Kakuro Extension
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Special Judge
Description
If you solved problem like this, forget it.Because you need to use a completely different algorithm to solve the following one.
Kakuro puzzle is played on a grid of "black" and "white" cells. Apart from the top row and leftmost column which are entirely black, the grid has some amount of white cells which form "runs" and some amount of black cells. "Run" is a vertical or horizontal maximal one-lined block of adjacent white cells. Each row and column of the puzzle can contain more than one "run". Every white cell belongs to exactly two runs — one horizontal and one vertical run. Each horizontal "run" always has a number in the black half-cell to its immediate left, and each vertical "run" always has a number in the black half-cell immediately above it. These numbers are located in "black" cells and are called "clues".The rules of the puzzle are simple:
1.place a single digit from 1 to 9 in each "white" cell
2.for all runs, the sum of all digits in a "run" must match the clue associated with the "run"
Given the grid, your task is to find a solution for the puzzle.
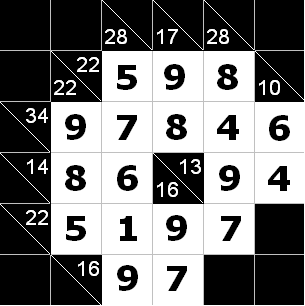
Picture of the first sample input Picture of the first sample output
Kakuro puzzle is played on a grid of "black" and "white" cells. Apart from the top row and leftmost column which are entirely black, the grid has some amount of white cells which form "runs" and some amount of black cells. "Run" is a vertical or horizontal maximal one-lined block of adjacent white cells. Each row and column of the puzzle can contain more than one "run". Every white cell belongs to exactly two runs — one horizontal and one vertical run. Each horizontal "run" always has a number in the black half-cell to its immediate left, and each vertical "run" always has a number in the black half-cell immediately above it. These numbers are located in "black" cells and are called "clues".The rules of the puzzle are simple:
1.place a single digit from 1 to 9 in each "white" cell
2.for all runs, the sum of all digits in a "run" must match the clue associated with the "run"
Given the grid, your task is to find a solution for the puzzle.
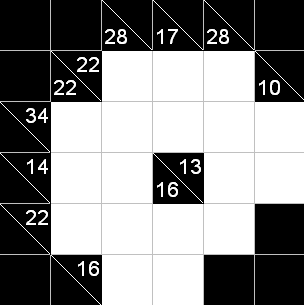
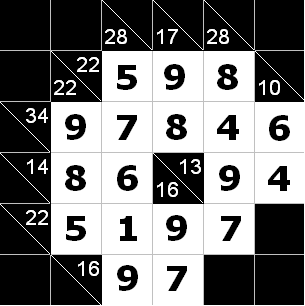
Picture of the first sample input Picture of the first sample output
Input
The first line of input contains two integers n and m (2 ≤ n,m ≤ 100) — the number of rows and columns correspondingly. Each of the next n lines contains descriptions of m cells. Each cell description is one of the following 7-character strings:
.......— "white" cell;
XXXXXXX— "black" cell with no clues;
AAABBB— "black" cell with one or two clues. AAA is either a 3-digit clue for the corresponding vertical run, or XXX if there is no associated vertical run. BBB is either a 3-digit clue for the corresponding horizontal run, or XXX if there is no associated horizontal run.
The first row and the first column of the grid will never have any white cells. The given grid will have at least one "white" cell.It is guaranteed that the given puzzle has at least one solution.
.......— "white" cell;
XXXXXXX— "black" cell with no clues;
AAABBB— "black" cell with one or two clues. AAA is either a 3-digit clue for the corresponding vertical run, or XXX if there is no associated vertical run. BBB is either a 3-digit clue for the corresponding horizontal run, or XXX if there is no associated horizontal run.
The first row and the first column of the grid will never have any white cells. The given grid will have at least one "white" cell.It is guaranteed that the given puzzle has at least one solution.
Output
Print n lines to the output with m cells in each line. For every "black" cell print '_' (underscore), for every "white" cell print the corresponding digit from the solution. Delimit cells with a single space, so that each row consists of 2m-1 characters.If there are many solutions, you may output any of them.
Sample Input
6 6
XXXXXXX XXXXXXX 028XXX 017XXX 028XXX XXXXXXX
XXXXXXX 022 22 ....... ....... ....... 010XXX
XXX 34 ....... ....... ....... ....... .......
XXX 14 ....... ....... 016 13 ....... .......
XXX 22 ....... ....... ....... ....... XXXXXXX
XXXXXXX XXX 16 ....... ....... XXXXXXX XXXXXXX
5 8
XXXXXXX 001XXX 020XXX 027XXX 021XXX 028XXX 014XXX 024XXX
XXX 35 ....... ....... ....... ....... ....... ....... .......
XXXXXXX 007 34 ....... ....... ....... ....... ....... .......
XXX 43 ....... ....... ....... ....... ....... ....... .......
XXX 30 ....... ....... ....... ....... ....... ....... XXXXXXX
Sample Output
_ _ _ _ _ _
_ _ 5 8 9 _
_ 7 6 9 8 4
_ 6 8 _ 7 6
_ 9 2 7 4 _
_ _ 7 9 _ _
_ _ _ _ _ _ _ _
_1 9 9 1 1 8 6
_ _ 1 7 7 9 1 9
_ 1 3 9 9 9 3 9
_ 6 7 2 4 9 2 _
分析
神奇的最大流
可以将左边的有数字的黑色格子看做源点,空白格看做节点,上面有数字的黑色格子看做汇点,跑最大流。
填入的数字是1~9,可以减一,就是容量为0~8,最后再加回来。
#include <stdio.h> #include <string.h> #include <iostream> #include <algorithm> using namespace std; const int MAXN=20010; const int MAXE=200010; const int INF=0x3f3f3f3f; struct Node { int to,next,cap; }edge[MAXE]; int tol; int head[MAXN]; int gap[MAXN],dis[MAXN],pre[MAXN],cur[MAXN]; void init() { tol=0; memset(head,-1,sizeof(head)); } void addedge(int u,int v,int w,int rw=0) { edge[tol].to=v;edge[tol].cap=w;edge[tol].next=head[u];head[u]=tol++; edge[tol].to=u;edge[tol].cap=rw;edge[tol].next=head[v];head[v]=tol++; } int sap(int start,int end,int nodenum) { memset(dis,0,sizeof(dis)); memset(gap,0,sizeof(gap)); memcpy(cur,head,sizeof(head)); int u=pre[start]=start,maxflow=0,aug=-1; gap[0]=nodenum; while(dis[start]<nodenum) { loop: for(int &i=cur[u];i!=-1;i=edge[i].next) { int v=edge[i].to; if(edge[i].cap&&dis[u]==dis[v]+1) { if(aug==-1||aug>edge[i].cap) aug=edge[i].cap; pre[v]=u; u=v; if(v==end) { maxflow+=aug; for(u=pre[u];v!=start;v=u,u=pre[u]) { edge[cur[u]].cap-=aug; edge[cur[u]^1].cap+=aug; } aug=-1; } goto loop; } } int mindis=nodenum; for(int i=head[u];i!=-1;i=edge[i].next) { int v=edge[i].to; if(edge[i].cap&&mindis>dis[v]) { cur[u]=i; mindis=dis[v]; } } if((--gap[dis[u]])==0)break; gap[dis[u]=mindis+1]++; u=pre[u]; } return maxflow; } char str[110][110][10]; int id[110][110]; int lx[110][110]; int ly[110][110]; int num[20010]; int main() { int n,m; while(scanf("%d%d",&n,&m)!=EOF){ for(int i=0;i<n;i++) for(int j=0;j<m;j++) scanf("%s",str[i][j]); init(); int tt=0; memset(lx,0,sizeof(lx)); memset(ly,0,sizeof(ly)); memset(num,0,sizeof(num)); for(int i=0;i<n;i++){ for(int j=0;j<m;j++){ if(strcmp(str[i][j],".......")==0){ if(j==0||lx[i][j-1]==0) lx[i][j]=++tt; else lx[i][j]=lx[i][j-1]; num[lx[i][j]]++; } } } for(int j=0;j<m;j++){ for(int i=0;i<n;i++){ if(strcmp(str[i][j],".......")==0){ if(i==0||ly[i-1][j]==0) ly[i][j]=++tt; else ly[i][j]=ly[i-1][j]; num[ly[i][j]]++; } } } int st=0,ed=tt+1,nodenum=tt+2; for(int i=0;i<n;i++){ for(int j=0;j<m;j++){ if(strcmp(str[i][j],".......")==0){ addedge(lx[i][j],ly[i][j],8); id[i][j]=tol-2; } } } for(int i=0;i<n;i++){ for(int j=0;j<m;j++){ if(str[i][j][3]!='\') continue; if(str[i][j][0]!='X'){ int tmp=(str[i][j][0]-'0')*100+(str[i][j][1]-'0')*10+(str[i][j][2]-'0'); if(ly[i+1][j]!=0) addedge(ly[i+1][j],ed,tmp-num[ly[i+1][j]]); } if(str[i][j][4]!='X'){ int tmp=(str[i][j][4]-'0')*100+(str[i][j][5]-'0')*10+(str[i][j][6]-'0'); if(lx[i][j+1]!=0) addedge(st,lx[i][j+1],tmp-num[lx[i][j+1]]); } } } sap(st,ed,nodenum); for(int i=0;i<n;i++){ for(int j=0;j<m;j++){ if(j>0) printf(" "); if(strcmp(str[i][j],".......")!=0) printf("_"); else printf("%c",'0'+9-edge[id[i][j]].cap); } printf(" "); } } return 0; }