student类:
package com.myschool.entity;
public class student{
private int studentno; //学号
private String loginpwd; //密码
private int studentno; //学号
private String loginpwd; //密码
private String studentname; //学生姓名
private int sex; //性别
private int gradeid; //年级ID
private String phone; //电话
private String address; //地址
private String borndate; //生日
private int gradeid; //年级ID
private String phone; //电话
private String address; //地址
private String borndate; //生日
private String email; //邮箱
private String IdentityCard; //身份证号
grade grade=new grade(); //年级对象
public grade getGrade() {
return grade;
}
public void setGrade(grade grade) {
this.grade = grade;
}
public int getStudentno() {
return studentno;
}
public void setStudentno(int studentno) {
this.studentno = studentno;
}
public String getLoginpwd() {
return loginpwd;
}
public void setLoginpwd(String loginpwd) {
this.loginpwd = loginpwd;
}
public String getStudentname() {
return studentname;
}
public void setStudentname(String studentname) {
this.studentname = studentname;
}
public int getSex() {
return sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public int getGradeid() {
return gradeid;
}
public void setGradeid(int gradeid) {
this.gradeid = gradeid;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getBorndate() {
return borndate;
}
public void setBorndate(String borndate) {
this.borndate = borndate;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getIdentityCard() {
return IdentityCard;
}
public void setIdentityCard(String identityCard) {
IdentityCard = identityCard;
}
//带参构造
private String IdentityCard; //身份证号
grade grade=new grade(); //年级对象
public grade getGrade() {
return grade;
}
public void setGrade(grade grade) {
this.grade = grade;
}
public int getStudentno() {
return studentno;
}
public void setStudentno(int studentno) {
this.studentno = studentno;
}
public String getLoginpwd() {
return loginpwd;
}
public void setLoginpwd(String loginpwd) {
this.loginpwd = loginpwd;
}
public String getStudentname() {
return studentname;
}
public void setStudentname(String studentname) {
this.studentname = studentname;
}
public int getSex() {
return sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public int getGradeid() {
return gradeid;
}
public void setGradeid(int gradeid) {
this.gradeid = gradeid;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getBorndate() {
return borndate;
}
public void setBorndate(String borndate) {
this.borndate = borndate;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getIdentityCard() {
return IdentityCard;
}
public void setIdentityCard(String identityCard) {
IdentityCard = identityCard;
}
//带参构造
public student(int studentno, String loginpwd, String studentname, int sex,
int gradeid, String phone, String address, String borndate,
String email, String identityCard) {
super();
this.studentno = studentno;
this.loginpwd = loginpwd;
this.studentname = studentname;
this.sex = sex;
this.gradeid = gradeid;
this.phone = phone;
this.address = address;
this.borndate = borndate;
this.email = email;
IdentityCard = identityCard;
}
//无参构造
public student(){
}
int gradeid, String phone, String address, String borndate,
String email, String identityCard) {
super();
this.studentno = studentno;
this.loginpwd = loginpwd;
this.studentname = studentname;
this.sex = sex;
this.gradeid = gradeid;
this.phone = phone;
this.address = address;
this.borndate = borndate;
this.email = email;
IdentityCard = identityCard;
}
//无参构造
public student(){
}
}
grade类:
package com.myschool.entity;
import java.util.List;
public class grade {
private int gradeid; //年级编号
private String gradename; //年级名称
public List<student> list; //学生表集合
public List<student> getList() {
return list;
}
public void setList(List<student> list) {
this.list = list;
}
public int getGradeid() {
return gradeid;
}
public void setGradeid(int gradeid) {
this.gradeid = gradeid;
}
public String getGradename() {
return gradename;
}
public void setGradename(String gradename) {
this.gradename = gradename;
}
//带参构造
public grade(int gradeid, String gradename) {
super();
this.gradeid = gradeid;
this.gradename = gradename;
}
private int gradeid; //年级编号
private String gradename; //年级名称
public List<student> list; //学生表集合
public List<student> getList() {
return list;
}
public void setList(List<student> list) {
this.list = list;
}
public int getGradeid() {
return gradeid;
}
public void setGradeid(int gradeid) {
this.gradeid = gradeid;
}
public String getGradename() {
return gradename;
}
public void setGradename(String gradename) {
this.gradename = gradename;
}
//带参构造
public grade(int gradeid, String gradename) {
super();
this.gradeid = gradeid;
this.gradename = gradename;
}
//无参构造
public grade(){
}
public grade(){
}
}
BaseDao接口:
package com.myschool.daos;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class BaseDao {
private final String DRIVER="com.mysql.jdbc.Driver";
private final String URL="jdbc:mysql:///myschools";
private final String USERNAME="root";
private final String PASSWORD="123";
private Connection con=null;
private PreparedStatement ps=null;
private ResultSet rs=null;
private final String URL="jdbc:mysql:///myschools";
private final String USERNAME="root";
private final String PASSWORD="123";
private Connection con=null;
private PreparedStatement ps=null;
private ResultSet rs=null;
//建立数据库连接
public Connection getConnection(){
try {
Class.forName(DRIVER);
if (con==null || con.isClosed()) {
con=DriverManager.getConnection(URL,USERNAME,PASSWORD);
}
} catch (ClassNotFoundException e) {
// TODO: handle exception
e.printStackTrace();
}catch (SQLException e) {
// TODO: handle exception
e.printStackTrace();
}
return con;
}
public Connection getConnection(){
try {
Class.forName(DRIVER);
if (con==null || con.isClosed()) {
con=DriverManager.getConnection(URL,USERNAME,PASSWORD);
}
} catch (ClassNotFoundException e) {
// TODO: handle exception
e.printStackTrace();
}catch (SQLException e) {
// TODO: handle exception
e.printStackTrace();
}
return con;
}
//增删改方法
public int executUpdate(String sql,Object...obj) throws Exception{
getConnection();
ps=con.prepareStatement(sql);
for (int i = 0; i < obj.length; i++) {
ps.setObject(i+1, obj[i]);
}
int count=ps.executeUpdate();
return count;
}
//查询方法
public ResultSet executeQuery(String sql,Object...obj) throws Exception{
getConnection();
ps=con.prepareStatement(sql);
for (int i = 0; i < obj.length; i++) {
ps.setObject(i+1, obj[i]);
}
rs = ps.executeQuery();
return rs;
}
public ResultSet executeQuery(String sql,Object...obj) throws Exception{
getConnection();
ps=con.prepareStatement(sql);
for (int i = 0; i < obj.length; i++) {
ps.setObject(i+1, obj[i]);
}
rs = ps.executeQuery();
return rs;
}
//回收资源
public void getclose() throws Exception{
if (rs!=null) {
rs.close();
}
if (ps!=null) {
ps.close();
}
if (con!=null) {
con.close();
}
}
}
public void getclose() throws Exception{
if (rs!=null) {
rs.close();
}
if (ps!=null) {
ps.close();
}
if (con!=null) {
con.close();
}
}
}
接口:
package com.myschool.daos;
import java.util.List;
import com.myschool.entity.grade;
import com.myschool.entity.student;
import com.myschool.entity.student;
public interface studentdao {
//查询所有学生,包含年级名称
public List<student> getstudent() throws Exception;
//查询S1下的学生记录
public grade getgrade(String gradeid) throws Exception;
}
public List<student> getstudent() throws Exception;
//查询S1下的学生记录
public grade getgrade(String gradeid) throws Exception;
}
实现类:
package com.myschool.dao.impl;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import java.util.ArrayList;
import java.util.List;
import com.myschool.daos.BaseDao;
import com.myschool.daos.studentdao;
import com.myschool.entity.grade;
import com.myschool.entity.student;
import com.myschool.daos.studentdao;
import com.myschool.entity.grade;
import com.myschool.entity.student;
public class studnetdaoimpl extends BaseDao implements studentdao{
private ResultSet rs=null;
@Override
public grade getgrade(String gradename) throws Exception {
List<student> list1=new ArrayList<student>();
String sql="select s.*,g.GradeName from student as s,grade as g where s.GradeId=g.GradeId and g.gradename=?";
Object[] obj={gradename};
rs=executeQuery(sql, obj);
grade grade=null;
if (rs!=null) {
while(rs.next()){
grade=new grade();
student student=new student();
student.setStudentno(rs.getInt("studentno"));
student.setStudentname(rs.getString("studentname"));
list1.add(student);
grade.setGradename(rs.getString("gradename"));
}
grade.setList(list1);
}
return grade;
}
@Override
public List<student> getstudent() throws Exception {
List<student> list=new ArrayList<student>();
String sql="select * from student as s,grade as g where s.GradeId=g.GradeId";
rs = executeQuery(sql);
if (rs!=null) {
while(rs.next()){
student stu=new student();
grade grade=new grade();
stu.setStudentno(rs.getInt("studentno"));
stu.setStudentname(rs.getString("studentname"));
grade.setGradename(rs.getString("gradename"));
stu.setGrade(grade);
list.add(stu);
}
}
return list;
}
}
main方法:
package ui;
import java.util.List;
import com.myschool.dao.impl.studnetdaoimpl;
import com.myschool.daos.studentdao;
import com.myschool.entity.grade;
import com.myschool.entity.student;
import com.myschool.daos.studentdao;
import com.myschool.entity.grade;
import com.myschool.entity.student;
public class ui {
static studentdao dao=new studnetdaoimpl();
public static void getstudent() throws Exception{
List<student> list=dao.getstudent();
for (student student : list) {
System.out.println(student.getStudentno()+" "+student.getStudentname()+" "+student.getGrade().getGradename());
}
}
public static void main(String[] args) throws Exception {
getstudent();
System.out.println("=================================");
grade grade=dao.getgrade("S1");
for (student stu : grade.getList()) {
System.out.println(stu.getStudentno()+" "+stu.getStudentname()+" "+grade.getGradename());
}
public static void getstudent() throws Exception{
List<student> list=dao.getstudent();
for (student student : list) {
System.out.println(student.getStudentno()+" "+student.getStudentname()+" "+student.getGrade().getGradename());
}
}
public static void main(String[] args) throws Exception {
getstudent();
System.out.println("=================================");
grade grade=dao.getgrade("S1");
for (student stu : grade.getList()) {
System.out.println(stu.getStudentno()+" "+stu.getStudentname()+" "+grade.getGradename());
}
}
}
执行结果:
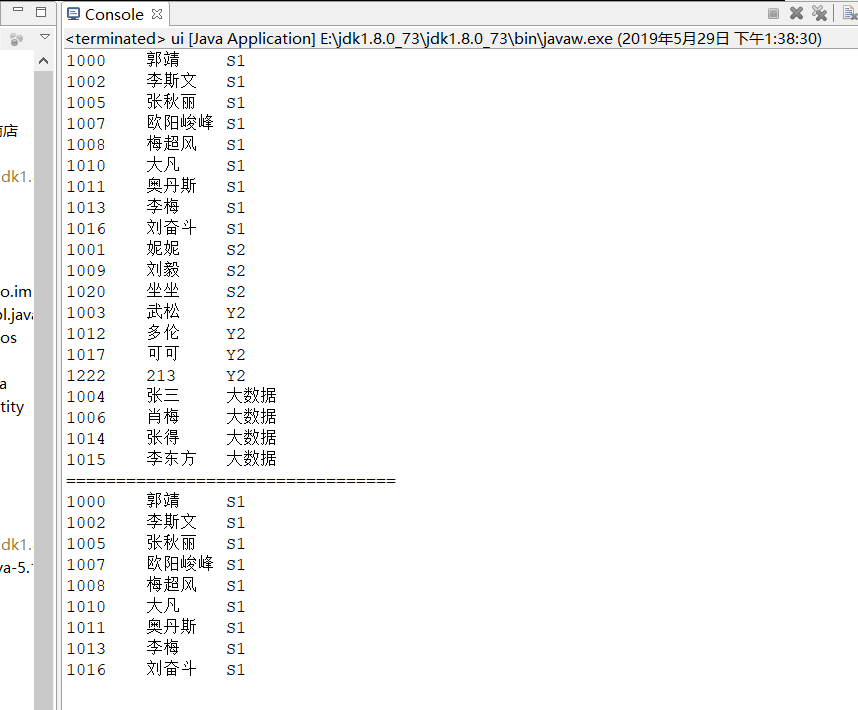