1.列表的抽象数据类型定义
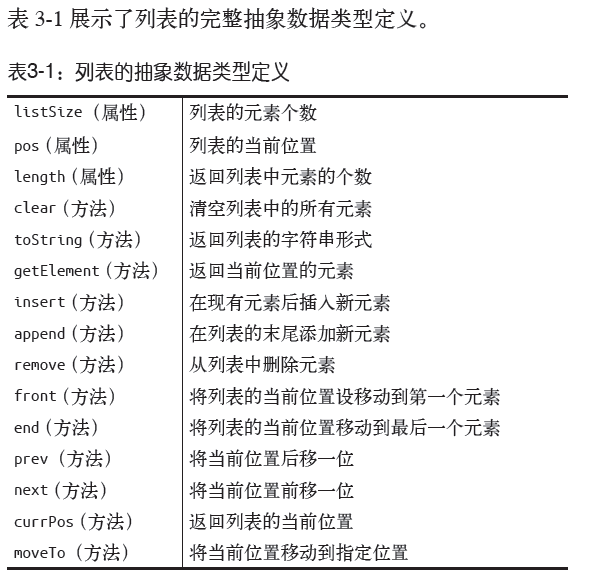
2.实现列表类:
2.1 append:给列表添加元素:
2.2 remove: 从列表中删除元素:
2.3 find方法:
2.4 length:列表中有多少个元素:
2.5 toString:显示列表中的元素
2.6 insert:向列表中插入一个元素
2.7 clear:清空列表中所有的元素
2.8 contains:判断给定值是否在列表中
2.9 遍历列表 front、end、prev、next;一组方法允许用户在列表上自由移动;getElement()返回列表的当前元素;
3.使用迭代器访问列表
使用迭代器,可以不必关心数据的内部存储方式,以实现对列表的遍历。前面提到的方法front()、end()、prev()、next() 和currPos 就实现了List 类的一个迭代器。
以下是和使用数组索引的方式相比,使用迭代器的一些优点:
•访问列表元素时不必关心底层的数据存储结构。
• 当为列表添加一个元素时,索引的值就不对了,此时只用更新列表,而不用更新迭代器。
• 可以用不同类型的数据存储方式实现 List 类,迭代器为访问列表里的元素提供了一种统一的方式。
例子:
for(names.front(); names.currPos() < names.length(); names.next()) {
print(names.getElement());
}
代码实现:
function List(){ this.listSize = 0; this.pos = 0; this.dataStore = []; // 初始化一个空数组来保存列表元素 this.clear = clear; this.find = find; this.toString = toString; this.insert = insert; this.append = append; this.remove = remove; this.front = front; this.end = end; this.prev = prev; this.next = next; this.length = length; this.currPos = currPos; this.moveTo = moveTo; this.getElement = getElement; this.contains = contains; } function append(element){ this.dataStore[this.listSize] = element; this.listSize ++; } function remove(element){ var fountAt = this.find(element); if(fountAt > -1){ this.dataStore.splice(fountAt,1); this.listSize -- ; return true; } return false; } function find(element){ for(var i=0; i<this.dataStore.length; ++i){ if(this.dataStore[i] == element){ return i; } } return -1; } function length(){ return this.listSize; } function toString(){ return this.dataStore; } function insert(element, after){ var insertPos = this.find(after); if (insertPos > -1) { this.dataStore.splice(insertPos+1, 0, element); this.listSize ++; return true; } return false; } function clear(){ delete this.dataStore; this.dataStore = []; this.listSize = 0; this.pos = 0; } function contains(element){ for(var i=0; i<this.dataStore.length; ++i){ if(this.dataStore[i] == element){ return true; } } return false; } function front(){ this.pos = 0; } function end(){ this.pos = this.listSize - 1; } function prev(){ if(this.pos > 0){ this.pos --; } } function next(){ if(this.pos < this.listSize - 1){ this.pos ++; } } function currPos(){ return this.pos; } function moveTo(position){ this.pos = position; } function getElement() { return this.dataStore[this.pos]; }
测试代码:

//测试代码 var names = new List(); names.append("Clayton"); names.append("Raymond"); names.append("Cynthia"); names.append("Jennifer"); names.append("Bryan"); names.append("Danny"); names.front(); console.log(names.getElement()); // 显示Clayton names.next(); console.log(names.getElement()); // 显示Raymond names.next(); names.next(); names.prev(); console.log(names.getElement()); // 显示Cynthia //迭代器遍历,由于names.next()得到的this.pos 永远小于 name.length这里有点问题 // for(names.front(); names.currPos() < names.length(); names.next()){ // console.log(names.getElement()); // } // for(names.end(); names.currPos() >= 0; names.prev()) { // console.log(names.getElement()); // }