在使用C++编写halcon之前,确定自己有较好的C++基础,并熟悉一套开发平台如VC
Programmers_guide.pdf chapter7中有关于creating Aplicatin with halcon/c++的详细介绍
以vs2008为例 工具---》选项 (有图介绍不配文字啦)
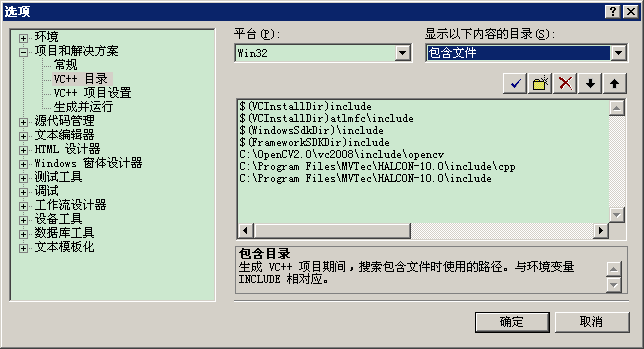
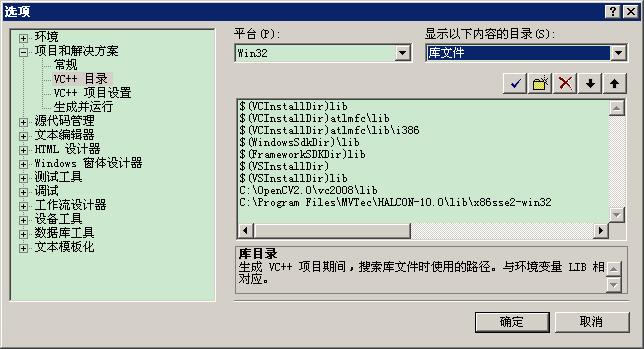
用C++写例子 ~~取狒狒的眼睛


//
#include "stdafx.h"
#include "HalconCpp.h"
#pragma comment(lib,"halconcpp.lib")
int main()
{
using namespace Halcon;
HImage Mandrill("monkey");
HWindow w;
w.Display(Mandrill);
w.Click();
HRegion Bright = (Mandrill >=128); // 取亮度大于128的区域
HRegionArray conn = Bright.Connection();
//取面积在500~9000的区域,狒狒的大鼻子也是大于128的,显然不是我们检测的重点根据面积舍去它
HRegionArray large = conn.SelectShape("area","and",500,9000);
//眼睛是椭圆形的anisometry 是离心率即ra/rb 两半径之比,目测来看取1.2 -1.7
HRegionArray eyes = large.SelectShape("anisometry","and",1.2,1.7);
eyes.Display(w);
w.Click();
return 0;
}//~~ 提取字符


#include "stdafx.h"
#include "HalconCpp.h"
#include <iostream>
#pragma comment(lib,"halconcpp.lib")
using namespace std;
using namespace Halcon;
int main()
{
HImage image("alpha1");
HRegion region;
HWindow w;
long threshold;
image.Display(w);
w.Click();
// image.GetDomain -- 获得图像region
//sigma --- 高斯平滑系数
//Percent --- 前景背景灰度不同百分比 95 代表 至多有5%是最大灰度值
region = image.CharThreshold(image.GetDomain(),2,95,&threshold);
image.Display(w);
region.Display(w);
w.Click();
cout<<"Threshold for 'alpha': "<<threshold;
w.Click();
return 0;
}注意:参考手册中的操作符不全,A complete list can be found in the file includecppHCPPGlobal.h.
1.关于参数:
①.halcon的输入参数 (input parameters)不会被操作符改变,也就是说是按值传递的
如下代码示例
HImage original_image("monkey"), smoothed_image;
smoothed_image = original_image.MeanImage(11, 11);
meanimage不会改变original_image,而中值后的图像保存在返回值 smoothed_image中
②.与之相反的是输出参数(output parameters),所以传的是指针或者引用
2.halcon /C++关于面向对象和过程
这是通过类来调用方法的 ,面向对象方式
HImage image("barcode/ean13/ean1301");
HTuple paramName, paramValue;
HBarCode barcode(paramName, paramValue);
HRegionArray code_region;
HTuple result;
code_region = barcode.FindBarCode(image, "EAN-13", &result);
code_region = image.FindBarCode(barcode, "EAN-13", &result);
面向过程的方式
Hobject image;
HTuple barcode;
Hobject code_region;
HTuple result;
read_image(&image, "barcode/ean13/ean1301");
create_bar_code_model(HTuple(), HTuple(), &barcode);
find_bar_code(image, &code_region, barcode, "EAN-13", &result);3.halcon/c++所有的 对象 可以在C:Program FilesMVTecHALCON-10.0includecpp 中找到 (以halcon安装在C盘为例)
4.halcon/c++的数组模式
我们可以用操作符来 打开多个图片 region 等等 看该操作是否支持 数组模式 要看它的定义了
如图所示

数组的几点说明:
① 创建,初始化数组
C++ 可以通过 []
C 通过 gen_empty_obj 增加数组 concat_obj
②操纵对象
C++ 通过[N] ,统计数组元素个数 num()
C select_obj count_obj
③iconic 数组以 0 开始 , Hobject 数组 以 1 开始
④通过例子说明第四点
#include "stdafx.h"
#include "HalconCpp.h"
#include <iostream>
#pragma comment(lib,"halconcpp.lib")
using namespace std;
using namespace Halcon;
//~~ 提取字符
int main()
{
HImageArray images;
HRegionArray regions;
HTuple thresholds;
HWindow w;
for (int i = 1; i <=2;++i)
{
//HTuple("alpha") +i 的解释
//HTuple 中有对 operater + 的重载
//HTuple("alpha") +i ~~~ alpha1 alpha2.....
images[i-1] = HImage::ReadImage(HTuple("alpha") +i);
}
//这一句相当于对 images中所有的对象进行了 charthreshold操作
regions = images.CharThreshold(images[0].GetDomain(),2,95,&thresholds);
for (int i = 0 ; i<regions.Num();++i)
{
images[i].Display(w);
w.Click();
regions[i].Display(w);
w.Click();
cout<<"threshold : "<<thresholds[i].L()<<endl;
}
w.Click();
return 0;
}
5. 关于参数转换
• Converting Hobject into iconic parameter classes
Hobject p_image;
read_image(&p_image, "barcode/ean13/ean1301");
HImage o_image(p_image);
Iconic parameters can be converted from Hobject to, e.g., HImage simply by calling the construc-
tor with the procedural variable as a parameter.
• Converting handles into handle classes
HTuple p_barcode;
create_bar_code_model(HTuple(), HTuple(), &p_barcode);
HBarCode o_barcode;
o_barcode.SetHandle(p_barcode[0]);
o_code_region = o_barcode.FindBarCode(o_image, "EAN-13", &result);
Handles cannot be converted directly via a constructor; instead, you call the method SetHandle()
with the procedural handle as a parameter.
• Converting handle classes into handles
p_barcode = o_barcode.GetHandle();
Similarly, a handle can be extracted from the corresponding class via the method GetHandle().
You can even omit the method, as the handle classes provide cast operators which convert them
automatically into handles.
p_barcode = o_barcode;
•Converting iconic parameter classes into Hobject
Hobject p_code_region = o_code_region.Id();
Iconic parameters can be converted from classes like HRegion back into Hobject via the method
Id(). In contrast to the handle classes no cast operator is provided.
• Converting HWindow into a window handle
long p_window;
open_window(0, 0, width/2, height/2, 0, "visible", "", &p_window);
HWindow o_window(0, 0, 100, 100, 0, "visible", "");
p_window = o_window.WindowHandle();
disp_obj(p_code_region, p_window);
In contrast to other handles, procedural window handles cannot be converted into instances of the
class HWindow! However, you can extract the handle from an instance of HWindow via the method
WindowHandle().
6 . The halcon Parameter classes
6.1 Iconic Objects
基类为Hobject
HImage ,HRegion,HXLD 基类为Hobject
6.1.1 Reions
region 是图像平面的一组坐标点 , 它可能不是连接的,可能含有洞,它可以比图像还大。
通过一个例子来了解HRegion
#include "stdafx.h"
#include "HalconCpp.h"
#include <iostream>
#pragma comment(lib,"halconcpp.lib")
using namespace std;
using namespace Halcon;
/*
例子介绍了region的用法 ,更详细的介绍在 progarma_guide chapter6.1.1
*/
int main()
{
HImage image("mreut");
HRegion region;
HWindow w;
region = image >=190;
w.SetColor("red");
region.Display(w);
w.Click();
//region.Fillup() 调用方法
HRegion filled = region.FillUp();
filled.Display(w);
w.Click();
//运算符重载 开运算 >> 腐蚀 <<膨胀 , 半径4.5
HRegion open = (filled >>4.5)<<4.5;
w.SetColor("green");
open.Display(w);
w.Click();
w.ClearWindow();
//平移
HDPoint2D trans(-100 , -150);
HRegion moved = open + trans;
HRegion zoomed = moved * 2.0;
zoomed.Display(w);
w.Click();
return 0;
}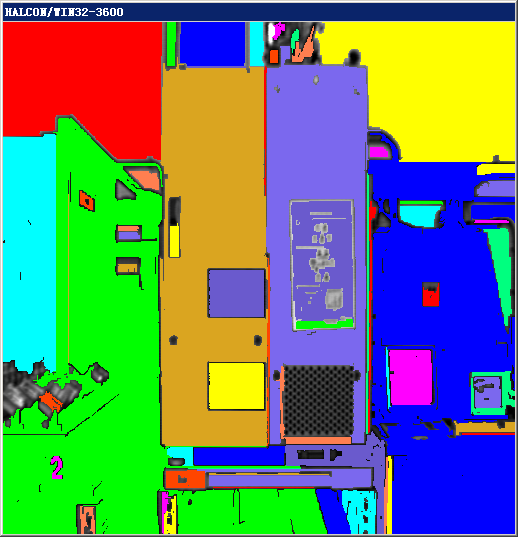
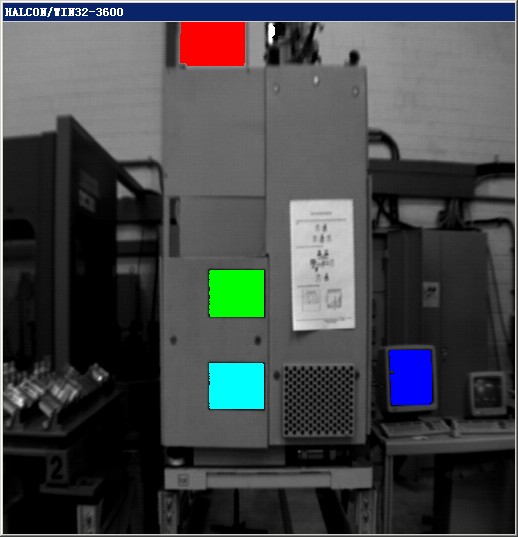
#include "stdafx.h"
#include "HalconCpp.h"
#include <iostream>
#pragma comment(lib,"halconcpp.lib")
using namespace std;
using namespace Halcon;
/*
例子介绍了regionArry的用法
*/
int main()
{
HImage image("fabrik");
// 1 1 row col 越小越精细
// 4 容差 种子和被检测像素值差
// 100 最小region size
HRegionArray regs = image.Regiongrowing(1,1,4,100);
HWindow w;
w.SetColored(12);
image.Display(w);
w.Click();
regs.Display(w);
w.Click();
HRegionArray rect;
for (int i = 0 ; i < regs.Num() ; ++ i )
{
// compactness ~~ 在图像处理中经常叫做圆形度 而这里用作 region的紧密度
//C = L^2 / (4 F pi)) L = 周长 F = 面积
//C = 1 是 为圆 ,region 较长是 或者region 有洞洞时 C >1
//这里就是得出了 比较方正的 没小洞洞的 region
if ( (regs[i].Area() > 1000) && ( regs[i].Compactness() < 1.5) )
rect.Append(regs[i]); //RegionArry 添加元素哦
}
image.Display(w);
rect.Display(w);
w.Click();
return 0;
}
6.1.2 Images
#include "stdafx.h"
#include "HalconCpp.h"
#include <iostream>
#pragma comment(lib,"halconcpp.lib")
using namespace std;
using namespace Halcon;
/*
drawregion 画感兴趣区域
meanimage 做个中值滤波
和中值滤波前图像比较
*/
int main()
{
HImage image("mreut");
HWindow w;
image.Display(w);
cout<< " width = " << image.Width()<<endl;
cout << " height = " << image.Height() <<endl;
HRegion mask= w.DrawRegion();
// & 重载 取感兴趣区域的图像
HImage reduced = image & mask;
w.ClearWindow();
reduced.Display(w);
w.Click() ;
HImage mean = reduced.MeanImage(61,61);
mean.Display(w);
HRegion reg = reduced.DynThreshold(mean,3,"light");
reg.Display(w);
w.Click();
return 0;
}
6.1.2.2 像素值
操纵像素的两种方法
int main()
{
HByteImage in("mreut");
HByteImage out = in ;
HWindow w;
in.Display(w);
w.Click() ;
int nwidht = in.Width();
int nheight = in.Height();
long end = nwidht * nheight;
for (int k = 0 ; k < end ; k++)
{
//方法1
//int pix = in.GetPixVal(k);
//out.SetPixVal(k,255 - pix);
//方法2
out[k]= 255 - in[k];
}
out.Display(w);
w.Click();
return 0;
}6.1.3 XLD Objects
XLD is the abbreviation for eXtended Line Description. This is a data structure used for describing areas
(e.g., arbitrarily sized regions or polygons) or any closed or open contour, i.e., also lines. In contrast to
regions, which represent all areas at pixel precision, XLD objects provide subpixel precision. There are
two basic XLD structures: contours and polygons.
Similarly to images, HALCON/C++ provides both a base class HXLD and a set of specialized classes
derived from HXLD, e.g., HXLDCont for contours or HXLDPoly for polygons. For all classes there exists
a corresponding container class, e.g., HXLDArray.
In contrast to the classes described in the previous sections, the XLD classes provide only member
functions corresponding to HALCON operators (see also section 5.2.2 on page 35).
6.1.4 Low - level iconic objects 底层iconic 对象
the class Hobject is used for all iconic parameters, be it an image, a region, or even an image array
In fact, the class Hobject is HALCON’s basic class for accessing the internal data management
Furthermore, Hobject serves as the basis for the class HObject and the derived classes, e.g., HImage.
As noted above, an instance of Hobject can also contain a tuple (array) of iconic objects. Unfortunately,
Hobject provides no special member functions to add objects or select them; instead, you must use the
operators gen_empty_obj, concat_obj, select_obj, and count_obj 。
一个Hobject实例可以包含一组iconic 对象,但Hobject 没有提供增加或选择它们的成员函数,所以必须通过
gen_empty_obj, concat_obj, select_obj, and count_obj 来解决。
6.2 Control parameters