java 多线程 目录:
概述
第1部分 配置
有关quartz的api文档地址:Quartz Enterprise Job Scheduler 1.8.6 API
主要接口目录:
重点看下Job,Scheduler,Trigger,JobDetail几个:
代表任务的类继承Job接口,该接口只有唯一一个方法 execute;当一个任务的触发器启动时,相应的调度器scheduler会调用该任务。
quartz并不保存一个实际的Job类,而是通过允许你定义一个JobDetail取代。
Scheduler代表JobDetails和Triggers的登记表,Scheduler由SchedulerFactory产生。
quartz的配置文件quartzConfig.xml如下:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:security="http://www.springframework.org/schema/security" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.1.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.1.xsd http://www.springframework.org/schema/security http://www.springframework.org/schema/security/spring-security-3.1.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.1.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.1.xsd http://www.springframework.org/schema/cache http://www.springframework.org/schema/cache/spring-cache-3.1.xsd http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.1.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.1.xsd http://www.springframework.org/schema/jms http://www.springframework.org/schema/jms/spring-jms-3.1.xsd http://www.springframework.org/schema/lang http://www.springframework.org/schema/lang/spring-lang-3.1.xsd http://www.springframework.org/schema/oxm http://www.springframework.org/schema/oxm/spring-oxm-3.1.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.1.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.1.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.1.xsd"> <!-- ====================================================================================== --> <!-- 拦截器配置文件 --> <!-- ====================================================================================== --> <!-- <bean id="startQuartz" lazy-init="false" autowire="no" class="org.springframework.scheduling.quartz.SchedulerFactoryBean"> </bean> --> <!-- ====================================================================================== --> <!-- 要调用的工作类 --> <bean id="quartzJob" class="com.util.CallQuartz"></bean> <!-- 定义调用对象和调用对象的方法 --> <bean id="jobtask" class="org.springframework.scheduling.quartz.MethodInvokingJobDetailFactoryBean"> <!-- 调用的类 --> <property name="targetObject"> <ref bean="quartzJob"/> </property> <!-- 调用类中的方法 --> <property name="targetMethod"> <value>callAllQuartz</value> </property> </bean> <!-- 定义触发时间 --> <bean id="doTime" class="org.springframework.scheduling.quartz.CronTriggerBean"> <property name="jobDetail"> <ref bean="jobtask"/> </property> <!-- cron表达式 --> <property name="cronExpression"> <value>*/10 * * * * ?</value> </property> </bean> <!-- 总管理类 如果将lazy-init='false'那么容器启动就会执行调度程序 --> <bean id="startQuertz" lazy-init="false" autowire="no" class="org.springframework.scheduling.quartz.SchedulerFactoryBean"> <property name="triggers"> <list> <ref bean="doTime"/> </list> </property> </bean> <!-- ====================================================================================== --> </beans>
web.xml文件中加入相应上述加载配置文件路径。
第2部分 代码示例
首先定义一个任务的类
1 package com.util.vo; 2 3 import java.io.IOException; 4 import java.net.HttpURLConnection; 5 import java.net.MalformedURLException; 6 import java.net.URL; 7 import java.util.ArrayList; 8 import java.util.HashMap; 9 import java.util.List; 10 import java.util.Map; 11 12 import org.apache.log4j.Logger; 13 import org.quartz.Job; 14 import org.quartz.JobExecutionContext; 15 import org.quartz.JobExecutionException; 16 17 18 /** 19 * 20 * @ClassName: QuartzVo 21 * 22 * @author Xingle 23 * @date 2014-8-14 下午12:49:25 24 */ 25 public class QuartzVo implements Job{ 26 27 public static int Id = 0; 28 29 private static Logger logger = Logger.getLogger(QuartzVo.class); 30 //缓存中任务列表 31 public static List<QuartzVo> quartzList = new ArrayList<QuartzVo>(); 32 //缓存中任务map 33 public static Map<String, QuartzVo> quartzMap = new HashMap<String, QuartzVo>(); 34 35 36 /** 37 * id 38 */ 39 public String id ; 40 /** 41 * 任务名称 42 */ 43 public String jobTitle; 44 /** 45 * 调度路径 46 */ 47 public String jcallpath; 48 /** 49 * 触发表达式 50 */ 51 public String jobcron; 52 /** 53 * @Fields s_date : 开始时间 54 */ 55 public String s_date; 56 /** 57 * @Fields cycle : 循环标示:1 循环;2 单次 58 */ 59 public String cycle; 60 61 public String getId() { 62 return id; 63 } 64 public void setId(String id) { 65 this.id = id; 66 } 67 public String getJobTitle() { 68 return jobTitle; 69 } 70 public void setJobTitle(String jobTitle) { 71 this.jobTitle = jobTitle; 72 } 73 public String getJcallpath() { 74 return jcallpath; 75 } 76 public void setJcallpath(String jcallpath) { 77 this.jcallpath = jcallpath; 78 } 79 public String getJobcron() { 80 return jobcron; 81 } 82 public void setJobcron(String jobcron) { 83 this.jobcron = jobcron; 84 } 85 public String getCycle() { 86 return cycle; 87 } 88 public void setCycle(String cycle) { 89 this.cycle = cycle; 90 } 91 92 public String getS_date() { 93 return s_date; 94 } 95 public void setS_date(String s_date) { 96 this.s_date = s_date; 97 } 98 /** 99 * 执行任务 100 * @Description: 101 * @param arg0 102 * @throws JobExecutionException 103 * @author xingle 104 * @data 2014-8-14 下午12:51:35 105 */ 106 @Override 107 public void execute(JobExecutionContext context) throws JobExecutionException { 108 String jobName = context.getJobDetail().getName(); 109 logger.debug("定时任务【" + jobName + "】 将要执行 start!!"); 110 QuartzVo quartzVo = QuartzVo.quartzMap.get(jobName); 111 String inurl = quartzVo.getJcallpath(); 112 URL url = null; 113 HttpURLConnection conn = null; 114 try { 115 url = new URL(inurl); 116 conn = (HttpURLConnection) url.openConnection(); 117 } catch (MalformedURLException e) { 118 e.printStackTrace(); 119 } catch (IOException e) { 120 System.out.println("***************** 连接失败,程序地址 : " + inurl); 121 e.printStackTrace(); 122 } 123 try { 124 if (conn.getResponseCode() != HttpURLConnection.HTTP_OK) { 125 System.out.println("****************** 调度失败!!,程序地址 : " + inurl); 126 } else { 127 System.out.println("定时任务【" + jobName + "】" + "已完成调度,程序地址: " 128 + inurl); 129 } 130 } catch (IOException e) { 131 e.printStackTrace(); 132 } 133 134 } 135 136 }
定义一个调用任务:
1 package com.util; 2 3 import java.io.BufferedReader; 4 import java.io.File; 5 import java.io.FileNotFoundException; 6 import java.io.FileReader; 7 import java.io.IOException; 8 import java.util.ArrayList; 9 import java.util.HashMap; 10 import java.util.Iterator; 11 import java.util.List; 12 import java.util.Map; 13 import java.util.Map.Entry; 14 15 import org.apache.log4j.Logger; 16 import org.springframework.beans.factory.annotation.Autowired; 17 import org.springframework.beans.factory.annotation.Qualifier; 18 19 20 import com.util.vo.QuartzVo; 21 import com.whalin.MemCached.MemCachedClient; 22 23 /** 24 * 调用任务 25 * @ClassName: CallQuartz 26 * TODO 27 * @author Xingle 28 * @date 2014-8-14 下午12:48:44 29 */ 30 public class CallQuartz { 31 private static Logger logger = Logger.getLogger(CallQuartz.class); 32 33 @Autowired 34 @Qualifier("memcachedClient") 35 private MemCachedClient memClient; 36 37 public void callAllQuartz(){ 38 List<QuartzVo> list =new ArrayList<QuartzVo>(); 39 Iterator<String> idIter = QuartzVo.quartzMap.keySet().iterator(); 40 String idStr = ""; 41 while(idIter.hasNext()){ 42 idStr = idStr+idIter.next()+","; 43 } 44 logger.info("当前任务有: "+idStr); 45 String flag = ""; 46 Map<String,List<QuartzVo> > map = this.getNewJobs(); 47 Iterator<Entry<String, List<QuartzVo>>> iter = map.entrySet().iterator(); 48 while(iter.hasNext()){ 49 Entry<String, List<QuartzVo>> entry = iter.next(); 50 flag = entry.getKey(); 51 list = entry.getValue(); 52 } 53 //新增 54 if("1".equals(flag)){ 55 logger.info("新增加定时任务的数量:【 "+list.size()+"】"); 56 for(int i =0;i<list.size();i++){ 57 QuartzVo vo = list.get(i); 58 QuartzVo.quartzMap.put(vo.getJobTitle(), vo); 59 QuartzManager.addJob(vo.getJobTitle(), vo, vo.getJobcron()); 60 61 } 62 } 63 else if("2".equals(flag)){ 64 logger.info("删除的定时任务的数量:【 "+list.size()+"】"); 65 for(int i =0;i<list.size();i++){ 66 QuartzVo vo = list.get(i); 67 QuartzManager.removeJob(vo.getJobTitle()); 68 QuartzVo.quartzMap.remove(vo.getJobTitle()); 69 } 70 71 } 72 73 } 74 75 /** 76 * 获取任务 77 * @return 78 * @author xingle 79 * @data 2014-8-14 下午12:59:58 80 */ 81 private Map<String, List<QuartzVo>> getNewJobs() { 82 //返回的map 83 Map<String,List<QuartzVo>> returnMap=new HashMap<String,List<QuartzVo>>(); 84 List<QuartzVo> returnLs = new ArrayList<>(); 85 //文件列表 86 List<QuartzVo> fileLs = new ArrayList<>(); 87 List<String> fileNameLs = new ArrayList<>(); 88 BufferedReader ins = null; 89 File f = new File("D:\test/tasklist1.txt"); 90 try { 91 92 int i = QuartzVo.Id++; 93 ins = new BufferedReader(new FileReader(f)); 94 String line = ""; 95 while ((line = ins.readLine()) != null) { 96 //增加一个是否执行标识,0 未执行 97 //line = line+"|0"; 98 String[] task = line.split("\|"); 99 100 QuartzVo quartzVo = new QuartzVo(); 101 String id = "quarzJob_"+ i; 102 quartzVo.setId(id); 103 quartzVo.setJobTitle(task[0]); 104 quartzVo.setJcallpath(task[1]); 105 quartzVo.setJobcron(task[2]); 106 quartzVo.setS_date(task[3]); 107 quartzVo.setCycle(task[4]); 108 109 fileLs.add(quartzVo); 110 fileNameLs.add(quartzVo.getJobTitle()); 111 } 112 ins.close(); 113 } catch (FileNotFoundException e) { 114 e.printStackTrace(); 115 } catch (IOException e) { 116 e.printStackTrace(); 117 } 118 119 String flag = ""; 120 int fileNum = fileLs.size(); 121 int quarzNum = QuartzVo.quartzMap.size(); 122 if(fileNum > quarzNum){ 123 flag = "1"; 124 for(int i =0;i<fileNum;i++){ 125 QuartzVo fileVo = fileLs.get(i); 126 if(!QuartzVo.quartzMap.keySet().contains(fileVo.getJobTitle())){ 127 QuartzVo.quartzList.add(fileVo); 128 //要增加的 129 returnLs.add(fileVo); 130 } 131 } 132 } 133 else if(fileNum<quarzNum){ 134 flag = "2"; 135 for(int i = 0;i<QuartzVo.quartzList.size() ; i++){ 136 if(!fileNameLs.contains(QuartzVo.quartzList.get(i).getJobTitle())){ 137 //需要要删除的任务 138 returnLs.add(QuartzVo.quartzList.get(i)); 139 } 140 } 141 for(int i = 0;i<QuartzVo.quartzList.size() ; i++){ 142 QuartzVo vo = QuartzVo.quartzList.get(i); 143 for(int j = 0;j<returnLs.size();j++){ 144 if(vo.getId().equals(returnLs.get(j).getId())){ 145 QuartzVo.quartzList.remove(i); 146 } 147 } 148 } 149 } 150 151 returnMap.put(flag, returnLs); 152 return returnMap; 153 } 154 155 }
任务管理:
1 package com.util; 2 3 import java.text.ParseException; 4 import java.text.SimpleDateFormat; 5 import java.util.Date; 6 7 import org.apache.log4j.Logger; 8 import org.quartz.CronTrigger; 9 import org.quartz.JobDetail; 10 import org.quartz.Scheduler; 11 import org.quartz.SchedulerException; 12 import org.quartz.SchedulerFactory; 13 import org.quartz.SimpleTrigger; 14 import org.quartz.impl.StdSchedulerFactory; 15 16 import com.util.vo.QuartzVo; 17 18 19 /** 20 * 任务管理 21 * @ClassName: QuartzManager 22 * 23 * @author Xingle 24 * @date 2014-8-14 下午2:34:16 25 */ 26 public class QuartzManager { 27 private static Logger logger = Logger.getLogger(QuartzManager.class); 28 29 //Create an uninitialized StdSchedulerFactory. 30 private static SchedulerFactory sf = new StdSchedulerFactory(); 31 32 private static String TRIGGER_GROUP_NAME = "quartzTrigger"; 33 34 /** 35 * 添加任务 36 * @param jobName 37 * @param job 38 * @param time 39 * @author xingle 40 * @data 2014-8-14 下午7:45:09 41 */ 42 public static void addJob(String jobName,QuartzVo job,String time){ 43 try { 44 Scheduler scheduler = sf.getScheduler(); 45 46 //任务名,任务组,任务执行类 47 JobDetail jobDetail = new JobDetail(jobName, jobName, job.getClass()); 48 49 if("1".equals(job.getCycle())){//循环 50 CronTrigger trigger = new CronTrigger(jobName, jobName); 51 trigger.setCronExpression(time); 52 scheduler.scheduleJob(jobDetail, trigger); 53 } 54 else{//单次 55 String s_Date = job.getS_date(); 56 logger.debug("*****时间:"+s_Date); 57 58 SimpleDateFormat formate= new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); 59 Date startTime = formate.parse(s_Date); 60 logger.debug("*****时间:"+startTime); 61 SimpleTrigger trigger = new SimpleTrigger(jobName, jobName, startTime); 62 scheduler.scheduleJob(jobDetail, trigger); 63 } 64 if(!scheduler.isShutdown()){ 65 scheduler.start(); 66 } 67 logger.debug("*********【添加】定时任务【"+jobName+"】 加载完成!*****************"); 68 69 70 } catch (SchedulerException e) { 71 e.printStackTrace(); 72 } catch (ParseException e) { 73 e.printStackTrace(); 74 } 75 } 76 77 /** 78 * 删除任务 79 * @param jobName 80 * @author xingle 81 * @data 2014-8-14 下午7:45:16 82 */ 83 public static void removeJob(String jobName){ 84 try { 85 Scheduler sched = sf.getScheduler(); 86 sched.pauseTrigger(jobName,jobName);//停止触发器 87 sched.unscheduleJob(jobName,jobName);//移除触发器 88 sched.deleteJob(jobName, jobName); 89 } catch (SchedulerException e) { 90 e.printStackTrace(); 91 } 92 logger.debug("*********定时任务【"+jobName+"】 已删除完成!*****************"); 93 } 94 95 }
读取的任务简单为下:
任务1|http://www.baidu.com|0 0/1 * * * ?||1
任务4|http://www.iteye.com/problems/99952||2014-08-15 11:34:15|2
任务5|https://pomotodo.com/app/|0 0/1 * * * ?||1
任务3|http://www.w3school.com.cn/html5/|0 0/1 * * * ?||1
任务6|http://tool.oschina.net/apidocs/apidoc?api=jdk-zh|0 0/2 * * * ?||1
其中Cron 触发器的时间设置可以参考有关内容配置,
以上任务可名称不可以重复,任务可随时添加和删除。
执行结果(截取):
中途删除任务3
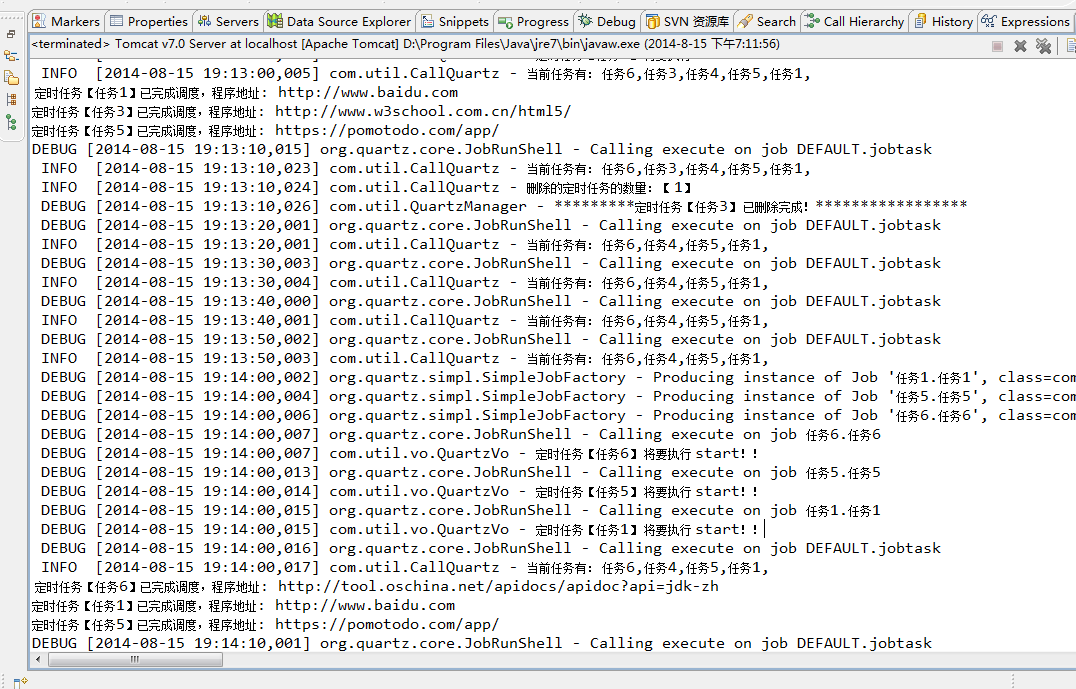