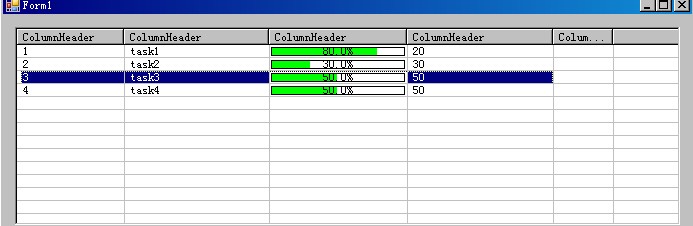
public class ListViewProgress : System.Windows.Forms.ListView
{
private string progressText;
private System.Drawing.Color progressBackColor;
public System.Drawing.Color ProgressBackColor
{
get { return progressBackColor; }
set { progressBackColor = value; }
}
private System.Drawing.Color progressTextColor;
public System.Drawing.Color ProgressTextColor
{
get { return progressTextColor; }
set { progressTextColor = value; }
}
private int progressColumnIndex = -1;
public int ProgressColumnIndex
{
get { return progressColumnIndex; }
set { progressColumnIndex = value; }
}
public ListViewProgress()
{
this.Name = "ProgressListView";
this.progressBackColor = System.Drawing.Color.YellowGreen;
this.progressTextColor = base.ForeColor;
this.OwnerDraw = true;
}
protected override void OnDrawColumnHeader(System.Windows.Forms.DrawListViewColumnHeaderEventArgs e)
{
e.DrawDefault = true;
base.OnDrawColumnHeader(e);
}
protected override void OnDrawSubItem(System.Windows.Forms.DrawListViewSubItemEventArgs e)
{
if (e.ColumnIndex == this.ProgressColumnIndex)
{
//画进度条
System.Drawing.Graphics ProgressGraphics = e.Graphics;
System.Drawing.Rectangle ProgressRect = new System.Drawing.Rectangle(
e.Bounds.Left, e.Bounds.Top, e.Bounds.Width, e.Bounds.Height);
if (ProgressRect.Height > 6 && ProgressRect.Width > 6)
{
//调整为新的区域,以便产生一定间距
ProgressRect = new System.Drawing.
Rectangle(e.Bounds.Left + 2, e.Bounds.Top + 2, e.Bounds.Width - 5, e.Bounds.Height - 5);
Single Percent = 0;
try
{
Percent = Convert.ToSingle(e.SubItem.Text);
}
catch
{
Percent = 0;
}
if (Percent > 1.0f)
Percent = Percent / 100.0f;
//外框
ProgressGraphics.FillRectangle(System.Drawing.Brushes.White, ProgressRect);
ProgressGraphics.DrawRectangle(new System.Drawing.Pen(e.SubItem.ForeColor), ProgressRect);
//内容
System.Drawing.Rectangle ProgressContentRect = new Rectangle(
ProgressRect.Left + 1, ProgressRect.Top + 1, (int)(ProgressRect.Width * Percent) - 1, ProgressRect.Height - 1 );
ProgressGraphics.FillRectangle(new System.Drawing.SolidBrush(progressBackColor), ProgressContentRect);
//输出文字
if (e.SubItem.Font.Height < ProgressRect.Height)
{
ProgressRect = new System.Drawing.Rectangle(ProgressRect.Left, ProgressRect.Top
- ((ProgressRect.Height - e.SubItem.Font.Height) / 2), ProgressRect.Width, ProgressRect.Height);
}
else
{
ProgressRect = new System.Drawing.Rectangle(e.Bounds.Left, e.Bounds.Top, e.Bounds.Width, e.Bounds.Height);
}
using (StringFormat sf = new StringFormat())
{
sf.Alignment = System.Drawing.StringAlignment.Center;
sf.LineAlignment = System.Drawing.StringAlignment.Center;
sf.Trimming = System.Drawing.StringTrimming.EllipsisCharacter;
e.Graphics.DrawString(Percent.ToString("p1"), e.SubItem.Font,
new System.Drawing.SolidBrush(this.progressTextColor), ProgressRect, sf);
}
}
base.OnDrawSubItem(e);
}
else
{
e.DrawDefault = true;
base.OnDrawSubItem(e);
}
}
}
{
private string progressText;
private System.Drawing.Color progressBackColor;
public System.Drawing.Color ProgressBackColor
{
get { return progressBackColor; }
set { progressBackColor = value; }
}
private System.Drawing.Color progressTextColor;
public System.Drawing.Color ProgressTextColor
{
get { return progressTextColor; }
set { progressTextColor = value; }
}
private int progressColumnIndex = -1;
public int ProgressColumnIndex
{
get { return progressColumnIndex; }
set { progressColumnIndex = value; }
}
public ListViewProgress()
{
this.Name = "ProgressListView";
this.progressBackColor = System.Drawing.Color.YellowGreen;
this.progressTextColor = base.ForeColor;
this.OwnerDraw = true;
}
protected override void OnDrawColumnHeader(System.Windows.Forms.DrawListViewColumnHeaderEventArgs e)
{
e.DrawDefault = true;
base.OnDrawColumnHeader(e);
}
protected override void OnDrawSubItem(System.Windows.Forms.DrawListViewSubItemEventArgs e)
{
if (e.ColumnIndex == this.ProgressColumnIndex)
{
//画进度条
System.Drawing.Graphics ProgressGraphics = e.Graphics;
System.Drawing.Rectangle ProgressRect = new System.Drawing.Rectangle(
e.Bounds.Left, e.Bounds.Top, e.Bounds.Width, e.Bounds.Height);
if (ProgressRect.Height > 6 && ProgressRect.Width > 6)
{
//调整为新的区域,以便产生一定间距
ProgressRect = new System.Drawing.
Rectangle(e.Bounds.Left + 2, e.Bounds.Top + 2, e.Bounds.Width - 5, e.Bounds.Height - 5);
Single Percent = 0;
try
{
Percent = Convert.ToSingle(e.SubItem.Text);
}
catch
{
Percent = 0;
}
if (Percent > 1.0f)
Percent = Percent / 100.0f;
//外框
ProgressGraphics.FillRectangle(System.Drawing.Brushes.White, ProgressRect);
ProgressGraphics.DrawRectangle(new System.Drawing.Pen(e.SubItem.ForeColor), ProgressRect);
//内容
System.Drawing.Rectangle ProgressContentRect = new Rectangle(
ProgressRect.Left + 1, ProgressRect.Top + 1, (int)(ProgressRect.Width * Percent) - 1, ProgressRect.Height - 1 );
ProgressGraphics.FillRectangle(new System.Drawing.SolidBrush(progressBackColor), ProgressContentRect);
//输出文字
if (e.SubItem.Font.Height < ProgressRect.Height)
{
ProgressRect = new System.Drawing.Rectangle(ProgressRect.Left, ProgressRect.Top
- ((ProgressRect.Height - e.SubItem.Font.Height) / 2), ProgressRect.Width, ProgressRect.Height);
}
else
{
ProgressRect = new System.Drawing.Rectangle(e.Bounds.Left, e.Bounds.Top, e.Bounds.Width, e.Bounds.Height);
}
using (StringFormat sf = new StringFormat())
{
sf.Alignment = System.Drawing.StringAlignment.Center;
sf.LineAlignment = System.Drawing.StringAlignment.Center;
sf.Trimming = System.Drawing.StringTrimming.EllipsisCharacter;
e.Graphics.DrawString(Percent.ToString("p1"), e.SubItem.Font,
new System.Drawing.SolidBrush(this.progressTextColor), ProgressRect, sf);
}
}
base.OnDrawSubItem(e);
}
else
{
e.DrawDefault = true;
base.OnDrawSubItem(e);
}
}
}
使用方法,数据加载 后,设置progressColumnIndex 属性即可