a)
print('15')
b=5+a
a+=1
a=5+1
"""这里输出结果为:1,15,后面结果不执行"""
#正确用法:
while a<=100:
if a%2!=0:
print(a)
a+=1
#使用while循环实现输出1-100内所有的偶数 a=1 while a<=100: if a%2==0: print(a) a+=1
#while循环中的break和continue的 用法 #用 for 循环或者 while 循环时,如果要在循环体内直接退出循环,可以使用 #break 语句
比如计算1至100的整
数和,我们用while来实现:
sum = 0
x = 1
while True:
sum = sum +x
x = x + 1
if x > 100:
break
print sum
while True 就是一个死循环,但是在循环体内,我们还判断了 x > 100 条件成立时,用break语句退出循环,这
样也可以实现循环的结束。
#continue继续循环
#在循环过程中,可以用break退出当前循环,还可以用continue跳过后续循环代码,继续下一次循环。
#例如:
L = [75, 98, 59, 81, 66, 43, 69, 85]
for x in L:
if x < 60:
contimue
print x
利用continue,可以只打印大于或等于60的L里的元素。
#关于while循环中if存在的问题,关于执行过程依旧有些问题,待解答,请留言,谢谢,
#a+=1在while循环if判断中的执行问题 #第一:正常执行 a=1 while a <10: if a>0: print(a) a+=1 print("-"*15) #正确用法 a=1 while a <10: if a%2==1: print(a) a+=1#这里位置有差异 print("-"*15) #错误用法 a=1 while a<15: if a%2==1: print(a) a+=1 elif a%3==1: #elif 不运行,但是一直占用内存空间,进程不停止 print(a) a+=1
#判断age是否等于31,如果等于直接跳出循环,如果连续输错3次也会跳出循环。
#https://www.cnblogs.com/lin-777/p/6941432.html age = 31 count = 0 while True: if count == 3 : break #跳出循环 guess_age = int(input("guess age:")) if guess_age == age: print("Yes,you got it") break elif guess_age > age: print("Think smaller..") else: print("Think bigger..") count += 1
#优化版 age= 31 #首先定义一个固定的变量值用于比较 count = 0 #定义循环次数,初始为0 while count < 3: #当count 小于 3的时候循环开始,否则停止循环 guess_age = int(input("guess age :")) #以数字整型方式输入内容 if guess_age == age: #如果输入内容等于之前定义的变量值,则跳出循环 print("Yes,you got it !") break elif guess_age > age: #如果输入的内容大于之前定义的变量值,则循环继续 print("Try smaller...") else: #如果输入的内容小于之前定义的变量值,则循环继续 print("Try bigger!") count += 1 #if count == 3: #当循环到第三次结束的时候,打印定义好的内容 else: #此处的else 相当于上面的 if count == 3 print("Try too many")
#自定义循环次数 #判断age_of_sd是否等于31,如果等于直接跳出循环,如果连续输错3次也会跳出循环. age = 31
count = 0 while count < 3 : guess_age = int(input("guess age:")) if guess_age == age: print("Yes,you got it") break elif guess_age > age: print("Think smaller..") else: print("Think bigger..") count += 1 #原链接内容缩进错误 #如果上面的while count < 3不成立,则执行第二次判断,是否继续执行循环, # 如果输入n,则跳出循环,如果输入其他,则循环继续。 if count == 3 : countine_confirm = input("Do you wang to keep guessing? ") if countine_confirm != 'n': count = 0
a=3 while a<20: print('<20的a:',a) if a<13: print('<13的a:',a)
elif a<8 and a>4: print('elif里的a:',a) else: print('最后的a:',a) a+=1
output:
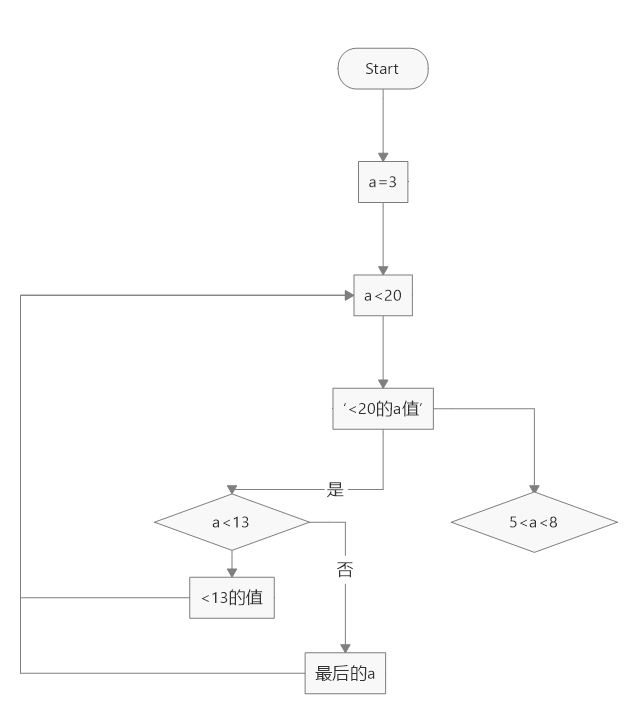