单例模式:系统运行时,有且仅有一个实例
- 一个类只有一个实例——最基本的
只提供私有构造器,构造方法私有化
2. 它必须自行创建这个实例
定义了静态的该类私有对象
3. 它必须自行向整个系统提供这个实例
提供了一个静态的公有方法,返回创建或者获取本身的静态私有对象
一. 懒汉模式:在类加载时不创建实例,采用延迟加载的方式,在运行调用时创建实例
特点:线程不安全
延迟加载(lazy Loading)
所用资源少
如何解决:同步(synchronized)
加锁
二. 饿汉模式:在类加载的时候,就完成了初始化
特点:线程安全,不具备延迟加载特性
两者对比:
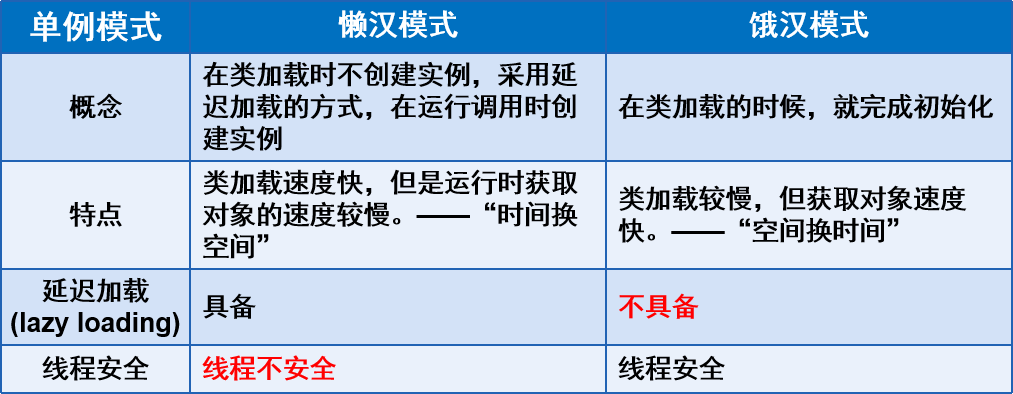
1 package cn.pojo; 2 3 import java.sql.Connection; 4 import java.sql.DriverManager; 5 import java.sql.SQLException; 6 7 /** 8 * 没有撤退可言 9 **/ 10 public class BaseDao1 { 11 private String url="jdbc:mysql://location:3306/wxy"; 12 private String driName="com.mysql.jdbc.Driver"; 13 private String dbname="root"; 14 private String dbpwd="1766241225"; 15 private static Connection conn=null; 16 private static BaseDao1 db=null; 17 18 private BaseDao1(){ 19 try { 20 Class.forName(driName); 21 conn= DriverManager.getConnection(url,dbname,dbpwd); 22 }catch (SQLException e){ 23 e.printStackTrace(); 24 }catch (ClassNotFoundException e){ 25 e.printStackTrace(); 26 } 27 } 28 29 // 懒汉模式+线程安全 30 public static synchronized Connection getConn(){ 31 if (conn==null){ 32 new BaseDao1(); 33 } 34 return conn; 35 } 36 // 静态内部类 37 public static class BaseDaoHelper{ 38 private static final BaseDao1 db=new BaseDao1(); 39 } 40 // 饿汉单例+延迟加载 41 public static BaseDao1 getbd(){ 42 if (db==null){ 43 db=BaseDaoHelper.db; 44 } 45 return db; 46 } 47 }
指示符之转发和重定向
重定向:return"redirect:/url请求"
转发:"forward"
使用servler API对象入参
Servler API对象作为处理方法的入参
HttpSession
HttpServletRequest
Spring MVC异常处理
异常处理
HandlerExceptionResolver
resolveException()
局部异常处理
仅能处理指定的Controller中的异常
@ExceptionHandler
全局异常处理
对所有异常进行统一处理
配置 SimpleMappingExceptionResolver
发生异常时使用对应的视图报告异常
1 <?xml version="1.0" encoding="UTF-8"?> 2 <beans xmlns="http://www.springframework.org/schema/beans" 3 xmlns:context="http://www.springframework.org/schema/context" 4 xmlns:p="http://www.springframework.org/schema/p" 5 xmlns:mvc="http://www.springframework.org/schema/mvc" 6 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 7 xsi:schemaLocation="http://www.springframework.org/schema/beans 8 http://www.springframework.org/schema/beans/spring-beans-3.0.xsd 9 http://www.springframework.org/schema/context 10 http://www.springframework.org/schema/context/spring-context.xsd 11 http://www.springframework.org/schema/mvc 12 http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd"> 13 <!--使用springMVC注解--> 14 15 <mvc:annotation-driven/> 16 <context:component-scan base-package="cn.controller"/> 17 <bean class="org.springframework.web.servlet.mvc.annotation.AnnotationMethodHandlerAdapter"/> 18 <bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> 19 <property name="prefix" value="/"></property> 20 <property name="suffix" value=".jsp"></property> 21 </bean> 22 <bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver"> 23 <property name="exceptionMappings"> 24 <props> 25 <prop key="java.lang.RuntimeException">异常跳转显示页面</prop> 26 </props> 27 </property> 28 </bean> 29 </beans>