昨天简单的学习了一些python的一些简单的语句与python的数据类型,今天继续学习python的基础语句 if 语句。
一、if 语句
- if 语句语法
if expression:
ifSuite
else:
elseSuite
如果表达式expression的值为非0或者为True,则代码组ifSuite将会被执行,否则执行elseSuite代码组。其实这里跟java的语法差不多,但是这里expression可以直接写数据类型。
- 数字,只要是非0,则会被认为是True。
- 字符串 只要是非“”, 都会被认为是True。
- 列表 只要非[], 都会被认为是True。
- 元组 只要非(), 都会被认为是True。
- 字典 只要非{}, 都会被认为是True。
- None 也为假
# -*- coding: utf-8 -*- ''' Created on 2018年12月17日 @author: Herrt灬凌夜 ''' if 0.1 : print("真"); if 0 : print("真"); else : print("假"); if "" : print("真"); else : print("假"); if " " : print("真"); else : print("假"); if [] : print("真"); else : print("假"); if () : print("真"); else : print("假"); if {} : print("真"); else : print("假"); if None : print("真"); else : print("假"); if "s" in "sdas" : print("真");
以下为上述if语句的执行结果:
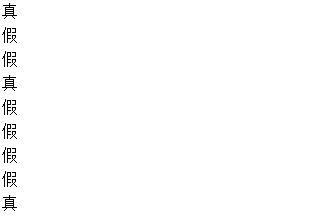
最后写一个简单的例子:
1.提示用户输入用户名密码
2.若用户名为"tom",密码为123456则返回Login否则返回error
# -*- coding: utf-8 -*- ''' Created on 2018年12月17日 1.提示用户输入用户名密码 2.若用户名为"tom",密码为123456则返回Login否则返回error @author: Herrt灬凌夜 ''' userName = input("请输入用户名:"); passWord = input("请输入密码:"); if userName == "tom" and passWord == "123456": print("Login"); else : print("error");
二、if elif 语句
- if elif 语句语法
if expression1 : ifSuite elif expression2 : elifSuite else : elseSuite
if elif 语句就相当于java语言中if else 语句一样,主要是用于多条件判断语句。我们以一个简单的实例来看这个语句。
1.输入一个数字,如果这个数字大于0,输出正数,如果小于0,输出负数,如果等于0则输出0
# -*- coding: utf-8 -*- ''' Created on 2018年12月17日 输入一个数字,如果这个数字大于0,输出正数,如果小于0,输出负数,如果等于0则输出0 @author: Herrt灬凌夜 ''' numStr = int(input("请输入一个数字:")); if numStr > 0 : print("正数"); elif numStr < 0 : print("负数"); else : print("0");
三、一些简单的例子
- 根据用户输入的成绩,输出,如果大于60则输出“及格”,如果大于70则输出“良好”,如果大于80则输出“好”, 如果大于90则输出优秀,否则输出“不及格”
# -*- coding: utf-8 -*- ''' Created on 2018年12月17日 根据用户输入的成绩,输出,如果大于60则输出“及格”,如果大于70则输出“良好”,如果大于80则输出“好”, 如果大于90则输出优秀,否则输出“不及格” @author: Herrt灬凌夜 ''' grade = int(input("请输入成绩")); if grade < 0 or grade > 100 : print("输入成绩有误!"); elif grade > 60 and grade <= 70 : print("及格"); elif grade > 70 and grade <= 80 : print("良好"); elif grade > 80 and grade <= 90 : print("好"); elif grade > 90 : print("优秀"); else : print("不及格");
- 写一个人机交互的猜拳游戏
# -*- coding: utf-8 -*- ''' Created on 2018年12月17日 猜拳游戏 @author: Herrt灬凌夜 ''' import random; computer = random.choice(["石头", "剪刀", "布"]); person = input("请出拳(石头,剪刀,布):"); if person == "石头" and computer == "石头" : print("平局"); elif person == "剪刀" and computer == "剪刀" : print("平局"); elif person == "布" and computer == "布" : print("平局"); elif person == "石头" and computer == "剪刀" : print("你赢了"); elif person == "剪刀" and computer == "布" : print("你赢了"); elif person == "布" and computer == "石头" : print("你赢了"); elif person == "石头" and computer == "剪刀" : print("你输了"); elif person == "剪刀" and computer == "布" : print("你输了"); elif person == "布" and computer == "石头" : print("你输了");
上述代码虽然可以实现猜拳游戏,但是显得非常的啰嗦,我们对上述代码进行优化,并且可以让用户有更好的体验。
# -*- coding: utf-8 -*- ''' Created on 2018年12月17日 猜拳游戏 @author: Herrt灬凌夜 ''' import random; computer = random.choice(["石头", "剪刀", "布"]); winList = [["石头", "剪刀"], ["剪刀", "布"], ["布", "石头"]]; personList = ["石头", "剪刀", "布"]; hint = """ 请出拳: (0)石头 (1)剪刀 (3)布 """; #获取下标 person = int(input(hint)); #字符串输出格式化 print("你出了%s,电脑出了%s" % (personList[person], computer)); if personList[person] == computer : #