父类方法:Car
public class Car {
//属性:车的颜色color、车主姓名userName
private String color;
private String userName;
public Car(){
}
//带参构造函数(参数为color和userName)
public Car(String color, String userName) {
this.color = color;
this.userName = userName;
}
//通过封装实现对私有属性的get/set操作
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
//创建无参无返回值得方法use(描述内容为:我是机动车!)
public void use(){
System.out.println("我是机动车!");
}
//重写equals方法,比较两个对象是否相等(比较color,userName)
public boolean equals(Object obj){
if (obj == null)
return false;
Car temp = (Car)obj;
if (this.getColor().equals(temp.getColor()) && (this.getUserName().equals(temp.getUserName())))
return true;
else
return false;
}
}
子类方法:Taxi
public class Taxi extends Car{
//私有属性:所属公司( company)
private String company;
public Taxi(){
}
//调用父类的构造方法,完成属性赋值
public Taxi(String color, String userName,String company){
super(color, userName);
this.setCompany(company);
}
//通过封装实现对私有属性的get/set操作
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
//创建不允许重写的ride方法,描述为:**出租车是所属于***公司的。
public final void ride(){
System.out.println(this.getUserName()+"的出租车是所属于"+this.getCompany()+"公司的。");
}
//重写父类use方法,描述为:出租车是提高市民生活质量的重要条件之一
@Override
public void use() {
System.out.println("出租车是提高市民生活质量的重要条件之一");
}
//重写toString方法,输出的表现形式不同(输出color,userName)
@Override
public String toString() {
return "taxi的信息是:"+this.getUserName()+"拥有一辆"+this.getColor()+"的出租车";
}
}
子类方法:HomeCar
public final class HomeCar extends Car{
//私有属性:载客数(num)
private int num;
public HomeCar(){
}
//带参构造方法为所有属性赋值
public HomeCar(int num) {
this.num = num;
}
public HomeCar(String color, String userName, int num) {
super(color, userName);
this.num = num;
}
//通过封装实现对私有属性的get/set操作
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
//创建无参无返回值的display方法,表现为:**拥有的**颜色的私家车,有**座位
public void display(){
System.out.println(this.getUserName()+"拥有的"+this.getColor()+"颜色的私家车,有"+this.getNum()+"座位");
}
//重载display方法(带参数num),描述为:家用汽车大多有**个座位
public void display(int num){
System.out.println("家用汽车大多有"+num+"个座位");
}
}
效果图如下:
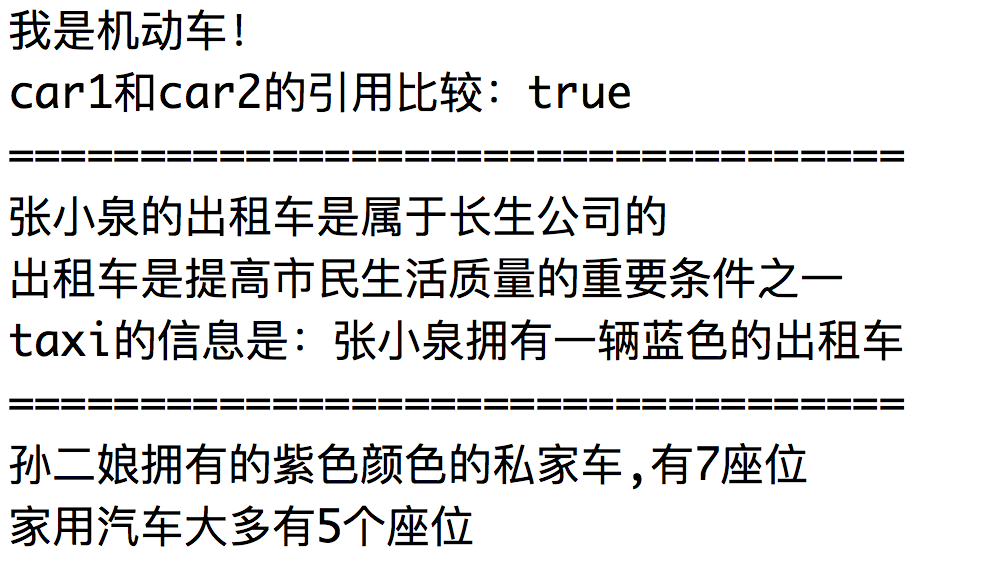