1 项目总体结构
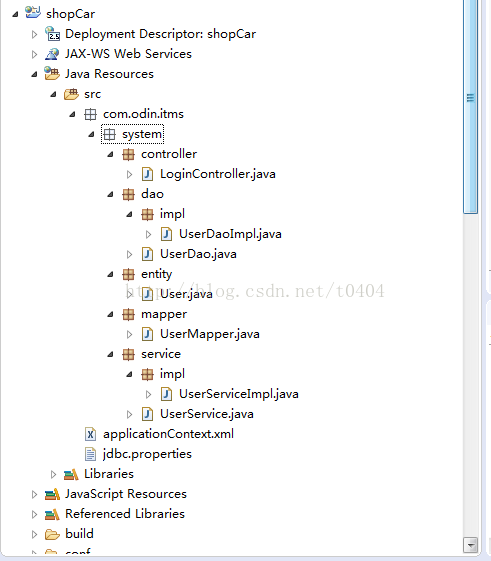
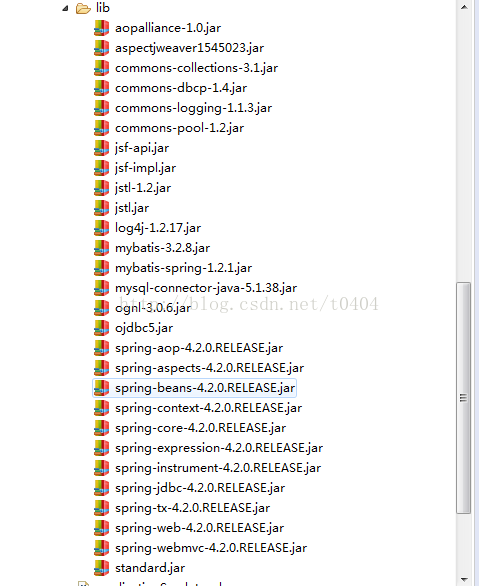
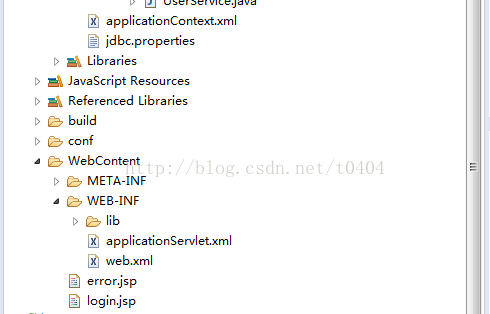
2 web.xml的配置
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns:javaee="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<!--
spring root配置文件的位置
-->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</context-param>
<!-- 通过监听器 在上下文被加载时 自动启动
并且创建 WebApplicationContext对象 去加载spring中所有的bean -->
<listener>
<listener-class>
org.springframework.web.context.ContextLoaderListener
</listener-class>
</listener>
<!-- springmvc的装载 -->
<servlet>
<servlet-name>SpringMvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
<!-- 读取springmvc的配置文件 -->
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/applicationServlet.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>SpringMvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
3 applicationContext.xml的配置
<?xml version="1.0" encoding="UTF-8"?>
<beans
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.1.xsd
">
<!-- 扫描除了@Controller的注解 -->
<context:component-scan base-package="com.odin.itms.*">
<context:exclude-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
</context:component-scan>
<!-- 加载jdbc文件 -->
<context:property-placeholder location="classpath:jdbc.properties"/>
<!-- 连接数据库,获取数据源 -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="url" value="${url}"></property>
<property name="driverClassName" value="${driverClassName}"></property>
<property name="username" value="${uname}"></property>
<property name="password" value="${password}"></property>
</bean>
<!--集成mybatis -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
</bean>
<!-- 创建SqlSessionTemplate用于控制事务自动提交 也可以是用 SqlSession中的所有方法-->
<bean id="sqlTemplate" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg index="0" ref="sqlSessionFactory"></constructor-arg>
</bean>
<!-- 配置接口映射扫描工具类 -->
<bean id="mapperScannerConfigurer" class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.odin.itms.*.mapper"></property>
<property name="beanName" value="sqlSessionFactory"></property>
</bean>
<!-- 配置全局事务管理器 -->
<bean id="dataSourceTx" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"></property>
</bean>
<!-- 配置事务的消息 -->
<tx:advice id="txAdvise" transaction-manager="dataSourceTx">
<tx:attributes>
<tx:method name="add*" isolation="DEFAULT" propagation="REQUIRED"/>
<tx:method name="update*" isolation="DEFAULT" propagation="REQUIRED"/>
<tx:method name="delete*" isolation="DEFAULT" propagation="REQUIRED"/>
<tx:method name="*" read-only="true"/>
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut expression="execution(* com.odin.itms.*.service.*(..))" id="pointCutRef"/>
<aop:advisor advice-ref="txAdvise" pointcut-ref="pointCutRef"/>
</aop:config>
</beans>
4 applicatonServlet.xml的配置
<?xml version="1.0" encoding="UTF-8"?>
<beans
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd"
>
<!-- 配置springmvc 加载含有@Controller的bean -->
<context:component-scan base-package="com.odin.itms.*">
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
</context:component-scan>
<!-- 启动注解驱动 -->
<mvc:annotation-driven></mvc:annotation-driven>
</beans>
5 jdbc.properties的配置
url=jdbc:oracle:thin:@localhost:1521:orcl
driverClassName=oracle.jdbc.OracleDriver
uname=zt
password=zt
6 login.jsp代码
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<form action="login/login.do" method="post">
用户:<input type="text" name="userName"/>
<br />
密码:<input type="password" name="password"/>
<br/>
<input type="submit" value="登录"/>
<input type="reset"/>
</form>
</body>
</html>
7 controller层的代码
package com.odin.itms.system.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import com.odin.itms.system.entity.User;
import com.odin.itms.system.service.UserService;
@Controller
@RequestMapping(value="login")
public class LoginController {
@Autowired
public UserService userService;
@RequestMapping("/login")
public String login(String userName,String password,ModelMap model){
String msg="";
User user=userService.login(userName);
if(user==null){
msg="用户不存在";
model.addAttribute("msg", msg);
return "forward:/error.jsp";
}else if(user!=null){
String pw=user.getPassword();
if(pw!=null&&!"".equals(pw)){
if(pw.equals(password)){
msg="登录成功";
model.addAttribute("msg", msg);
}else{
model.addAttribute("msg", msg);
msg="账号或密码错误";
}
}else{
model.addAttribute("msg", msg);
msg="账号或密码错误";
}
}
return "forward:/error.jsp";
}
}
8 service层代码(dao层类似)
1 实现类
package com.odin.itms.system.service.impl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.odin.itms.system.dao.UserDao;
import com.odin.itms.system.entity.User;
import com.odin.itms.system.service.UserService;
@Service
public class UserServiceImpl implements UserService {
@Autowired
public UserDao userDao;
public User login(String username) {
return userDao.login(username);
}
}
2 接口
package com.odin.itms.system.service;
import com.odin.itms.system.entity.User;
public interface UserService {
public User login(String username);
}
9 mapper层代码
package com.odin.itms.system.mapper;
import org.apache.ibatis.annotations.Select;
import com.odin.itms.system.entity.User;
public interface UserMapper {
@Select("select * from use t where username=#{userName}")
public User login(String userName);
}