1 GreenDao 简介
GreenDao是一款操作SQLite数据库的神器,是一款ORM数据库工具。
ORM(Object Relationship Mapping)对象关系映射,将对数据库的操作通过GreenDao和Bean对象关联起来,通过Dao操作Bean对象,实现对数据库的增删改查。类似于JavaEE中Hibernate的作用。
相似的工具还有OrmLite。其表现形式如图:
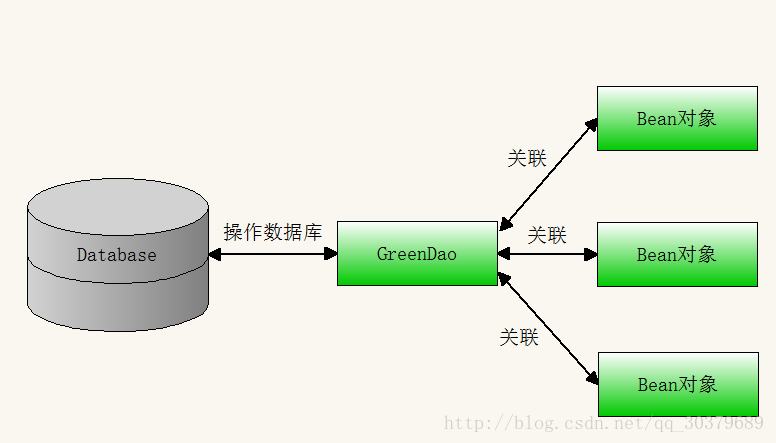
它的优点:
- 存取数据库快
- 轻量级
- 支持数据库加密
- 支持缓存
- 代码自动生成
2 GreenDao3.2 配置
2.1在工程(Project)的build.gradle中添加依赖
dependencies { classpath 'com.android.tools.build:gradle:2.2.3' //GreenDao3依赖 classpath 'org.greenrobot:greendao-gradle-plugin:3.2.1' }
2.2在项目(Module)的build.gradle中添加依赖
apply plugin: 'org.greenrobot.greendao' compile 'org.greenrobot:greendao:3.2.0'//greendao依赖
2.3配置自动生成的代码路径(DaoMaster DaoSession)
在项目(Module)的build.gradle中,android节点下新增
greendao { //指定数据库schema版本号,迁移等操作会用到 schemaVersion 1 //DaoSession、DaoMaster以及所有实体类的dao生成的目录,默认为entity所在的包名 daoPackage 'com.zc.myapp.greendao' //工程路径 targetGenDir 'src/main/java' }
这样,DaoMaster,DaoSession生成在项目中
3 GreenDao3.2 使用
3.1 创建Bean对象(表名和字段名)
//告诉GreenDao该对象为实体,只有被@Entity注释的Bean类才能被dao类操作 @Entity public class Goods { //主键 自增 不能用int @Id(autoincrement = true) private Long id; //属性值唯一 @Unique private String name;//商品名称 //普通属性 private String price;//价格 private int sell_num;//已售数量 private String image_url;//商品图片地址 //不能为空 @NotNull private String address;//商家地址 @Generated(hash = 149856672) public Goods(Long id, String name, String price, int sell_num, String image_url, @NotNull String address) { this.id = id; this.name = name; this.price = price; this.sell_num = sell_num; this.image_url = image_url; this.address = address; } @Generated(hash = 1770709345) public Goods() { } }
主键注意要使用Long类型而不是long类型。
3.2 创建数据库(数据库名)
自定义MyApplication,在onCreat方法,初始化数据库
private static DaoSession daoSession; /** * 配置数据库 */ private void setupDatabase() { //创建数据库zc.db DevOpenHelper:创建SQLite数据库的SQLiteOpenHelper具体实现。 DaoMaster.DevOpenHelper helper = new DaoMaster.DevOpenHelper(this,"zc.db",null); //获取可写数据库 SQLiteDatabase db = helper.getWritableDatabase(); //获取数据库对象 用于创建、删除表 DaoMaster daoMaster = new DaoMaster(db); //获取Dao对象管理者 管理所有的Dao,Dao对象中存在增删改查api daoSession = daoMaster.newSession(); } public static DaoSession getDaoInstance(){ return daoSession; }
3.3 数据库的增删改查
/** * 实现Goods表的增删改查 * Created by zhangc on 2018/7/13. */ public class GoodsDaoUtil { /** * 添加数据 * * @param goods */ public static void insertGoods(Goods goods) { MyApplication.getDaoInstance().getGoodsDao().insert(goods); } /** * 删除数据 * * @param id */ public static void deleteGoods(long id) { MyApplication.getDaoInstance().getGoodsDao().deleteByKey(id); } /** * 更新 * * @param goods */ public static void updateGoods(Goods goods) { MyApplication.getDaoInstance().getGoodsDao().update(goods); } /** * 查询全部数据 * * @return */ public static List<Goods> queryAll() { return MyApplication.getDaoInstance().getGoodsDao().loadAll(); } }
其他查询api在实际开发中具体应用。