1.目的,因为在做前端开发的时候需要进行原生事件处理,以下代码是使用两个不同的点击事件来触发对应的内容
比如第一个是演示获取之前的按钮上的内容,第二个是获取事件属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2>1.事件处理方法v-on或@</h2>
<!--click,显示的内容是Say加上data.msg的值,点击之后调用say函数-->
<button v-on:click="say"> Say {{msg}} </button>
<button @click="warn('hello', $event)">Warn</button>
</div>
<script src="./node_modules/vue/dist/vue.js"></Script>
<script>
var vm=new Vue({
el:'#app',
data:{
msg:'hello xiaotaozi',
name:'亲爱的小桃子姐姐'
},
methods: {
say: function(event){//定义事件处理函数
//被触发时进行弹窗,弹窗内容是data.msg中的值
alert(this.name)
//event代表的是Dom原生事件,Vue.js它会自动的将它传入进来
alert(event.target.innerHTML)
},
//如果存在多个参数的情况下也需要进行Dom原生事件,
//则需要在调用的时候使用$event来进行传入,函数中使用event进行接收,为什么要传这个是为了进行Dom原生事件
warn: function (msg, event) {
alert(msg + "," + event.target.tagName)
}
},
})
</script>
</body>
</html>
事件处理
.stop
.prevent
.once
<h2>2.事件修饰符</h2>
<!--防止单击事件继续传播-->
<div @click="todo">
<!--.stop阻止事件继续传播,首先会调用doThis函数,然后再调用todo函数-->
<button @click="doThis">单击事件会继续传播</button>
</div>
<div @click="todo">
<button @click.stop="doThis">阻止单击事件会继续传播</button>
</div>
<!-- .prevent阻止事件默认行为-->
<a href="http://192.168.111.119:8888/user/login" @click.prevent="doStop">xiaotaozi网</a><br/>
<a href="http://192.168.111.119:8888/user/login">小桃子网</a><br/>
<!-- 点击事件将只会触发一次 -->
<button @click.once="doOnly">点击事件将只会触发一次: {{num}}</button>
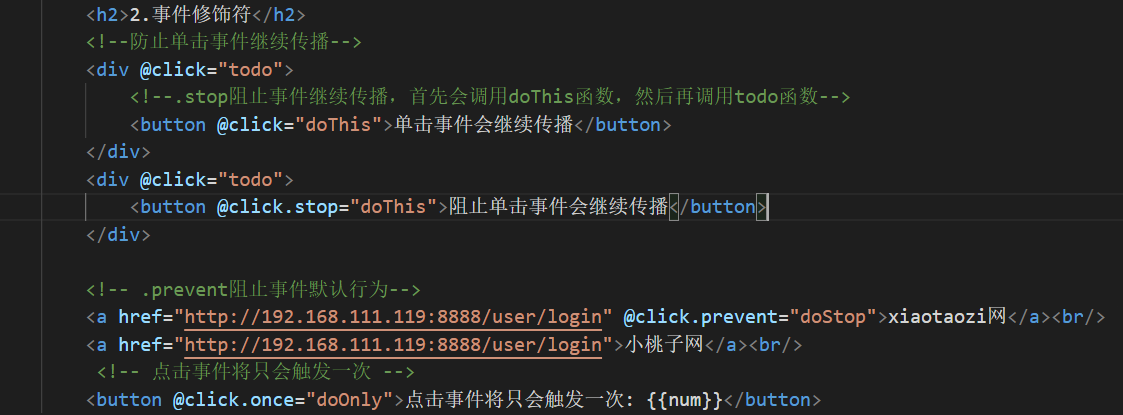
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2>1.事件处理方法v-on或@</h2>
<!--click,显示的内容是Say加上data.msg的值,点击之后调用say函数-->
<button v-on:click="say"> Say {{msg}} </button>
<button @click="warn('hello', $event)">Warn</button>
<h2>2.事件修饰符</h2>
<!--防止单击事件继续传播-->
<div @click="todo">
<!--.stop阻止事件继续传播,首先会调用doThis函数,然后再调用todo函数-->
<button @click="doThis">单击事件会继续传播</button>
</div>
<div @click="todo">
<button @click.stop="doThis">阻止单击事件会继续传播</button>
</div>
<!-- .prevent阻止事件默认行为-->
<a href="http://192.168.111.119:8888/user/login" @click.prevent="doStop">xiaotaozi网</a><br/>
<a href="http://192.168.111.119:8888/user/login">小桃子网</a><br/>
<!-- 点击事件将只会触发一次 -->
<button @click.once="doOnly">点击事件将只会触发一次: {{num}}</button>
</div>
<script src="./node_modules/vue/dist/vue.js"></Script>
<script>
var vm=new Vue({
el:'#app',
data:{
msg:'hello xiaotaozi',
name:'亲爱的小桃子姐姐',
num:0
},
methods: {
say: function(event){//定义事件处理函数
//被触发时进行弹窗,弹窗内容是data.msg中的值
alert(this.name)
//event代表的是Dom原生事件,Vue.js它会自动的将它传入进来
alert(event.target.innerHTML)
},
//如果存在多个参数的情况下也需要进行Dom原生事件,
//则需要在调用的时候使用$event来进行传入,函数中使用event进行接收,为什么要传这个是为了进行Dom原生事件
warn: function (msg, event) {
alert(msg + "," + event.target.tagName)
} ,
todo: function () {
alert("todo....");
},
doThis: function () {
alert("doThis....");
},
doStop: function () {
alert("href默认跳转被阻止....")
},
doOnly: function() {
this.num++
}
},
})
</script>
</body>
</html>
按键修饰符
.enter
.tab
.delete
.esc
.space
.up
.down
.left
.right
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2>1.事件处理方法v-on或@</h2>
<!--click,显示的内容是Say加上data.msg的值,点击之后调用say函数-->
<button v-on:click="say"> Say {{msg}} </button>
<button @click="warn('hello', $event)">Warn</button>
<h2>2.事件修饰符</h2>
<!--防止单击事件继续传播-->
<div @click="todo">
<!--.stop阻止事件继续传播,首先会调用doThis函数,然后再调用todo函数-->
<button @click="doThis">单击事件会继续传播</button>
</div>
<div @click="todo">
<button @click.stop="doThis">阻止单击事件会继续传播</button>
</div>
<!-- .prevent阻止事件默认行为-->
<a href="http://192.168.111.119:8888/user/login" @click.prevent="doStop">xiaotaozi网</a><br/>
<a href="http://192.168.111.119:8888/user/login">小桃子网</a><br/>
<!-- 点击事件将只会触发一次 -->
<button @click.once="doOnly">点击事件将只会触发一次: {{num}}</button>
<h2>3.按键修饰符</h2>
<input @keyup.enter="keyEnter"><!--进入输入框按回车时调用keyEnter-->
<input @keyup.space="keySpace"><!--进入输入框按回车时调用keySpace-->
</div>
<script src="./node_modules/vue/dist/vue.js"></Script>
<script>
var vm=new Vue({
el:'#app',
data:{
msg:'hello xiaotaozi',
name:'亲爱的小桃子姐姐',
num:0
},
methods: {
say: function(event){//定义事件处理函数
//被触发时进行弹窗,弹窗内容是data.msg中的值
alert(this.name)
//event代表的是Dom原生事件,Vue.js它会自动的将它传入进来
alert(event.target.innerHTML)
},
//如果存在多个参数的情况下也需要进行Dom原生事件,
//则需要在调用的时候使用$event来进行传入,函数中使用event进行接收,为什么要传这个是为了进行Dom原生事件
warn: function (msg, event) {
alert(msg + "," + event.target.tagName)
} ,
todo: function () {
alert("todo....");
},
doThis: function () {
alert("doThis....");
},
doStop: function () {
alert("href默认跳转被阻止....")
},
doOnly: function() {
this.num++
},
keyEnter: function () {
alert("已按:回车键")
},
keySpace: function () {
alert("已按:空格键")
}
},
})
</script>
</body>
</html>