编写一个C程序,输入3个数,并按由大到小的顺序输出。
1 #include <stdio.h> 2 void main(){ 3 int a,b,c,t; 4 printf("请输入三个整数:"); 5 scanf("%d%d%d",&a,&b,&c); 6 if(a<b){ 7 t = a; 8 a = b; 9 b = t; 10 } 11 if(b>c){ 12 printf("%d\t%d\t%d\n",a,b,c); 13 } 14 else if(c>a){ 15 printf("%d\t%d\t%d\n",c,a,b); 16 } 17 else{ 18 printf("%d\t%d\t%d\n",a,c,b); 19 } 20 }
实验要求:从键盘上输入x的值,并根据计算输出y的值
提示:
- 使用数据函数需要#include <math.h>
- 开方函数:sqrt(x)
- 绝对值函数:fabs(x)
源码
#include <math.h>
void
main(){
int
x,y;
printf (
"输入X:"
);
scanf(
"%d"
,&x);
if
(x>4){
y=sqrt(x-4);
printf(
"%d\n"
,y);
}
else
if
(x<-5){
y=fabs(x);
printf(
"%d\n"
,y);
}
else
{
y=x+3;
printf(
"%d\n"
,y);
}
}

实验要求:从键盘上输入一个字母,如果是小写字母,将其转换成大写字母并输出。
提示:
- 输入字符给变量c
char c;
方法一:c = getchar();
方法二:scanf("%c",&c);
- 输出字符变量c
方法一:putchar(c);
方法二:printf("%c",c);
程序源码
#include <stdio.h>
int
main(){
char
c;
printf(
"请输入一个字母:"
);
scanf(
"%c"
,&c);
printf(
"%c\n"
,c-32);
}
#include <stdio.h>
int
main(){
printf(
"请输入一个字母:"
);
char
c;
c=getchar();
if
(c<=
'z'
&& c>=
'a'
)
c=c-32;
putchar(c);
}

实验要求:从键盘上输入x的值,并根据计算输出y的值
程序源码
#include <math.h>
#include <stdio.h>
int
main(){
int
x,y;
printf(
"输入X:"
);
scanf(
"%d"
,&x);
if
(x<1){
y=x;
printf(
"%d\n"
,y);
}
else
if
(1<=x && x<10){
y=(2*x-1);
printf(
"%d\n"
,y);
}
else
{
y=(3*x-11);
printf(
"%d\n"
,y);
}
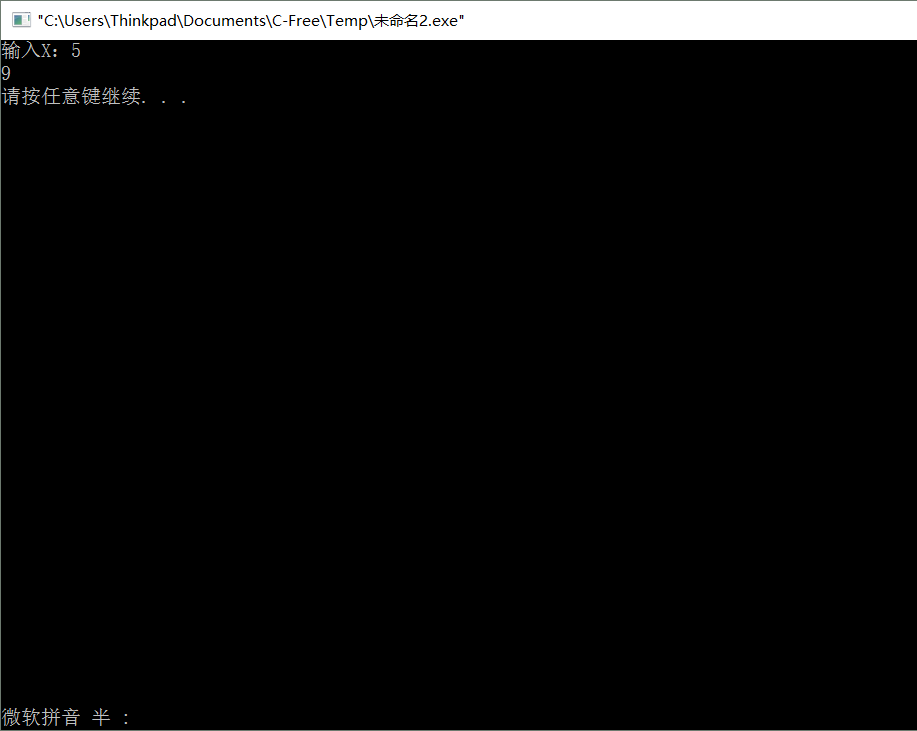
实验要求:
给出一个百分制的成绩,要求出成绩等级’A’、’B’、’C’、’D’、’E’,其中90分以上输出’A’,80~89输出’B’,70~79输出’C’,60~69输出’D’,60分以下输出’E’。
提示:
本实验要求同学们采用两种方法来完成:
方法一:使用if语句完成
方法二:使用switch语句完成。
程序源码
#include <stdio.h>
void
main(){
int
x;
printf (
"输入成绩:"
);
scanf(
"%d"
,&x);
if
(x>=90)
printf(
"A"
);
else
if
(x>=80 && x<=89)
printf(
"B"
);
else
if
(x>=70 && x<=79)
printf(
"C"
);
else
if
(x>=60 && x<=69)
printf(
"D"
);
else
printf(
"E"
);
}
#include <stdio.h>
int
main(){
int
x;
printf(
"请输入成绩:\n"
);
scanf(
"%d"
,&x);
switch
(x/10)
{
case
9:
printf(
"A\n"
);
break
;
case
8:
printf(
"B\n"
);
break
;
case
7:
printf(
"C\n"
);
break
;
case
6:
printf(
"D\n"
);
break
;
default
:
printf(
"E\n"
);
}
return
0;
}

实验心得
总体来说还是感觉做的不是很好,也是经过同学的一些提示才能做出来,对自己不太满意,解决问题这方面不太好,希望自己能多改进