Segment set
Time Limit: 3000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 3457 Accepted Submission(s): 1290
Problem Description
A segment and all segments which are connected with it compose a segment set. The size of a segment set is the number of segments in it. The problem is to find the size of some segment set.
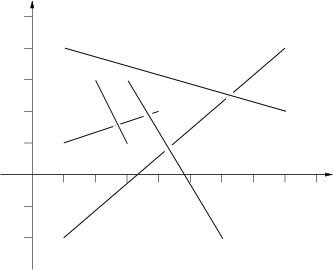
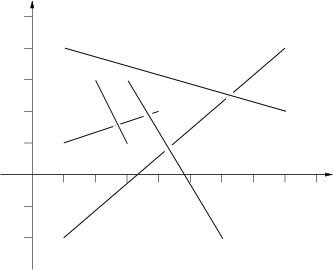
Input
In the first line there is an integer t - the number of test case. For each test case in first line there is an integer n (n<=1000) - the number of commands.
There are two different commands described in different format shown below:
P x1 y1 x2 y2 - paint a segment whose coordinates of the two endpoints are (x1,y1),(x2,y2).
Q k - query the size of the segment set which contains the k-th segment.
k is between 1 and the number of segments in the moment. There is no segment in the plane at first, so the first command is always a P-command.
There are two different commands described in different format shown below:
P x1 y1 x2 y2 - paint a segment whose coordinates of the two endpoints are (x1,y1),(x2,y2).
Q k - query the size of the segment set which contains the k-th segment.
k is between 1 and the number of segments in the moment. There is no segment in the plane at first, so the first command is always a P-command.
Output
For each Q-command, output the answer. There is a blank line between test cases.
Sample Input
1
10
P 1.00 1.00 4.00 2.00
P 1.00 -2.00 8.00 4.00
Q 1
P 2.00 3.00 3.00 1.00
Q 1
Q 3
P 1.00 4.00 8.00 2.00
Q 2
P 3.00 3.00 6.00 -2.00
Q 5
Sample Output
1
2
2
2
5
Author
LL
Source
题目大意:有n个指令,p加入一条线段,q查询id线段所在集合(两线段有交点为同一集合)的元素个数。
思路:用并查集路径压缩记录各个线段间的关系,根据叉积的定义有:Cross(v,w)=0时w在v上,>0时w在v上方,<0时w在v下方。
两线段有交点的必要条件:必须每条线段的两个端点在另一线段的两侧或直线上。
1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <cmath> 5 using namespace std; 6 7 const double eps=1e-8; 8 const int maxn=1005; 9 int f[maxn]; 10 struct Point 11 { 12 double x,y; 13 Point(){} 14 Point(double x,double y):x(x),y(y){} 15 }; 16 struct Line 17 { 18 Point a,b; 19 }L[maxn]; 20 typedef Point Vector; 21 Vector operator -(Vector A,Vector B){return Vector(A.x-B.x,A.y-B.y);} 22 int dcmp(double x) 23 { 24 if(fabs(x)<eps) return 0; 25 else return x<0?-1:1; 26 } 27 double Cross(Vector A,Vector B){ return A.x*B.y-A.y*B.x;}//叉积 28 29 bool judge(Line a,Line b)//Cross(v,w)=0时w在v上,>0时w在v上方,<0时w在v下方 30 { 31 if(dcmp(Cross(a.a-b.a,b.b-b.a)*Cross(a.b-b.a,b.b-b.a))<=0 32 &&dcmp(Cross(b.a-a.a,a.b-a.a)*Cross(b.b-a.a,a.b-a.a))<=0) 33 return true; 34 return false; 35 } 36 int findset(int x){return f[x]!=x?f[x]=findset(f[x]):x;} 37 void Union(int a,int b) 38 { 39 a=findset(a);b=findset(b); 40 if(a!=b) f[a]=b; 41 } 42 int main() 43 { 44 int t,n,i,j,id; 45 char op[5]; 46 double x1,y1,x2,y2; 47 scanf("%d",&t); 48 while(t--) 49 { 50 scanf("%d",&n); 51 int cnt=0; 52 for(i=0;i<n;i++) 53 { 54 scanf("%s",op); 55 if(op[0]=='P') 56 { 57 scanf("%lf%lf%lf%lf",&x1,&y1,&x2,&y2); 58 L[++cnt].a=Point(x1,y1);L[cnt].b=Point(x2,y2); 59 f[cnt]=cnt; 60 for(j=1;j<=cnt-1;j++) 61 if(judge(L[cnt],L[j])) 62 Union(j,cnt); 63 } 64 else 65 { 66 int ans=0;scanf("%d",&id); 67 id=findset(id); 68 for(j=1;j<=cnt;j++) 69 if(findset(j)==id) 70 ans++; 71 printf("%d ",ans); 72 } 73 } 74 if(t) printf(" "); 75 } 76 return 0; 77 }