实验内容:
抽象类:
package exercise;
public abstract class Shape {
public abstract double getArea();
}
三角形:
package exercise;
public class Delta extends Shape {
private double a;
private double b;
private double c;
public Delta(double a,double b,double c) {
this.setA(a);
this.setB(b);
this.setC(c);
}
public void setA(double a) {
this.a=a;
}
public double getA() {
return a;
}
public void setB(double b) {
this.b=b;
}
public double get() {
return b;
}
public void setC(double c) {
this.c=c;
}
public double getC() {
return c;
}
public double getArea() {
return Math.sqrt((a+b+c)/2*((a+b+c)/2-a)*((a+b+c)/2-b)*((a+b+c)/2-c));
}
}
圆:
package exercise;
public class Round extends Shape {
private double radius;
public Round(double radius) {
this.setRadius(radius);
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
}
矩形:
package exercise;
public class Rectangle extends Shape {
private double hight;
private double width;
public Rectangle(double width, double hight) {
this.setWidth(width);
this.setHight(hight);
}
public void setWidth(double width) {
this.width = width;
}
public double getWidth() {
return width;
}
public void setHight(double hight) {
this.hight = hight;
}
public double getArea() {
return hight * width;
}
}
测试类:
package exercise;
public class text {
public static void main(String[] args) {
Shape rectangle1 = new Rectangle(30,30);
Shape delta1 = new Delta(3,4,5);
Shape round1 = new Round(20);
System.out.println("矩形的面积:"+rectangle1.getArea());
System.out.println("三角形的面积:"+delta1.getArea());
System.out.println("圆的面积:"+round1.getArea());
}
}
运行结果:
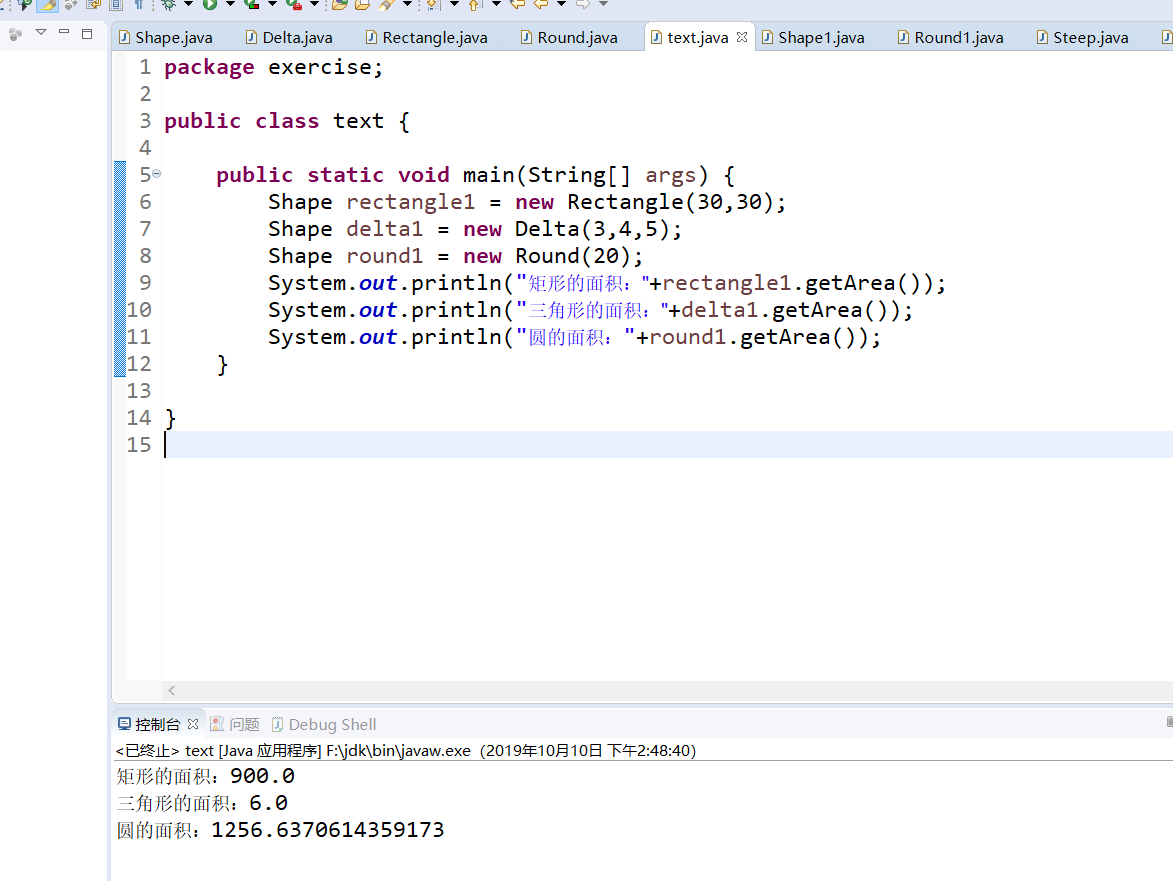
遇到的问题:求三角形面积时,给的sqrt函数总是报错,然后一直找不出来问题,百度之后,发现要用Math.sqrt就好了,而且圆周率是Math.pI,涨知识了。还有在继承抽象类时,总是会出现重名的情况,就是普通的一个类也会有,看书后才知道,原来所有的没有明确父类的,都是继承的Object类。
实验2:
抽象类:
package exercise;
public interface Shape1 {
public abstract double getArea();
public abstract double getPerimeter();
}
class Factory {
public static Shape1 getInstance(String name) {
Shape1 s = null;
if ("Round1".equals(name)) {
s = new Round1(30);
}
if ("Steep".equals(name)) {
s = new Steep(30);
}
return s;
}
}
直线类
package exercise;
public class Steep implements Shape1 {
private double hight;
public Steep(double hight) {
this.setHight(hight);
}
public void setHight(double hight) {
this.hight = hight;
}
public double getHight() {
return hight;
}
public double getPerimeter() {
return this.hight;
}
public double getArea() {
return 0;
}
}
圆类
package exercise;
public class Round1 implements Shape1 {
private double radius;
public Round1() {
}
public Round1(double radius) {
this.setRadius(radius);
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
public double getPerimeter() {
return Math.PI * 2 * radius;
}
}
测试类
package exercise;
public class test {
public static void main(String[] args) {
Shape1 c = new Round1(30);
Shape1 s = new Steep(30);
System.out.println("圆的面积:" + c.getArea());
System.out.println("圆的周长:" + c.getPerimeter());
System.out.println("直线的周长:" + s.getPerimeter());
}
}
运行结果

遇到的问题:在刚开始看题目的时候就觉得奇怪,直线怎么样求大小呢?让我十分迷惑,然后我就只有求了一个周长。还有在实现接口后的类中的实例化,遇到了一些问题,如果像工厂一样的实例化方法,那么可不可以在进行赋值呢?在经过我的测试以后,发现是不行的,因为早已经实例化了,赋值也完成了,所以对于这个题目就只能采用直接实例化了,因为要进行赋值。
学习总结
Object类:
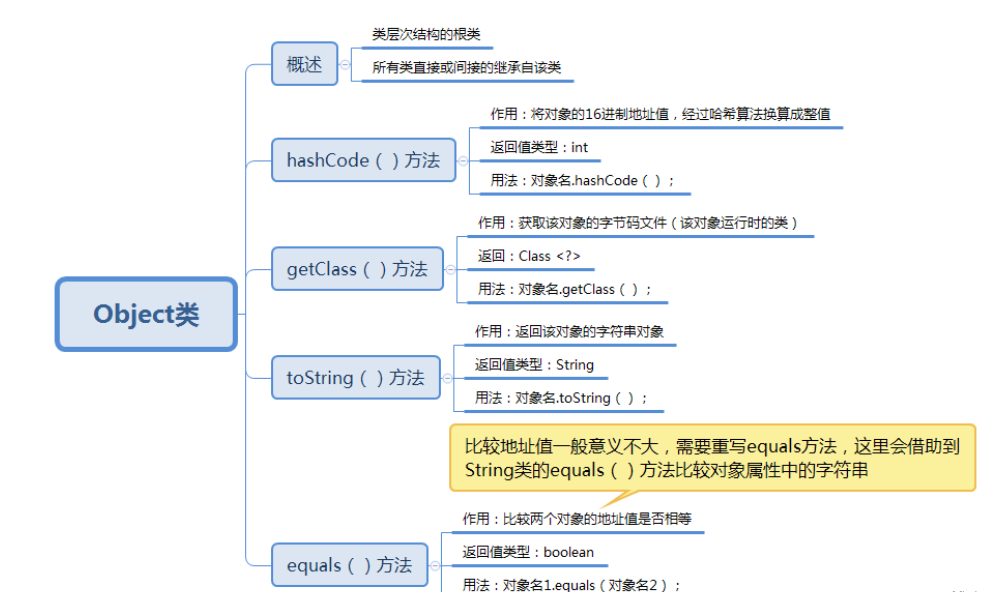
toString方法:
1、java语言很多地方会默认调用对象的toString方法。
如果不重写toString方法,将会 使用Object的toString方法,其逻辑为 类名@散列码,toString方法是非常有用的调试工具,如果重写了toString方法,调试时断电到对象会显示出对象各个属性及其值,如果不重写则显示 类名@散列码
所以最好是为自己定义的每个类加toString方法
eg:字符串+对象 ,自动调用对象的toString方法。
eg:system.out.print(任意对象) 自动调用对象的toSt方法。
equals方法:
1、equals()是Object中的方法,作用在于检测一个对象是否等于另一个对象。
2、在Object类中equals()方法是判断两个对象是否有相同的引用 ,即是否是相同的对象。
3、String重写equals()方法,String的equals()方法用途比较两个字符对象的字符序列是否相同