参考
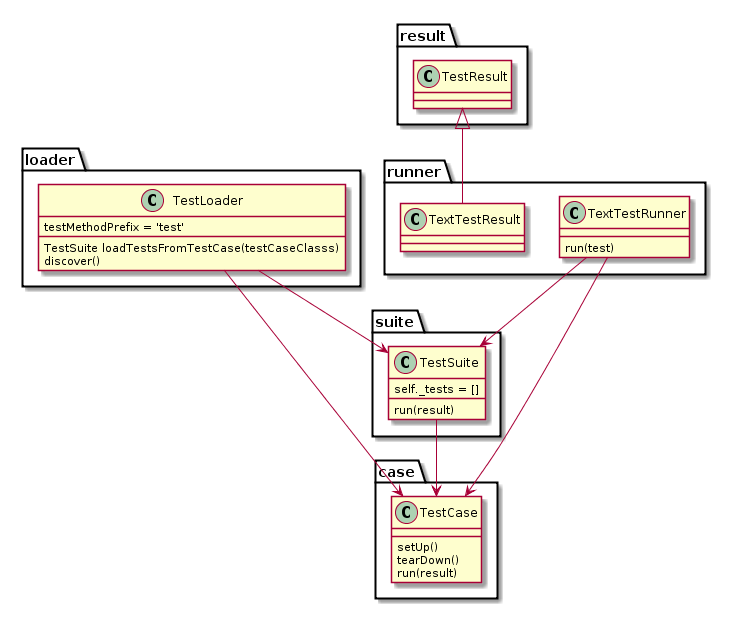
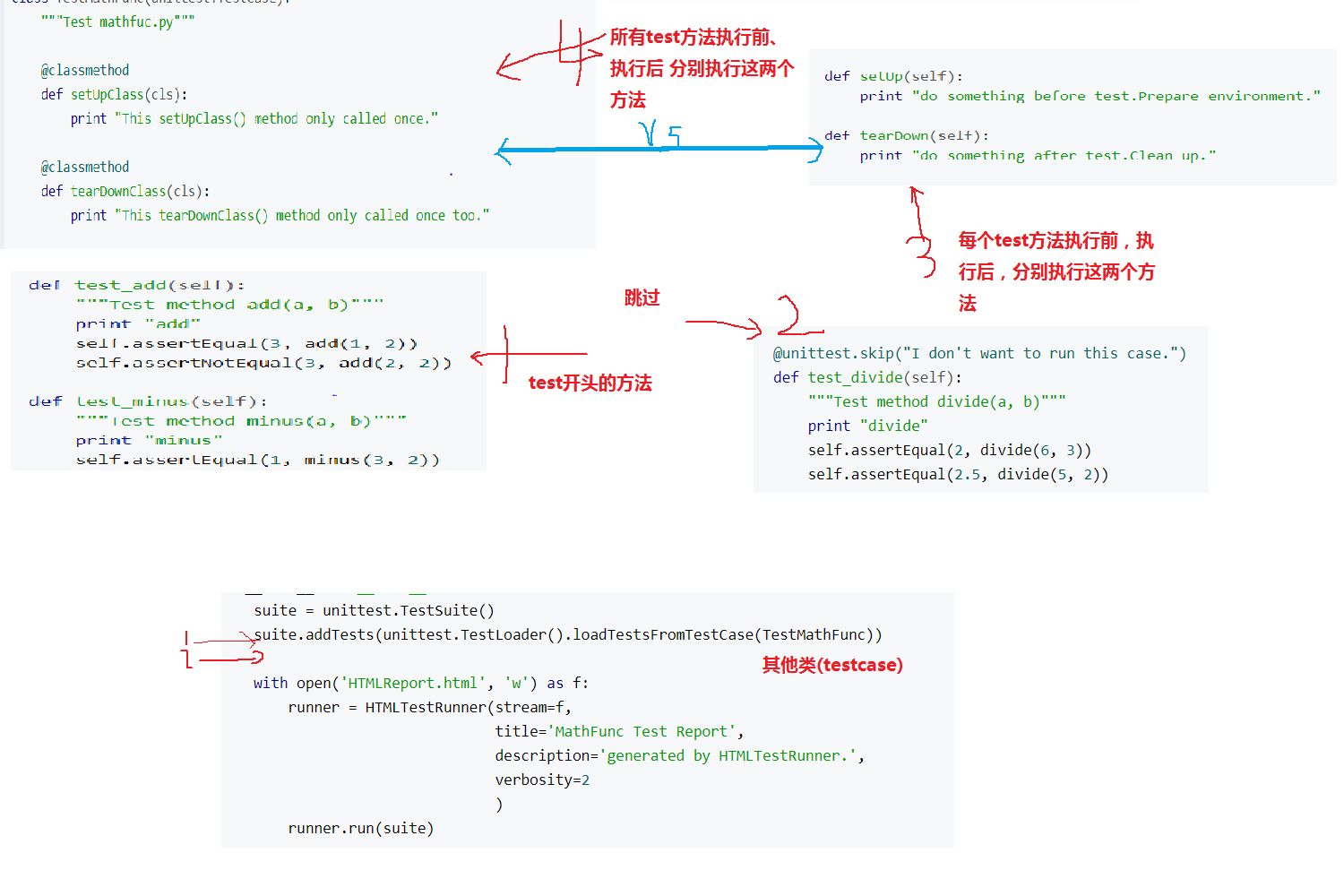
1 # -*- coding: utf-8 -*-
2 """
3 测试代码组织,整理( 非可执行)
4
5 """
6
7 import unittest
8 from test_mathfunc import TestMathFunc
9 from HTMLTestRunner import HTMLTestRunner
10
11
12 ### 1
13
14 def add(a, b):
15 return a+b
16
17 def minus(a, b):
18 return a-b
19
20 def multi(a, b):
21 return a*b
22
23 def divide(a, b):
24 return a/b
25
26
27 ### 2
28 class TestMathFunc(unittest.TestCase):
29 """Test mathfuc.py"""
30
31 ## 1
32
33 @classmethod
34 def setUpClass(cls):
35 print "This setUpClass() method only called once."
36
37 @classmethod
38 def tearDownClass(cls):
39 print "This tearDownClass() method only called once too."
40
41
42 ## 2
43 def setUp(self):
44 print "do something before test.Prepare environment."
45
46 def tearDown(self):
47 print "do something after test.Clean up."
48
49
50
51 ## 3
52 def test_add(self):
53 """Test method add(a, b)"""
54 print "add"
55 self.assertEqual(3, add(1, 2))
56 self.assertNotEqual(3, add(2, 2))
57
58 def test_minus(self):
59 """Test method minus(a, b)"""
60 print "minus"
61 self.assertEqual(1, minus(3, 2))
62
63 def test_multi(self):
64 """Test method multi(a, b)"""
65 print "multi"
66 self.assertEqual(6, multi(2, 3))
67
68 @unittest.skip("I don't want to run this case.")
69 def test_divide(self):
70 """Test method divide(a, b)"""
71 print "divide"
72 self.assertEqual(2, divide(6, 3))
73 self.assertEqual(2.5, divide(5, 2))
74
75
76
77 if __name__ == '__main__':
78 suite = unittest.TestSuite()
79 suite.addTests(unittest.TestLoader().loadTestsFromTestCase(TestMathFunc))
80 # suite.addTests(unittest.TestLoader().loadTestsFromTestCase(TestMathFunc2))
81 # suite.addTests(unittest.TestLoader().loadTestsFromTestCase(TestMathFunc3))
82
83 # tests = [TestMathFunc("test_add"), TestMathFunc("test_minus"), TestMathFunc("test_divide")]
84 # suite.addTests(tests)
85
86 with open('HTMLReport.html', 'w') as f:
87 # runner = unittest.TextTestRunner(stream=f, verbosity=2)
88 runner = HTMLTestRunner(stream=f,
89 title='MathFunc Test Report',
90 description='generated by HTMLTestRunner.',
91 verbosity=2
92 )
93 runner.run(suite)
94