先要把word或ppt转换为pdf; 以pdf的格式展示,防止文件拷贝。
注意:需安装Microsoft.Office.Interop.WordExcelPowerPoint组件。
程序集如下:
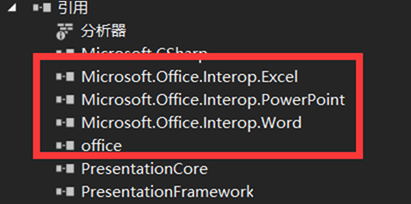
代码:
using Microsoft.Office.Core; using System; using System.IO; using System.Windows.Forms; using Excel = Microsoft.Office.Interop.Excel; using PowerPoint = Microsoft.Office.Interop.PowerPoint; using Word = Microsoft.Office.Interop.Word; namespace WindowsFormsApp4 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { bool isSuccess = DOCConvertToPDF(Directory.GetCurrentDirectory() + "\aa.docx", Directory.GetCurrentDirectory() + "\aa.pdf"); if (isSuccess) { pdfViewer1.LoadFromFile(Directory.GetCurrentDirectory() + "\aa.pdf"); } } /// <summary> /// Word转换成pdf /// </summary> /// <param name="sourcePath">源文件路径</param> /// <param name="targetPath">目标文件路径</param> /// <returns>true=转换成功</returns> public static bool DOCConvertToPDF(string sourcePath, string targetPath) { bool result = false; Word.Application app = new Word.Application(); Word.Document doc = null; object missing = System.Reflection.Missing.Value; object saveChanges = Word.WdSaveOptions.wdDoNotSaveChanges; try { app.Visible = false; doc = app.Documents.Open(sourcePath); doc.ExportAsFixedFormat(targetPath, Word.WdExportFormat.wdExportFormatPDF); result = true; } catch (Exception ex) { result = false; throw new ApplicationException(ex.Message); } finally { if (doc != null) { doc.Close(ref saveChanges, ref missing, ref missing); doc = null; } if (app != null) { app.Quit(ref missing, ref missing, ref missing); app = null; } GC.Collect(); GC.WaitForPendingFinalizers(); } return result; } /// <summary> /// 把Excel文件转换成PDF格式文件 /// </summary> /// <param name="sourcePath">源文件路径</param> /// <param name="targetPath">目标文件路径</param> /// <returns>true=转换成功</returns> public static bool XLSConvertToPDF(string sourcePath, string targetPath) { bool result = false; Excel.XlFixedFormatType targetType = Excel.XlFixedFormatType.xlTypePDF; object missing = Type.Missing; Excel.Application app = null; Excel.Workbook book = null; try { app = new Excel.Application(); object target = targetPath; object type = targetType; book = app.Workbooks.Open(sourcePath, missing, missing, missing, missing, missing, missing, missing, missing, missing, missing, missing, missing, missing, missing); book.ExportAsFixedFormat(targetType, target, Excel.XlFixedFormatQuality.xlQualityStandard, true, false, missing, missing, missing, missing); result = true; } catch (Exception ex) { result = false; throw new ApplicationException(ex.Message); } finally { if (book != null) { book.Close(true, missing, missing); book = null; } if (app != null) { app.Quit(); app = null; } GC.Collect(); GC.WaitForPendingFinalizers(); } return result; } ///<summary> /// 把PowerPoint文件转换成PDF格式文件 ///</summary> ///<param name="sourcePath">源文件路径</param> ///<param name="targetPath">目标文件路径</param> ///<returns>true=转换成功</returns> public static bool PPTConvertToPDF(string sourcePath, string targetPath) { bool result = false; PowerPoint.PpSaveAsFileType targetFileType = PowerPoint.PpSaveAsFileType.ppSaveAsPDF; object missing = Type.Missing; PowerPoint.Application app = null; PowerPoint.Presentation pres = null; try { app = new PowerPoint.Application(); pres = app.Presentations.Open(sourcePath, MsoTriState.msoTrue, MsoTriState.msoFalse, MsoTriState.msoFalse); pres.SaveAs(targetPath, targetFileType, Microsoft.Office.Core.MsoTriState.msoTrue); result = true; } catch (Exception ex) { result = false; throw new ApplicationException(ex.Message); } finally { if (pres != null) { pres.Close(); pres = null; } if (app != null) { app.Quit(); app = null; } GC.Collect(); GC.WaitForPendingFinalizers(); } return result; } } }