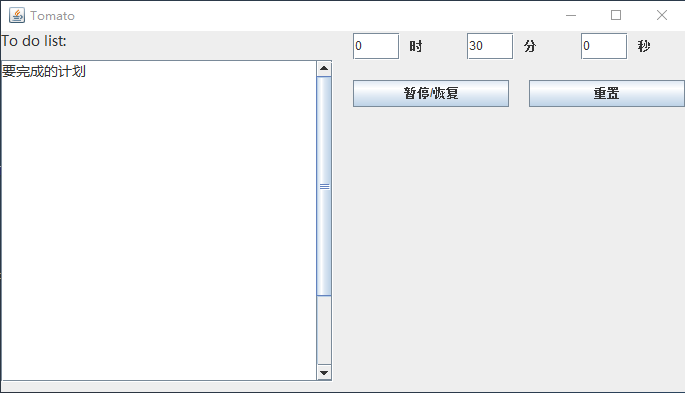

一个可执行文件,产生的一个计划事件txt,可手动编辑,每次打开软件会读取,每次关闭软件会保存。
贴上源码,由于比较简单,没有分层。
package pers.yuanzi.tomoto;
import java.awt.GridLayout;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Scanner;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
//主函数类,分别调用左右两视图
public class UI extends JFrame {
Text panel1 = new Text();
Time panel2 = new Time();
File file = new File("toDoList.txt");
public UI() {
try
{ //每次打开是读取文件,没有则创建
file.createNewFile();
Scanner input = new Scanner(file);
StringBuffer buffer = new StringBuffer();
while (input.hasNextLine()) {
buffer.append(input.nextLine());
}
panel1.text.setText(buffer.toString());
input.close();
}catch(Exception e)
{
e.printStackTrace();
throw new RuntimeException(e);
}
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
//关闭时保存计划
String str = new String(panel1.text.getText());
try {
PrintWriter output = new PrintWriter(file);
output.print(str);
output.flush();
output.close();
} catch (FileNotFoundException e1) {
e1.printStackTrace();
throw new RuntimeException(e1);
}
//退出时提醒
if (JOptionPane.showConfirmDialog(null, "退出", "提示", JOptionPane.YES_NO_OPTION,
JOptionPane.QUESTION_MESSAGE) == JOptionPane.YES_OPTION) {
System.exit(0);
}
}
});
setLayout(new GridLayout(1, 2, 20, 20));
setTitle("Tomato");
setSize(700, 400);
setDefaultCloseOperation(DO_NOTHING_ON_CLOSE);
setVisible(true);
add(panel1);
add(panel2);
}
public static void main(String[] args) {
UI ui =new UI();
}
}
package pers.yuanzi.tomoto;
import java.awt.BorderLayout;
import java.awt.Font;
import java.awt.GridLayout;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
//左边计划视图
public class Text extends JPanel{
JTextArea text = new JTextArea();
JLabel label = new JLabel("To do list:");
JLabel empty = new JLabel("");
int i = 0;
public Text() {
setLayout(new BorderLayout(10,10));
label.setFont(new Font("微软雅黑", Font.PLAIN, 14));
empty.setFont(new Font("微软雅黑", Font.PLAIN, 14));
text.setColumns(22);
text.setRows(22);
text.setLineWrap(true);
text.setWrapStyleWord(true);
text.setFont(new Font("微软雅黑", Font.PLAIN, 14));
JScrollPane scrollPanel = new JScrollPane(text);
add(label,BorderLayout.NORTH);
add(scrollPanel,BorderLayout.CENTER);
add(empty,BorderLayout.SOUTH);
}
}
package pers.yuanzi.tomoto;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Timer;
import java.util.TimerTask;
import javax.sound.sampled.AudioInputStream;
import javax.sound.sampled.AudioSystem;
import javax.sound.sampled.Clip;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class Time extends JPanel {
JLabel label1 = new JLabel("时");
JLabel label2 = new JLabel("分");
JLabel label3 = new JLabel("秒");
JTextField text1 = new JTextField("0", 3);
JTextField text2 = new JTextField("30", 3);
JTextField text3 = new JTextField("0", 3);
JButton button2 = new JButton("暂停/恢复");
JButton button3 = new JButton("重置");
int hours = 0, minutes = 0, seconds = 0;
int stop = 1;
Timer timer = new Timer();
TimerTask timerTask = null;
public Time() {
//重置按钮默认30秒
button3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
if (timerTask != null) {
stop = 1;
timerTask.cancel();
}
text1.setText("0");
text2.setText("30");
text3.setText("0");
}
});
//开始暂停按钮
button2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
checkTime();
hours = Integer.parseInt(text1.getText());
minutes = Integer.parseInt(text2.getText());
seconds = Integer.parseInt(text3.getText());
if (1 == stop) {
stop = 0;
} else {
stop = 1;
}
//倒计时核心,创建一个倒计时更新视图
timer.schedule(timerTask = new TimerTask() {
@Override
public void run() {
if (1 == stop) {
text1.setText(hours + "");
text2.setText(minutes + "");
text3.setText(seconds + "");
this.cancel();
} else {
if (0 != seconds) {
seconds -= 1;
text3.setText(seconds + "");
} else if (0 != minutes) {
minutes -= 1;
text2.setText(minutes + "");
seconds = 59;
text3.setText(seconds + "");
} else if (0 != hours) {
hours -= 1;
text1.setText(hours + "");
minutes = 59;
text2.setText(minutes + "");
seconds = 59;
text3.setText(seconds + "");
} else {
this.cancel();
playSound();
JOptionPane.showMessageDialog(null, "时间到了!", "提示", JOptionPane.INFORMATION_MESSAGE);
stop = 1;
}
}
}
}, 0, 1000);
}
});
JPanel jPanel1 = new JPanel();
jPanel1.setLayout(new GridLayout(1, 6, 10, 10));
jPanel1.add(text1);
jPanel1.add(label1);
jPanel1.add(text2);
jPanel1.add(label2);
jPanel1.add(text3);
jPanel1.add(label3);
JPanel jPanel2 = new JPanel();
jPanel2.setLayout(new GridLayout(1, 2, 20, 20));
jPanel2.add(button2);
jPanel2.add(button3);
setLayout(new GridLayout(8, 1, 10, 20));
add(jPanel1);
add(jPanel2);
}
//检查时间是否正确输入
private void checkTime() {
if (!isDigit(text1.getText()) || !isDigit(text2.getText()) || !isDigit(text3.getText())
|| Integer.parseInt(text2.getText()) < 0 || Integer.parseInt(text2.getText()) > 59
|| Integer.parseInt(text2.getText()) < 0 || Integer.parseInt(text3.getText()) > 59
|| Integer.parseInt(text3.getText()) < 0) {
JOptionPane.showMessageDialog(null, "请输入合法范围的时间!", "提示", JOptionPane.INFORMATION_MESSAGE);
button3.doClick();
}
}
private boolean isDigit(String str) {
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i)))
return false;
}
return true;
}
//警告时的声音方法
private void playSound() {
/*AudioClip audioClip=getAudioClip(getCodeBase(),"directory of audio");
audioClip.play();*/
/*AudioClip au = null;
try {
au = Applet.newAudioClip(new File("近藤浩治 - Nintendo Mark.mid").toURL());
au.play();
} catch (MalformedURLException e) {
e.printStackTrace();
throw new RuntimeException(e);
}*/
try {
Clip clip = AudioSystem.getClip();
AudioInputStream inputStream = AudioSystem
.getAudioInputStream(Time.class.getResourceAsStream("近藤浩治 - Nintendo Mark.mid"));
clip.open(inputStream);
clip.start();
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}