Strategic game
Time Limit: 2000MS | Memory Limit: 10000K | |
Total Submissions: 9582 | Accepted: 4516 |
Description
Bob enjoys playing computer games, especially strategic games, but sometimes he cannot find the solution fast enough and then he is very sad. Now he has the following problem. He must defend a medieval city, the roads of which form a tree. He has to put the minimum number of soldiers on the nodes so that they can observe all the edges. Can you help him?
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
For example for the tree:
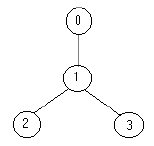
the solution is one soldier ( at the node 1).
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
For example for the tree:
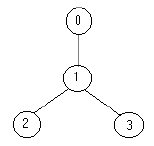
the solution is one soldier ( at the node 1).
Input
The input contains several data sets in text format. Each data set represents a tree with the following description:
The node identifiers are integer numbers between 0 and n-1, for n nodes (0 < n <= 1500);the number_of_roads in each line of input will no more than 10. Every edge appears only once in the input data.
- the number of nodes
- the description of each node in the following format
node_identifier:(number_of_roads) node_identifier1 node_identifier2 ... node_identifiernumber_of_roads
or
node_identifier:(0)
The node identifiers are integer numbers between 0 and n-1, for n nodes (0 < n <= 1500);the number_of_roads in each line of input will no more than 10. Every edge appears only once in the input data.
Output
The output should be printed on the standard output. For each given input data set, print one integer number in a single line that gives the result (the minimum number of soldiers). An example is given in the following:
Sample Input
4 0:(1) 1 1:(2) 2 3 2:(0) 3:(0) 5 3:(3) 1 4 2 1:(1) 0 2:(0) 0:(0) 4:(0)
Sample Output
1 2
Source
题意:
给定一棵n个节点的树,如果在一个节点上放上一个士兵,所有与这个节点相连的边都可以被看守。现在希望所有的边都可以被看守,问需要最少放多少士兵。
思路:
比较典型的一个树形DP,我们用dp[i]表示以i为根的子树的最少士兵数。但是我们并不知道i上有没有士兵,转移方程就写不出来。
所以我们给dp再加一维,dp[i][1]表示以i为根并且i上有士兵的子树的最少士兵数,dp[i][0]为i上没有士兵。
那么对于某个节点rt,假设他的所有孩子的dp均已得到。
那么dp[rt][0] = dp[son][1]之和,因为他的每一个孩子都要有一个士兵。
dp[rt][1] = min(dp[son][0], dp[son][1])之和,即他的每一个孩子可以放士兵也可以不放士兵。
随便取一个节点作为树根,最后输出这个节点dp[rt][0],dp[rt][1]的较小值即可。
1 //#include <bits/stdc++.h> 2 #include<iostream> 3 #include<cmath> 4 #include<algorithm> 5 #include<stdio.h> 6 #include<cstring> 7 #include<vector> 8 #include<map> 9 #include<set> 10 11 #define inf 0x3f3f3f3f 12 using namespace std; 13 typedef long long LL; 14 15 int n; 16 const int maxn = 1505; 17 vector<int>edge[maxn]; 18 int dp[maxn][2]; 19 20 void dfsdp(int rt, int fa) 21 { 22 dp[rt][0] = 0; 23 dp[rt][1] = 1; 24 for(int i = 0; i < edge[rt].size(); i++){ 25 int son = edge[rt][i]; 26 if(son == fa)continue; 27 else dfsdp(son, rt); 28 dp[rt][0] += dp[son][1]; 29 dp[rt][1] += min(dp[son][0], dp[son][1]); 30 } 31 32 } 33 34 int main(){ 35 36 while(scanf("%d", &n) != EOF){ 37 for(int i = 1; i <= n; i++){ 38 edge[i].clear(); 39 } 40 for(int i = 1; i <= n; i++){ 41 int u, num; 42 scanf("%d:(%d)", &u, &num); 43 for(int j = 1; j <= num; j++){ 44 int v; 45 scanf(" %d", &v); 46 edge[u + 1].push_back(v + 1); 47 edge[v + 1].push_back(u + 1); 48 } 49 } 50 51 dfsdp(1, 0); 52 printf("%d ", min(dp[1][1], dp[1][0])); 53 } 54 return 0; 55 }