作者:ssslinppp
2. tree的相关介绍
3. 异步加载tree数据,并实现tree的折叠展开
3.1 功能说明:
3.2 前台代码
3.3 后台代码
4. 其他
1. 摘要
easyui相关的介绍可以上其官网或者百度去搜索,这里不做介绍。
Easyui Tree的使用,官网或者easyui中文网站,也有相关介绍,但是官方提供的实例所使用的json是写死的,不是后台实时读取的。在实际的项目中,要显示的tree数据,一般都是从数据库中读取,然后通过通过ajax或者其他技术将tree的json数据发送到前台,然后显示。
本文将介绍easyui tree的异步加载,以及手动展开tree。
2. tree的相关介绍

上图是一个tree,它对应json数据格式为:
[{
"id":1,
"text":"My Documents",
"children":[{
"id":11,
"text":"Photos",
"state":"closed",
"children":[{
"id":111,
"text":"Friend"
},{
"id":112,
"text":"Wife"
},{
"id":113,
"text":"Company"
}]
},{
"id":12,
"text":"Program Files",
"children":[{
"id":121,
"text":"Intel"
},{
"id":122,
"text":"Java",
"attributes":{
"p1":"Custom Attribute1",
"p2":"Custom Attribute2"
}
},{
"id":123,
"text":"Microsoft Office"
},{
"id":124,
"text":"Games",
"checked":true
}]
},{
"id":13,
"text":"index.html"
},{
"id":14,
"text":"about.html"
},{
"id":15,
"text":"welcome.html"
}]
}]
从上面的json数据可以看出,tree的数据有固定格式,一般都包括下面几个:
- id: 唯一标示;
- text: 显示的文本;
- children:子节点;
- state:closed或open,表示节点是展开还是折叠;
- attributes:属性,这里可以自定义若干属性;
等,还有其他一些属性,这里没有一一列举。
如果tree的内容不变,可以采用静态的方式显示,这个在官网上有实例,不再详述。
若果想通过异步的方式加载tree的json数据,则后台只需按照tree的数据格式生成相应的json,然后返回前台即可。下面将介绍异步加载tree数据。
3. 异步加载tree数据,并实现tree的折叠展开
3.1 功能说明:

上图是整个tree的节点信息,这些数据都是从数据库中读取并显示的。但是在实际的项目中,可能tree的节点(children)会很多,若是一次全部加载,可能会很耗时,通常我们都是先加载父节点信息,然后点击“展开”,再加载子节点信息,如下图所示:
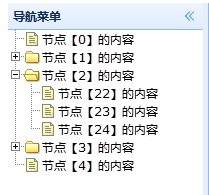
上图中,我们首次加载时,【节点1】和【节点3】的子节点没有展开(加载),而【节点2】的子节点全部展开,当点击【节点1】的展开按钮时,再加载【节点1】的子节点,如下图所示:

3.2 前台代码
jsp界面:

<%@ page language="java" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
response.setHeader("Pragma", "no-cache");
response.setHeader("Cache-Control", "no-cache");
response.setDateHeader("Expires", 0);
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html>
<head>
<title>测试系统</title>
<script type="text/javascript">var basePath = "<%=basePath%>";</script>
<link rel="stylesheet" type="text/css" href="<%=basePath%>js/easyui/themes/default/easyui.css">
<link rel="stylesheet" type="text/css" href="<%=basePath%>js/easyui/themes/icon.css">
<link rel="stylesheet" type="text/css" href="<%=basePath%>js/easyui/demo.css">
<script type="text/javascript" src="<%=basePath%>js/easyui/jquery.min.js"></script>
<script type="text/javascript" src="<%=basePath%>js/easyui/jquery.easyui.min.js"></script>
<script type="text/javascript" src="<%=basePath%>js/mytreeTest.js"></script>
</head>
<body>
<h2>easyui tree</h2>
<div class="easyui-layout" style="width:1300px;height:550px;">
<div data-options="region:'west',split:true,border:false" title="导航菜单" style="width:200px">
<ul id="myTree" class="easyui-tree"></ul>
</div>
<div data-options="region:'center'">
</div>
</div>
</body>
</html>
mytreeTest.js

$(function() {
$('#myTree').tree({
// checkbox: true,
animate : true,
lines : true,
url : basePath + "loadTreeJson.action", //默认会将节点的id传递到后台
loadFilter : function(data) { //必须有这个函数,否则出不来,不知道为什么
return data.treeJson;
},
onClick : function(node) {
alert("自己添加的属性: 【URL】"+node.attributes.url+", 【info】"+node.attributes.info);
}
});
});
3.3 后台代码
我们采用了spring mvc。
为了实现tree的json格式数据的返回,我们在后台定义了一个类:TreeNodeInfo.java
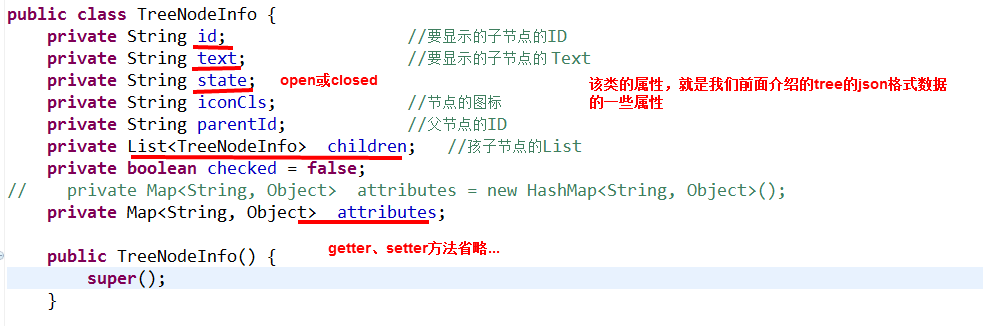
package com.ll.domain;
import java.util.List;
import java.util.Map;
public class TreeNodeInfo {
private String id; //要显示的子节点的ID
private String text; //要显示的子节点的 Text
private String state;
private String iconCls; //节点的图标
private String parentId; //父节点的ID
private List<TreeNodeInfo> children; //孩子节点的List
private boolean checked = false;
// private Map<String, Object> attributes = new HashMap<String, Object>();
private Map<String, Object> attributes;
public TreeNodeInfo() {
super();
}
public TreeNodeInfo(String id, String text, String state, String iconCls,
String parentId, List<TreeNodeInfo> children, boolean checked,
Map<String, Object> attributes) {
super();
this.id = id;
this.text = text;
this.state = state;
this.iconCls = iconCls;
this.parentId = parentId;
this.children = children;
this.checked = checked;
this.attributes = attributes;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getIconCls() {
return iconCls;
}
public void setIconCls(String iconCls) {
this.iconCls = iconCls;
}
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public List<TreeNodeInfo> getChildren() {
return children;
}
public void setChildren(List<TreeNodeInfo> children) {
this.children = children;
}
public boolean isChecked() {
return checked;
}
public void setChecked(boolean checked) {
this.checked = checked;
}
public Map<String, Object> getAttributes() {
return attributes;
}
public void setAttributes(Map<String, Object> attributes) {
this.attributes = attributes;
}
}
loadTreeJson.action




package com.ll.web;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import com.ll.domain.TreeNodeInfo;
import com.ll.domain.User;
import com.ll.service.IUserService;
@Controller
public class LoginController {
@Autowired
private IUserService userService;
@RequestMapping(value = "/index.action")
public String loginPage() {
// return "login";
return "myEasyuiTree";
}
@RequestMapping(value = "/loadTreeJson.action")
public String loadTreeJson(ModelMap mm, String id,String info) {
List<TreeNodeInfo> treeList = new ArrayList<TreeNodeInfo>();
if((id==null) || "".equals(id)){ //首次加载tree节点
//模拟从数据库读数据,并将读出的数据赋值给treelist
for (int i = 0; i < 5; i++) {
TreeNodeInfo e = new TreeNodeInfo();
e.setId(i+"");
e.setText("节点【"+i+"】的内容");
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("url", "www.baidu.com");
attributes.put("info", "可以设置许多属性值,这是第"+i+"个节点");
e.setAttributes(attributes);
//模拟子节点的数量-第1个和第3个有子节点,默认closed;
if ((i==1) || (i==3)) {
// 节点状态,'open' 或 'closed',默认是 'open'。
// 当设置为 'closed'时,该节点有子节点,并且将从远程站点加载它们
e.setState("closed");
}
//第2个节点也有子节点,但是默认open
if((i==2)){
List<TreeNodeInfo> node2ChildrenList = new ArrayList<TreeNodeInfo>();
for (int j = 22; j < 25; j++) {
TreeNodeInfo e2 = new TreeNodeInfo();
e2.setId(j + "");
e2.setText("节点【" + j + "】的内容");
Map<String, Object> attributes2 = new HashMap<String, Object>();
attributes2.put("url", "www.baidu.com");
attributes2.put("info", "这是子节点【" + j + "】");
e2.setAttributes(attributes2);
node2ChildrenList.add(e2);
}
e.setChildren(node2ChildrenList);
}
treeList.add(e);
}
}else{ //展开节点
//判断节点的id号
if("1".equals(id)){ //有3个子节点
for (int i = 10; i < 13; i++) {
TreeNodeInfo e = new TreeNodeInfo();
e.setId(i + "");
e.setText("节点【" + i + "】的内容");
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("url", "www.baidu.com");
attributes.put("info", "这是子节点【" + i + "】");
e.setAttributes(attributes);
treeList.add(e);
}
}else if ("3".equals(id)) { //有4个子节点
for (int i = 30; i < 34; i++) {
TreeNodeInfo e = new TreeNodeInfo();
e.setId(i + "");
e.setText("节点【" + i + "】的内容");
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("url", "www.baidu.com");
attributes.put("info", "这是子节点【" + i + "】");
e.setAttributes(attributes);
treeList.add(e);
}
}
}
mm.addAttribute("treeJson", treeList);
return "treeJsonBean";
}
@RequestMapping(value = "/test.action")
public String test(HttpServletRequest request, LoginCommand loginCommand) {
System.out.println("用户名:" + loginCommand.getUserName() + "--密码:"
+ loginCommand.getPassword());
User user = new User();
user.setUserName(loginCommand.getUserName());
user.setPassword(loginCommand.getPassword());
userService.save(user);
request.getSession().setAttribute("user", user);
return "main";
}
}
当首次加载时,tree如下图所示:

当点击展开【节点1】和【节点3】时,如下图所示:

当点击tree节点时,会弹出:

spring mvc配置:


<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd">
<!-- 扫描web包,应用Spring的注解 -->
<context:component-scan base-package="com.ll.web" />
<mvc:annotation-driven />
<!-- 配置视图解析器,将ModelAndView及字符串解析为具体的页面 -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver"
p:viewClass="org.springframework.web.servlet.view.JstlView" p:prefix="/jsp/"
p:suffix=".jsp" />
<!-- bean 视图解析器 -->
<bean class="org.springframework.web.servlet.view.BeanNameViewResolver"
p:order="10" />
<!-- 返回tree-json 状态 -->
<bean id="treeJsonBean"
class="org.springframework.web.servlet.view.json.MappingJacksonJsonView">
<property name="renderedAttributes">
<set>
<value>treeJson</value>
</set>
</property>
</bean>
</beans>