从深度图中提取物体边界
一、深度图像的边界
从深度图像中提取边界。 其中我们对3种类型的点感兴趣:物体边界,物体最外层和阴影边界的可见点集;
阴影边界,连接于遮挡的背景上的点集; Veil点集,在被遮挡物边界和阴影边界之间的内插点。
如下图所示:
- 边界
- 从前景跨越到背景的位置定义为边界
提取边界信息时很重要的一点是区分深度图像中的当前视点(从当前的视角看过去)不可见点集合(被遮挡等)和应该可见但处于传感器获取距离范围外的点集(即典型边界)。 当前视点不可见点则不能成为边界。
因此如果能提供应该可见但超出传感器距离获取范围外的数据,对开边界提取是非常有用的。
二、示例解析
2.1 主要使用到的头文件、类及函数
- 头文件
- #include <pcl/range_image/range_image.h>
- #include <pcl/features/range_image_border_extractor.h>
- 类
- RangeImage 深度图像类
- RangeImageBorderExtractor 从深度图像中提取障碍物边界
- 数据结构
- BorderDescription
- PointWithRange
/** rief Data type to store extended information about a transition from foreground to backgroundSpecification of the fields for BorderDescription::traits.
* ingroup common
*/
typedef std::bitset<32> BorderTraits;
/** rief A structure to store if a point in a range image lies on a border between an obstacle and the background.
* ingroup common
*/
struct BorderDescription
{
int x, y;
BorderTraits traits;
//std::vector<const BorderDescription*> neighbors;
friend std::ostream& operator << (std::ostream& os, const BorderDescription& p);
};
struct EIGEN_ALIGN16 _PointWithRange
{
PCL_ADD_POINT4D; // This adds the members x,y,z which can also be accessed using the point (which is float[4])
union
{
struct
{
float range;
};
float data_c[4];
};
EIGEN_MAKE_ALIGNED_OPERATOR_NEW
};
struct PointWithRange : public _PointWithRange
{
inline PointWithRange (const _PointWithRange &p)
{
x = p.x; y = p.y; z = p.z; data[3] = 1.0f;
range = p.range;
}
inline PointWithRange ()
{
x = y = z = 0.0f;
data[3] = 1.0f;
range = 0.0f;
}
friend std::ostream& operator << (std::ostream& os, const PointWithRange& p);
};
- RangeImage 函数
- createFromPointCloud 从点云数据创建深度图像
- integrateFarRanges 把超出传感器范围的点并入到深度图像中
- setUnseenToMaxRange 所有不能观察到的点都为远距离的点
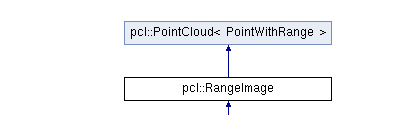
类 pcl::RangeImage 继承关系图:
RangeImageBorderExtractor
: compute 提取边界
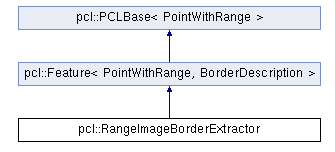
类 pcl::RangeImageBorderExtractor 继承关系图
2.2 示例解析
std::string far_ranges_filename = pcl::getFilenameWithoutExtension (filename)+"_far_ranges.pcd";
if (pcl::io::loadPCDFile(far_ranges_filename.c_str(), far_ranges) == -1)
std::cout << "Far ranges file ""<<far_ranges_filename<<"" does not exists.
";
以上代码读取输入的点去数据文件的同时,也搜索是否有后缀为_far_ranges.pcd
的文件作为超出传感器距离范围外的点数据。
// -----------------------------------------------
// -----从点云创建深度图像-----
// -----------------------------------------------
float noise_level = 0.0;
float min_range = 0.0f;
int border_size = 1;
boost::shared_ptr<pcl::RangeImage> range_image_ptr (new pcl::RangeImage);
pcl::RangeImage& range_image = *range_image_ptr;
range_image.createFromPointCloud (point_cloud, angular_resolution, pcl::deg2rad (360.0f), pcl::deg2rad (180.0f), scene_sensor_pose, coordinate_frame, noise_level, min_range, border_size);
range_image.integrateFarRanges (far_ranges); //并入到点云数据当中
此部分从点云数据中创建深度图像,并把远距离的点云并入进来。
if (setUnseenToMaxRange) //如果没有远距离的点云,则设置不能观察到的点都为远距离的点。
range_image.setUnseenToMaxRange ();
如果没有远距离的点云,则设置不能观察到的点都为远距离的点。
// -------------------------
// -----提取边界-----
// -------------------------
pcl::RangeImageBorderExtractor border_extractor (&range_image);
pcl::PointCloud<pcl::BorderDescription> border_descriptions;
border_extractor.compute (border_descriptions);
提取深度图像的边界信息,存储在border_descriptions
中
//-------------------------------------
// -----在深度图像中显示点集-----
// ------------------------------------
pcl::visualization::RangeImageVisualizer* range_image_borders_widget = NULL;
range_image_borders_widget =
pcl::visualization::RangeImageVisualizer::getRangeImageBordersWidget (range_image, -std::numeric_limits<float>::infinity (), std::numeric_limits<float>::infinity (), false, border_descriptions, "Range image with borders" );
2.3实验结果
实验的数据,没有提供超出传感器范围的数据,所以边界提取的效果并不是非常理想。
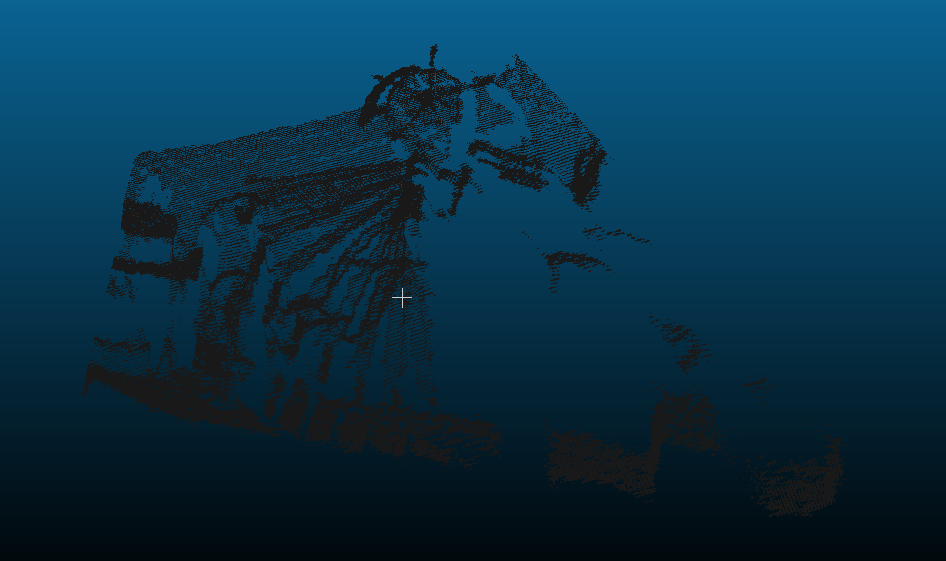
未边界提取
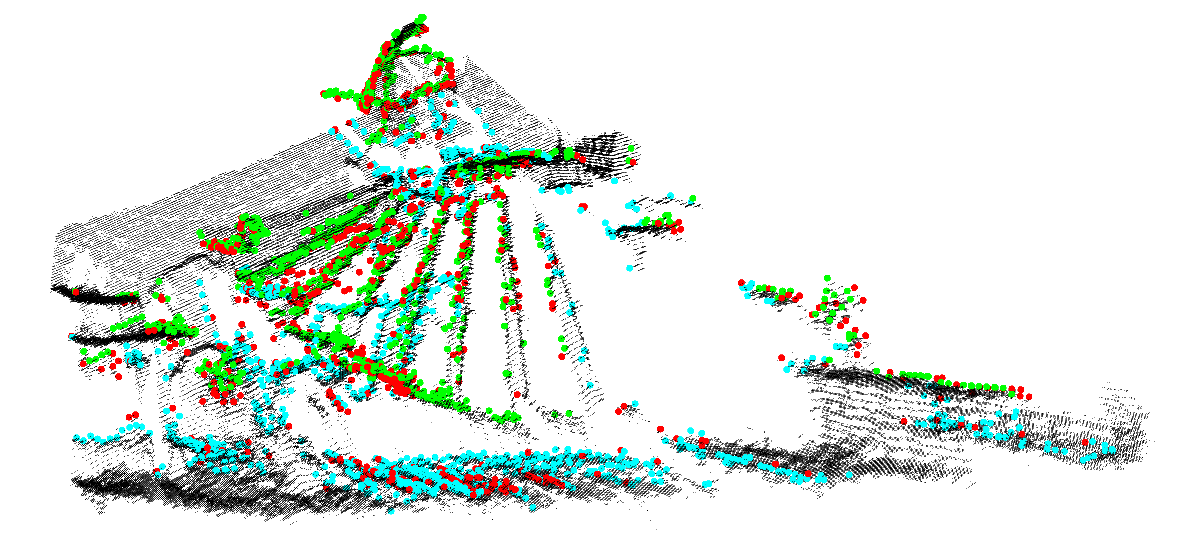
实验结果
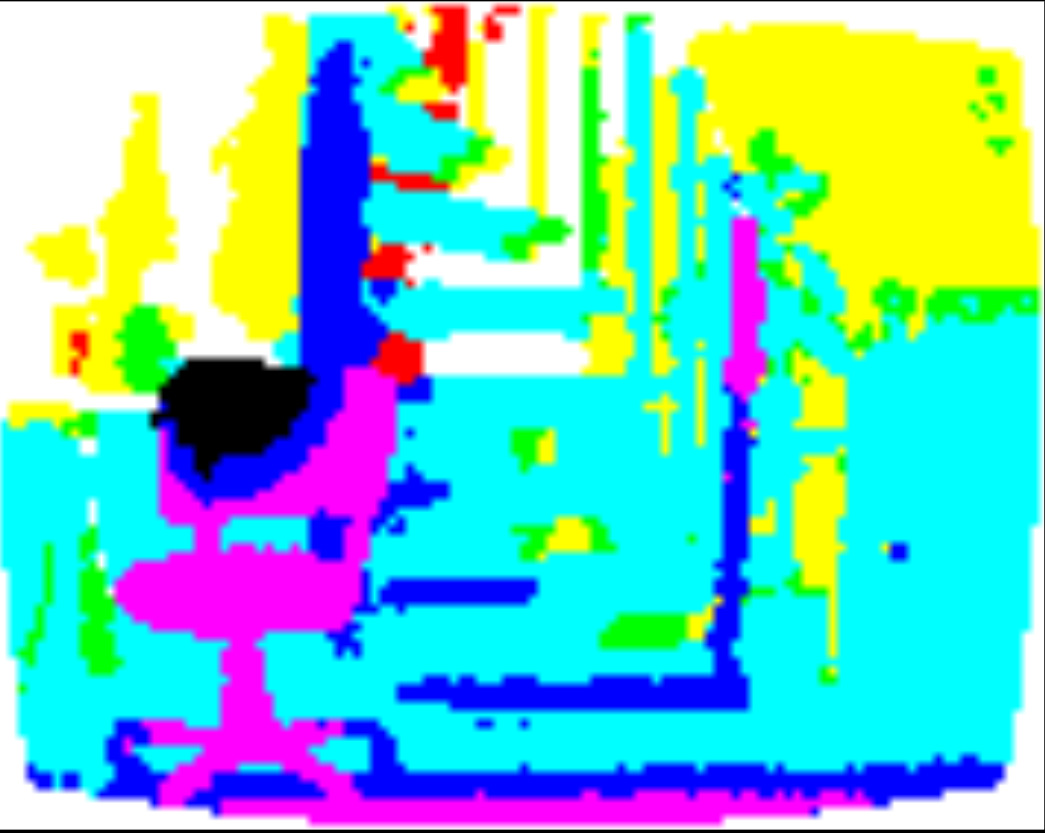
点的深度图像
2.4 完整的代码
#include <iostream>
#include <boost/thread/thread.hpp>
#include <pcl/range_image/range_image.h>
#include <pcl/io/pcd_io.h>
#include <pcl/visualization/range_image_visualizer.h>
#include <pcl/visualization/pcl_visualizer.h>
#include <pcl/features/range_image_border_extractor.h>
#include <pcl/console/parse.h>
typedef pcl::PointXYZ PointType;
// --------------------
// -----参数-----
// --------------------
float angular_resolution = 0.5f;
pcl::RangeImage::CoordinateFrame coordinate_frame = pcl::RangeImage::CAMERA_FRAME;
bool setUnseenToMaxRange = false;
// --------------
// -----帮助-----
// --------------
void
printUsage (const char* progName)
{
std::cout << "
Usage: "<<progName<<" [options] <scene.pcd>
"
<< "Options:
"
<< "-------------------------------------------
"
<< "-r <float> angular resolution in degrees (default "<<angular_resolution<<")
"
<< "-c <int> coordinate frame (default "<< (int)coordinate_frame<<")
"
<< "-m Treat all unseen points to max range
"
<< "-h this help
"
<< "
";
}
// --------------
// -----主函数-----
// --------------
int
main (int argc, char** argv)
{
// --------------------------------------
// -----解析命令行参数-----
// --------------------------------------
if (pcl::console::find_argument (argc, argv, "-h") >= 0)
{
printUsage (argv[0]);
return 0;
}
if (pcl::console::find_argument (argc, argv, "-m") >= 0)
{
setUnseenToMaxRange = true;
cout << "Setting unseen values in range image to maximum range readings.
";
}
int tmp_coordinate_frame;
if (pcl::console::parse (argc, argv, "-c", tmp_coordinate_frame) >= 0)
{
coordinate_frame = pcl::RangeImage::CoordinateFrame (tmp_coordinate_frame);
cout << "Using coordinate frame "<< (int)coordinate_frame<<".
";
}
if (pcl::console::parse (argc, argv, "-r", angular_resolution) >= 0)
cout << "Setting angular resolution to "<<angular_resolution<<"deg.
";
angular_resolution = pcl::deg2rad (angular_resolution);
// ------------------------------------------------------------------
// -----读取pcd文件,如果没有给出pcd文件则创建一个示例点云-----
// ------------------------------------------------------------------
pcl::PointCloud<PointType>::Ptr point_cloud_ptr (new pcl::PointCloud<PointType>);
pcl::PointCloud<PointType>& point_cloud = *point_cloud_ptr;
pcl::PointCloud<pcl::PointWithViewpoint> far_ranges;
Eigen::Affine3f scene_sensor_pose (Eigen::Affine3f::Identity ());
std::vector<int> pcd_filename_indices = pcl::console::parse_file_extension_argument (argc, argv, "pcd");
if (!pcd_filename_indices.empty ())
{
std::string filename = argv[pcd_filename_indices[0]];
if (pcl::io::loadPCDFile (filename, point_cloud) == -1)
{
cout << "Was not able to open file ""<<filename<<"".
";
printUsage (argv[0]);
return 0;
}
scene_sensor_pose = Eigen::Affine3f (Eigen::Translation3f (point_cloud.sensor_origin_[0], point_cloud.sensor_origin_[1], point_cloud.sensor_origin_[2])) *Eigen::Affine3f (point_cloud.sensor_orientation_);
std::string far_ranges_filename = pcl::getFilenameWithoutExtension (filename)+"_far_ranges.pcd";
if (pcl::io::loadPCDFile(far_ranges_filename.c_str(), far_ranges) == -1)
std::cout << "Far ranges file ""<<far_ranges_filename<<"" does not exists.
";
}
else
{
cout << "
No *.pcd file given => Genarating example point cloud.
";
for (float x=-0.5f; x<=0.5f; x+=0.01f)
{
for (float y=-0.5f; y<=0.5f; y+=0.01f)
{
PointType point; point.x = x; point.y = y; point.z = 2.0f - y;
point_cloud.points.push_back (point);
}
}
point_cloud.width = (int) point_cloud.points.size (); point_cloud.height = 1;
}
// -----------------------------------------------
// -----从点云创建深度图像-----
// -----------------------------------------------
float noise_level = 0.0;
float min_range = 0.0f;
int border_size = 1;
boost::shared_ptr<pcl::RangeImage> range_image_ptr (new pcl::RangeImage);
pcl::RangeImage& range_image = *range_image_ptr;
range_image.createFromPointCloud (point_cloud, angular_resolution, pcl::deg2rad (360.0f), pcl::deg2rad (180.0f), scene_sensor_pose, coordinate_frame, noise_level, min_range, border_size);
range_image.integrateFarRanges (far_ranges);
if (setUnseenToMaxRange)
range_image.setUnseenToMaxRange ();
// --------------------------------------------
// -----打开三维浏览器并添加点云-----
// --------------------------------------------
pcl::visualization::PCLVisualizer viewer ("3D Viewer");
viewer.setBackgroundColor (1, 1, 1);
viewer.addCoordinateSystem (1.0f);
pcl::visualization::PointCloudColorHandlerCustom<PointType> point_cloud_color_handler (point_cloud_ptr, 0, 0, 0);
viewer.addPointCloud (point_cloud_ptr, point_cloud_color_handler, "original point cloud");
//PointCloudColorHandlerCustom<pcl::PointWithRange> range_image_color_handler (range_image_ptr, 150, 150, 150);
//viewer.addPointCloud (range_image_ptr, range_image_color_handler, "range image");
//viewer.setPointCloudRenderingProperties (PCL_VISUALIZER_POINT_SIZE, 2, "range image");
// -------------------------
// -----提取边界-----
// -------------------------
pcl::RangeImageBorderExtractor border_extractor (&range_image);
pcl::PointCloud<pcl::BorderDescription> border_descriptions;
border_extractor.compute (border_descriptions);
// ----------------------------------
// -----在三维浏览器中显示点集-----
// ----------------------------------
pcl::PointCloud<pcl::PointWithRange>::Ptr border_points_ptr(new pcl::PointCloud<pcl::PointWithRange>), veil_points_ptr(new pcl::PointCloud<pcl::PointWithRange>), shadow_points_ptr(new pcl::PointCloud<pcl::PointWithRange>);
pcl::PointCloud<pcl::PointWithRange>& border_points = *border_points_ptr, & veil_points = * veil_points_ptr, & shadow_points = *shadow_points_ptr;
for (int y=0; y< (int)range_image.height; ++y)
{
for (int x=0; x< (int)range_image.width; ++x)
{
if (border_descriptions.points[y*range_image.width + x].traits[pcl::BORDER_TRAIT__OBSTACLE_BORDER])
border_points.points.push_back (range_image.points[y*range_image.width + x]);
if (border_descriptions.points[y*range_image.width + x].traits[pcl::BORDER_TRAIT__VEIL_POINT])
veil_points.points.push_back (range_image.points[y*range_image.width + x]);
if (border_descriptions.points[y*range_image.width + x].traits[pcl::BORDER_TRAIT__SHADOW_BORDER])
shadow_points.points.push_back (range_image.points[y*range_image.width + x]);
}
}
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointWithRange> border_points_color_handler (border_points_ptr, 0, 255, 0);
viewer.addPointCloud<pcl::PointWithRange> (border_points_ptr, border_points_color_handler, "border points");
viewer.setPointCloudRenderingProperties (pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 7, "border points");
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointWithRange> veil_points_color_handler (veil_points_ptr, 255, 0, 0);
viewer.addPointCloud<pcl::PointWithRange> (veil_points_ptr, veil_points_color_handler, "veil points");
viewer.setPointCloudRenderingProperties (pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 7, "veil points");
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointWithRange> shadow_points_color_handler (shadow_points_ptr, 0, 255, 255);
viewer.addPointCloud<pcl::PointWithRange> (shadow_points_ptr, shadow_points_color_handler, "shadow points");
viewer.setPointCloudRenderingProperties (pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 7, "shadow points");
//-------------------------------------
// -----在深度图像中显示点集-----
// ------------------------------------
pcl::visualization::RangeImageVisualizer* range_image_borders_widget = NULL;
range_image_borders_widget =
pcl::visualization::RangeImageVisualizer::getRangeImageBordersWidget (range_image, -std::numeric_limits<float>::infinity (), std::numeric_limits<float>::infinity (), false, border_descriptions, "Range image with borders" );
// -------------------------------------
//--------------------
// -----主循环-----
//--------------------
while (!viewer.wasStopped ())
{
range_image_borders_widget->spinOnce ();
viewer.spinOnce ();
pcl_sleep(0.01);
}
}