// 按顺序比较大小
public class equals_线性查询 {
public static void main(String[] args) {
String[] arr = new String[]{"AA", "BB", "CC", "DD", "FF"};
String dest = "BB";
for (int i = 0; i < arr.length; i++){
if (dest.equals(arr[i])){
System.out.println("元素的索引是" + i);
System.out.println(dest.equals(arr[i]));
break;
}
}
}
}
// 前提: 必须是有序数组 优势:快
public class 二分法查找 {
public static void main(String [] args){
int[] arr = new int[]{-98,-34,2,34,54,66,79,105,210,333};
int dest = -34;
int head = 0; // 初始的首索引
int end = arr.length - 1; // 末索引
// 对半比较
while (head <= end ){
int middle = (head + end) / 2;
if (dest == arr[middle]){
System.out.println("元素的索引是" + middle);
break;
}
else if( arr[middle] > dest ){
end = middle - 1;
}else {
head = middle + 1;
}
}
}
}
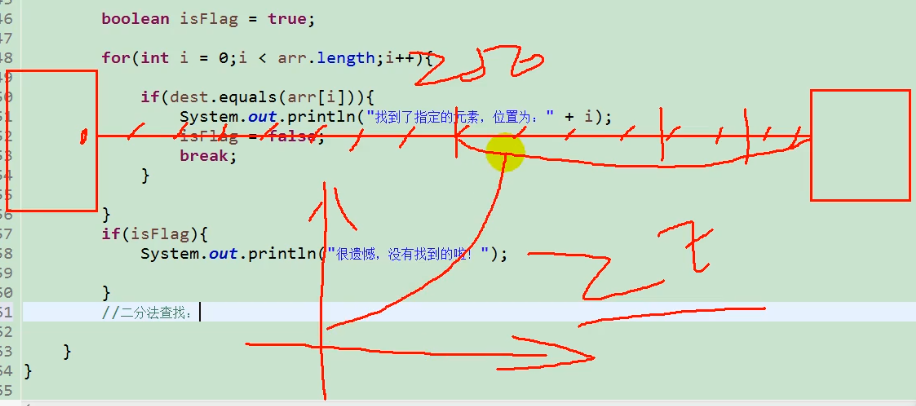
// 循环遍历判断两个相邻的数值,大小调换位置
public class 冒泡排序 {
public static void main(String[] args) {
int[] arr = new int[]{98, 34, 2, 34, 54, 66, 79, 105, 210, 333};
// 冒泡排序
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - 1;j++ ){
// 如果后面一个数 大于 前面一个数 就调换位置
if (arr[j] > arr[j+1]){
int temp = arr[j];
arr[i] = arr[j+1];
arr[j+1] = temp;
}
}
}
// 显示重置后的
for (int x : arr) {
System.out.println(x);
}
}
}
// 输出:
// 79
// 98
// 98
// 98
// 98
// 98
// 98
// 105
// 210
// 333
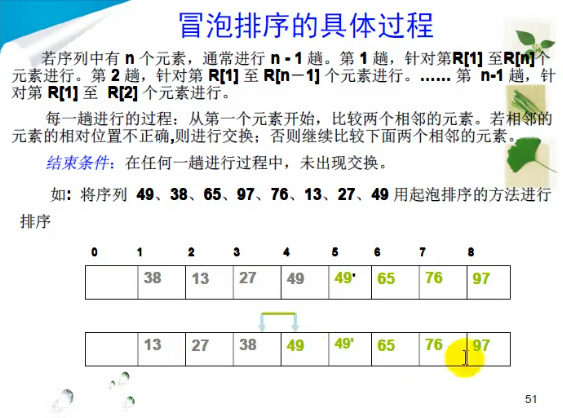
// 快速排序
/*
每次循环都会是以1 2 4 8。。。 的比例进行重组排序
说明:
1:循环取数组第一个值(Pivot),
2:定义两个指针,一个在第一个值的后面(low),另外一个是最尾巴的数值(high)
3:接着类似于 low向尾巴移动,high向头移动, 在移动中,只要满足low 大于 Pivot 并且 high 小于 Pivot 的两个数就调换位置
4:当停止时,相当于high与low的位置相邻,判断 如果high 小于 Pivot的值,就调换两个位置
5:这个时候会发现,左边都是小于Pivot的,右边都是大于Pivot的
6:针对左右两个数组进行一次 1~5的步骤
*/
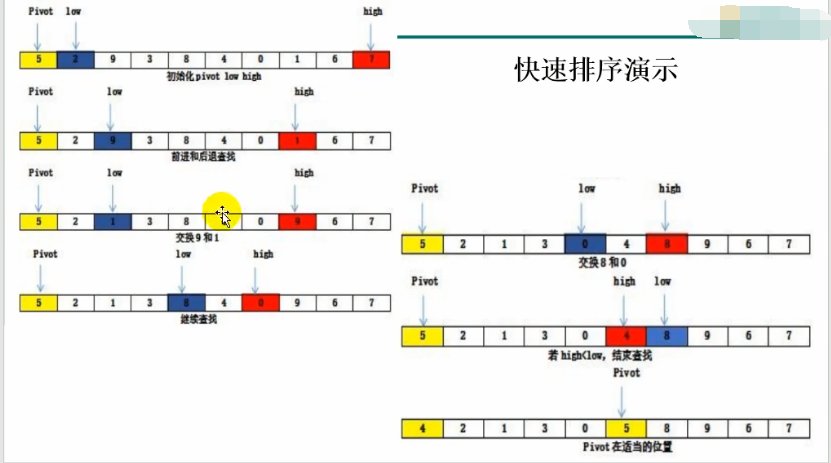