项目的model一旦多了起来,复杂了起来,我们很自然的想到使用mybatis的逆向工程来生成相应的pojo和mapper,能节省很多精力。
MyBatis Generator(MBG)是 MyBatis 和 iBATIS 的代码生成工具。它可以为所有 MyBatis
版本以及 iBATIS 版本 2.2.0 及以上自动生成代码。
它会逆向查找一张或多张数据库表的信息,生成操作数据库表所需要的组件。基本上省去了
自已手动创建实体类以及配置文件的麻烦。
MBG 只是对单表的增删改查(CRUD (Create, Retrieve, Update, Delete))生成了大部分的代
码,对于像连接查询或者存储过程之类的,还是需要手动编写 sql 和实体类的。MBG 会生成对应于表结构的 java POJO 类。包括一个支持动态查询、更新和删除的类。
MBG 为单表的增删改查生成了配置文件和映射文件。
生成的 SQL 语句包括:
insert
update by primary key
update by example (使用动态 where 子句)
delete by primary key
delete by example (使用动态 where 子句)
select by primary key
select by example (使用动态 where 子句)
select by exampleWithBLOBS (含有大字段(text)的where)
count by example
mybatis的排序问题
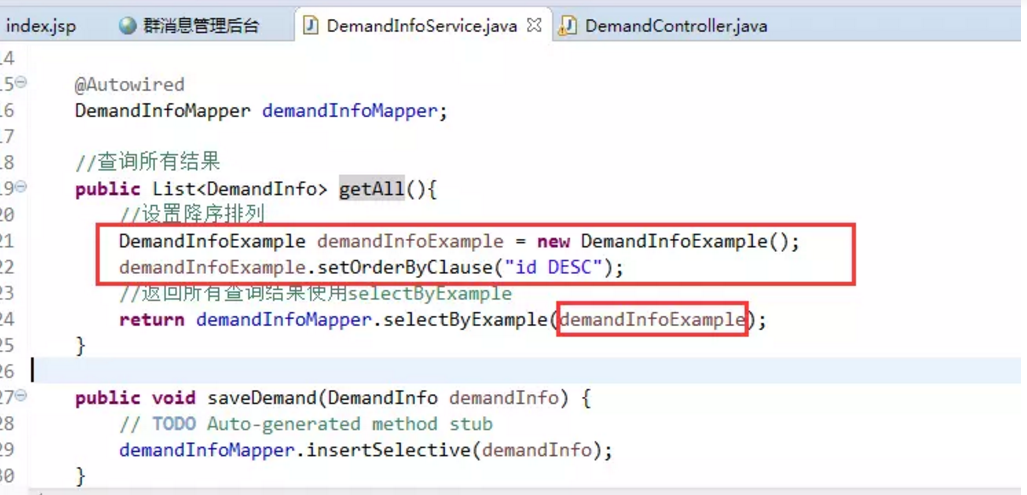
根据表结构的不同,这些语句会有一些变化,比如有的表没有主键,则 MBG 不会生成根据主键更新表的记录的方法。
2 Mybatis Generator 的使用
2.1 建立 MybatisGenerator 项目
建立 Java 项目即可
2.2 添加 jar 包支持
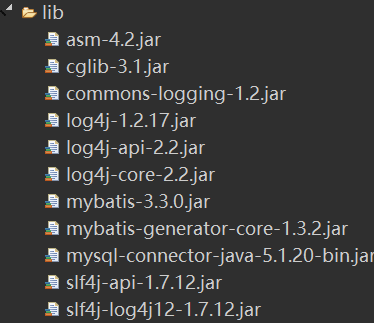
2.3 建立配置文件
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration> <context id="testTables" targetRuntime="MyBatis3"> <commentGenerator> <!-- 是否去除自动生成的注释 true:是 : false:否 --> <property name="suppressAllComments" value="true" /> </commentGenerator> <!--数据库连接的信息:驱动类、连接地址、用户名、密码 --> <jdbcConnection driverClass="com.mysql.jdbc.Driver" connectionURL="jdbc:mysql://192.168.30.10:3306/ego" userId="root" password="root"> </jdbcConnection> <!-- 默认false,把JDBC DECIMAL 和 NUMERIC 类型解析为 Integer,为 true时 把JDBC DECIMAL 和 NUMERIC 类型解析为java.math.BigDecimal --> <javaTypeResolver> <property name="forceBigDecimals" value="false" /> </javaTypeResolver> <!-- targetProject:生成POJO类的位置 --> <javaModelGenerator targetPackage="com.bjsxt.ego.rpc.pojo" targetProject=".src"> <!-- enableSubPackages:是否让schema作为包的后缀 --> <property name="enableSubPackages" value="false" /> <!-- 从数据库返回的值被清理前后的空格 --> <property name="trimStrings" value="true" /> </javaModelGenerator> <!-- targetProject:mapper映射文件生成的位置 --> <sqlMapGenerator targetPackage="com.bjsxt.ego.rpc.mapper" targetProject=".src"> <!-- enableSubPackages:是否让schema作为包的后缀 --> <property name="enableSubPackages" value="false" /> </sqlMapGenerator> <!-- targetPackage:mapper接口生成的位置 --> <javaClientGenerator type="XMLMAPPER" targetPackage="com.bjsxt.ego.rpc.mapper" targetProject=".src"> <!-- enableSubPackages:是否让schema作为包的后缀 --> <property name="enableSubPackages" value="false" /> </javaClientGenerator> <!-- 指定数据库表 --> <table schema="" tableName="tb_content"></table> <table schema="" tableName="tb_content_category"></table> <table schema="" tableName="tb_item"></table> <table schema="" tableName="tb_item_cat"></table> <table schema="" tableName="tb_item_desc"></table> <table schema="" tableName="tb_item_param"></table> <table schema="" tableName="tb_item_param_item"></table> <table schema="" tableName="tb_order"></table> <table schema="" tableName="tb_order_item"></table> <table schema="" tableName="tb_order_shipping"></table> <table schema="" tableName="tb_user"></table> </context> </generatorConfiguration>
2.4 建立项目启动类

package com.mybatis.generator.test; import java.io.File; import java.util.ArrayList; import java.util.List; import org.mybatis.generator.api.MyBatisGenerator; import org.mybatis.generator.config.Configuration; import org.mybatis.generator.config.xml.ConfigurationParser; import org.mybatis.generator.internal.DefaultShellCallback; public class MybatisGeneratorTest { public void generator() throws Exception { List<String>warnings = new ArrayList<String>(); boolean overwrite = true; // 指定 逆向工程配置文件 File configFile = new File(System.getProperty("user.dir")+"/src/config.xml"); ConfigurationParser cp = new ConfigurationParser(warnings); Configuration config = cp.parseConfiguration(configFile); DefaultShellCallback callback = new DefaultShellCallback(overwrite); MyBatisGenerator myBatisGenerator = new MyBatisGenerator(config, callback, warnings); myBatisGenerator.generate(null); } public static void main(String[] args) throws Exception { try { MybatisGeneratorTest generatorSqlmap = new MybatisGeneratorTest(); generatorSqlmap.generator(); } catch (Exception e) { e.printStackTrace(); } } }
mybatis逆向工程
2.5 生成的mapper

package com.bjsxt.ego.rpc.mapper; import com.bjsxt.ego.rpc.pojo.TbItem; import com.bjsxt.ego.rpc.pojo.TbItemExample; import java.util.List; import org.apache.ibatis.annotations.Param; public interface TbItemMapper { int countByExample(TbItemExample example); int deleteByExample(TbItemExample example); int deleteByPrimaryKey(Long id); int insert(TbItem record); int insertSelective(TbItem record); List<TbItem> selectByExample(TbItemExample example); TbItem selectByPrimaryKey(Long id); int updateByExampleSelective(@Param("record") TbItem record, @Param("example") TbItemExample example); int updateByExample(@Param("record") TbItem record, @Param("example") TbItemExample example); int updateByPrimaryKeySelective(TbItem record); int updateByPrimaryKey(TbItem record); }

<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.bjsxt.ego.rpc.mapper.TbItemMapper" > <resultMap id="BaseResultMap" type="com.bjsxt.ego.rpc.pojo.TbItem" > <id column="id" property="id" jdbcType="BIGINT" /> <result column="title" property="title" jdbcType="VARCHAR" /> <result column="sell_point" property="sellPoint" jdbcType="VARCHAR" /> <result column="price" property="price" jdbcType="BIGINT" /> <result column="num" property="num" jdbcType="INTEGER" /> <result column="barcode" property="barcode" jdbcType="VARCHAR" /> <result column="image" property="image" jdbcType="VARCHAR" /> <result column="cid" property="cid" jdbcType="BIGINT" /> <result column="status" property="status" jdbcType="TINYINT" /> <result column="created" property="created" jdbcType="TIMESTAMP" /> <result column="updated" property="updated" jdbcType="TIMESTAMP" /> </resultMap> <sql id="Example_Where_Clause" > <where > <foreach collection="oredCriteria" item="criteria" separator="or" > <if test="criteria.valid" > <trim prefix="(" suffix=")" prefixOverrides="and" > <foreach collection="criteria.criteria" item="criterion" > <choose > <when test="criterion.noValue" > and ${criterion.condition} </when> <when test="criterion.singleValue" > and ${criterion.condition} #{criterion.value} </when> <when test="criterion.betweenValue" > and ${criterion.condition} #{criterion.value} and #{criterion.secondValue} </when> <when test="criterion.listValue" > and ${criterion.condition} <foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," > #{listItem} </foreach> </when> </choose> </foreach> </trim> </if> </foreach> </where> </sql> <sql id="Update_By_Example_Where_Clause" > <where > <foreach collection="example.oredCriteria" item="criteria" separator="or" > <if test="criteria.valid" > <trim prefix="(" suffix=")" prefixOverrides="and" > <foreach collection="criteria.criteria" item="criterion" > <choose > <when test="criterion.noValue" > and ${criterion.condition} </when> <when test="criterion.singleValue" > and ${criterion.condition} #{criterion.value} </when> <when test="criterion.betweenValue" > and ${criterion.condition} #{criterion.value} and #{criterion.secondValue} </when> <when test="criterion.listValue" > and ${criterion.condition} <foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," > #{listItem} </foreach> </when> </choose> </foreach> </trim> </if> </foreach> </where> </sql> <sql id="Base_Column_List" > id, title, sell_point, price, num, barcode, image, cid, status, created, updated </sql> <select id="selectByExample" resultMap="BaseResultMap" parameterType="com.bjsxt.ego.rpc.pojo.TbItemExample" > select <if test="distinct" > distinct </if> <include refid="Base_Column_List" /> from tb_item <if test="_parameter != null" > <include refid="Example_Where_Clause" /> </if> <if test="orderByClause != null" > order by ${orderByClause} </if> </select> <select id="selectByPrimaryKey" resultMap="BaseResultMap" parameterType="java.lang.Long" > select <include refid="Base_Column_List" /> from tb_item where id = #{id,jdbcType=BIGINT} </select> <delete id="deleteByPrimaryKey" parameterType="java.lang.Long" > delete from tb_item where id = #{id,jdbcType=BIGINT} </delete> <delete id="deleteByExample" parameterType="com.bjsxt.ego.rpc.pojo.TbItemExample" > delete from tb_item <if test="_parameter != null" > <include refid="Example_Where_Clause" /> </if> </delete> <insert id="insert" parameterType="com.bjsxt.ego.rpc.pojo.TbItem" > insert into tb_item (id, title, sell_point, price, num, barcode, image, cid, status, created, updated) values (#{id,jdbcType=BIGINT}, #{title,jdbcType=VARCHAR}, #{sellPoint,jdbcType=VARCHAR}, #{price,jdbcType=BIGINT}, #{num,jdbcType=INTEGER}, #{barcode,jdbcType=VARCHAR}, #{image,jdbcType=VARCHAR}, #{cid,jdbcType=BIGINT}, #{status,jdbcType=TINYINT}, #{created,jdbcType=TIMESTAMP}, #{updated,jdbcType=TIMESTAMP}) </insert> <insert id="insertSelective" parameterType="com.bjsxt.ego.rpc.pojo.TbItem" > insert into tb_item <trim prefix="(" suffix=")" suffixOverrides="," > <if test="id != null" > id, </if> <if test="title != null" > title, </if> <if test="sellPoint != null" > sell_point, </if> <if test="price != null" > price, </if> <if test="num != null" > num, </if> <if test="barcode != null" > barcode, </if> <if test="image != null" > image, </if> <if test="cid != null" > cid, </if> <if test="status != null" > status, </if> <if test="created != null" > created, </if> <if test="updated != null" > updated, </if> </trim> <trim prefix="values (" suffix=")" suffixOverrides="," > <if test="id != null" > #{id,jdbcType=BIGINT}, </if> <if test="title != null" > #{title,jdbcType=VARCHAR}, </if> <if test="sellPoint != null" > #{sellPoint,jdbcType=VARCHAR}, </if> <if test="price != null" > #{price,jdbcType=BIGINT}, </if> <if test="num != null" > #{num,jdbcType=INTEGER}, </if> <if test="barcode != null" > #{barcode,jdbcType=VARCHAR}, </if> <if test="image != null" > #{image,jdbcType=VARCHAR}, </if> <if test="cid != null" > #{cid,jdbcType=BIGINT}, </if> <if test="status != null" > #{status,jdbcType=TINYINT}, </if> <if test="created != null" > #{created,jdbcType=TIMESTAMP}, </if> <if test="updated != null" > #{updated,jdbcType=TIMESTAMP}, </if> </trim> </insert> <select id="countByExample" parameterType="com.bjsxt.ego.rpc.pojo.TbItemExample" resultType="java.lang.Integer" > select count(*) from tb_item <if test="_parameter != null" > <include refid="Example_Where_Clause" /> </if> </select> <update id="updateByExampleSelective" parameterType="map" > update tb_item <set > <if test="record.id != null" > id = #{record.id,jdbcType=BIGINT}, </if> <if test="record.title != null" > title = #{record.title,jdbcType=VARCHAR}, </if> <if test="record.sellPoint != null" > sell_point = #{record.sellPoint,jdbcType=VARCHAR}, </if> <if test="record.price != null" > price = #{record.price,jdbcType=BIGINT}, </if> <if test="record.num != null" > num = #{record.num,jdbcType=INTEGER}, </if> <if test="record.barcode != null" > barcode = #{record.barcode,jdbcType=VARCHAR}, </if> <if test="record.image != null" > image = #{record.image,jdbcType=VARCHAR}, </if> <if test="record.cid != null" > cid = #{record.cid,jdbcType=BIGINT}, </if> <if test="record.status != null" > status = #{record.status,jdbcType=TINYINT}, </if> <if test="record.created != null" > created = #{record.created,jdbcType=TIMESTAMP}, </if> <if test="record.updated != null" > updated = #{record.updated,jdbcType=TIMESTAMP}, </if> </set> <if test="_parameter != null" > <include refid="Update_By_Example_Where_Clause" /> </if> </update> <update id="updateByExample" parameterType="map" > update tb_item set id = #{record.id,jdbcType=BIGINT}, title = #{record.title,jdbcType=VARCHAR}, sell_point = #{record.sellPoint,jdbcType=VARCHAR}, price = #{record.price,jdbcType=BIGINT}, num = #{record.num,jdbcType=INTEGER}, barcode = #{record.barcode,jdbcType=VARCHAR}, image = #{record.image,jdbcType=VARCHAR}, cid = #{record.cid,jdbcType=BIGINT}, status = #{record.status,jdbcType=TINYINT}, created = #{record.created,jdbcType=TIMESTAMP}, updated = #{record.updated,jdbcType=TIMESTAMP} <if test="_parameter != null" > <include refid="Update_By_Example_Where_Clause" /> </if> </update> <update id="updateByPrimaryKeySelective" parameterType="com.bjsxt.ego.rpc.pojo.TbItem" > update tb_item <set > <if test="title != null" > title = #{title,jdbcType=VARCHAR}, </if> <if test="sellPoint != null" > sell_point = #{sellPoint,jdbcType=VARCHAR}, </if> <if test="price != null" > price = #{price,jdbcType=BIGINT}, </if> <if test="num != null" > num = #{num,jdbcType=INTEGER}, </if> <if test="barcode != null" > barcode = #{barcode,jdbcType=VARCHAR}, </if> <if test="image != null" > image = #{image,jdbcType=VARCHAR}, </if> <if test="cid != null" > cid = #{cid,jdbcType=BIGINT}, </if> <if test="status != null" > status = #{status,jdbcType=TINYINT}, </if> <if test="created != null" > created = #{created,jdbcType=TIMESTAMP}, </if> <if test="updated != null" > updated = #{updated,jdbcType=TIMESTAMP}, </if> </set> where id = #{id,jdbcType=BIGINT} </update> <update id="updateByPrimaryKey" parameterType="com.bjsxt.ego.rpc.pojo.TbItem" > update tb_item set title = #{title,jdbcType=VARCHAR}, sell_point = #{sellPoint,jdbcType=VARCHAR}, price = #{price,jdbcType=BIGINT}, num = #{num,jdbcType=INTEGER}, barcode = #{barcode,jdbcType=VARCHAR}, image = #{image,jdbcType=VARCHAR}, cid = #{cid,jdbcType=BIGINT}, status = #{status,jdbcType=TINYINT}, created = #{created,jdbcType=TIMESTAMP}, updated = #{updated,jdbcType=TIMESTAMP} where id = #{id,jdbcType=BIGINT} </update> </mapper>
2.6生成的pojo

package com.bjsxt.ego.rpc.pojo; import java.io.Serializable; import java.util.Date; public class TbItem implements Serializable{ private Long id; private String title; private String sellPoint; private Long price; private Integer num; private String barcode; private String image; private Long cid; private Byte status; private Date created; private Date updated; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title == null ? null : title.trim(); } public String getSellPoint() { return sellPoint; } public void setSellPoint(String sellPoint) { this.sellPoint = sellPoint == null ? null : sellPoint.trim(); } public Long getPrice() { return price; } public void setPrice(Long price) { this.price = price; } public Integer getNum() { return num; } public void setNum(Integer num) { this.num = num; } public String getBarcode() { return barcode; } public void setBarcode(String barcode) { this.barcode = barcode == null ? null : barcode.trim(); } public String getImage() { return image; } public void setImage(String image) { this.image = image == null ? null : image.trim(); } public Long getCid() { return cid; } public void setCid(Long cid) { this.cid = cid; } public Byte getStatus() { return status; } public void setStatus(Byte status) { this.status = status; } public Date getCreated() { return created; } public void setCreated(Date created) { this.created = created; } public Date getUpdated() { return updated; } public void setUpdated(Date updated) { this.updated = updated; } }

package com.bjsxt.ego.rpc.pojo; import java.util.ArrayList; import java.util.Date; import java.util.List; public class TbItemExample { protected String orderByClause; protected boolean distinct; protected List<Criteria> oredCriteria; public TbItemExample() { oredCriteria = new ArrayList<Criteria>(); } public void setOrderByClause(String orderByClause) { this.orderByClause = orderByClause; } public String getOrderByClause() { return orderByClause; } public void setDistinct(boolean distinct) { this.distinct = distinct; } public boolean isDistinct() { return distinct; } public List<Criteria> getOredCriteria() { return oredCriteria; } public void or(Criteria criteria) { oredCriteria.add(criteria); } public Criteria or() { Criteria criteria = createCriteriaInternal(); oredCriteria.add(criteria); return criteria; } public Criteria createCriteria() { Criteria criteria = createCriteriaInternal(); if (oredCriteria.size() == 0) { oredCriteria.add(criteria); } return criteria; } protected Criteria createCriteriaInternal() { Criteria criteria = new Criteria(); return criteria; } public void clear() { oredCriteria.clear(); orderByClause = null; distinct = false; } protected abstract static class GeneratedCriteria { protected List<Criterion> criteria; protected GeneratedCriteria() { super(); criteria = new ArrayList<Criterion>(); } public boolean isValid() { return criteria.size() > 0; } public List<Criterion> getAllCriteria() { return criteria; } public List<Criterion> getCriteria() { return criteria; } protected void addCriterion(String condition) { if (condition == null) { throw new RuntimeException("Value for condition cannot be null"); } criteria.add(new Criterion(condition)); } protected void addCriterion(String condition, Object value, String property) { if (value == null) { throw new RuntimeException("Value for " + property + " cannot be null"); } criteria.add(new Criterion(condition, value)); } protected void addCriterion(String condition, Object value1, Object value2, String property) { if (value1 == null || value2 == null) { throw new RuntimeException("Between values for " + property + " cannot be null"); } criteria.add(new Criterion(condition, value1, value2)); } public Criteria andIdIsNull() { addCriterion("id is null"); return (Criteria) this; } public Criteria andIdIsNotNull() { addCriterion("id is not null"); return (Criteria) this; } public Criteria andIdEqualTo(Long value) { addCriterion("id =", value, "id"); return (Criteria) this; } public Criteria andIdNotEqualTo(Long value) { addCriterion("id <>", value, "id"); return (Criteria) this; } public Criteria andIdGreaterThan(Long value) { addCriterion("id >", value, "id"); return (Criteria) this; } public Criteria andIdGreaterThanOrEqualTo(Long value) { addCriterion("id >=", value, "id"); return (Criteria) this; } public Criteria andIdLessThan(Long value) { addCriterion("id <", value, "id"); return (Criteria) this; } public Criteria andIdLessThanOrEqualTo(Long value) { addCriterion("id <=", value, "id"); return (Criteria) this; } public Criteria andIdIn(List<Long> values) { addCriterion("id in", values, "id"); return (Criteria) this; } public Criteria andIdNotIn(List<Long> values) { addCriterion("id not in", values, "id"); return (Criteria) this; } public Criteria andIdBetween(Long value1, Long value2) { addCriterion("id between", value1, value2, "id"); return (Criteria) this; } public Criteria andIdNotBetween(Long value1, Long value2) { addCriterion("id not between", value1, value2, "id"); return (Criteria) this; } public Criteria andTitleIsNull() { addCriterion("title is null"); return (Criteria) this; } public Criteria andTitleIsNotNull() { addCriterion("title is not null"); return (Criteria) this; } public Criteria andTitleEqualTo(String value) { addCriterion("title =", value, "title"); return (Criteria) this; } public Criteria andTitleNotEqualTo(String value) { addCriterion("title <>", value, "title"); return (Criteria) this; } public Criteria andTitleGreaterThan(String value) { addCriterion("title >", value, "title"); return (Criteria) this; } public Criteria andTitleGreaterThanOrEqualTo(String value) { addCriterion("title >=", value, "title"); return (Criteria) this; } public Criteria andTitleLessThan(String value) { addCriterion("title <", value, "title"); return (Criteria) this; } public Criteria andTitleLessThanOrEqualTo(String value) { addCriterion("title <=", value, "title"); return (Criteria) this; } public Criteria andTitleLike(String value) { addCriterion("title like", value, "title"); return (Criteria) this; } public Criteria andTitleNotLike(String value) { addCriterion("title not like", value, "title"); return (Criteria) this; } public Criteria andTitleIn(List<String> values) { addCriterion("title in", values, "title"); return (Criteria) this; } public Criteria andTitleNotIn(List<String> values) { addCriterion("title not in", values, "title"); return (Criteria) this; } public Criteria andTitleBetween(String value1, String value2) { addCriterion("title between", value1, value2, "title"); return (Criteria) this; } public Criteria andTitleNotBetween(String value1, String value2) { addCriterion("title not between", value1, value2, "title"); return (Criteria) this; } public Criteria andSellPointIsNull() { addCriterion("sell_point is null"); return (Criteria) this; } public Criteria andSellPointIsNotNull() { addCriterion("sell_point is not null"); return (Criteria) this; } public Criteria andSellPointEqualTo(String value) { addCriterion("sell_point =", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointNotEqualTo(String value) { addCriterion("sell_point <>", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointGreaterThan(String value) { addCriterion("sell_point >", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointGreaterThanOrEqualTo(String value) { addCriterion("sell_point >=", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointLessThan(String value) { addCriterion("sell_point <", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointLessThanOrEqualTo(String value) { addCriterion("sell_point <=", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointLike(String value) { addCriterion("sell_point like", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointNotLike(String value) { addCriterion("sell_point not like", value, "sellPoint"); return (Criteria) this; } public Criteria andSellPointIn(List<String> values) { addCriterion("sell_point in", values, "sellPoint"); return (Criteria) this; } public Criteria andSellPointNotIn(List<String> values) { addCriterion("sell_point not in", values, "sellPoint"); return (Criteria) this; } public Criteria andSellPointBetween(String value1, String value2) { addCriterion("sell_point between", value1, value2, "sellPoint"); return (Criteria) this; } public Criteria andSellPointNotBetween(String value1, String value2) { addCriterion("sell_point not between", value1, value2, "sellPoint"); return (Criteria) this; } public Criteria andPriceIsNull() { addCriterion("price is null"); return (Criteria) this; } public Criteria andPriceIsNotNull() { addCriterion("price is not null"); return (Criteria) this; } public Criteria andPriceEqualTo(Long value) { addCriterion("price =", value, "price"); return (Criteria) this; } public Criteria andPriceNotEqualTo(Long value) { addCriterion("price <>", value, "price"); return (Criteria) this; } public Criteria andPriceGreaterThan(Long value) { addCriterion("price >", value, "price"); return (Criteria) this; } public Criteria andPriceGreaterThanOrEqualTo(Long value) { addCriterion("price >=", value, "price"); return (Criteria) this; } public Criteria andPriceLessThan(Long value) { addCriterion("price <", value, "price"); return (Criteria) this; } public Criteria andPriceLessThanOrEqualTo(Long value) { addCriterion("price <=", value, "price"); return (Criteria) this; } public Criteria andPriceIn(List<Long> values) { addCriterion("price in", values, "price"); return (Criteria) this; } public Criteria andPriceNotIn(List<Long> values) { addCriterion("price not in", values, "price"); return (Criteria) this; } public Criteria andPriceBetween(Long value1, Long value2) { addCriterion("price between", value1, value2, "price"); return (Criteria) this; } public Criteria andPriceNotBetween(Long value1, Long value2) { addCriterion("price not between", value1, value2, "price"); return (Criteria) this; } public Criteria andNumIsNull() { addCriterion("num is null"); return (Criteria) this; } public Criteria andNumIsNotNull() { addCriterion("num is not null"); return (Criteria) this; } public Criteria andNumEqualTo(Integer value) { addCriterion("num =", value, "num"); return (Criteria) this; } public Criteria andNumNotEqualTo(Integer value) { addCriterion("num <>", value, "num"); return (Criteria) this; } public Criteria andNumGreaterThan(Integer value) { addCriterion("num >", value, "num"); return (Criteria) this; } public Criteria andNumGreaterThanOrEqualTo(Integer value) { addCriterion("num >=", value, "num"); return (Criteria) this; } public Criteria andNumLessThan(Integer value) { addCriterion("num <", value, "num"); return (Criteria) this; } public Criteria andNumLessThanOrEqualTo(Integer value) { addCriterion("num <=", value, "num"); return (Criteria) this; } public Criteria andNumIn(List<Integer> values) { addCriterion("num in", values, "num"); return (Criteria) this; } public Criteria andNumNotIn(List<Integer> values) { addCriterion("num not in", values, "num"); return (Criteria) this; } public Criteria andNumBetween(Integer value1, Integer value2) { addCriterion("num between", value1, value2, "num"); return (Criteria) this; } public Criteria andNumNotBetween(Integer value1, Integer value2) { addCriterion("num not between", value1, value2, "num"); return (Criteria) this; } public Criteria andBarcodeIsNull() { addCriterion("barcode is null"); return (Criteria) this; } public Criteria andBarcodeIsNotNull() { addCriterion("barcode is not null"); return (Criteria) this; } public Criteria andBarcodeEqualTo(String value) { addCriterion("barcode =", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeNotEqualTo(String value) { addCriterion("barcode <>", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeGreaterThan(String value) { addCriterion("barcode >", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeGreaterThanOrEqualTo(String value) { addCriterion("barcode >=", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeLessThan(String value) { addCriterion("barcode <", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeLessThanOrEqualTo(String value) { addCriterion("barcode <=", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeLike(String value) { addCriterion("barcode like", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeNotLike(String value) { addCriterion("barcode not like", value, "barcode"); return (Criteria) this; } public Criteria andBarcodeIn(List<String> values) { addCriterion("barcode in", values, "barcode"); return (Criteria) this; } public Criteria andBarcodeNotIn(List<String> values) { addCriterion("barcode not in", values, "barcode"); return (Criteria) this; } public Criteria andBarcodeBetween(String value1, String value2) { addCriterion("barcode between", value1, value2, "barcode"); return (Criteria) this; } public Criteria andBarcodeNotBetween(String value1, String value2) { addCriterion("barcode not between", value1, value2, "barcode"); return (Criteria) this; } public Criteria andImageIsNull() { addCriterion("image is null"); return (Criteria) this; } public Criteria andImageIsNotNull() { addCriterion("image is not null"); return (Criteria) this; } public Criteria andImageEqualTo(String value) { addCriterion("image =", value, "image"); return (Criteria) this; } public Criteria andImageNotEqualTo(String value) { addCriterion("image <>", value, "image"); return (Criteria) this; } public Criteria andImageGreaterThan(String value) { addCriterion("image >", value, "image"); return (Criteria) this; } public Criteria andImageGreaterThanOrEqualTo(String value) { addCriterion("image >=", value, "image"); return (Criteria) this; } public Criteria andImageLessThan(String value) { addCriterion("image <", value, "image"); return (Criteria) this; } public Criteria andImageLessThanOrEqualTo(String value) { addCriterion("image <=", value, "image"); return (Criteria) this; } public Criteria andImageLike(String value) { addCriterion("image like", value, "image"); return (Criteria) this; } public Criteria andImageNotLike(String value) { addCriterion("image not like", value, "image"); return (Criteria) this; } public Criteria andImageIn(List<String> values) { addCriterion("image in", values, "image"); return (Criteria) this; } public Criteria andImageNotIn(List<String> values) { addCriterion("image not in", values, "image"); return (Criteria) this; } public Criteria andImageBetween(String value1, String value2) { addCriterion("image between", value1, value2, "image"); return (Criteria) this; } public Criteria andImageNotBetween(String value1, String value2) { addCriterion("image not between", value1, value2, "image"); return (Criteria) this; } public Criteria andCidIsNull() { addCriterion("cid is null"); return (Criteria) this; } public Criteria andCidIsNotNull() { addCriterion("cid is not null"); return (Criteria) this; } public Criteria andCidEqualTo(Long value) { addCriterion("cid =", value, "cid"); return (Criteria) this; } public Criteria andCidNotEqualTo(Long value) { addCriterion("cid <>", value, "cid"); return (Criteria) this; } public Criteria andCidGreaterThan(Long value) { addCriterion("cid >", value, "cid"); return (Criteria) this; } public Criteria andCidGreaterThanOrEqualTo(Long value) { addCriterion("cid >=", value, "cid"); return (Criteria) this; } public Criteria andCidLessThan(Long value) { addCriterion("cid <", value, "cid"); return (Criteria) this; } public Criteria andCidLessThanOrEqualTo(Long value) { addCriterion("cid <=", value, "cid"); return (Criteria) this; } public Criteria andCidIn(List<Long> values) { addCriterion("cid in", values, "cid"); return (Criteria) this; } public Criteria andCidNotIn(List<Long> values) { addCriterion("cid not in", values, "cid"); return (Criteria) this; } public Criteria andCidBetween(Long value1, Long value2) { addCriterion("cid between", value1, value2, "cid"); return (Criteria) this; } public Criteria andCidNotBetween(Long value1, Long value2) { addCriterion("cid not between", value1, value2, "cid"); return (Criteria) this; } public Criteria andStatusIsNull() { addCriterion("status is null"); return (Criteria) this; } public Criteria andStatusIsNotNull() { addCriterion("status is not null"); return (Criteria) this; } public Criteria andStatusEqualTo(Byte value) { addCriterion("status =", value, "status"); return (Criteria) this; } public Criteria andStatusNotEqualTo(Byte value) { addCriterion("status <>", value, "status"); return (Criteria) this; } public Criteria andStatusGreaterThan(Byte value) { addCriterion("status >", value, "status"); return (Criteria) this; } public Criteria andStatusGreaterThanOrEqualTo(Byte value) { addCriterion("status >=", value, "status"); return (Criteria) this; } public Criteria andStatusLessThan(Byte value) { addCriterion("status <", value, "status"); return (Criteria) this; } public Criteria andStatusLessThanOrEqualTo(Byte value) { addCriterion("status <=", value, "status"); return (Criteria) this; } public Criteria andStatusIn(List<Byte> values) { addCriterion("status in", values, "status"); return (Criteria) this; } public Criteria andStatusNotIn(List<Byte> values) { addCriterion("status not in", values, "status"); return (Criteria) this; } public Criteria andStatusBetween(Byte value1, Byte value2) { addCriterion("status between", value1, value2, "status"); return (Criteria) this; } public Criteria andStatusNotBetween(Byte value1, Byte value2) { addCriterion("status not between", value1, value2, "status"); return (Criteria) this; } public Criteria andCreatedIsNull() { addCriterion("created is null"); return (Criteria) this; } public Criteria andCreatedIsNotNull() { addCriterion("created is not null"); return (Criteria) this; } public Criteria andCreatedEqualTo(Date value) { addCriterion("created =", value, "created"); return (Criteria) this; } public Criteria andCreatedNotEqualTo(Date value) { addCriterion("created <>", value, "created"); return (Criteria) this; } public Criteria andCreatedGreaterThan(Date value) { addCriterion("created >", value, "created"); return (Criteria) this; } public Criteria andCreatedGreaterThanOrEqualTo(Date value) { addCriterion("created >=", value, "created"); return (Criteria) this; } public Criteria andCreatedLessThan(Date value) { addCriterion("created <", value, "created"); return (Criteria) this; } public Criteria andCreatedLessThanOrEqualTo(Date value) { addCriterion("created <=", value, "created"); return (Criteria) this; } public Criteria andCreatedIn(List<Date> values) { addCriterion("created in", values, "created"); return (Criteria) this; } public Criteria andCreatedNotIn(List<Date> values) { addCriterion("created not in", values, "created"); return (Criteria) this; } public Criteria andCreatedBetween(Date value1, Date value2) { addCriterion("created between", value1, value2, "created"); return (Criteria) this; } public Criteria andCreatedNotBetween(Date value1, Date value2) { addCriterion("created not between", value1, value2, "created"); return (Criteria) this; } public Criteria andUpdatedIsNull() { addCriterion("updated is null"); return (Criteria) this; } public Criteria andUpdatedIsNotNull() { addCriterion("updated is not null"); return (Criteria) this; } public Criteria andUpdatedEqualTo(Date value) { addCriterion("updated =", value, "updated"); return (Criteria) this; } public Criteria andUpdatedNotEqualTo(Date value) { addCriterion("updated <>", value, "updated"); return (Criteria) this; } public Criteria andUpdatedGreaterThan(Date value) { addCriterion("updated >", value, "updated"); return (Criteria) this; } public Criteria andUpdatedGreaterThanOrEqualTo(Date value) { addCriterion("updated >=", value, "updated"); return (Criteria) this; } public Criteria andUpdatedLessThan(Date value) { addCriterion("updated <", value, "updated"); return (Criteria) this; } public Criteria andUpdatedLessThanOrEqualTo(Date value) { addCriterion("updated <=", value, "updated"); return (Criteria) this; } public Criteria andUpdatedIn(List<Date> values) { addCriterion("updated in", values, "updated"); return (Criteria) this; } public Criteria andUpdatedNotIn(List<Date> values) { addCriterion("updated not in", values, "updated"); return (Criteria) this; } public Criteria andUpdatedBetween(Date value1, Date value2) { addCriterion("updated between", value1, value2, "updated"); return (Criteria) this; } public Criteria andUpdatedNotBetween(Date value1, Date value2) { addCriterion("updated not between", value1, value2, "updated"); return (Criteria) this; } } public static class Criteria extends GeneratedCriteria { protected Criteria() { super(); } } public static class Criterion { private String condition; private Object value; private Object secondValue; private boolean noValue; private boolean singleValue; private boolean betweenValue; private boolean listValue; private String typeHandler; public String getCondition() { return condition; } public Object getValue() { return value; } public Object getSecondValue() { return secondValue; } public boolean isNoValue() { return noValue; } public boolean isSingleValue() { return singleValue; } public boolean isBetweenValue() { return betweenValue; } public boolean isListValue() { return listValue; } public String getTypeHandler() { return typeHandler; } protected Criterion(String condition) { super(); this.condition = condition; this.typeHandler = null; this.noValue = true; } protected Criterion(String condition, Object value, String typeHandler) { super(); this.condition = condition; this.value = value; this.typeHandler = typeHandler; if (value instanceof List<?>) { this.listValue = true; } else { this.singleValue = true; } } protected Criterion(String condition, Object value) { this(condition, value, null); } protected Criterion(String condition, Object value, Object secondValue, String typeHandler) { super(); this.condition = condition; this.value = value; this.secondValue = secondValue; this.typeHandler = typeHandler; this.betweenValue = true; } protected Criterion(String condition, Object value, Object secondValue) { this(condition, value, secondValue, null); } } }
补充
maven的pom.xml

<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>cn.mybatis.generator</groupId> <artifactId>mybatis-generator</artifactId> <version>0.0.1-SNAPSHOT</version> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.35</version> </dependency> <dependency> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-core</artifactId> <version>1.3.2</version> </dependency> </dependencies> <build> <pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> </configuration> <version>3.3</version> </plugin> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <version>1.3.2</version> <dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.35</version> </dependency> </dependencies> <configuration> <!--配置文件的路径--> <configurationFile>src/main/resources/generatorConfig.xml</configurationFile> <overwrite>true</overwrite> </configuration> </plugin> </plugins> </pluginManagement> </build> </project>
mybatis3Spimle

package com.hainei.service.impl.ldar; import com.hainei.common.exception.BusinessException; import com.hainei.common.exception.LdarException; import com.hainei.common.exception.code.LdarResponseCode; import com.hainei.common.utils.CommonUtils; import com.hainei.common.utils.DataResult; import com.hainei.common.utils.ExcelUtil; import com.hainei.mapper.ldar.*; import com.hainei.pojo.model.ldar.LdarDevice; import com.hainei.pojo.model.ldar.LdarDrain; import com.hainei.pojo.model.ldar.LdarEquipment; import com.hainei.pojo.model.ldar.LdarProduce; import com.hainei.service.ldar.ExcelService; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import org.springframework.web.multipart.MultipartFile; import tk.mybatis.mapper.entity.Example; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.*; import java.util.Date; import java.util.List; import java.util.Map; import java.util.UUID; /** * Created with IntelliJ IDEA. * User:wq * Date:2020/4/8 * Time: 17:00 * Description: No Description */ @Service @Slf4j public class ExcelServiceImpl implements ExcelService { @Autowired private LdarDrainMapper ldarDrainMapper; @Autowired private LdarFirmMapper ldarFirmMapper; @Autowired private LdarProduceMapper ldarProduceMapper; @Autowired private LdarDeviceMapper ldarDeviceMapper; @Autowired private LdarEquipmentMapper ldarEquipmentMapper; @Override public DataResult download(HttpServletRequest request, HttpServletResponse response) throws UnsupportedEncodingException { String fileName = "模板.zip"; if (fileName != null){ // String realPath = "E:\海内demo集\海内\模板\少量数据"; String realPath = "E:\海内demo集\海内"; File file = new File(realPath,fileName); fileName = new String(file.getName().getBytes("utf-8")); String suffixNmae = fileName.substring(fileName.lastIndexOf(".")); String name = CommonUtils.generateUUID().toString(); fileName = name + suffixNmae; if (file.exists()){ response.setContentType("application/force-download"); response.addHeader("Content-Disposition","attachment;fileName="+fileName); byte[] buffer = new byte[1024]; FileInputStream fis = null; BufferedInputStream bis = null; try{ fis = new FileInputStream(file); bis = new BufferedInputStream(fis); OutputStream os = response.getOutputStream(); int i = bis.read(buffer); while(i != -1){ os.write(buffer,0,i); i = bis.read(buffer); } System.out.println("success"); }catch (Exception e){ e.printStackTrace(); }finally { if (bis != null){ try{ bis.close(); }catch (IOException e){ e.printStackTrace(); } } if (fis != null){ try{ fis.close(); }catch (IOException e){ e.printStackTrace(); } } } } } System.out.println(fileName); return DataResult.success(fileName); } @Override public void importExeclProduce(MultipartFile filePath) throws Exception { List<Map<String, String>> mapList = ExcelUtil.readExcel(filePath, 0); log.info("mapList:" + mapList); for (Map<String, String> map : mapList) { if (map.get("生产区名称") != null && map.get("所属公司") !=""&& map.get("生产区状态") != null ) { Example example = new Example(LdarProduce.class); Example.Criteria criteria = example.createCriteria(); criteria.andEqualTo("produceName",map.get("生产区名称")); criteria.andEqualTo("firm",map.get("所属公司")); List<LdarProduce> ldarProduces = ldarProduceMapper.selectByExample(example); if(ldarProduces.size()>0){ for (LdarProduce produce: ldarProduces){ if(produce.getProduceName().equals(map.get("生产区名称"))) { Example example1 = new Example(LdarProduce.class); Example.Criteria criteria1 = example1.createCriteria(); criteria1.andEqualTo("produceName", map.get("生产区名称")); criteria1.andEqualTo("firm", map.get("所属公司")); List<LdarProduce> ldarProduces1 = ldarProduceMapper.selectByExample(example1); if (ldarProduces1.size() > 0) { break; } else { String id = UUID.randomUUID().toString().replace("-", ""); LdarProduce entity = new LdarProduce(id, map.get("生产区名称"), map.get("所属公司"), map.get("生产区状态"), map.get("管理者"), map.get("联系方式"), map.get("其他信息")); entity.setGmtCreatedOn(new Date()); ldarProduceMapper.insertSelective(entity); } } } }else{ String id = UUID.randomUUID().toString().replace("-", ""); LdarProduce entity = new LdarProduce(id,map.get("生产区名称"),map.get("所属公司"), map.get("生产区状态"),map.get("管理者"),map.get("联系方式"),map.get("其他信息")); entity.setGmtCreatedOn(new Date()); ldarProduceMapper.insertSelective(entity); } } else { //throw new Exception("关键字段为空"); throw new LdarException(LdarResponseCode.DATA_ERROR); } } } @Override public void importExeclDevice(MultipartFile filePath) throws Exception { List<Map<String, String>> mapList = ExcelUtil.readExcel(filePath, 0); log.info("mapList:" + mapList); for (Map<String, String> map : mapList) { if (map.get("装置状态") != null && map.get("所属公司") !="" && map.get("所在生产区") != null && map.get("装置名称")!=null) { Example example = new Example(LdarDevice.class); Example.Criteria criteria = example.createCriteria(); criteria.andEqualTo("name",map.get("装置名称")); criteria.andEqualTo("firm",map.get("所属公司")); criteria.andEqualTo("produceArea",map.get("所在生产区")); List<LdarDevice> ldarDevices = ldarDeviceMapper.selectByExample(example); if(ldarDevices.size()>0) { for (LdarDevice device : ldarDevices){ if (device.getName().equals(map.get("装置名称"))) { Example example1 = new Example(LdarDevice.class); Example.Criteria criteria1 = example1.createCriteria(); criteria1.andEqualTo("produceArea", map.get("所在生产区")); criteria1.andEqualTo("name", map.get("装置名称")); criteria1.andEqualTo("firm", map.get("所属公司")); List<LdarDevice> ldarDevices1 = ldarDeviceMapper.selectByExample(example1); if (ldarDevices1.size() > 0) { break; } else { String id = UUID.randomUUID().toString().replace("-", ""); LdarDevice entity = new LdarDevice(id, map.get("装置名称"), map.get("所属公司"), map.get("所在生产区"), map.get("装置状态"), map.get("管理者"), map.get("其他信息")); entity.setGmtCreatedOn(new Date()); ldarDeviceMapper.insertSelective(entity); } } } }else{ String id = UUID.randomUUID().toString().replace("-", ""); LdarDevice entity = new LdarDevice(id,map.get("装置名称"),map.get("所属公司"), map.get("所在生产区"),map.get("装置状态"),map.get("管理者"),map.get("其他信息")); entity.setGmtCreatedOn(new Date()); ldarDeviceMapper.insertSelective(entity); } } else { throw new LdarException(LdarResponseCode.DATA_ERROR); } } } @Override public void importExeclEquipment(MultipartFile filePath) throws Exception { List<Map<String, String>> mapList = ExcelUtil.readExcel(filePath, 0); log.info("mapList:" + mapList); for (Map<String, String> map : mapList) { if (map.get("设备名称") != null && map.get("所属公司") != "" && map.get("所在生产区") != null && map.get("所在装置")!=null&&map.get("设备状态")!=null) { Example example = new Example(LdarEquipment.class); Example.Criteria criteria = example.createCriteria(); criteria.andEqualTo("name",map.get("设备名称")); criteria.andEqualTo("firm",map.get("所属公司")); criteria.andEqualTo("produceArea",map.get("所在生产区")); criteria.andEqualTo("device",map.get("所在装置")); List<LdarEquipment> ldarEquipments = ldarEquipmentMapper.selectByExample(example); if(ldarEquipments.size()>0) { break; } else { String id = UUID.randomUUID().toString().replace("-", ""); LdarEquipment entity = new LdarEquipment(id, map.get("设备名称"), map.get("设备编码"), map.get("所属公司"), map.get("所在生产区"), map.get("所在装置"),map.get("设备状态"),map.get("管理者"), map.get("其他信息")); entity.setGmtCreatedOn(new Date()); ldarEquipmentMapper.insertSelective(entity); } }else { //throw new Exception("关键字段为空"); throw new LdarException(LdarResponseCode.DATA_ERROR); } } } }
pom.xml

<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.imooc</groupId> <artifactId>mybatis-generator-for-imooc</artifactId> <version>1.0-SNAPSHOT</version> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <!-- 引入log4j日志依赖 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-log4j</artifactId> <version>1.3.8.RELEASE</version> </dependency> <!-- 阿里开源数据源 --> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.1.0</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid-spring-boot-starter</artifactId> <version>1.1.0</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.41</version> </dependency> <!--mybatis--> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.3.1</version> </dependency> <!--mapper--> <dependency> <groupId>tk.mybatis</groupId> <artifactId>mapper-spring-boot-starter</artifactId> <version>1.2.4</version> </dependency> <!--pagehelper--> <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper-spring-boot-starter</artifactId> <version>1.2.3</version> </dependency> <!-- mybatis 逆向生成工具 --> <dependency> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-core</artifactId> <version>1.3.2</version> <scope>compile</scope> <optional>true</optional> </dependency> </dependencies> </project>
genneratorConfig.xml

<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration> <context id="MysqlContext" targetRuntime="MyBatis3Simple" defaultModelType="flat"> <property name="beginningDelimiter" value="`"/> <property name="endingDelimiter" value="`"/> <!-- 通用mapper所在目录 --> <plugin type="tk.mybatis.mapper.generator.MapperPlugin"> <property name="mappers" value="com.imooc.my.mapper.MyMapper"/> </plugin> <jdbcConnection driverClass="com.mysql.jdbc.Driver" connectionURL="jdbc:mysql://10.100.10.129:3306/newldar" userId="root" password="ZHEtang403~!"> </jdbcConnection> <!-- 对应生成的pojo所在包 --> <javaModelGenerator targetPackage="com.hainei.pojo.model.ldar" targetProject="src/main/java"/> <!-- 对应生成的mapper所在目录 --> <sqlMapGenerator targetPackage="mapper.ldar" targetProject="src/main/java"/> <!-- 配置mapper对应的java映射 --> <javaClientGenerator targetPackage="com.hainei.mapper.ldar" targetProject="src/main/java" type="XMLMAPPER"/> <!--<table tableName="ldar_third_party"></table>--> <!--<table tableName="ldar_notice"></table>--> <!-- <table tableName="ldar_dynamic_list"></table>--> <!-- <table tableName="ldar_factor"></table>--> <!--<table tableName="ldar_firm"></table>--> <!--<table tableName="ldar_produce"></table>--> <!-- <table tableName="ldar_device"></table>--> <!--<table tableName="ldar_equipment"></table>--> <table tableName="ldar_drain"></table> </context> </generatorConfiguration>
GeneratorDisplay.java

import org.mybatis.generator.api.MyBatisGenerator; import org.mybatis.generator.config.Configuration; import org.mybatis.generator.config.xml.ConfigurationParser; import org.mybatis.generator.internal.DefaultShellCallback; import java.io.File; import java.util.ArrayList; import java.util.List; public class GeneratorDisplay { public void generator() throws Exception { List<String> warnings = new ArrayList<String>(); boolean overwrite = true; //指定 逆向工程配置文件 File configFile = new File("generatorConfig.xml"); ConfigurationParser cp = new ConfigurationParser(warnings); Configuration config = cp.parseConfiguration(configFile); DefaultShellCallback callback = new DefaultShellCallback(overwrite); MyBatisGenerator myBatisGenerator = new MyBatisGenerator(config, callback, warnings); myBatisGenerator.generate(null); } public static void main(String[] args) throws Exception { try { GeneratorDisplay generatorSqlmap = new GeneratorDisplay(); generatorSqlmap.generator(); } catch (Exception e) { e.printStackTrace(); } } }
MyMapper

/* * The MIT License (MIT) * * Copyright (c) 2014-2016 abel533@gmail.com * * Permission is hereby granted, free of charge, to any person obtaining a copy * of this software and associated documentation files (the "Software"), to deal * in the Software without restriction, including without limitation the rights * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell * copies of the Software, and to permit persons to whom the Software is * furnished to do so, subject to the following conditions: * * The above copyright notice and this permission notice shall be included in * all copies or substantial portions of the Software. * * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN * THE SOFTWARE. */ package com.imooc.my.mapper; import tk.mybatis.mapper.common.Mapper; import tk.mybatis.mapper.common.MySqlMapper; /** * 继承自己的MyMapper */ public interface MyMapper<T> extends Mapper<T>, MySqlMapper<T> { }
DeviceServiceImpl的tkmybatis的使用

package com.hainei.service.impl.ldar; import com.github.pagehelper.PageHelper; import com.hainei.common.enums.YesOrNo; import com.hainei.common.exception.LdarException; import com.hainei.common.exception.code.LdarResponseCode; import com.hainei.common.utils.PageUtil; import com.hainei.common.utils.PageVO; import com.hainei.mapper.ldar.LdarDeviceMapper; import com.hainei.pojo.bo.ldar.DeviceBO; import com.hainei.pojo.bo.ldar.DeviceSelectiveBO; import com.hainei.pojo.model.ldar.LdarDevice; import com.hainei.pojo.model.ldar.LdarProduce; import com.hainei.service.ldar.DeviceService; import org.springframework.beans.BeanUtils; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.stereotype.Service; import tk.mybatis.mapper.entity.Example; import java.util.Date; import java.util.List; import java.util.UUID; /** * Created with IntelliJ IDEA. * User:wq * Date:2020/4/2 * Time: 11:31 * Description: No Description */ @Service public class DeviceServiceImpl implements DeviceService{ @Autowired private LdarDeviceMapper ldarDeviceMapper; @Override public void saveDevice(DeviceBO deviceBO) { LdarDevice entity = new LdarDevice(); BeanUtils.copyProperties(deviceBO,entity); entity.setId(UUID.randomUUID().toString().replace("-","")); entity.setGmtCreatedOn(new Date()); Example example = new Example(LdarDevice.class); Example.Criteria criteria = example.createCriteria(); criteria.andEqualTo("name",entity.getName()); List<LdarDevice> ldarDevices = ldarDeviceMapper.selectByExample(example); if (ldarDevices.size()>0){ throw new LdarException(LdarResponseCode.DATA_ALREADY_EXIST); }else{ ldarDeviceMapper.insertSelective(entity); } } @Override public void updateDevice(DeviceBO deviceBO) { LdarDevice ldarDevice = new LdarDevice(); BeanUtils.copyProperties(deviceBO,ldarDevice); ldarDevice.setGmtUpdatedOn(new Date()); ldarDeviceMapper.updateByPrimaryKeySelective(ldarDevice); } @Override public void deleteDevice(List<String> ids) { LdarDevice ldarDevice = new LdarDevice(); ldarDevice.setIsDeleted(YesOrNo.YES.type); Example example = new Example(LdarDevice.class); Example.Criteria criteria = example.createCriteria(); criteria.andIn("id",ids); ldarDeviceMapper.updateByExampleSelective(ldarDevice,example); } @Override public PageVO<LdarDevice> listDevice(Integer pageNum, Integer pageSize) { PageHelper.startPage(pageNum,pageSize); Example example = new Example(LdarDevice.class); example.orderBy("gmtCreatedOn").desc(); Example.Criteria criteria = example.createCriteria(); criteria.andEqualTo("isDeleted",YesOrNo.NO.type); List<LdarDevice> ldarDevices = ldarDeviceMapper.selectByExample(example); PageVO<LdarDevice> pageVO = PageUtil.getPageVO(ldarDevices); return pageVO; } @Override public LdarDevice getDeviceById(String id) { LdarDevice ldarDevice = ldarDeviceMapper.selectByPrimaryKey(id); return ldarDevice; } @Override public PageVO<LdarDevice> listDeviceByProduceId(DeviceSelectiveBO deviceSelectiveBO) { PageHelper.startPage(deviceSelectiveBO.getPageNum(),deviceSelectiveBO.getPageSize()); List<LdarDevice> ldarDevices = ldarDeviceMapper.selectByProduceId(deviceSelectiveBO); PageVO<LdarDevice> pageVO = PageUtil.getPageVO(ldarDevices); return pageVO; } }
改下驱动,url,用户名和密码,相关依赖,就能连接orale、mysql、sqlserver。
连接oracle的时候,maven官网的依赖jar包只支持jdk1.4,需要到oracle官网下载ojdc7或者8,此时就可以使用jdk1.8了。然后将ojdbc的jar包放入本地仓库中。mvn -install 项目名 包名,版本号,地址就能正常使用。