原文:https://blog.csdn.net/qq_37936542/article/details/78549233
access_token:公众号的全局唯一接口调用凭据,公众号调用各接口时都需使用access_token。开发者需要进行妥善保存。access_token的存储至少要保留512个字符空间。access_token的有效期目前为2个小时,需定时刷新,重复获取将导致上次获取的access_token失效。
jsapi_ticket:jsapi_ticket是公众号用于调用微信JS接口的临时票据。正常情况下,jsapi_ticket的有效期为7200秒,通过access_token来获取。由于获取jsapi_ticket的api调用次数非常有限,频繁刷新jsapi_ticket会导致api调用受限,影响自身业务,开发者必须在自己的服务全局缓存jsapi_ticket 。
由于上述两个标识获取都有限制,而且有效期是两个小时,所以开发时设置为每一小时获取一次
(一):导入httpclient依赖和jackson依赖
- <!-- httpclient -->
- <dependency>
- <groupId>org.apache.httpcomponents</groupId>
- <artifactId>httpclient</artifactId>
- <version>4.1.2</version>
- </dependency>
- <dependency>
- <groupId>org.apache.httpcomponents</groupId>
- <artifactId>httpclient-cache</artifactId>
- <version>4.1.2</version>
- </dependency>
- <dependency>
- <groupId>org.apache.httpcomponents</groupId>
- <artifactId>httpmime</artifactId>
- <version>4.1.2</version>
- </dependency>
- <!-- jack json -->
- <dependency>
- <groupId>com.fasterxml.jackson.core</groupId>
- <artifactId>jackson-core</artifactId>
- <version>2.7.3</version>
- </dependency>
- <dependency>
- <groupId>com.fasterxml.jackson.core</groupId>
- <artifactId>jackson-annotations</artifactId>
- <version>2.7.3</version>
- </dependency>
- <dependency>
- <groupId>com.fasterxml.jackson.core</groupId>
- <artifactId>jackson-databind</artifactId>
- <version>2.7.3</version>
- </dependency>
(二):封装httpclient处理get和post请求
- import java.io.IOException;
- import java.util.List;
- import org.apache.http.HttpResponse;
- import org.apache.http.NameValuePair;
- import org.apache.http.client.HttpClient;
- import org.apache.http.client.entity.UrlEncodedFormEntity;
- import org.apache.http.client.methods.HttpGet;
- import org.apache.http.client.methods.HttpPost;
- import org.apache.http.entity.StringEntity;
- import org.apache.http.impl.client.DefaultHttpClient;
- import org.apache.http.util.EntityUtils;
- import com.fasterxml.jackson.databind.ObjectMapper;
- public class CommonUtils {
- private static HttpClient CLIENT = new DefaultHttpClient();
- private static ObjectMapper MAPPER = new ObjectMapper();
- public static String APPID ="wx273a3bf59a7d2fee";
- public static String SECRET="0ca672094780b3ecf9cedcf35891b129";
- /**
- * 根据url获取返回对象
- * @param <T>
- * @param url
- * @return
- * @throws Exception
- */
- public static <T> T returnMsg(String url,Class<T> clazz) throws Exception{
- HttpPost msgPost = new HttpPost(url);
- String str = EntityUtils.toString(CLIENT.execute(msgPost).getEntity(),"UTF-8");
- return MAPPER.readValue(str, clazz);
- }
- /**
- * httpclient模拟get请求
- */
- public static String Get(String url) throws Exception{
- HttpGet httpGet = new HttpGet(url);
- String str = EntityUtils.toString(CLIENT.execute(httpGet).getEntity(),"UTF-8");
- return str;
- }
- /**
- * http模拟post请求,请求参数是json数据
- * @param url
- * @param param
- * @return
- * @throws IOException
- * @throws Exception
- */
- public static String Post_Json(String url, String param) throws Exception{
- HttpPost httpPost = new HttpPost(url);
- //防止参数乱码
- httpPost.addHeader("Content-type","application/json; charset=utf-8");
- httpPost.setEntity(new StringEntity(param, "UTF-8"));
- //执行请求
- HttpResponse execute = CLIENT.execute(httpPost);
- String resp = EntityUtils.toString(execute.getEntity());
- return resp;
- }
- /**
- * httpclient模拟表单提交
- * @param url
- * @param param
- * @return
- * @throws Exception
- */
- public static String Post_From(String url, List<NameValuePair> list) throws Exception{
- HttpPost httpPost = new HttpPost(url);
- //防止参数乱码
- httpPost.addHeader("Content-type","application/json; charset=utf-8");
- UrlEncodedFormEntity entity = new UrlEncodedFormEntity(list, "UTF-8");
- httpPost.setEntity(entity);
- //执行请求
- HttpResponse execute = CLIENT.execute(httpPost);
- String resp = EntityUtils.toString(execute.getEntity());
- return resp;
- }
- }
(三):获取access_token和jsapi_ticket
- import org.springframework.stereotype.Service;
- import com.mote.weixin.utils.CommonUtils;
- /**
- * 定时刷新access_token--微信全局凭证
- * 定时刷新jsapi_ticket--调用微信js接口的临时票据
- * @author lenovo
- *
- */
- @Service("tokenService")
- public class TokenService {
- private String access_token;
- private String jsapi_ticket;
- public String getAccess_token() {
- return access_token;
- }
- public void setAccess_token(String access_token) {
- this.access_token = access_token;
- }
- public String getJsapi_ticket() {
- return jsapi_ticket;
- }
- public void setJsapi_ticket(String jsapi_ticket) {
- this.jsapi_ticket = jsapi_ticket;
- }
- /**
- * 将该方法配置成定时任务,程序启动后五秒自动调用,
- * 之后每隔一小时刷新一次
- */
- public void flush(){
- System.out.println("===================我被调用了=================");
- try {
- //获取access_token
- String accessToken = CommonUtils.getAccessToken();
- this.access_token = accessToken;
- //获取jsapi_ticket
- String jsApiTicket = CommonUtils.getJsApiTicket(accessToken);
- this.jsapi_ticket = jsApiTicket;
- } catch (Exception e) {
- System.out.println("刷新access_token失败");
- e.printStackTrace();
- }
- }
- }
(四):定义AccessToken对象,然后在CommonUtils中添加获取access_token和jsapi_ticket的方法
- public class AccessToken {
- // 接口访问凭证
- private String accessToken;
- // 凭证有效期,单位:秒
- private int expiresIn;
- public String getAccessToken() {
- return accessToken;
- }
- public void setAccessToken(String accessToken) {
- this.accessToken = accessToken;
- }
- public int getExpiresIn() {
- return expiresIn;
- }
- public void setExpiresIn(int expiresIn) {
- this.expiresIn = expiresIn;
- }
- }
- /**
- * 获取access_token
- * @return
- * @throws Exception
- */
- public static String getAccessToken() throws Exception{
- String url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid="+APPID+"&secret="+SECRET+"";
- AccessToken token = returnMsg(url, AccessToken.class);
- return token.getAccessToken();
- }
- /**
- * 获取调用微信JS接口的临时票据
- *
- * @param access_token 微信全局凭证
- * @return
- * @throws IOException
- * @throws Exception
- */
- public static String getJsApiTicket(String access_token) throws Exception{
- String url = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?access_token="+access_token+"&type=jsapi";
- // 发起GET请求获取凭证
- String resq = Get(url);
- JsonNode tree = MAPPER.readTree(resq);
- return tree.get("ticket").toString().replaceAll(""", "");
- }
(五):配置TokenService中的flush方法,使其生效
注意:引入xml约束
- xmlns:task="http://www.springframework.org/schema/task"
- http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-4.0.xsd">
配置如下:
- <!-- 如果TokeService添加了注解,bean可以省略 -->
- <bean id="tokenService" class="com.mote.wx.service.TokenService">
- </bean>
- <!-- spring自带的定时刷新任务 -->
- <task:scheduled-tasks>
- <!-- 程序启动5秒后执行方法,之后每隔一小时执行 -->
- <task:scheduled ref="tokenService" method="flush" initial-delay="5000" fixed-delay="3600000" />
- <!-- <task:scheduled ref="statJob" method="statLgj" cron="0 59 23 * * ?" /> -->
- </task:scheduled-tasks>
文末福利:
福利一:前端,Java,产品经理,微信小程序,Python等10G资源合集大放送:https://www.jianshu.com/p/e8197d4d9880
福利二:微信小程序入门与实战全套详细视频教程。
【领取方法】
关注 【编程微刊】微信公众号:
回复【小程序demo】一键领取130个微信小程序源码demo资源。
回复【领取资源】一键领取前端,Java,产品经理,微信小程序,Python等资源合集10G资源大放送。
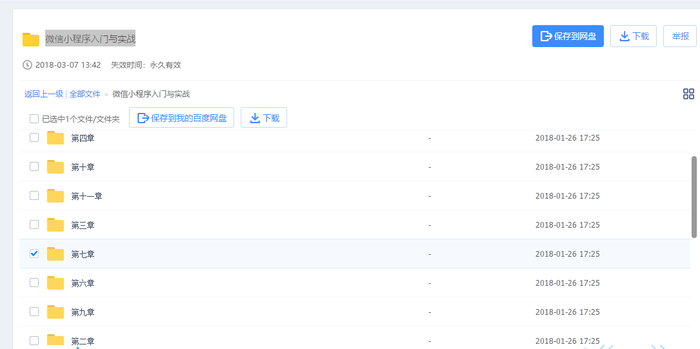
原文作者:祈澈姑娘
原文链接:https://www.jianshu.com/u/05f416aefbe1
创作不易,转载请告知
90后前端妹子,爱编程,爱运营,爱折腾。
坚持总结工作中遇到的技术问题,坚持记录工作中所所思所见,欢迎大家一起探讨交流。