Fox Ciel is playing a mobile puzzle game called "Two Dots". The basic levels are played on a board of size n × m cells, like this:
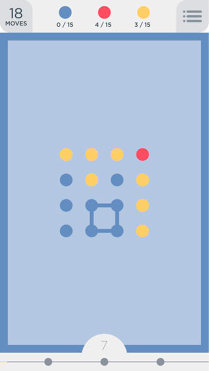
Each cell contains a dot that has some color. We will use different uppercase Latin characters to express different colors.
The key of this game is to find a cycle that contain dots of same color. Consider 4 blue dots on the picture forming a circle as an example. Formally, we call a sequence of dots d1, d2, ..., dk a cycle if and only if it meets the following condition:
- These k dots are different: if i ≠ j then di is different from dj.
- k is at least 4.
- All dots belong to the same color.
- For all 1 ≤ i ≤ k - 1: di and di + 1 are adjacent. Also, dk and d1 should also be adjacent. Cells x and y are called adjacent if they share an edge.
Determine if there exists a cycle on the field.
The first line contains two integers n and m (2 ≤ n, m ≤ 50): the number of rows and columns of the board.
Then n lines follow, each line contains a string consisting of m characters, expressing colors of dots in each line. Each character is an uppercase Latin letter.
Output "Yes" if there exists a cycle, and "No" otherwise.
3 4
AAAA
ABCA
AAAA
Yes
3 4
AAAA
ABCA
AADA
No
4 4
YYYR
BYBY
BBBY
BBBY
Yes
7 6
AAAAAB
ABBBAB
ABAAAB
ABABBB
ABAAAB
ABBBAB
AAAAAB
Yes
2 13
ABCDEFGHIJKLM
NOPQRSTUVWXYZ
No
In first sample test all 'A' form a cycle.
In second sample there is no such cycle.
The third sample is displayed on the picture above ('Y' = Yellow, 'B' = Blue, 'R' = Red).
给定n*m的方格,每个小格子里有A~Z的字母,代表一种颜色,问这个n*m的方格里是否包含一个颜色相同的环.
枚举方格中的每一个格子以其作为起点进行深度优先遍历,看是否能回到起点,能的话就能找到这么一个环,否则就不行.
判断是否回到起点的一个小技巧是:先记录下起点位置,深搜的过程将路径给'堵'住,如果到达一个"新位置"如果可以到达的
下一位置是起点,并且颜色相同.那么这个环存在.这样做的目的是为了防止递归回溯的时候会"误判"下一位置是起点从而认为存在环.
需要注意一点是路径长度要大于3,故还需要记录长度
#include <iostream> using namespace std; int count; int n,m; bool flag=false; int dir1[4]={1,-1,0,0}; int dir2[4]={0,0,1,-1}; int use[100][100]; char map[100][100]; int x,y; int begin,end; #include <string.h> bool jud(int i,int j) { if(i<=0||i>n||j<=0||j>m) return false; return true; } void dfs(int a,int b,int k) { if(flag) return; for(int i=0;i<4;i++) { if(a+dir1[i]==begin&&b+dir2[i]==end&&use[a][b]==0&&(int)map[a+dir1[i]][b+dir2[i]]==k&&count>=3) { flag=true; break; } if((int)map[a+dir1[i]][b+dir2[i]]==k&&use[a+dir1[i]][b+dir2[i]]==0&&jud(a+dir1[i],b+dir2[i])) { use[a][b]=1; count++; dfs(a+dir1[i],b+dir2[i],k); count--; } } } int main() { cin>>n>>m; x=y=0; memset(map,'.',sizeof(map)); for(int i=1;i<=n;i++) { for(int j=1;j<=m;j++) { cin>>map[i][j]; } } for(int i=1;i<=n;i++) { for(int j=1;j<=m;j++) { memset(use,0,sizeof(use)); begin=i; end=j; count=0; dfs(i,j,(int)map[i][j]); } } if(flag) cout<<"Yes"<<endl; else cout<<"No"<<endl; return 0; }