PreparedStatement介绍
- 可以通过调用 Connection 对象的 prepareStatement(String sql) 方法获取 PreparedStatement 对象
- PreparedStatement 接口是 Statement 的子接口,它表示一条预编译过的 SQL 语句
- PreparedStatement 对象所代表的 SQL 语句中的参数用问号(?)来表示(?在SQL中表示占位符),调用 PreparedStatement 对象的 setXxx() 方法来设置这些参数. setXxx() 方法有两个参数,第一个参数是要设置的 SQL 语句中的参数的索引(从 1 开始),第二个是设置的 SQL 语句中的参数的值
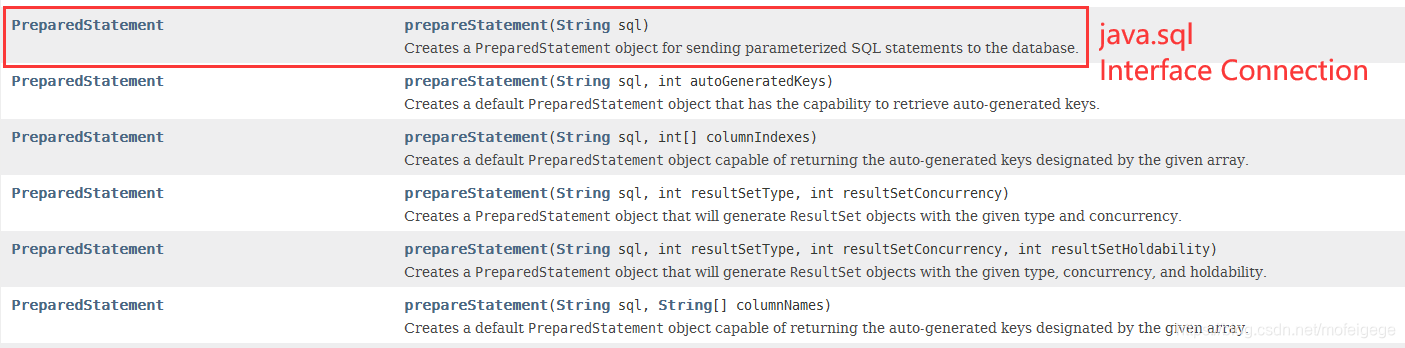
PreparedStatement vs Statement
- 代码的可读性和可维护性。
- PreparedStatement 能最大可能提高性能:
- DBServer会对预编译语句提供性能优化。因为预编译语句有可能被重复调用,所以语句在被DBServer的编译器编译后的执行代码被缓存下来,那么下次调用时只要是相同的预编译语句就不需要编译,只要将参数直接传入编译过的语句执行代码中就会得到执行。
- 在statement语句中,即使是相同操作但因为数据内容不一样,所以整个语句本身不能匹配,没有缓存语句的意义.事实是没有数据库会对普通语句编译后的执行代码缓存。这样每执行一次都要对传入的语句编译一次。
- (语法检查,语义检查,翻译成二进制命令,缓存)
- PreparedStatement 可以防止 SQL 注入
示例:
import a.utils.JDBCutil;
import java.sql.*;
public class sqlzhuru {
private static Connection connection=null;
private static PreparedStatement statement=null;
private static ResultSet resultSet=null;
public static void main(String[] args) throws SQLException {
login("zhansan","123456");
}
public static void login(String name,String pwd) throws SQLException {
try {
connection = JDBCutil.getConnection();
String sql="select * from users WHERE `NAME`=? and `PASSWORD`=?";
statement=connection.prepareStatement(sql);//预编译
//执行sql的对象去执行sql语句
statement.setString(1,name);
statement.setString(2,pwd);
resultSet = statement.executeQuery();
while (resultSet.next()){
System.out.println(resultSet.getObject("NAME"));
System.out.println(resultSet.getObject("PASSWORD"));
}
} catch (SQLException throwable) {
throwable.printStackTrace();
}
finally {
JDBCutil.release(resultSet,statement,connection);
}
}
}
自己编程的数据库工具类JDBCutil
package a.utils;
import java.io.IOException;
import java.io.InputStream;
import java.sql.*;
import java.util.Properties;
public class JDBCutil {
private static String driver=null;
private static String url=null;
private static String pwd=null;
private static String name=null;
static {
try {
InputStream in = JDBCutil.class.getClassLoader().getResourceAsStream("db.properties");
Properties properties = new Properties();
properties.load(in);
driver=properties.getProperty("driver");
url=properties.getProperty("url");
name=properties.getProperty("name");
pwd=properties.getProperty("pwd");
Class.forName(driver);
} catch (ClassNotFoundException | IOException e) {
e.printStackTrace();
}
}
public static Connection getConnection() throws SQLException {
Connection connection = DriverManager.getConnection(url, name, pwd);
return connection;
}
public static void release(ResultSet resultSet, Statement statement,Connection connection) throws SQLException {
//释放连接
if (resultSet!=null)
{
try {
resultSet.close();
} catch (SQLException throwable) {
throwable.printStackTrace();
}
}
if (statement!=null)
{
try {
statement.close();
} catch (SQLException throwable) {
throwable.printStackTrace();
}
}
if (connection!=null)
{
try {
connection.close();
} catch (SQLException throwable) {
throwable.printStackTrace();
}
}
}
}