1.
#王者荣耀选择对战模式及人物游戏
class king:
#变量初始化,后面可以调用初始化变量更简单(战力,技能也可以)
# def __init__(self):
# self.dianwei = '[1] 典韦'
# self.zhaoyun = '[2] 赵云'
# self.luban = '[3] 鲁班'
def chooice(self):
chooice_ = input('请选择对战模式:')
a = '人机对战'
b = '多人对战'
if chooice_ == a :
print('进入人机对战模式')
self.people_machine()
else:
print('多人对战正在建设!')
def people_machine(self):
print('请选择人物:')
chooice_people = input()
a = '典韦'
b = '赵云'
c = '鲁班'
if chooice_people == a:
print('您选择的是典韦!')
self.dianwei()
elif chooice_people == b:
print('您选择的是赵云!')
self.zhaoyun()
elif chooice_people == c:
print('您选择的是鲁班!')
self.luban()
else:
print('不懂你的选择!')
self.people_machine()
def dianwei(self):
print('典韦战斗力:200')
print('典韦技能:杀!')
print('典韦防御力:300')
print('人物确定!')
self.random_people()
def zhaoyun(self):
print('赵云战斗力:120')
print('赵云技能:杀!')
print('赵云防御力:300')
self.random_people()
def luban(self):
print('鲁班战斗力:120')
print('鲁班技能:杀!')
print('鲁班防御力:300')
self.random_people()
def random_people(self):
import numpy as np
res = np.random.choice(['典韦','赵云','鲁班'])
print('和你对战的是: {}'.format(res))
def start(self):
print('正在加载,请稍等!')
if __name__ == '__main__':
start = king()
start.chooice()
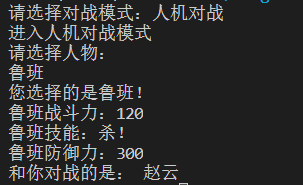
2.
import requests
import re
response = requests.get('http://www.89ip.cn')
HTML = response.text
compile_=re.compile(r'(((2(5[0-5]|[0-4]d))|[0-1]?d{1,2})(.((2(5[0-5]|[0-4]d))|[0-1]?d{1,2})){3})')
res = compile_.findall(str_)
for ip_ in res:
print(ip_[0])
compile_ = re.compile('s+(d{3,6})s+</td>')
res = compile_.findall(str_)
print(res,len(res))
compile_1 = re.compile('s+(d{4}/d{2}/d{2}s+d{2}:d{2}:d{2})s+</td>')
res1 = compile_1.findall(str_)
print(res1,len(res))
compile_2 = re.compile('s+([u4e00-u9fa5]{1,9}[?:省|市|县])s+</td>')
res2 =compile_2.findall(str_)
print(res2)
compile_3 = re.compile('s+([u4e00-u9fa5]{1,9}[?:省|市|县])s+</td>')
res3 =compile_3.findall(str_)
print(res3)
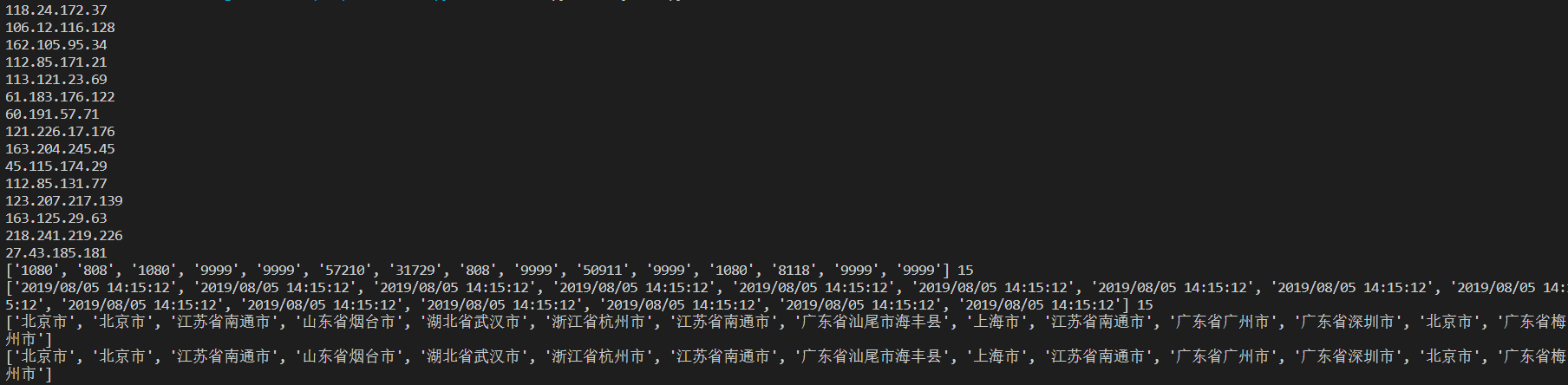
3.
class Account:
def __init__(self):
self._id = 1122
self._balance = 20000
self.annuallInterestRate = 4.5
self.getMonthlyInterest()
def getMonthlyInterestRate(self):
pass
def getMonthlyInterest(self):
#balance >金额
#monthlyInterestRate >月利息
self.monthlyInterestRate = self.annuallInterestRate / 100 / 12
self.M = self._balance * self.monthlyInterestRate
print(self.M)
def whitdraw(self):
qu_monoy = int(input('请输入取款数:'))
self.sheng_monoy = self._balance - qu_monoy
print('账户还剩:{}'.format(self.sheng_monoy))
self.deposit()
def deposit(self):
cun_monoy = int(input('请输入存款数:'))
sheng_monoy1 = self.sheng_monoy + cun_monoy
print('账户还剩:{}'.format(sheng_monoy1))
def xi(self):
print('用户id为:{}'.format(self._id))
print('月利率为:{}'.format(self.monthlyInterestRate))
print('月利息为:{}'.format(self.M))
if __name__ == '__main__':
a = Account()
a.getMonthlyInterest()
a.whitdraw()
a.xi()
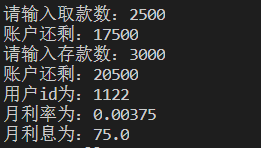
4.
import math
class RegularPolygon:
def qq(self,_n,_side,_x,_y):
self._n = int(_n)
self._side = float(_side)
self._x = float(_x)
self._y = float(_y)
def getArea(self):
area = (self._n * (self._side ** 2)) / (4 * math.tan(3.14/self._n))
print('多边型面积为:{}'.format(area))
def getPerimeter(self):
perimeter = self._side * self._n
print('多边型周长为:{}'.format(perimeter))
if __name__ == '__main__':
R1 = RegularPolygon()
R1.qq(10,4.5,6.7,8)
R1.getArea()
R1.getPerimeter()
