92. 反转链表 II
给你单链表的头节点
head
和两个整数 left
和 right
,其中 left <= right
。请你反转从位置 left
到位置 right
的链表节点,返回 反转后的链表 。
示例 1:
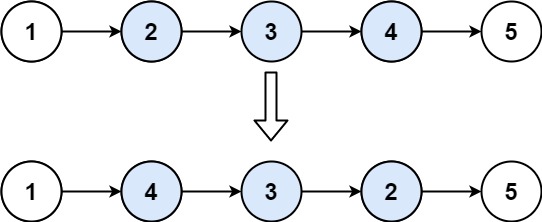
输入:head = [1,2,3,4,5], left = 2, right = 4 输出:[1,4,3,2,5]
示例 2:
输入:head = [5], left = 1, right = 1 输出:[5]
提示:
- 链表中节点数目为
n
1 <= n <= 500
-500 <= Node.val <= 500
1 <= left <= right <= n
直接拿图举例,首先建立一个头结点,找到要反转的一段,记录下1这个结点,和5这个结点,然后切断1->4 , 2->5 ,将 4->3->2 反转,改变指针方向变为 4<-3<-2,然后利用记录下的结点,将1连接4,2连接5,完成,返回虚拟头结点的下一个结点。
/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode() : val(0), next(nullptr) {} * ListNode(int x) : val(x), next(nullptr) {} * ListNode(int x, ListNode *next) : val(x), next(next) {} * }; */ class Solution { public: ListNode * relist(ListNode * head) { ListNode * pre = nullptr; ListNode * cur = head; ListNode * tmp; while(cur) { tmp = cur->next; cur->next = pre; pre = cur; cur = tmp; } return pre; } public: ListNode* reverseBetween(ListNode* head, int left, int right) { ListNode * newhead = new ListNode(0); newhead->next = head; ListNode * cur = newhead; ListNode * end = newhead; ListNode * pre = newhead; for(int i = 0 ; i < left - 1 ; i++) pre = pre->next; for(int i = 0 ; i < right ; i++) end = end->next; ListNode * ls = pre->next; ListNode * re = end->next; pre->next = nullptr; end->next = nullptr; pre->next = relist(ls); ls->next = re; return newhead->next; } };