本文介绍mybatis在spring-boot中使用的几种方式
项目结构
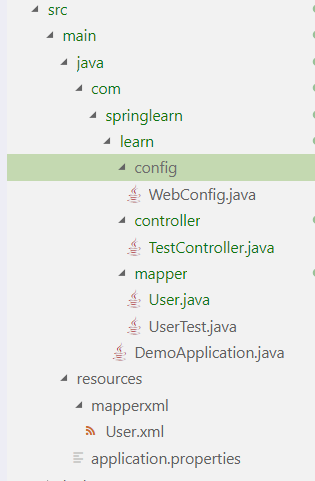
依赖
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.2</version>
</dependency>
WebConfig
package com.springlearn.learn.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
@EnableScheduling
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("*").allowedMethods("GET", "POST", "PUT", "DELETE").allowedOrigins("*")
.allowedHeaders("*");
}
}
DemoApplication
package com.springlearn.learn;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
方式一——@Select
User
package com.springlearn.learn.mapper;
import java.util.Map;
import java.util.Map;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
@Mapper
public interface User {
@Select("select * from test where id=#{id}")
Map GetUserbyId(@Param("id") Integer id);
}
DemoApplication
@RestController
public class TestController {
@Autowired
User user;
@ResponseBody
@RequestMapping(value = "/test1", method = RequestMethod.GET)
public Map Test1(HttpServletRequest request){
Map result = user.GetUserbyId(1);
return result;
}
}
方式二——@Select和SqlSession结合
User
package com.springlearn.learn.mapper;
import java.util.Map;
import java.util.Map;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
@Mapper
public interface User {
@Select("select * from test where id=#{id}")
Map GetUserbyId(@Param("id") Integer id);
}
DemoApplication
@RestController
public class TestController {
@Autowired
User user;
@Autowired
SqlSession sqlSession;
@ResponseBody
@RequestMapping(value = "/test2", method = RequestMethod.GET)
public Map Test2(HttpServletRequest request){
// 用法一
Map result = sqlSession.selectOne("com.springlearn.learn.mapper.User.GetUserbyId", 1);
// 用法二
User mapper = sqlSession.getMapper(User.class);
Map result = mapper.GetUserbyId(1);
return result;
}
}
方式三——xml和SqlSession结合
applicationproperties
mybatis.mapper-locations=classpath:mapperxml/*.xml
UserTest
package com.springlearn.learn.mapper;
import java.util.Map;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
@Mapper
public interface UserTest {
Map<String, Object> GetUserbyId(@Param("id") Integer id);
}
Userxml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.springlearn.learn.mapper.UserTest">
<select id="GetUserbyId" parameterType="int" resultType="java.util.Map">
select * from test where id=#{id}
</select>
</mapper>
TestController
@RestController
public class TestController {
@Autowired
User user;
@Autowired
SqlSession sqlSession;
@ResponseBody
@RequestMapping(value = "/test3", method = RequestMethod.GET)
public Map<String, Object> Test3(HttpServletRequest request, @RequestParam("id") int id){
// 用法一
Map<String, Object> result = sqlSession.selectOne("com.springlearn.learn.mapper.UserTest.GetUserbyId", id);
// 用法二
UserTest mapper = sqlSession.getMapper(UserTest.class);
System.out.println(mapper.GetUserbyId(id));
Map<String, Object> result = mapper.GetUserbyId(id);
return result;
}
}