1.alert消息弹出框
alert(字符串或变量);
- 消息对话框通常可以用于调试程序。
- 与document.write 相似。
2.确认:confirm消息对话框
confirm(弹出时要显示的文本);
返回值时Boolean值;
返回值:
当点击“确定”时,返回“true”;
当点击“取消”时,返回“false”;
通过返回值可以知道用户点击了哪个按钮。
1 <!DOCTYPE HTML>
2 <html>
3 <head>
4 <meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
5 <title>confirm</title>
6 <script type="text/javascript">
7 function rec(){
8 var mymessage=confirm("请问你是女神/男神吗?");
9 if(mymessage==true)
10 {
11 document.write("我是女神!");
12 }
13 else
14 {
15 document.write("不,我是男神!");
16 }
17 }
18 </script>
19 </head>
20 <body>
21 <input name="button" type="button" onClick="rec()" value="点击我,弹出确认对话框" />
22 </body>
23 </html>
3.prompt提问消息对话框:
弹出消息对话框,通常用于询问一些需要与用户交互的信息。弹出消息对话框(包含一个确定按钮、取消按钮与一个文本输入框)。
prompt(str1, str2);
str1: 要显示在消息对话框中的文本,不可修改
str2:文本框中的内容,可以修改
1. 点击确定按钮,文本框中的内容将作为函数返回值
2. 点击取消按钮,将返回null
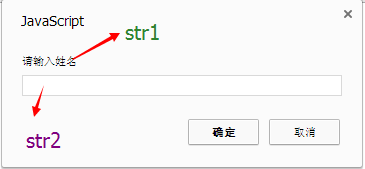
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>prompt</title>
<script type="text/javascript">
function rec(){
var myname=prompt("请输入姓名");
if(myname!=null)
{ alert("你好"+myname); }
else
{ alert("你好 my friend."); }
}
</script>
</head>
<body>
<input name="button" type="button" onClick="rec()" value="点击我,弹出确认对话框" />
</body>
</html>
效果图如上
4.window.open:打开新窗口
window.open(<URL>, <窗口打开的方法>, <参数字符串>);
参数说明:
URL:打开窗口的网址或路径。
窗口名称:被打开窗口的名称。可以是"_top"、"_blank"、"_selft"等。
参数字符串:设置窗口参数,各参数用逗号隔开。
参数表:
1 <!DOCTYPE HTML>
2 <html>
3 <head>
4 <meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
5 <title>打开新窗口</title>
6 <script type="text/javascript"> window.open('http://www.imooc.com','_blank','width=300,height=200,menubar=no,toolbar=no, status=no,scrollbars=yes')
7 </script>
8 </head>
9 <body>
10 <input name="button" type="button" onClick="rec()" value="点击我,弹出确认对话框" />
11 </body>
12 </html>
5.window.close:关闭窗口
用法:
window.close(); //关闭本窗口
或
<窗口对象>.close(); //关闭指定的窗口
例如:关闭新建的窗口。
1 <!DOCTYPE HTML>
2 <html>
3 <head>
4 <meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
5 <title>close()</title>
6 <script type="text/javascript">
7 var mywin=window.open("http://www.imooc.com");
8 mywin.close();
9 </script>
10 </head>
11 <body>
12 </body>
13 </html>
注意:上面代码在打开新窗口的同时,关闭该窗口,看不到被打开的窗口。
编程练习
制作新按钮,“新窗口打开网站” ,点击打开新窗口。
任务
1、新窗口打开时弹出确认框,是否打开
提示: 使用 if 判断确认框是否点击了确定,如点击弹出输入对话框,否则没有任何操作。
2、通过输入对话框,确定打开的网址,默认为 http://www.imooc.com/
3、打开的窗口要求,宽400像素,高500像素,无菜单栏、无工具栏。
自己的答案:
1 <!DOCTYPE html> 2 <html> 3 <head> 4 <title> new document </title> 5 <meta http-equiv="Content-Type" content="text/html; charset=gbk"/> 6 <script type="text/javascript"> 7 8 // 新窗口打开时弹出确认框,是否打开 9 function openWindow(){ 10 var newwind=confirm("是否打开新窗口?"); 11 if(newwind==true){ 12 13 var open1=prompt("请输入网址"); 14 if(open1!=null){ 15 window.open(open1,'_blank','width=400,height=500','menubar=no,toolbar=no'); 16 } 17 else{ 18 window.open('http://www.imooc.com/','_blank','width=400,height=500','men ubar=no,toolbar=no'); 19 } 20 21 } 22 else{ 23 24 document.write("我不想打开"); 25 } 26 } 27 </script> 28 </head> 29 <body> 30 <input type="button" value="新窗口打开网站" onclick="openWindow()" /> 31 </body> 32 </html>
这个是标准答案:
1 <!DOCTYPE html> 2 <html> 3 <head> 4 <title> new document </title> 5 <meta http-equiv="Content-Type" content="text/html; charset=gbk"/> 6 <script type="text/javascript"> 7 8 // 新窗口打开时弹出确认框,是否打开 9 function openWindow(){ 10 var newwind=confirm("是否打开新窗口?"); 11 if(newwind==true){ 12 13 var open1=prompt("请输入网址","http://www.imooc.com"); 14 if(open1!=null){ 15 window.open(open1,'_blank','width=400,height=500','menubar=no,toolbar=no'); 16 } 17 /* else{ 18 window.open('http://www.imooc.com/','_blank','width=400,height=500','menubar=no,toolbar=no'); 19 } */ 20 21 } 22 else{ 23 document.write("我不想打开"); 24 } 25 } 27 </script> 28 </head> 29 <body> 30 <input type="button" value="新窗口打开网站" onclick="openWindow()" /> 31 </body> 32 </html>
两者的不同之处在于:
自己的:在输入框中为空白,单击“取消”按钮会跳到默认页面,单击“确定”无法打开。是不成功的;
一个输入框里能显示出默认的网址;