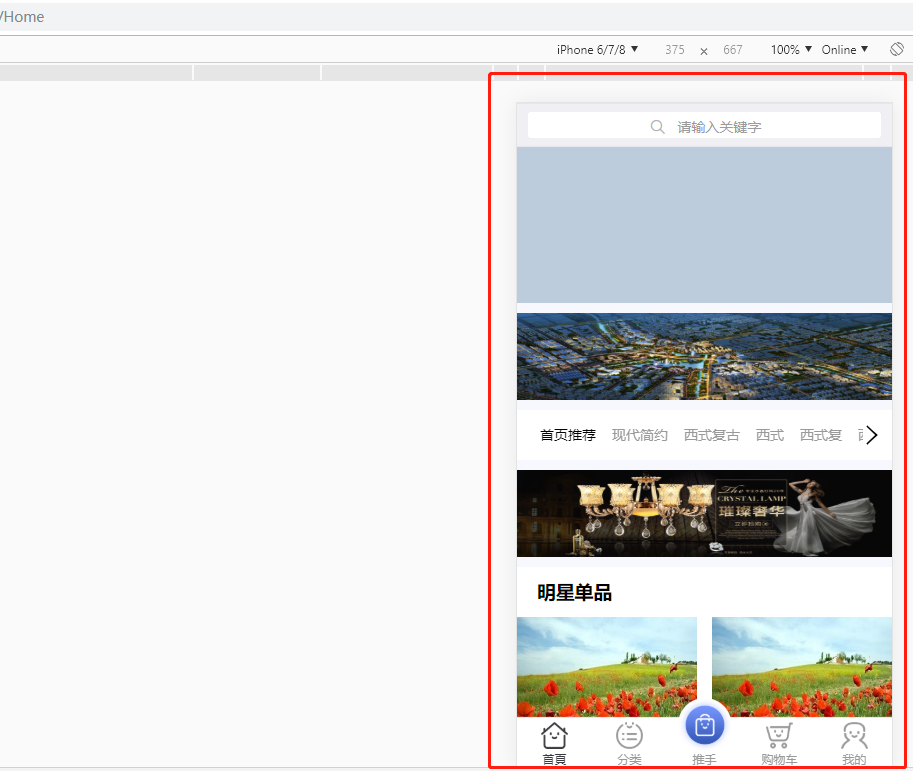
//home.vue
<template>
<div class="home">
<div class="top-info">
<search v-model="searchValue" position="absolute" auto-scroll-to-top top="0px" @on-cancel="onCancel" @on-submit="onSubmit" placeholder="请输入关键字" ref="search"></search>
</div>
<div class="nearby-store">
<img src="../../assets/img-demo1.png"/>
</div>
<div class="screen-list">
<div class="screen-box">
<ul :style="{ screenWidth + 'px'}" ref="screenBox">
<li v-for="item in screenList" :class="{active:item.active}" @click="changeScreen(item)">{{item.name}}</li>
</ul>
</div>
<x-icon class="icon-right" type="ios-arrow-right" size="30"></x-icon>
</div>
<div class="special-banner">
<img src="../../assets/img-demo2.png"/>
</div>
<div class="good-list">
<div class="star-single">
<h3>明星单品</h3>
<ul>
<li v-for="item in goodsList">
<div class="good-img-box">
<img src="./../images/demo01.jpg" height="182" width="273" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
</div>
</li>
</ul>
</div>
<div class="star-single">
<h3>新品速递</h3>
<ul>
<li v-for="item in goodsList">
<div class="good-img-box">
<img src="./../images/demo01.jpg" height="182" width="273" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
</div>
</li>
</ul>
</div>
<div class="star-single">
<h3>特惠闪购</h3>
<ul>
<li v-for="item in goodsList">
<div class="good-img-box">
<img src="./../images/demo01.jpg" height="182" width="273" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
</div>
</li>
</ul>
</div>
<div class="star-single">
<h3>套餐特惠</h3>
<ul>
<li v-for="item in goodsList">
<div class="good-img-box">
<img src="./../images/demo01.jpg" height="182" width="273" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
</div>
</li>
</ul>
</div>
</div>
</div>
</template>
<script>
import { Search, Toast } from 'vux'
export default {
name: 'home',
data() {
return {
screenList: [{
name: '首页推荐',
active: true
}, {
name: '现代简约',
active: false
}, {
name: '西式复古',
active: false
}, {
name: '西式',
active: false
}, {
name: '西式复',
active: false
}, {
name: '西式复1',
active: false
}],
searchValue: '',//搜索框内容
screenWidth: '', //筛选框宽度
goodsList:[{
name:'漂亮花花',
price:2143
},{
name:'一台灯',
price:2143
},{
name:'漂亮花花',
price:2143
}]
}
},
components: {
Search,
Toast
},
methods: {
setFocus() {
this.$refs.search.setFocus()
},
onSubmit() {
this.$refs.search.setBlur()
this.$vux.toast.show({
type: 'text',
position: 'top',
text: 'on submit'
})
},
onCancel() {
console.log('on cancel')
},
calcScreenWidth() {
var tempWidth = 0;
for(var i = 0; i < this.screenList.length; i++) {
// console.log(this.screenList[i].name.length)
tempWidth += this.$refs.screenBox.children[i].clientWidth + 16;
}
return tempWidth + 1;
},
changeScreen(item){
for(var i=0; i<this.screenList.length; i++){
this.screenList[i].active = false;
}
item.active = !item.active;
}
},
mounted() {
this.screenWidth = this.calcScreenWidth();
},
computed: {
}
}
</script>
<style scoped lang="less">
@borderColor: #F7F8Fd;
@whiteColor: #FFFFFF;
.home {
.weui-search-bar {
background-color: red;
}
.top-info {
100%;
height: 200px;
background-color: #bcccdc;
border-bottom: 10px solid @borderColor;
}
.nearby-store,.special-banner {
100%;
height: 87px;
background-color: #bcccdc;
border-bottom: 10px solid @borderColor;
img{
100%;
height: 100%;
}
}
.screen-list {
padding: 0px 15px;
height: 50px;
background-color: @whiteColor;
border-bottom: 10px solid @borderColor;
position: relative;
.screen-box {
96%;
height: 100%;
overflow-x: scroll;
overflow-y: hidden;
ul {
auto;
white-space:nowrap;
line-height: 50px;
float: left;
li {
float: left;
margin: 0px 8px;
font-size: 14px;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
color: #999999;
}
li.active{
color: #000000;
border-bottom: 2px solid #000000;
}
}
}
.icon-right {
position: absolute;
right: 5px;
top: 10px;
}
}
.good-list {
.star-single {
border-bottom: 10px solid @borderColor;
background-color: @whiteColor;
h3 {
height: 50px;
line-height: 50px;
text-indent: 20px;
}
ul {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
li {
48%;
.good-img-box {
100%;
height: 182px;
background-color: #eaeeef;
img {
100%;
height: 100%;
}
}
.good-info-box {
height: 78px;
padding: 10px 20px 0px;
font-size: 14px;
.good-name {
height: 43px;
-webkit-line-clamp: 2;
/*用来限制在一个块元素显示的文本的行数*/
display: -webkit-box;
/*必须结合的属性,将对象作为弹性伸缩盒子模型显示*/
-webkit-box-orient: vertical;
/*必须结合的属性 ,设置或检索伸缩盒对象的子元素的排列方式*/
overflow: hidden;
}
span{
font-size: 16px;
}
}
}
}
}
}
}
</style>
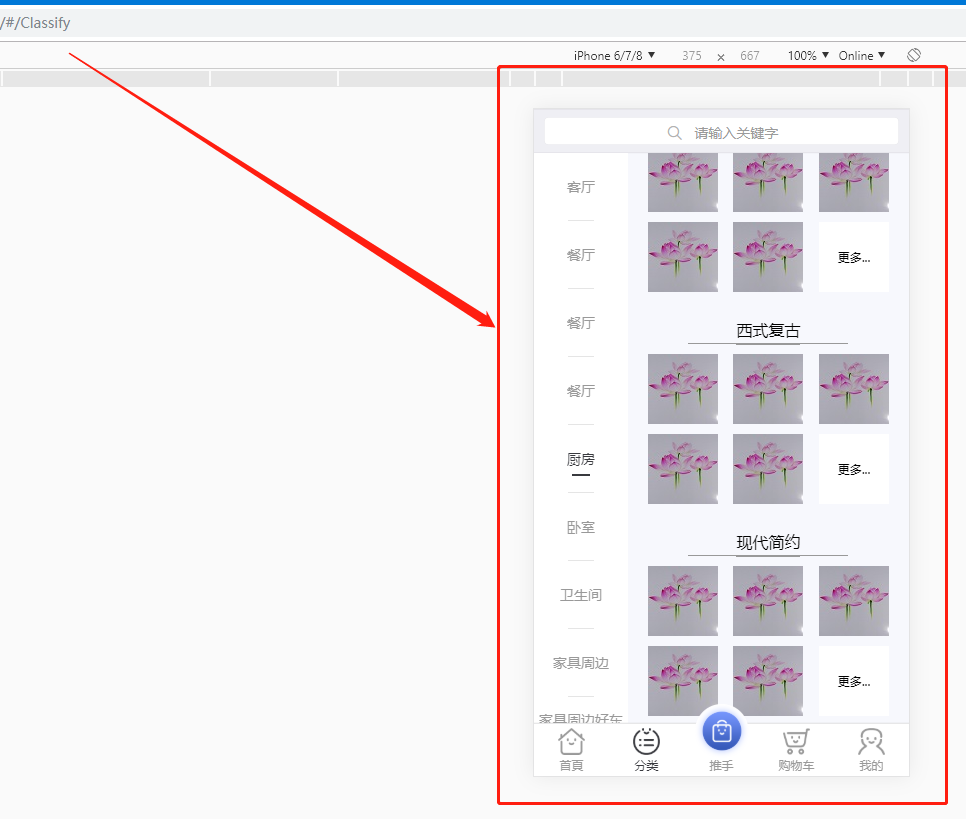
//Classify.vue
<template>
<div class="type">
<div class="search-box">
<search v-model="searchValue" position="absolute" auto-scroll-to-top top="0px" @on-cancel="onCancel" @on-submit="onSubmit" placeholder="请输入关键字" ref="search"></search>
</div>
<!-- 左侧固定部分 -->
<div class="type-list" ref="typeListHeight">
<ul>
<li v-for="item in typeList" :class="{active:item.active}" @click="getTypeMain(item)">
<span>{{item.name}}<i></i></span>
<i></i>
</li>
</ul>
</div>
<div class="type-main" ref="typeMainHeight">
<div class="type-main-banner">
<!--banner图-->
</div>
<div class="type-main-content">
<div class="main-content-title">
<span>现代简约</span>
<i></i>
</div>
<ul>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li @click="toClassifyDetail">更多...</li>
</ul>
</div>
<div class="type-main-content">
<div class="main-content-title">
<span>西式复古</span>
<i></i>
</div>
<ul>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li>更多...</li>
</ul>
</div>
<div class="type-main-content">
<div class="main-content-title">
<span>现代简约</span>
<i></i>
</div>
<ul>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li><img src="./../images/7070.jpg" height="70" width="70" alt=""></li>
<li>更多...</li>
</ul>
</div>
</div>
</div>
</template>
<script>
import { Search, Toast } from 'vux'
window.document.title = "商品分类";
export default {
name: 'type',
data() {
return {
searchValue: '',//搜索框内容
typeList:[{name:'客厅',active:true},{name:'餐厅',active:false},{name:'餐厅',active:false},{name:'餐厅',active:false},{name:'厨房',active:false},{name:'卧室',active:false},{name:'卫生间',active:false},{name:'家具周边',active:false},{name:'家具周边好东西',active:false}]
}
},
components: {
Search,
Toast
},
methods: {
setFocus() {
this.$refs.search.setFocus()
},
onSubmit() {
this.$refs.search.setBlur()
this.$vux.toast.show({
type: 'text',
position: 'top',
text: 'on submit'
})
},
onCancel() {
console.log('on cancel')
},
getTypeMain(item){
for(var i=0; i<this.typeList.length; i++){
this.typeList[i].active = false;
}
item.active = !item.active;
},
toClassifyDetail(){
//跳转到ClassifyDetail页面
this.$router.push({
path:'/ClassifyDetail'
})
}
},
mounted(){
var tempHeight = document.documentElement.clientHeight - 94.4;
this.$refs.typeListHeight.style.height = tempHeight + "px";
this.$refs.typeMainHeight.style.height = tempHeight + "px";
}
}
</script>
<style scoped lang="less">
@color999:#999999;
@whiteColor: #FFFFFF;
.search-box{
100%;
height: 44.4px;
}
.type-list{
25%;
height: auto;
float: left;
background-color: @whiteColor;
text-align: center;
overflow-y: scroll;
overflow-x: hidden;
ul{
100%;
height: auto;
li{
100%;
height: auto;
min-height: 68px;
display: flex;
justify-content: center;
align-items: center;
position: relative;
color: @color999;
font-size: 14px;
>i{
26px;
height: 0px;
position: absolute;
bottom: 0px;
left: 50%;
margin-left: -13px;
border-bottom: 1px solid #e5e5e5;
}
}
li:last-of-type{
>i{
border: none;
}
}
li.active{
color: #393A3F;
span{
position:relative;
i{
18px;
height: 0px;
position: absolute;
bottom: -6px;
left: 50%;
margin-left: -9px;
border-bottom: 2px solid #393A3F;
}
}
}
}
}
.type-main{
75%;
float: left;
box-sizing: border-box;
padding: 0px 20px;
overflow-y: scroll;
overflow-x: hidden;
.type-main-banner{
100%;
height: 80px;
margin-top: 13px;
border-radius: 6px;
overflow: hidden;
background: blue;
}
.type-main-content{
text-align: center;
margin-top: 15px;
.main-content-title{
margin-bottom: 12px;
position: relative;
>span{
padding-bottom: 3px;
border-bottom: 1.5px solid @color999;
}
>i{
160px;
height: 0px;
display: block;
position: absolute;
bottom: -2px;
left: 50%;
margin-left: -80px;
border-bottom: 1px solid @color999;
}
}
ul{
display: flex;
justify-content: space-between;
flex-wrap: wrap;
li{
70px;
height:70px;
line-height: 70px;
font-size: 12px;
background-color: @whiteColor;
margin-bottom: 10px;
}
}
}
}
</style>
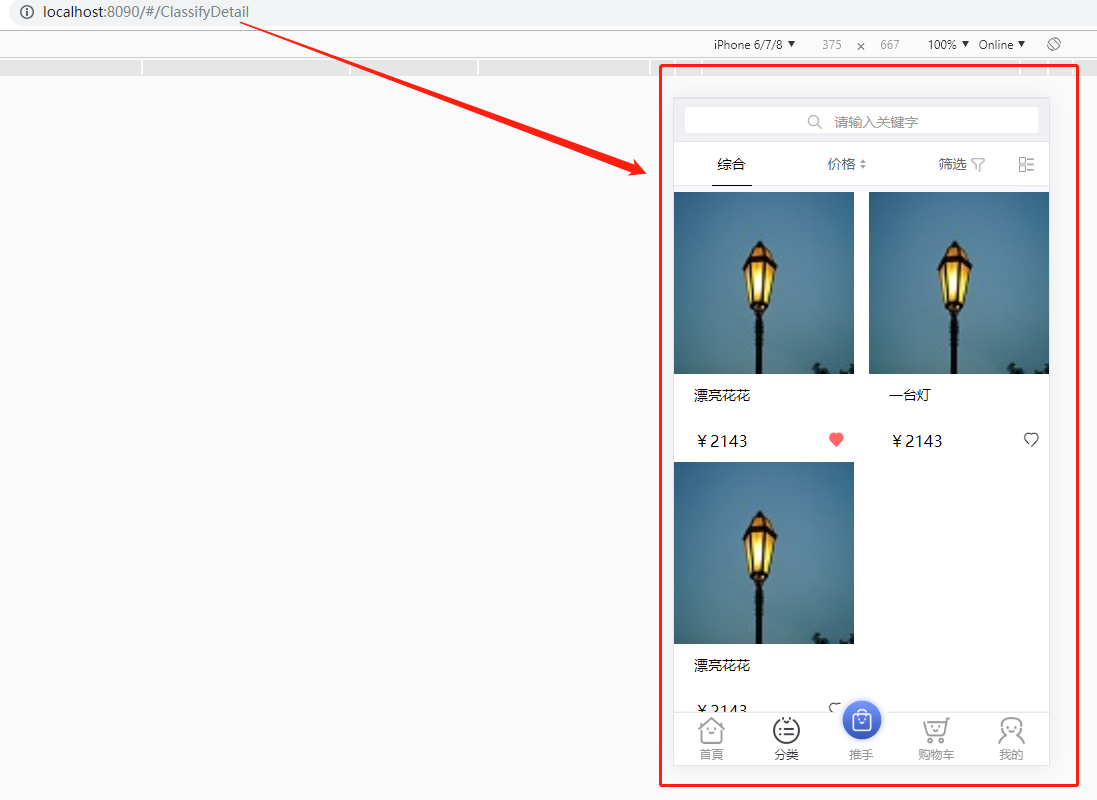
//ClassifyDetail.vue
<template>
<div class="classifyDetail">
<div class="search-box">
<search v-model="searchValue" position="absolute" auto-scroll-to-top top="0px" @on-cancel="onCancel" @on-submit="onSubmit" placeholder="请输入关键字" ref="search"></search>
</div>
<div class="screen-nav">
<div>
<tab :line-width="1" custom-bar-width="40px">
<tab-item selected @on-item-click="handleDefault">综合</tab-item>
<tab-item @on-item-click="handlePriceOrder">价格
<img class="icon-order" :src="priceOrder.img" ref="priceOrderImg" />
</tab-item>
<tab-item @on-item-click="handleScreen">筛选
<img class="icon-screen" :src="screenType.img" />
</tab-item>
</tab>
</div>
<div class="vux-1px-b" @click="changeType">
<img :src="model.img" />
</div>
</div>
<div v-show="showScreenList == true" class="screen-type-list">
<div>
<p>价格区间</p>
<div style="justify-content: space-around;">
<input type="number" name="" placeholder="最低价格" /> <i>—</i>
<input type="number" name="" placeholder="最高价格" />
</div>
</div>
<div v-for="item in screenTypeList" :style="{height:item.height + 'px'}">
<p>{{item.type}}<span v-show="item.list.length > 3" @click="showAll(item)">全部</span></p>
<checker v-model="screenChecked" type="checkbox" default-item-class="list-item" selected-item-class="list-item-selected">
<checker-item v-for="list in item.list" :value="list.id" :key="list.id"><div>{{list.name}}</div></checker-item>
</checker>
</div>
<p><span @click="confirmScreen">确定</span><span>|</span><span @click="handelReset">重置</span></p>
</div>
<div v-if="model.type == 1 && showScreenList == false" class="good-list">
<ul>
<li v-for="item in goodsList">
<div class="good-img-box">
<img src="./../images/8888.jpg" height="88" width="88" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
<img :src="item.collect.img" @click="handleCollect(item)" />
</div>
</li>
</ul>
</div>
<ul v-if="model.type == 2 && showScreenList == false" class="list-type2">
<li v-for="item in goodsList">
<div class="goods-img">
<img src="./../images/8888.jpg" height="88" width="88" />
</div>
<div class="goods-info">
<p>{{item.name}}</p>
<p><strong>¥{{item.price}}</strong></p>
</div>
<img :src="item.collect.img" @click="handleCollect(item)" />
</li>
</ul>
</div>
</template>
<script>
import { Search, Tab, TabItem, Checker, CheckerItem } from 'vux'
export default {
name: 'classifyDetail',
data() {
return {
selected: 0, //tab选中项
searchValue: '', //搜索框内容
model: { //列表展示类型
img: require('../../assets/icon-type2.png'),
type: 1
},
priceOrder: { //价格排序类型,asc升序,desc降序
type: 'default',
img: require('../../assets/icon-order.png')
},
screenType: {//筛选状态
active: false,
img: require('../../assets/icon-screen.png')
},
showScreenList:false,
screenChecked:[],//筛选项选中的内容
screenTypeList: [{
id:1,
height:'70',
type: '风格',
list: [{id: '1',name: '现代简约',}, {id: '12',name: '现代中式',}, {id: '123',name: '现代中式中式',}, {id: '1234',name: '现代中式',}, {id: '12345',name: '现代中式',}]
},{
id:2,
height:'70',
type: '控制方式',
list: [{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',}, {id: '12',name: '现代中式',}, {id: '123',name: '现代中式中式',}, {id: '1234',name: '现代中式',}, {id: '12345',name: '现代中式',}]
}],
goodsList: [{
name: '漂亮花花',
price: 2143,
collect: {
type: true,
img: require('../../assets/icon-collect-active.png')
}
}, {
name: '一台灯',
price: 2143,
collect: {
type: false,
img: require('../../assets/icon-collect.png')
}
}, {
name: '漂亮花花',
price: 2143,
collect: {
type: false,
img: require('../../assets/icon-collect.png')
}
}]
}
},
watch: {
screenChecked:function(value){
console.log(value)
}
},
methods: {
setFocus() {
this.$refs.search.setFocus()
},
onSubmit() {
this.$refs.search.setBlur()
this.$vux.toast.show({
type: 'text',
position: 'top',
text: 'on submit'
})
},
onCancel() {
console.log('on cancel')
},
changeType() {
if(this.model.type == 1) {
this.model.img = require('../../assets/icon-type1.png');
this.model.type = 2;
} else {
this.model.img = require('../../assets/icon-type2.png');
this.model.type = 1;
}
},
handleCollect(item) {
if(item.collect.type) {
item.collect.img = require('../../assets/icon-collect.png');
item.collect.type = false;
} else {
item.collect.img = require('../../assets/icon-collect-active.png');
item.collect.type = true;
}
},
resetState() {
var self = this;
function priceOrderDefault() {
self.priceOrder.type = 'default';
self.priceOrder.img = require('../../assets/icon-order.png');
self.$refs.priceOrderImg.style.transform = "rotate(0deg)";
}
function screenDefault() {
self.screenType.active = false;
self.screenType.img = require('../../assets/icon-screen.png');
}
if(this.selected == 0) {
priceOrderDefault();
screenDefault();
} else if(this.selected == 1) {
screenDefault();
} else if(this.selected == 2) {
priceOrderDefault();
}
},
handleDefault() {
this.selected = 0;
this.resetState();
},
handlePriceOrder() {
this.selected = 1;
this.resetState();
if(this.priceOrder.type == 'default') {
this.priceOrder.type = 'asc';
this.priceOrder.img = require('../../assets/icon-order-active.png');
} else if(this.priceOrder.type == 'asc') {
this.priceOrder.type = 'desc';
this.$refs.priceOrderImg.style.transform = "rotate(180deg)";
} else if(this.priceOrder.type == 'desc') {
this.priceOrder.type = 'asc';
this.$refs.priceOrderImg.style.transform = "rotate(0deg)";
}
},
handleScreen() {
this.selected = 2;
this.resetState();
if(!this.screenType.acitve) {
this.screenType.active = true;
this.showScreenList = true;//显示筛选列表
this.screenType.img = require('../../assets/icon-screen-active.png');
}
},
showAll(item){
if(item.height>70){
item.height = 70;
}else{
var num = parseInt(item.list.length/3);
num = item.list.length > num*3 ? num + 1 : num;
console.log(num)
var height = 30 + 45*num;
item.height = height;
}
},
handelReset(){//筛选项重置事件
this.screenChecked.splice(0,this.screenChecked.length);
},
confirmScreen(){//筛选项确认事件
this.showScreenList = false;//关闭筛选列表
}
},
components: {
Search,
Tab,
TabItem,
Checker,
CheckerItem
}
}
</script>
<style scoped lang="less">
@whiteColor: #FFFFFF;
.search-box {
100%;
height: 44.4px;
}
.screen-nav {
overflow: hidden;
>div:first-of-type {
float: left;
calc(~'100% - 30px');
}
>div:last-of-type {
30px;
height: 44px;
float: right;
background-color: #FFFFFF;
img {
15px;
height: 15px;
margin-top: 14.5px;
}
}
.icon-order {
6px;
height: 10px;
}
.icon-screen {
14px;
height: 14px;
vertical-align: middle;
margin-top: -2px;
}
}
.screen-type-list {
100%;
background-color: @whiteColor;
overflow: hidden;
margin-bottom: 38px;
>div {
height: 70px;
overflow: hidden;
font-size: 12px;
padding: 15px 15px 0px;
color: #646464;
transition: 0.5s;
>p {
margin-bottom: 10px;
span{
float: right;
color: #000000;
}
}
>div {
display: flex;
align-items: center;
flex-wrap: wrap;
input {
height: 35px;
38%;
border: 1px solid #E8EAF6;
text-align: center;
}
i {
color: #CCCCCC;
}
>div{
33%;
}
}
}
>p{
100%;
padding: 0px 15px;
font-size: 14px;
overflow: hidden;
position: fixed;
bottom: 50px;
left: 0px;
background-color: @whiteColor;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
span{
float: right;
padding: 8px 10px;
display: block;
}
}
}
.list-item >div{
80px;
height: 35px;
margin: 0 auto;
margin-bottom: 10px;
background-color: #f7f8fd;
text-align: center;
line-height: 35px;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
}
.list-item-selected >div{
border: 1px solid #393A3E;
color: #393A3E;
}
.good-list {
margin-top: 6px;
background-color: @whiteColor;
ul {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
li {
48%;
.good-img-box {
100%;
height: 182px;
background-color: #eaeeef;
img {
100%;
height: 100%;
}
}
.good-info-box {
height: 78px;
padding: 10px 20px 0px;
position: relative;
.good-name {
font-size: 14px;
height: 43.2px;
-webkit-line-clamp: 2;
/*用来限制在一个块元素显示的文本的行数*/
display: -webkit-box;
/*必须结合的属性,将对象作为弹性伸缩盒子模型显示*/
-webkit-box-orient: vertical;
/*必须结合的属性 ,设置或检索伸缩盒对象的子元素的排列方式*/
overflow: hidden;
}
span {
font-size: 16px;
}
img {
16px;
height: 16px;
position: absolute;
right: 10px;
bottom: 14px;
}
}
}
}
}
.list-type2 {
padding: 14px 10px 0px;
li {
100%;
height: 80px;
border-radius: 6px;
overflow: hidden;
background-color: @whiteColor;
margin-bottom: 10px;
font-size: 13px;
.goods-img {
80px;
height: 80px;
margin-right: 15px;
overflow: hidden;
background-color: #ebefef;
float: left;
img {
100%;
height: 100%;
}
}
.goods-info {
height: 80px;
float: left;
calc(~"100% - 135px");
display: flex;
flex-direction: column;
justify-content: space-around;
>p:first-of-type {
line-height: 18px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
>p:last-of-type {
float: right;
font-size: 12px;
}
}
>img {
20px;
height: 20px;
margin-top: 30px;
}
}
}
</style>
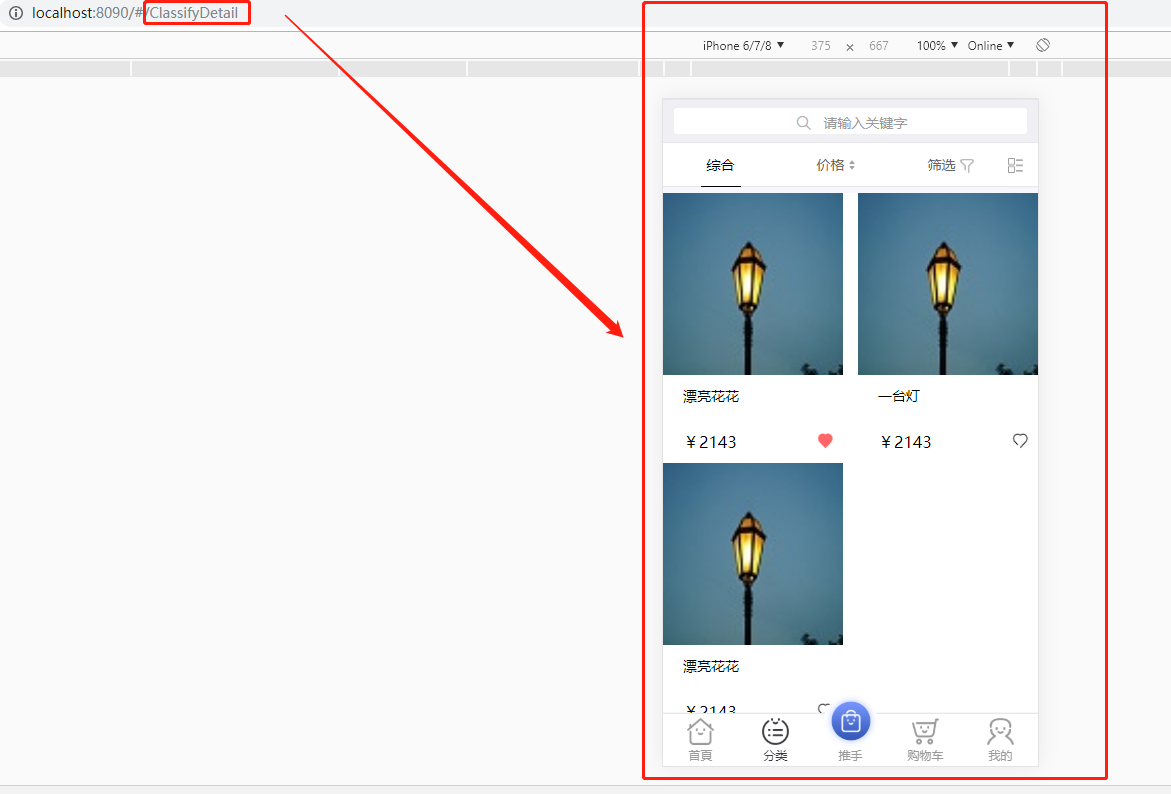
//ClassifyDetail.vue
<template>
<div class="classifyDetail">
<div class="search-box">
<search v-model="searchValue" position="absolute" auto-scroll-to-top top="0px" @on-cancel="onCancel" @on-submit="onSubmit" placeholder="请输入关键字" ref="search"></search>
</div>
<div class="screen-nav">
<div>
<tab :line-width="1" custom-bar-width="40px">
<tab-item selected @on-item-click="handleDefault">综合</tab-item>
<tab-item @on-item-click="handlePriceOrder">价格
<img class="icon-order" :src="priceOrder.img" ref="priceOrderImg" />
</tab-item>
<tab-item @on-item-click="handleScreen">筛选
<img class="icon-screen" :src="screenType.img" />
</tab-item>
</tab>
</div>
<div class="vux-1px-b" @click="changeType">
<img :src="model.img" />
</div>
</div>
<div v-show="showScreenList == true" class="screen-type-list">
<div>
<p>价格区间</p>
<div style="justify-content: space-around;">
<input type="number" name="" placeholder="最低价格" /> <i>—</i>
<input type="number" name="" placeholder="最高价格" />
</div>
</div>
<div v-for="item in screenTypeList" :style="{height:item.height + 'px'}">
<p>{{item.type}}<span v-show="item.list.length > 3" @click="showAll(item)">全部</span></p>
<checker v-model="screenChecked" type="checkbox" default-item-class="list-item" selected-item-class="list-item-selected">
<checker-item v-for="list in item.list" :value="list.id" :key="list.id"><div>{{list.name}}</div></checker-item>
</checker>
</div>
<p><span @click="confirmScreen">确定</span><span>|</span><span @click="handelReset">重置</span></p>
</div>
<div v-if="model.type == 1 && showScreenList == false" class="good-list">
<ul>
<li v-for="item in goodsList">
<div class="good-img-box">
<img src="./../images/8888.jpg" height="88" width="88" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
<img :src="item.collect.img" @click="handleCollect(item)" />
</div>
</li>
</ul>
</div>
<ul v-if="model.type == 2 && showScreenList == false" class="list-type2">
<li v-for="item in goodsList">
<div class="goods-img">
<img src="./../images/8888.jpg" height="88" width="88" />
</div>
<div class="goods-info">
<p>{{item.name}}</p>
<p><strong>¥{{item.price}}</strong></p>
</div>
<img :src="item.collect.img" @click="handleCollect(item)" />
</li>
</ul>
</div>
</template>
<script>
import { Search, Tab, TabItem, Checker, CheckerItem } from 'vux'
export default {
name: 'classifyDetail',
data() {
return {
selected: 0, //tab选中项
searchValue: '', //搜索框内容
model: { //列表展示类型
img: require('../../assets/icon-type2.png'),
type: 1
},
priceOrder: { //价格排序类型,asc升序,desc降序
type: 'default',
img: require('../../assets/icon-order.png')
},
screenType: {//筛选状态
active: false,
img: require('../../assets/icon-screen.png')
},
showScreenList:false,
screenChecked:[],//筛选项选中的内容
screenTypeList: [{
id:1,
height:'70',
type: '风格',
list: [{id: '1',name: '现代简约',}, {id: '12',name: '现代中式',}, {id: '123',name: '现代中式中式',}, {id: '1234',name: '现代中式',}, {id: '12345',name: '现代中式',}]
},{
id:2,
height:'70',
type: '控制方式',
list: [{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',},{id: '1',name: '现代简约',}, {id: '12',name: '现代中式',}, {id: '123',name: '现代中式中式',}, {id: '1234',name: '现代中式',}, {id: '12345',name: '现代中式',}]
}],
goodsList: [{
name: '漂亮花花',
price: 2143,
collect: {
type: true,
img: require('../../assets/icon-collect-active.png')
}
}, {
name: '一台灯',
price: 2143,
collect: {
type: false,
img: require('../../assets/icon-collect.png')
}
}, {
name: '漂亮花花',
price: 2143,
collect: {
type: false,
img: require('../../assets/icon-collect.png')
}
}]
}
},
watch: {
screenChecked:function(value){
console.log(value)
}
},
methods: {
setFocus() {
this.$refs.search.setFocus()
},
onSubmit() {
this.$refs.search.setBlur()
this.$vux.toast.show({
type: 'text',
position: 'top',
text: 'on submit'
})
},
onCancel() {
console.log('on cancel')
},
changeType() {
if(this.model.type == 1) {
this.model.img = require('../../assets/icon-type1.png');
this.model.type = 2;
} else {
this.model.img = require('../../assets/icon-type2.png');
this.model.type = 1;
}
},
handleCollect(item) {
if(item.collect.type) {
item.collect.img = require('../../assets/icon-collect.png');
item.collect.type = false;
} else {
item.collect.img = require('../../assets/icon-collect-active.png');
item.collect.type = true;
}
},
resetState() {
var self = this;
function priceOrderDefault() {
self.priceOrder.type = 'default';
self.priceOrder.img = require('../../assets/icon-order.png');
self.$refs.priceOrderImg.style.transform = "rotate(0deg)";
}
function screenDefault() {
self.screenType.active = false;
self.screenType.img = require('../../assets/icon-screen.png');
}
if(this.selected == 0) {
priceOrderDefault();
screenDefault();
} else if(this.selected == 1) {
screenDefault();
} else if(this.selected == 2) {
priceOrderDefault();
}
},
handleDefault() {
this.selected = 0;
this.resetState();
},
handlePriceOrder() {
this.selected = 1;
this.resetState();
if(this.priceOrder.type == 'default') {
this.priceOrder.type = 'asc';
this.priceOrder.img = require('../../assets/icon-order-active.png');
} else if(this.priceOrder.type == 'asc') {
this.priceOrder.type = 'desc';
this.$refs.priceOrderImg.style.transform = "rotate(180deg)";
} else if(this.priceOrder.type == 'desc') {
this.priceOrder.type = 'asc';
this.$refs.priceOrderImg.style.transform = "rotate(0deg)";
}
},
handleScreen() {
this.selected = 2;
this.resetState();
if(!this.screenType.acitve) {
this.screenType.active = true;
this.showScreenList = true;//显示筛选列表
this.screenType.img = require('../../assets/icon-screen-active.png');
}
},
showAll(item){
if(item.height>70){
item.height = 70;
}else{
var num = parseInt(item.list.length/3);
num = item.list.length > num*3 ? num + 1 : num;
console.log(num)
var height = 30 + 45*num;
item.height = height;
}
},
handelReset(){//筛选项重置事件
this.screenChecked.splice(0,this.screenChecked.length);
},
confirmScreen(){//筛选项确认事件
this.showScreenList = false;//关闭筛选列表
}
},
components: {
Search,
Tab,
TabItem,
Checker,
CheckerItem
}
}
</script>
<style scoped lang="less">
@whiteColor: #FFFFFF;
.search-box {
100%;
height: 44.4px;
}
.screen-nav {
overflow: hidden;
>div:first-of-type {
float: left;
calc(~'100% - 30px');
}
>div:last-of-type {
30px;
height: 44px;
float: right;
background-color: #FFFFFF;
img {
15px;
height: 15px;
margin-top: 14.5px;
}
}
.icon-order {
6px;
height: 10px;
}
.icon-screen {
14px;
height: 14px;
vertical-align: middle;
margin-top: -2px;
}
}
.screen-type-list {
100%;
background-color: @whiteColor;
overflow: hidden;
margin-bottom: 38px;
>div {
height: 70px;
overflow: hidden;
font-size: 12px;
padding: 15px 15px 0px;
color: #646464;
transition: 0.5s;
>p {
margin-bottom: 10px;
span{
float: right;
color: #000000;
}
}
>div {
display: flex;
align-items: center;
flex-wrap: wrap;
input {
height: 35px;
38%;
border: 1px solid #E8EAF6;
text-align: center;
}
i {
color: #CCCCCC;
}
>div{
33%;
}
}
}
>p{
100%;
padding: 0px 15px;
font-size: 14px;
overflow: hidden;
position: fixed;
bottom: 50px;
left: 0px;
background-color: @whiteColor;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
span{
float: right;
padding: 8px 10px;
display: block;
}
}
}
.list-item >div{
80px;
height: 35px;
margin: 0 auto;
margin-bottom: 10px;
background-color: #f7f8fd;
text-align: center;
line-height: 35px;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
}
.list-item-selected >div{
border: 1px solid #393A3E;
color: #393A3E;
}
.good-list {
margin-top: 6px;
background-color: @whiteColor;
ul {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
li {
48%;
.good-img-box {
100%;
height: 182px;
background-color: #eaeeef;
img {
100%;
height: 100%;
}
}
.good-info-box {
height: 78px;
padding: 10px 20px 0px;
position: relative;
.good-name {
font-size: 14px;
height: 43.2px;
-webkit-line-clamp: 2;
/*用来限制在一个块元素显示的文本的行数*/
display: -webkit-box;
/*必须结合的属性,将对象作为弹性伸缩盒子模型显示*/
-webkit-box-orient: vertical;
/*必须结合的属性 ,设置或检索伸缩盒对象的子元素的排列方式*/
overflow: hidden;
}
span {
font-size: 16px;
}
img {
16px;
height: 16px;
position: absolute;
right: 10px;
bottom: 14px;
}
}
}
}
}
.list-type2 {
padding: 14px 10px 0px;
li {
100%;
height: 80px;
border-radius: 6px;
overflow: hidden;
background-color: @whiteColor;
margin-bottom: 10px;
font-size: 13px;
.goods-img {
80px;
height: 80px;
margin-right: 15px;
overflow: hidden;
background-color: #ebefef;
float: left;
img {
100%;
height: 100%;
}
}
.goods-info {
height: 80px;
float: left;
calc(~"100% - 135px");
display: flex;
flex-direction: column;
justify-content: space-around;
>p:first-of-type {
line-height: 18px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
>p:last-of-type {
float: right;
font-size: 12px;
}
}
>img {
20px;
height: 20px;
margin-top: 30px;
}
}
}
</style>
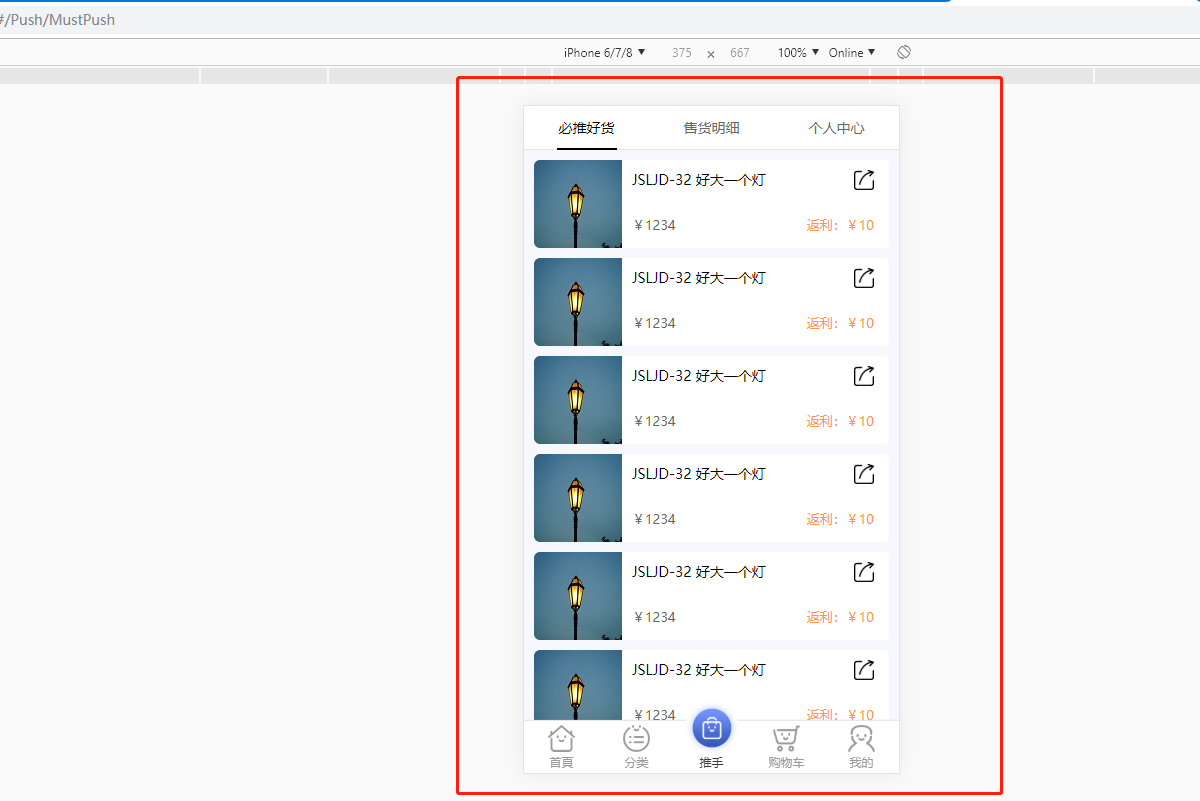
//MustPush.vue
<template>
<div class="mustPush">
<box gap="10px 10px">
<div class="mustpush-item" v-for="item in mustPushGoods">
<div class="img-box">
<img :src="item.imgSrc" />
</div>
<div class="info-box">
<flexbox :gutter="0">
<flexbox-item :span="9">
<div class="goods-name">{{item.name}}</div>
</flexbox-item>
<flexbox-item :span="1"></flexbox-item>
<flexbox-item :span="2">
<div class="icon-share"><img src="../../assets/icon-share.png" /></div>
</flexbox-item>
</flexbox>
<flexbox>
<flexbox-item>
<div class="goods-price">¥{{item.price}}</div>
</flexbox-item>
<flexbox-item>
<div class="return-price">返利:¥{{item.returnPrice}}</div>
</flexbox-item>
</flexbox>
</div>
</div>
</box>
</div>
</template>
<script>
import { Box, Flexbox, FlexboxItem } from 'vux'
export default {
name: 'mustPush',
data() {
return {
mustPushGoods: [{
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}, {
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}, {
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}, {
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}, {
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}, {
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}, {
imgSrc: require('./../images/8888.jpg'),
name: 'JSLJD-32 好大一个灯',
price: '1234',
returnPrice: '10'
}]
}
},
components: {
Box,
Flexbox,
FlexboxItem,
},
}
</script>
<style scoped lang="less">
@width88:88px;
.mustpush-item{
background: #FFFFFF;
100%;
height: @width88;
border-radius: 6px;
overflow: hidden;
margin-bottom: 10px;
.img-box{
@width88;
height: @width88;
background-color:#eaeeef;
float:left;
img{
100%;
height: 100%;
}
}
.info-box{
float: right;
width : calc(~"100% - @{width88}");
height: 100%;
padding: 10px 15px 10px 10px;
font-size: 14px;
box-sizing: border-box;
.goods-name{
height: 40px;
line-height: 20px;
-webkit-line-clamp: 2;
/*用来限制在一个块元素显示的文本的行数*/
display: -webkit-box;
/*必须结合的属性,将对象作为弹性伸缩盒子模型显示*/
-webkit-box-orient: vertical;
/*必须结合的属性 ,设置或检索伸缩盒对象的子元素的排列方式*/
overflow: hidden;
}
.icon-share{
text-align: right;
height: 40px;
img{
20px;
height: 20px;
}
}
.goods-price{
margin-top: 5px;
font-size: 13px;
color: #666666;
}
.return-price{
float: right;
margin-top: 5px;
font-size: 13px;
color: #ff9446;
}
}
}
</style>
//SaleDetails.vue
<template>
<div class="saleDetails">
<box gap="10px 10px">
<div class="mustpush-item" v-for="item in mustPushGoods">
<div class="img-box">
<img :src="item.imgSrc" />
</div>
<div class="info-box">
<flexbox :gutter="0">
<flexbox-item :span="9">
<div class="goods-name">{{item.name}}</div>
</flexbox-item>
<flexbox-item :span="1"></flexbox-item>
<flexbox-item :span="2">
<!-- 这种方式不错 -->
<div class="icon-state" v-if="item.state == 'getBill'"><img src="../../assets/icon-bill-get.png" /></div>
<div class="icon-state" v-if="item.state == 'waitBill'"><img src="../../assets/icon-bill-wait.png" /></div>
<div class="icon-state" v-if="item.state == 'billUnavailable'"><img src="../../assets/icon-bill-unavailable.png" /></div>
</flexbox-item>
</flexbox>
<flexbox>
<flexbox-item>
<div class="goods-price">¥{{item.price}}</div>
</flexbox-item>
<flexbox-item>
<div class="return-price">返利:¥{{item.returnPrice}}</div>
</flexbox-item>
</flexbox>
</div>
</div>
</box>
</div>
</template>
<script>
import { Box, Flexbox, FlexboxItem } from 'vux'
export default {
name: 'saleDetails',
data() {
return {
mustPushGoods: [{
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'getBill',
}, {
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'waitBill',
}, {
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'billUnavailable',
}, {
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'waitBill',
}, {
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'billUnavailable',
}, {
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'waitBill',
}, {
imgSrc: require('./../images/8888.jpg'),
name: '好亮的灯',
price: '1234',
returnPrice: '10',
state:'billUnavailable',
}]
}
},
components: {
Box,
Flexbox,
FlexboxItem,
},
}
</script>
<style scoped lang="less">
@width88:88px;
.mustpush-item{
background: #FFFFFF;
100%;
height: @width88;
border-radius: 6px;
/*overflow: hidden;*/
margin-bottom: 10px;
.img-box{
@width88;
height: @width88;
background-color:#eaeeef;
float:left;
border-top-left-radius:6px;
border-bottom-left-radius:6px;
overflow:hidden;
img{
100%;
height: 100%;
}
}
.info-box{
float: right;
width : calc(~"100% - @{width88}");
height: 100%;
padding: 10px 15px 10px 10px;
font-size: 14px;
box-sizing: border-box;
.goods-name{
height: 40px;
line-height: 20px;
-webkit-line-clamp: 2;
/*用来限制在一个块元素显示的文本的行数*/
display: -webkit-box;
/*必须结合的属性,将对象作为弹性伸缩盒子模型显示*/
-webkit-box-orient: vertical;
/*必须结合的属性 ,设置或检索伸缩盒对象的子元素的排列方式*/
overflow: hidden;
}
.icon-state{
text-align: right;
height: 40px;
img{
/* 20px;*/
height: 26px;
margin-right: -20px;
}
}
.goods-price{
margin-top: 5px;
font-size: 13px;
color: #666666;
}
.return-price{
float: right;
margin-top: 5px;
font-size: 13px;
color: #ff9446;
}
}
}
</style>
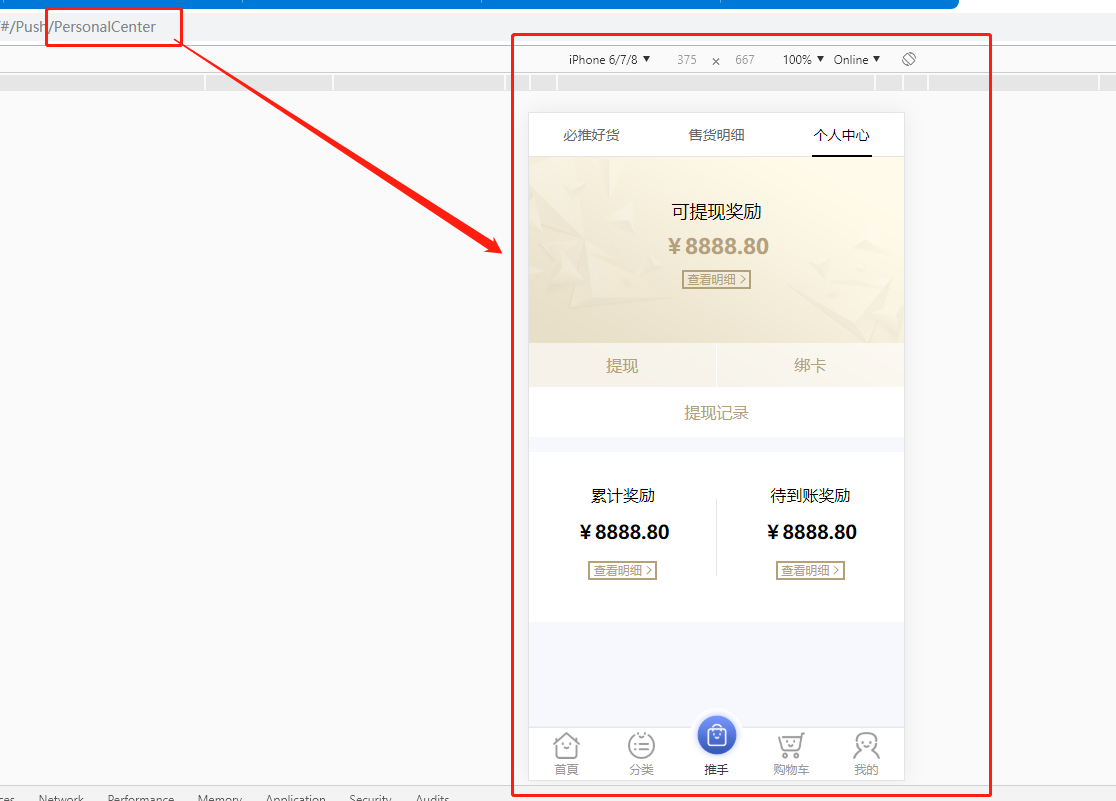
//PersonalCenter.vue
<template>
<div class="personalCenter">
<div class="info-panel">
<p>可提现奖励</p>
<h3>¥8888.80</h3>
<a href="javascript:;" class="watch-details">查看明细<x-icon type="ios-arrow-right" size="14"></x-icon></a>
<p>
<a href="javascript:;" class="withdraw">提现</a>
<a href="javascript:;">绑卡</a>
</p>
</div>
<p class="record">提现记录</p>
<div class="reward-box">
<div class="grand-total">
<p>累计奖励</p>
<h3>¥8888.80</h3>
<a href="javascript:;" class="watch-details">查看明细<x-icon type="ios-arrow-right" size="14"></x-icon></a>
<div class="border-right"></div>
</div>
<div class="waitfor-account">
<p>待到账奖励</p>
<h3>¥8888.80</h3>
<a href="javascript:;" class="watch-details">查看明细<x-icon type="ios-arrow-right" size="14"></x-icon></a>
</div>
</div>
</div>
</template>
<script>
export default {
name: 'personalCenter',
data() {
return {
}
}
}
</script>
<style scoped lang="less">
@color:#b4a179;
.vux-x-icon {
fill: @color;
vertical-align: middle;
margin-top: -3px;
}
.info-panel{
100%;
height: 230px;
background: url(../../assets/bg-center-panel.png) no-repeat center;
background-size: 100% 100%;
position: relative;
font-size: 18px;
text-align: center;
p:first-of-type{
padding-top: 40px;
margin-bottom: 5px;
}
h3{
color: @color;
}
p:last-of-type{
position: absolute;
bottom: 0px;
left: 0px;
100%;
height: 44px;
font-size: 16px;
a{
color: #000000;
float: left;
50%;
height: 100%;
text-align: center;
line-height: 44px;
color: @color;
background-color: rgba(255,255,255,0.6);
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
}
.withdraw{
border-right: 1px solid #FFFFFF;
}
}
}
.watch-details{
57px;
height: 16px;
border: 1.5px solid @color;
color: @color;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
padding-left: 4px;
text-align: center;
line-height: 16px;
font-size: 0.6em;
}
.record{
100%;
height: 50px;
line-height: 50px;
background-color: #FFFFFF;
text-align: center;
color: @color;
margin-bottom: 15px;
cursor: pointer;
}
.reward-box{
100%;
height: 170px;
background-color: #FFFFFF;
>div{
50%;
height: 100%;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
text-align: center;
float: left;
position: relative;
p{
padding-top: 30px;
margin-bottom: 10px;
}
h3{
margin-bottom: 10px;
}
.border-right{
0px;
height: 77px;
border-right: 1px solid #e6e6e6;
position: absolute;
right: 0px;
top: 50%;
margin-top: -38px;
}
}
}
</style>

//Push.vue
<template>
<div class="push">
<tab :line-width="2" custom-bar-width="60px">
<tab-item selected @on-item-click="toMustPush">必推好货</tab-item>
<tab-item @on-item-click="toSaleDetails">售货明细</tab-item>
<tab-item @on-item-click="toCenter">个人中心</tab-item>
</tab>
<view-box ref="viewBox">
<router-view></router-view>
</view-box>
</div>
</template>
<script>
import { Tab, TabItem, ViewBox } from 'vux'
export default {
name: 'push',
data() {
return {
selected:'mustPush',//选中状态
mustPushGoods:[{
imgSrc:'',
name:'JSLJD-32 好大一个灯',
price:'1234',
returnPrice:'10'
},{
imgSrc:'',
name:'JSLJD-32 好大一个灯',
price:'1234',
returnPrice:'10'
},{
imgSrc:'',
name:'JSLJD-32 好大一个灯',
price:'1234',
returnPrice:'10'
}]
}
},
components: {
Tab,
TabItem,
ViewBox
},
methods: {
toMustPush(){
this.$router.push({
path:'/Push/MustPush'
})
},
toSaleDetails(){
this.$router.push({
path:'/Push/SaleDetails'
})
},
toCenter(){
this.$router.push({
path:'/Push/PersonalCenter'
})
},
}
}
</script>
<style scoped lang="less">
</style>
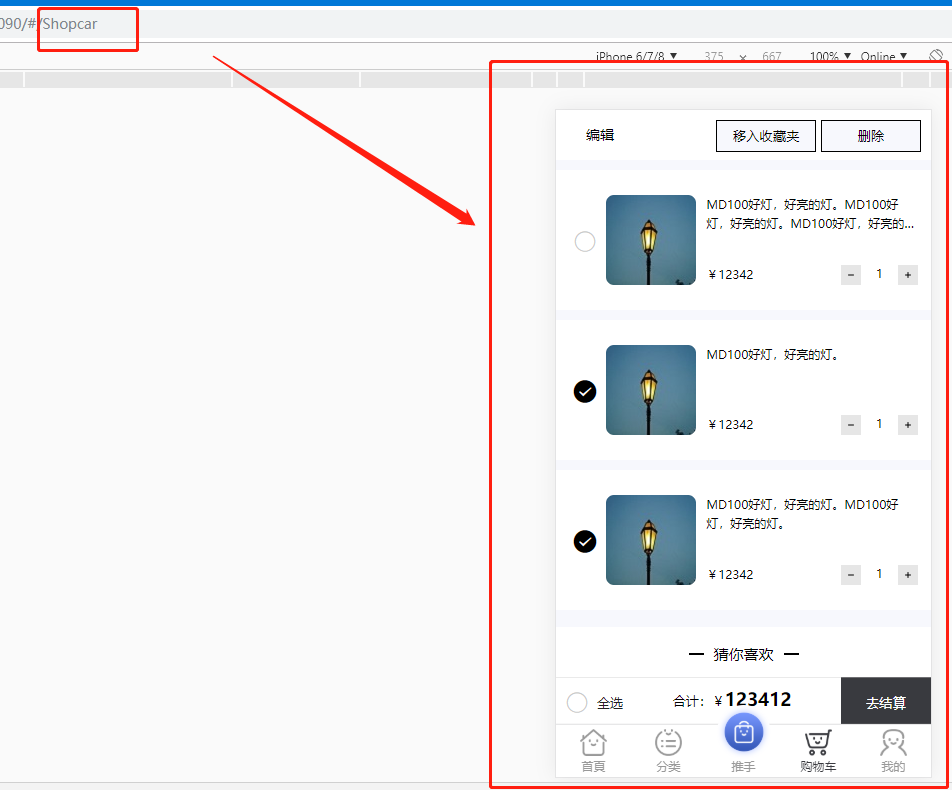
//Shopcar.vue
<template>
<div class="shopcar">
<div class="handle">
<span>编辑</span>
<button>移入收藏夹</button>
<button>删除</button>
</div>
<div class="car-item" v-for="item in shopcarList">
<check-icon :value.sync="item.checked" @click.native="handleCheckedItem(item)"></check-icon>
<div class="car-img">
<img src="./../images/9999.jpg" />
</div>
<div class="car-info">
<div class="goods-name">{{item.name}}</div>
<div class="price-count">
<span>¥{{item.price}}</span>
<CalcNumber :count.sync="item.count"></CalcNumber>
</div>
</div>
</div>
<!--猜你喜欢-->
<div class="guess-like">
<div class="guess-title">
<i></i>
<span>猜你喜欢</span>
<i></i>
</div>
<ul>
<li v-for="item in recommendList">
<div class="good-img-box">
<img src="./../images/demo01.jpg" height="182" width="273" />
</div>
<div class="good-info-box">
<div class="good-name">
{{item.name}}
</div>
<span class="good-price">¥{{item.price}}</span>
</div>
</li>
</ul>
</div>
<!--合计结算-->
<div class="total-settlement vux-1px-t">
<check-icon :value.sync="checkedAll" @click.native="handleCheckedAll">全选</check-icon>
<span>合计:¥<strong>123412</strong></span>
<button>去结算</button>
</div>
</div>
</template>
<script>
import { XButton, CheckIcon } from 'vux'
import CalcNumber from '../../components/CalcNumber.vue'
export default {
name: 'shopcar',
data() {
return {
checkedAll: false,//全选
shopcarList: [{//购物车的商品
name: 'MD100好灯,好亮的灯。MD100好灯,好亮的灯。MD100好灯,好亮的灯。',
price: 12342,
count: 1,
checked:false,
}, {
name: 'MD100好灯,好亮的灯。',
price: 12342,
count: 1,
checked:true,
}, {
name: 'MD100好灯,好亮的灯。MD100好灯,好亮的灯。',
price: 12342,
count: 1,
checked:true,
}],
recommendList:[{//推荐的商品
name:'这是一个推荐的灯,好靓的灯。',
price:2143
},{
name:'这是一个推荐的灯',
price:2143
}]
}
},
components: {
XButton,
CheckIcon,
CalcNumber
},
methods: {
change(val) {
console.log('change', val)
},
handleCheckedItem(item){
var isAll = true;
this.shopcarList.forEach((obj)=>{
if(obj.checked == false){
isAll = false;
}
})
if(isAll){
this.checkedAll = true;
}else{
this.checkedAll = false;
}
},
handleCheckedAll(){
if(this.checkedAll == true){
this.shopcarList.forEach((obj)=>{
obj.checked = true;
})
}else{
this.shopcarList.forEach((obj)=>{
obj.checked = false;
})
}
}
}
}
</script>
<style scoped lang="less">
@white: #FFFFFF;
.handle {
height: 50px;
padding: 0px 10px;
background-color: @white;
text-align: right;
line-height: 50px;
span {
float: left;
margin-left: 20px;
font-size: 14px;
}
button {
100px;
height: 32px;
background-color: #F7F8FD;
border: 1px solid #000000;
}
}
.car-item {
height: 90px;
padding: 25px 13px;
background-color: @white;
margin-top: 10px;
display: flex;
align-items: center;
>div {
float: left;
}
.car-img {
90px;
height: 90px;
border-radius: 8px;
overflow: hidden;
background-color: #eaeeef;
margin: 0px 10px 0px 5px;
img {
100%;
height: 100%;
}
}
.car-info {
calc(~"100% - 137.2px");
height: 100%;
text-wrap: wrap;
font-size: 12px;
display: flex;
flex-direction: column;
justify-content: space-between;
.goods-name {
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
.price-count {
display: flex;
justify-content: space-between;
align-items: center;
button {
20px;
height: 20px;
border: none;
}
span {
display: inline-block;
30px;
text-align: center;
}
}
}
}
.guess-like {
100%;
background-color: @white;
margin-top: 17px;
margin-bottom:50px;
.guess-title {
100%;
height: 54px;
display: flex;
justify-content: center;
align-items: center;
font-size: 15px;
i {
display: inline-block;
15px;
height: 2px;
background-color: #000000;
margin: 0px 10px;
}
}
ul {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
li {
48%;
.good-img-box {
100%;
height: 182px;
background-color: #eaeeef;
img {
100%;
height: 100%;
}
}
.good-info-box {
height: 78px;
padding: 10px 20px 0px;
font-size: 14px;
.good-name {
height: 43px;
-webkit-line-clamp: 2;
/*用来限制在一个块元素显示的文本的行数*/
display: -webkit-box;
/*必须结合的属性,将对象作为弹性伸缩盒子模型显示*/
-webkit-box-orient: vertical;
/*必须结合的属性 ,设置或检索伸缩盒对象的子元素的排列方式*/
overflow: hidden;
}
span {
font-size: 16px;
}
}
}
}
}
.total-settlement{
100%;
height: 50px;
padding-left: 5px;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
background-color: @white;
position: fixed;
bottom: 50px;
left: 0px;
display: flex;
justify-content: space-between;
align-items: center;
font-size: 13px;
button{
90px;
height: 100%;
border: none;
background-color: #393a3f;
color: @white;
}
strong{
display: inline-block;
font-size: 18px;
padding-bottom: 6px;
}
}
</style>
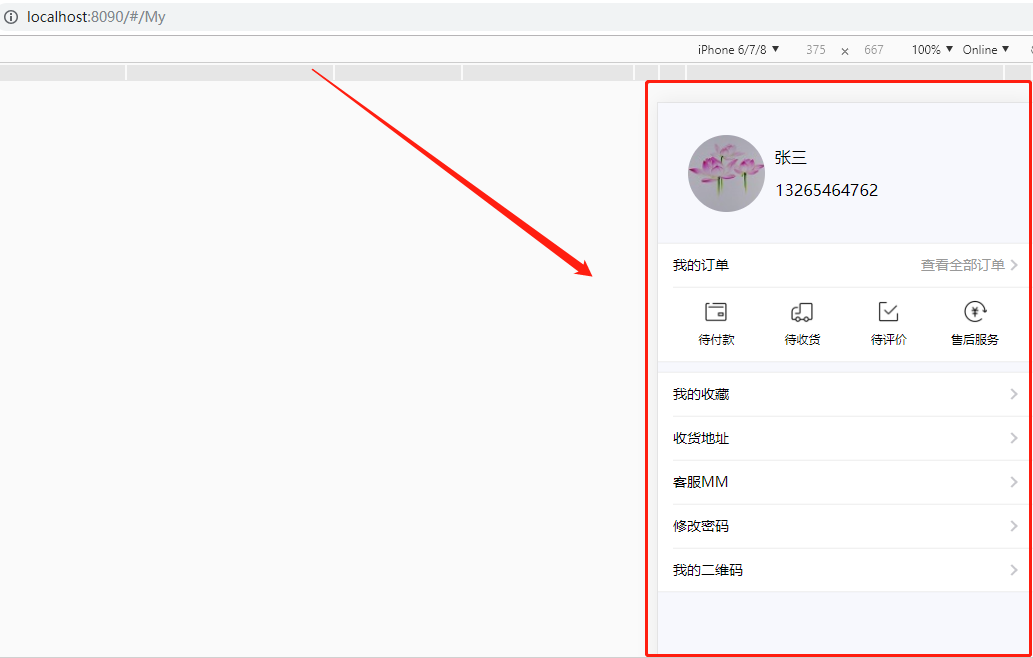
//My.vue
<template>
<div class="my">
<div class="user-info">
<div class="user-img">
<img src="./../images/7070.jpg" height="70" width="70" />
</div>
<div class="name-mobile">
<p>{{name}}</p>
<p>{{mobile}}</p>
</div>
</div>
<group style="margin-bottom: 10px;">
<cell title="我的订单" value="查看全部订单" is-link :link="{path:'/MyOrders',query:{selected:'All'}}"></cell>
<cell-box>
<div class="order-type-box">
<div class="type-item" @click="">
<img src="../../assets/icon-type-wait.png"/>
<span>待付款</span>
</div>
<div class="type-item" @click="toWaitGet">
<img src="../../assets/icon-type-get.png"/>
<span>待收货</span>
</div>
<div class="type-item" @click="toWaitAccess">
<img src="../../assets/icon-type-assess.png"/>
<span>待评价</span>
</div>
<div class="type-item" @click="toService">
<img src="../../assets/icon-type-service.png"/>
<span>售后服务</span>
</div>
</div>
</cell-box>
</group>
<group>
<cell title="我的收藏" is-link :link="{path:'/MyCollection'}"></cell>
<cell title="收货地址" is-link :link="{path:'/MyAddress'}"></cell>
<cell title="客服MM" is-link></cell>
<cell title="修改密码" is-link></cell>
<cell title="我的二维码" is-link :link="{path:'/MyQR'}"></cell>
</group>
</div>
</template>
<script>
import { Group, Cell, CellBox, Flexbox, FlexboxItem } from 'vux'
export default {
name: 'my',
data() {
return {
name: '张三',
mobile: '13265464762'
}
},
methods:{
toWaitPay(){
this.$router.push({
path:'/MyOrders',
query:{
selected:'waitPay'
}
})
},
toWaitGet(){
this.$router.push({
path:'/MyOrders',
query:{
selected:'waitGet'
}
})
},
toWaitAccess(){
this.$router.push({
path:'/MyOrders',
query:{
selected:'waitAccess'
}
})
},
toService(){
}
},
components: {
Group,
Cell,
CellBox,
Flexbox,
FlexboxItem,
}
}
</script>
<style scoped lang="less">
.user-info {
100%;
height: 140px;
display: flex;
align-items: center;
@width77: 77px;
>div {
height: @width77;
}
.user-img {
@width77;
border-radius: 50%;
overflow: hidden;
margin-left: 30px;
background-color: #b0b6c4;
img {
100%;
height: 100%;
}
}
.name-mobile {
padding: 5px 10px;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
display: flex;
flex-direction: column;
justify-content: space-around;
}
}
.order-type-box{
100%;
display: flex;
>div{
flex: 1;
height: 55px;
font-size: 12px;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
img{
24px;
height: 24px;
margin-bottom: 5px;
}
}
}
</style>
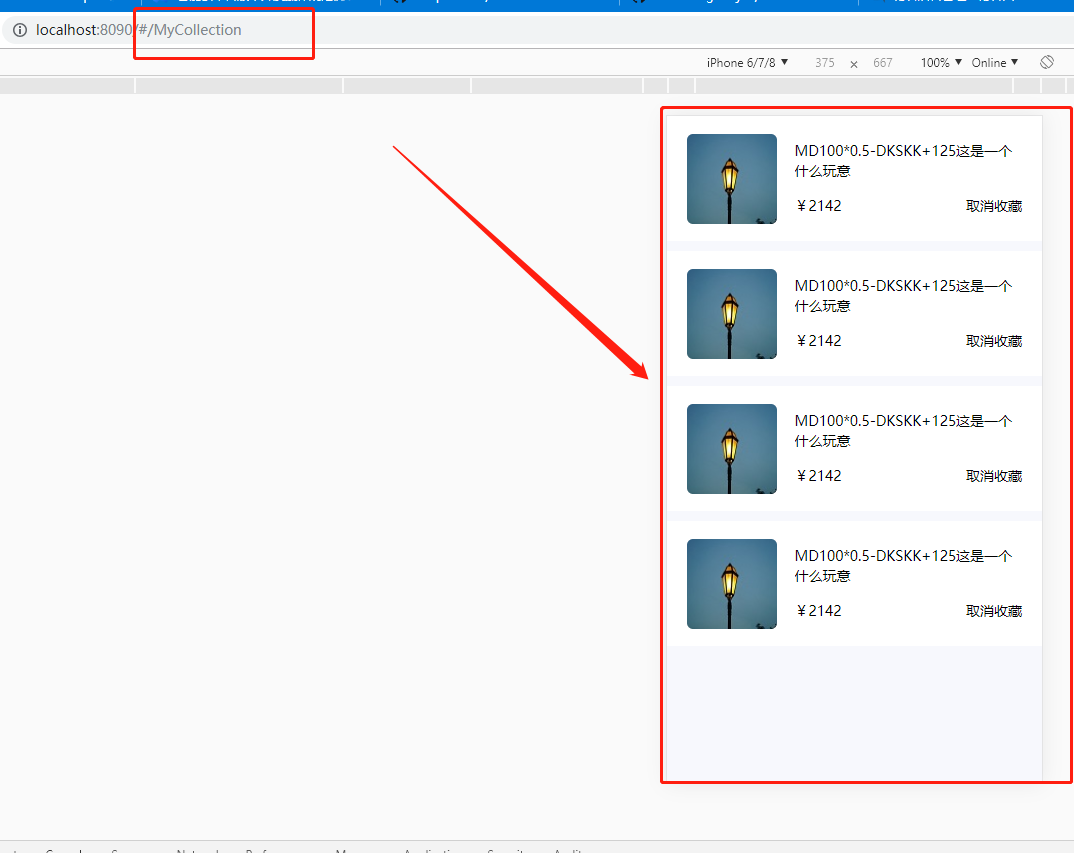
//MyCollection.vue
<template>
<div class="myCollection">
<div v-for="item in collectionList" key="item.id">
<div class="goods-img">
<img :src="item.img"/>
</div>
<div class="goods-info">
<p>{{item.name}}</p>
<div><span>¥{{item.price}}</span><a href="javascript:;">取消收藏</a></div>
</div>
</div>
</div>
</template>
<script>
export default {
name: 'myCollection',
data() {
return {
collectionList:[{
id:'1',
name:'MD100*0.5-DKSKK+125这是一个什么玩意',
price:'2142',
img:require('./../images/8888.jpg')
},{
id:'2',
name:'MD100*0.5-DKSKK+125这是一个什么玩意',
price:'2142',
img:require('./../images/8888.jpg')
},{
id:'3',
name:'MD100*0.5-DKSKK+125这是一个什么玩意',
price:'2142',
img:require('./../images/8888.jpg')
},{
id:'4',
name:'MD100*0.5-DKSKK+125这是一个什么玩意',
price:'2142',
img:require('./../images/8888.jpg')
}]
}
}
}
</script>
<style scoped lang="less">
.myCollection >div{
padding: 17.5px 20px;
height: 90px;
background-color: #FFFFFF;
font-size: 14px;
margin-bottom: 10px;
.goods-img{
90px;
height: 90px;
margin-right: 17.5px;
overflow: hidden;
border-radius: 6px;
background-color: #eaeeef;
float: left;
img{
100%;
height: 100%;
}
}
.goods-info{
height: 90px;
float: left;
calc(~"100% - 107.5px");
display: flex;
flex-direction: column;
justify-content: space-around;
>p{
line-height: 20px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
>div a{
float: right;
}
}
}
</style>
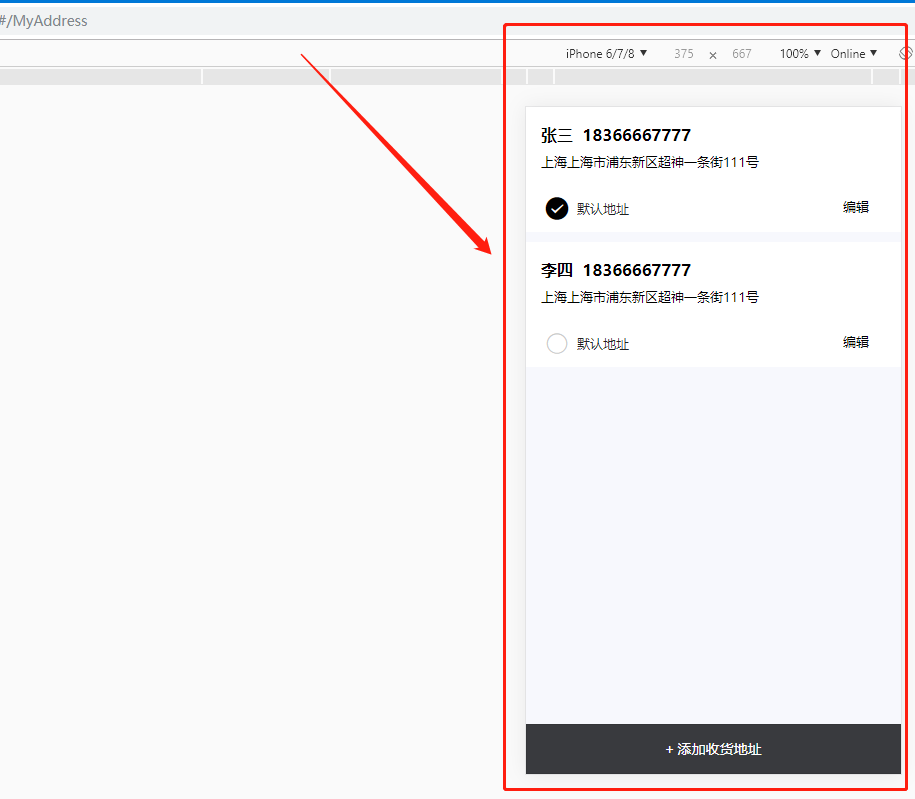
//MyAddress.vue
<template>
<div class="receiptAddress">
<div class="addres-item" v-for="item in addressList" @click="handleSelect">
<p>{{item.name}} {{item.mobile}}</p>
<p>{{item.address}}</p>
<div>
<check-icon :value.sync="item.isDefault" @click.native.stop="handleCheckedItem(item)">默认地址</check-icon>
<router-link @click.native.stop to="/AddAddress">编辑</router-link>
</div>
</div>
<x-button type="primary" class="add-btn" @click.native="handleAddAddress">+ 添加收货地址</x-button>
</div>
</template>
<script>
import { CheckIcon, XButton } from 'vux'
export default {
name: 'receiptAddress',
data() {
return {
checked:true,
addressList:[{
name:'张三',
mobile:'18366667777',
address:'上海上海市浦东新区超神一条街111号',
isDefault:true
},{
name:'李四',
mobile:'18366667777',
address:'上海上海市浦东新区超神一条街111号',
isDefault:false
}]
}
},
methods:{
handleCheckedItem(item){
this.addressList.forEach((item) => {
item.isDefault = false;
})
item.isDefault = true;
},
handleAddAddress(){
this.$router.push({
path:'/AddAddress'
})
},
handleSelect(){
this.$router.push({
path:'/PerfectOrder'
})
}
},
components: {
CheckIcon,
XButton
},
}
</script>
<style scoped lang="less">
.receiptAddress{
padding-bottom: 50px;
}
.addres-item{
height: 95px;
padding: 15px;
background-color: #FFFFFF;
font-size: 13px;
margin-bottom: 10px;
p:first-of-type{
font-weight: bold;
font-size: 16px;
margin-bottom: 5px;
}
p:last-of-type{
height: 40px;
line-height: 20px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
>div{
height: 30px;
line-height: 30px;
a{
float: right;
display: inline-block;
60px;
height: 100%;
text-align: center;
}
}
}
.add-btn{
position: fixed;
bottom: 0px;
left: 0px;
font-size: 14px;
height: 50px;
}
</style>
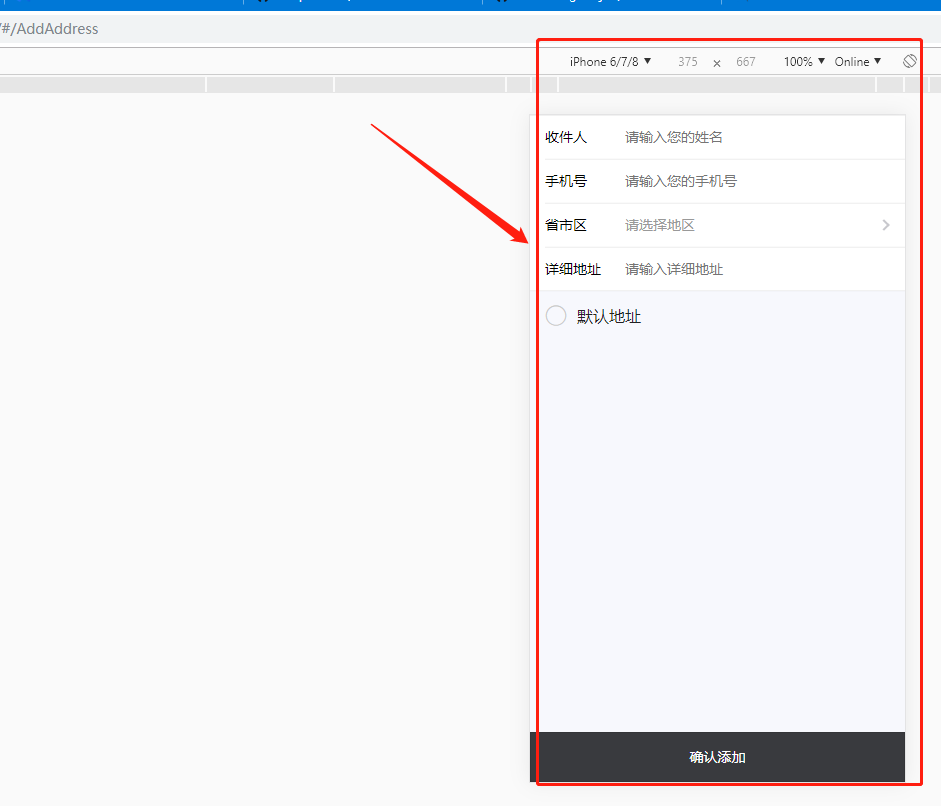
//AddAddress.vue
<template>
<div class="addAddress">
<group>
<x-input title="收件人" placeholder="请输入您的姓名" v-model="name" required text-align="left" label-width="80px"></x-input>
<x-input title="手机号" name="mobile" placeholder="请输入您的手机号" v-model="mobile" keyboard="number" is-type="china-mobile" required text-align="left" label-width="80px"></x-input>
<x-address title="省市区" placeholder="请选择地区" v-model="region" :list="addressData" value-text-align="left" label-width="80px"></x-address>
<x-input title="详细地址" placeholder="请输入详细地址" v-model="address" required text-align="left" label-width="80px"></x-input>
</group>
<check-icon class="is-default" :value.sync="isDefault" @click.native="handleCheckedItem">默认地址</check-icon>
<x-button type="primary" class="add-btn" @click.native="handleAddAddress">确认添加</x-button>
</div>
</template>
<script>
import { XInput, Group, XButton, XAddress, ChinaAddressV4Data, CheckIcon } from 'vux'
export default {
name: 'addAddress',
data() {
return {
addressData: ChinaAddressV4Data,
name:'',//收件人
mobile:'',//手机号
province:'',//省,
city:'',//市
country:'',//区
region:[],//地区
address:'',//详细地址
isDefault:false,
}
},
methods:{
handleCheckedItem(){
},
handleAddAddress(){
this.$router.push({
path:'/MyAddress'
})
}
},
components: {
XInput,
XButton,
Group,
XAddress,
CheckIcon
},
}
</script>
<style scoped lang="less">
.is-default{
margin-left: 10px;
margin-top: 10px;
}
.add-btn{
position: fixed;
bottom: 0px;
left: 0px;
font-size: 14px;
height: 50px;
}
</style>
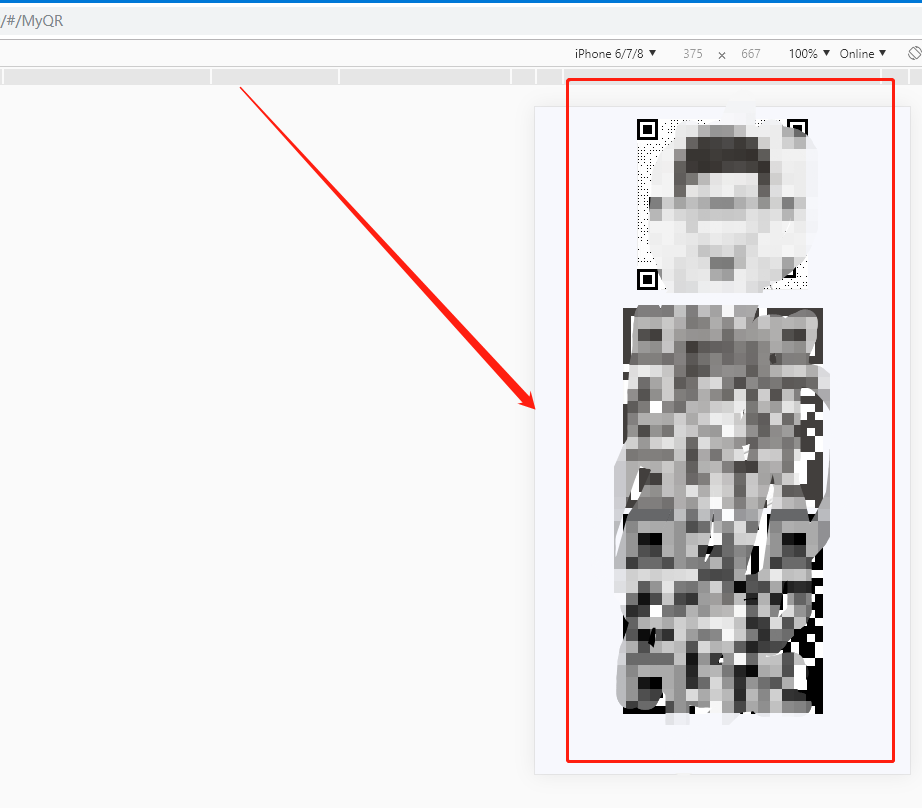
//MyQR.vue
<template>
<div class="myQR">
<vue-q-art :config="config"></vue-q-art>
<vue-qr :bgSrc='config.imagePath' :logoSrc="config.imagePath" :text="config.value" :size="200" :margin="0"></vue-qr>
<vue-qr :logoSrc="config.imagePath" :text="config.value" :size="200" :margin="0"></vue-qr>
</div>
</template>
<script>
import VueQArt from 'vue-qart'
import VueQr from 'vue-qr'
export default {
name: 'myQR',
data() {
return {
config: {
value: 'https://www.baidu.com',
imagePath: require('../../assets/img-demoUser.jpg'),
filter: 'color'
}
}
},
components: {
VueQArt,
VueQr
}
}
</script>
<style scoped lang="less">
.myQR{
text-align: center;
}
</style>
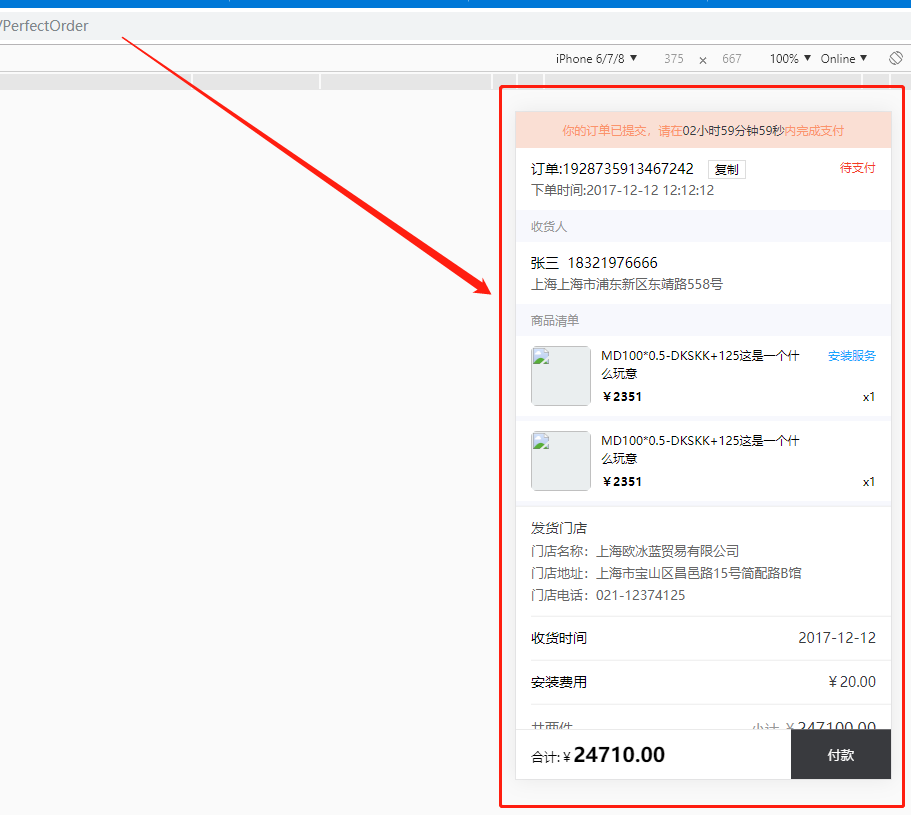
//PerfectOrder.vue
<template>
<div class="perfectOrder">
<div v-show="orderInfo.state == 'waitPay'" class="bill-warn">你的订单已提交,请在<span>02小时59分钟59秒</span>内完成支付</div>
<div class="order-info">
<p>订单:{{orderInfo.orderNumber}} <button>复制</button>
<span v-if="orderInfo.state == 'waitPay'" style="color: #f43b26;">{{orderInfo.state | filtState}}</span>
<span v-if="orderInfo.state == 'waitGet'" style="color: #f69b28;">{{orderInfo.state | filtState}}</span>
<span v-if="orderInfo.state == 'waitAccess'" style="color: #393a3e;">{{orderInfo.state | filtState}}</span>
<span v-if="orderInfo.state == 'return'" style="color: #999999;">{{orderInfo.state | filtState}}</span>
</p>
<p>下单时间:{{orderInfo.time}}</p>
</div>
<p>收货人</p>
<div class="receipt-info">
<p>{{receiptAddress.receipter}} {{receiptAddress.mobile}}</p>
<p>{{receiptAddress.address}}</p>
</div>
<p>商品清单</p>
<div class="goods-item" v-for="item in goodsList" key="item.id">
<div class="goods-img">
<img src="../images/7070.jpg" height="70" width="70" />
</div>
<div class="goods-info">
<p>{{item.name}}</p>
<span v-show="item.serviceChecked">安装服务</span>
<div><strong>¥{{item.price}}</strong><span>x{{item.count}}</span></div>
</div>
</div>
<group title="" style="margin-bottom: 50px;">
<cell-box>
<div class="store">
<p>发货门店</p>
<p><span>门店名称:</span><span>{{storeInfo.name}}</span></p>
<p><span>门店地址:</span><span>{{storeInfo.address}}</span></p>
<p><span>门店电话:</span><span>{{storeInfo.mobile}}</span></p>
</div>
</cell-box>
<cell title="收货时间">
<div>
<span style="color: #393a3e;">2017-12-12</span>
</div>
</cell>
<cell title="安装费用">
<div>
<span style="color: #393a3e;">¥20.00</span>
</div>
</cell>
<cell inline-desc="共两件">
<div>
小计 ¥<span style="color:#393a3e;font-size: 16px;">247100.00</span>
</div>
</cell>
</group>
<div v-if="orderInfo.state != 'return'" class="pay-box vux-1px-t">
<button v-if="orderInfo.state == 'waitPay'">付款</button>
<button v-if="orderInfo.state == 'waitGet'">确认收货</button>
<button v-if="orderInfo.state == 'waitAccess'">立即评价</button>
<button v-if="orderInfo.state == 'waitAccess'" style="background-color: #ff6666;">申请售后</button>
<span v-if="orderInfo.state == 'waitAccess'">合计:¥24710.00</span>
<span v-else="orderInfo.state != 'waitAccess'">合计:¥<strong>24710.00</strong></span>
</div>
</div>
</template>
<script>
import { Group, Cell, CellBox, Datetime, CheckIcon } from 'vux'
import CalcNumber from '../../components/CalcNumber.vue'
export default {
name: 'perfectOrder',
data() {
return {
orderInfo: {
orderNumber: '1928735913467242', //订单编号
time: '2017-12-12 12:12:12',
state: 'waitPay',//订单状态,(waitPay,waitGet,waitAccess,return),根据订单的状态改变页面的样式内容
},
receiptAddress: { //收货地址信息
receipter: '张三',
mobile: '18321976666',
address: '上海上海市浦东新区东靖路558号'
},
storeInfo: { //发货门店信息
name: '上海欧冰蓝贸易有限公司',
mobile: '021-12374125',
address: '上海市宝山区昌邑路15号简配路B馆'
},
receiptTime: '', //收货时间
goodsList: [{
id: '132412352',
name: 'MD100*0.5-DKSKK+125这是一个什么玩意',
price: 2351,
count: 1,
serviceChecked: true,
servicePrice: '20.00',
serviceCount: 1
}, {
id: '13247232',
name: 'MD100*0.5-DKSKK+125这是一个什么玩意',
price: 2351,
count: 1,
serviceChecked: false,
servicePrice: '20.00',
serviceCount: 1
}]
}
},
methods: {
change(value) {
console.log('change', value)
},
handleCheckedItem() {
},
toChooseAddress() {
this.$router.push({
path: '/MyAddress'
})
}
},
filters: {
filtState: function(value) {
if(value == 'waitPay') {
value = '待支付';
} else if(value == 'waitGet') {
value = '待收货';
} else if(value == 'waitAccess') {
value = '待评价';
} else if (value == 'return') {
value = '退货中';
}
return value;
}
},
components: {
Group,
Cell,
CellBox,
Datetime,
CheckIcon,
CalcNumber
}
}
</script>
<style scoped lang="less">
@white: #FFFFFF;
@black: #393a3e;
.vux-x-icon {
fill: #AAAAAA;
}
.perfectOrder>p {
font-size: 12px;
color: #959595;
line-height: 32px;
padding: 0px 15px;
}
.bill-warn {
padding: 0px 15px;
height: 36px;
text-align: center;
line-height: 36px;
font-size: 12px;
background-color: #fadfd4;
color: #fb946b;
span {
color: #393A3F;
}
}
.order-info,
.receipt-info {
padding: 10px 15px;
height: auto;
background-color: @white;
display: flex;
flex-direction: column;
justify-content: center;
p:first-of-type {
font-size: 14px;
color: #000000;
}
p:last-of-type {
font-size: 13px;
color: #626262;
}
}
.order-info {
button {
38px;
height: 19px;
border: 1px solid #DDDDDD;
background-color: #FFFFFF;
font-size: 0.7em;
margin-left: 10px;
}
span {
float: right;
font-size: 12px;
}
}
.goods-item {
padding: 10px 15px;
overflow: hidden;
font-size: 12px;
background-color: #FFFFFF;
margin-bottom: 5px;
.goods-img {
60px;
height: 60px;
margin-right: 10px;
overflow: hidden;
border-radius: 6px;
background-color: #eaeeef;
float: left;
img {
100%;
height: 100%;
}
}
.goods-info {
height: 60px;
float: left;
calc(~"100% - 70px");
display: flex;
flex-direction: column;
justify-content: space-between;
position: relative;
>p {
line-height: 18px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
>p:first-of-type {
75%;
}
>span {
position: absolute;
right: 0px;
top: 0px;
color: #19a6fe;
}
>div span:last-of-type {
float: right;
}
}
.goods-service {
100%;
display: flex;
justify-content: space-between;
align-items: center;
font-size: 12px;
}
}
.store {
>p {
100%;
font-size: 13px;
overflow: hidden;
span {
color: #666666;
display: block;
float: left;
}
span:last-of-type {
calc(~'100% - 65px');
}
}
>p:first-of-type {
font-size: 14px;
color: @black;
}
}
.pay-box {
100%;
height: 50px;
line-height: 50px;
background-color: @white;
position: fixed;
bottom: 0px;
left: 0px;
font-size: 13px;
button {
float: right;
7.5em;
height: 100%;
background-color: @black;
color: @white;
border: none;
}
>span {
float: left;
margin-left: 15px;
strong {
font-size: 20px;
}
}
}
</style>
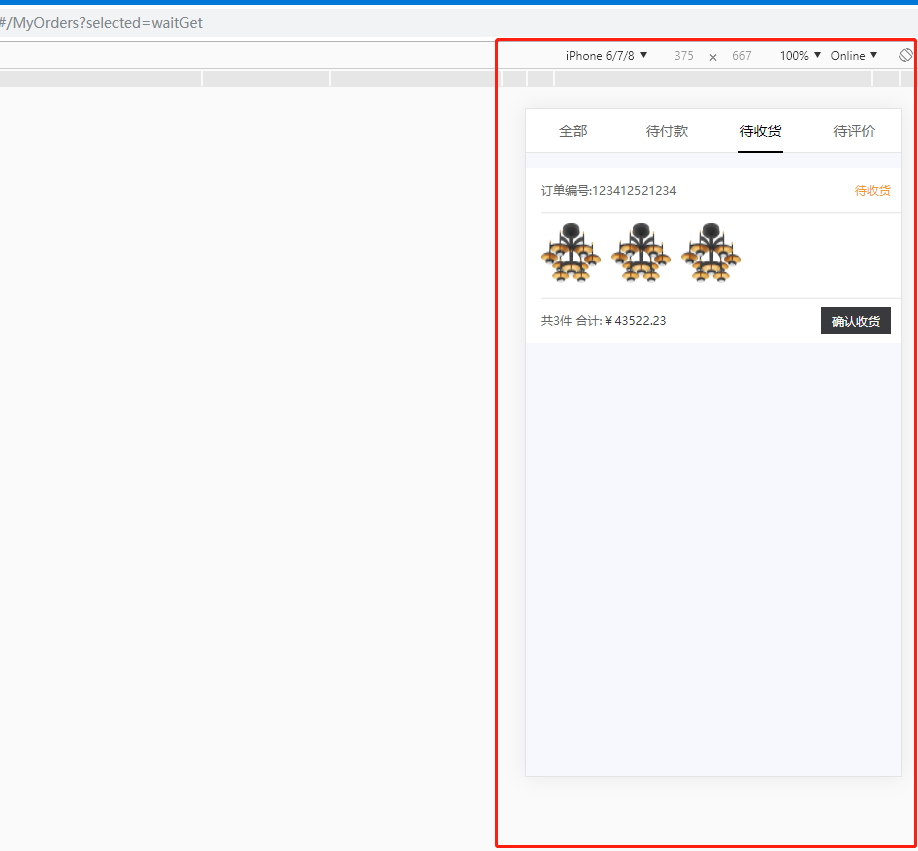
//MyOrders.vue
<template>
<div class="myOrders">
<tab :line-width="2" custom-bar-width="45px">
<tab-item :selected="selected == 'All'" @on-item-click="selected = 'All'">全部</tab-item>
<tab-item :selected="selected == 'waitPay'" @on-item-click="selected = 'waitPay'">待付款</tab-item>
<tab-item :selected="selected == 'waitGet'" @on-item-click="selected = 'waitGet'">待收货</tab-item>
<tab-item :selected="selected == 'waitAccess'" @on-item-click="selected = 'waitAccess'">待评价</tab-item>
</tab>
<!--显示全部-->
<div class="order-all">
<div v-for="item in orderAll" v-show="item.state == selected || selected == 'All'">
<div class="vux-1px-b">
<span>订单编号:{{item.number}}</span>
<span v-if="item.state == 'waitPay'" style="color:#f53c27">{{item.state | filterState}}</span>
<span v-if="item.state == 'waitGet'" style="color:#f59a27 ">{{item.state | filterState}}</span>
<span v-if="item.state=='waitAccess'" style="color:#3a3a3c ">{{item.state | filterState}}</span>
</div>
<div class="img-box ">
<div>
<img v-for="imgItem in item.imgs" :src="imgItem"/>
</div>
</div>
<div class="vux-1px-t ">
<span>共{{item.count}}件 合计:<span>¥{{item.totalPrice}}</span></span>
<button class="btn ">{{item.state | filterBtnTxt}}</button>
</div>
</div>
</div>
</div>
</template>
<script>
import { Tab, TabItem } from 'vux'
export default {
name: 'myOrders',
data() {
return {
orderAll:[{
number:'123412521234',
count:3,
totalPrice:43522.23,
state:'waitPay',
imgs:[require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png')]
},{
number:'123412521234',
count:3,
totalPrice:43522.23,
state:'waitGet',
imgs:[require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png')]
},{
number:'123412521234',
count:3,
totalPrice:43522.23,
state:'waitAccess',
imgs:[require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png')]
},{
number:'123412521234',
count:3,
totalPrice:43522.23,
state:'waitPay',
imgs:[require('../../assets/demo.png'),require('../../assets/demo.png'),require('../../assets/demo.png')]
}],
orderImgWidth:300,
selected:this.$route.query.selected ? this.$route.query.selected : 'All',//tab选中状态
}
},
components: {
Tab,
TabItem,
},
methods: {
},
mounted(){
console.log(this.$route.query)
},
filters: {
filterState: function(value){
if(value == 'waitPay'){
value = '待付款';
}else if(value == 'waitGet'){
value = '待收货';
}else if(value == 'waitAccess'){
value = '待评价';
}
return value;
},
filterBtnTxt: function(value){
if(value == 'waitPay'){
value = '立即支付';
}else if(value == 'waitGet'){
value = '确认收货';
}else if(value == 'waitAccess'){
value = '立即评价';
}
return value;
}
}
}
</script>
<style scoped lang="less">
@white:#FFFFFF;
@black:#393a3e;
.order-all{
margin-bottom: 15px;
>div{
padding-left: 15px;
background-color: @white;
font-size:12px;
margin-top:15px;
overflow-x:scroll;
color:#666666;
>div:first-of-type,>div:last-of-type{
height: 45px;
line-height: 45px;
padding-right: 10px;
display: flex;
justify-content: space-between;
align-items: center;
}
>div:last-of-type{
>span span{
color: @black;
}
button{
70px;
height: 27px;
border: none;
background-color: @black;
color: @white;
font-size: 0.85em;
}
}
.img-box{
padding: 10px 15px 10px 0px;
>div{
overflow: scroll;
auto;
white-space:nowrap;
img{
60px;
height: 60px;
margin-right: 10px;
}
}
}
}
}
</style>
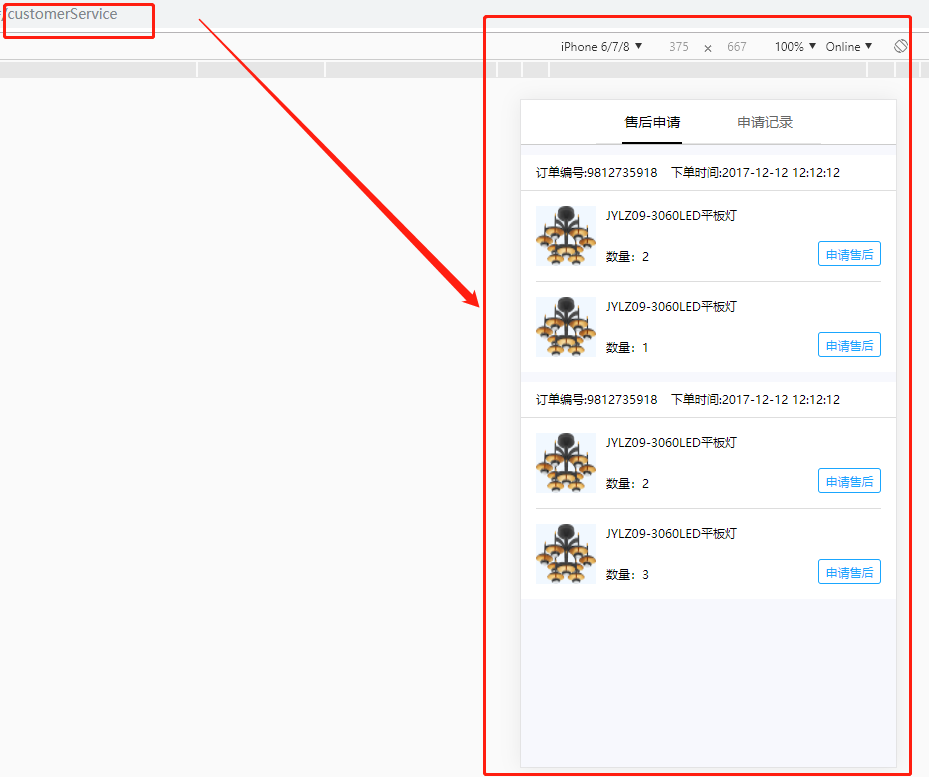
//CustomerService.vue
<template>
<div class="customerService">
<div class="tab-box">
<tab :line-width="2" custom-bar-width="60px">
<tab-item :selected="selected == 0" @on-item-click="selected = 0">售后申请</tab-item>
<tab-item :selected="selected == 1" @on-item-click="selected = 1">申请记录</tab-item>
</tab>
</div>
<div v-if="selected == 0" class="apply">
<div class="apply-item" v-for="item in orderList">
<div class="identifier-time">
<span>订单编号:{{item.identifier}}</span>
<span>下单时间:{{item.time}}</span>
</div>
<div class="list-box">
<div class="goods-list" v-for="list in item.goodsList">
<div class="img-box">
<img :src="list.img" />
</div>
<div class="name-count">
<p>{{list.name}}</p>
<p>数量:{{list.count}}</p>
<button>申请售后</button>
</div>
</div>
</div>
</div>
</div>
<div v-if="selected == 1" class="records">
<div class="list-box">
<div class="goods-list" v-for="item in recordsList">
<div class="img-box">
<img :src="item.img" />
</div>
<div class="name-count">
<p>{{item.name}}</p>
<p>数量:{{item.count}}</p>
<button v-if="item.state == 'return'">退货中</button>
<button v-if="item.state == 'exchange'" style="border-color: #e19d54;color:#e19d54;">换货中</button>
<button v-if="item.state == 'finish'" style="border-color: #999999;color:#999999;">已完成</button>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
import { Tab, TabItem } from 'vux'
export default {
name: 'customerService',
data() {
return {
selected: 0,
orderList: [{
identifier: '9812735918', //订单编号
time: '2017-12-12 12:12:12', //下单时间
goodsList: [{
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 2
}, {
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 1
}]
}, {
identifier: '9812735918', //订单编号
time: '2017-12-12 12:12:12', //下单时间
goodsList: [{
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 2
}, {
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 3
}]
}],
recordsList: [{
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 2,
state: 'return'
}, {
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 3,
state: 'exchange'
}, {
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
count: 3,
state: 'finish'
}]
}
},
components: {
Tab,
TabItem,
}
}
</script>
<style scoped lang="less">
.tab-box {
background: #FFFFFF;
border-bottom: 1px solid #CCCCCC;
padding: 0px 20%;
margin-bottom: 10px;
}
.apply-item {
background-color: #FFFFFF;
margin-bottom: 10px;
.identifier-time {
height: 35px;
line-height: 35px;
padding: 0px 15px;
font-size: 12px;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
border-bottom: 1px solid #DEDEDE;
span:last-of-type {
margin-left: 10px;
}
}
.list-box {
padding: 0px 15px;
}
}
.goods-list {
100%;
height: 60px;
padding: 15px 0px;
border-bottom: 1px solid #DDDDDD;
.img-box {
60px;
height: 100%;
float: left;
margin-right: 10px;
background-color: #f1f8ff;
overflow: hidden;
img {
100%;
height: 100%;
}
}
.name-count {
calc(~"100% - 70px");
height: 100%;
float: left;
font-size: 12px;
display: flex;
flex-direction: column;
justify-content: space-between;
position: relative;
p:first-of-type {
line-height: 18px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
button {
position: absolute;
right: 0px;
bottom: 0px;
63px;
height: 25px;
border: 1px solid #1aa6fe;
border-radius: 3px;
background-color: #FFFFFF;
color: #1aa6fe;
font-size: 0.9em;
}
}
}
.goods-list:last-of-type {
border-bottom: none;
}
.records {
background-color: #FFFFFF;
padding: 0px 15px;
}
</style>
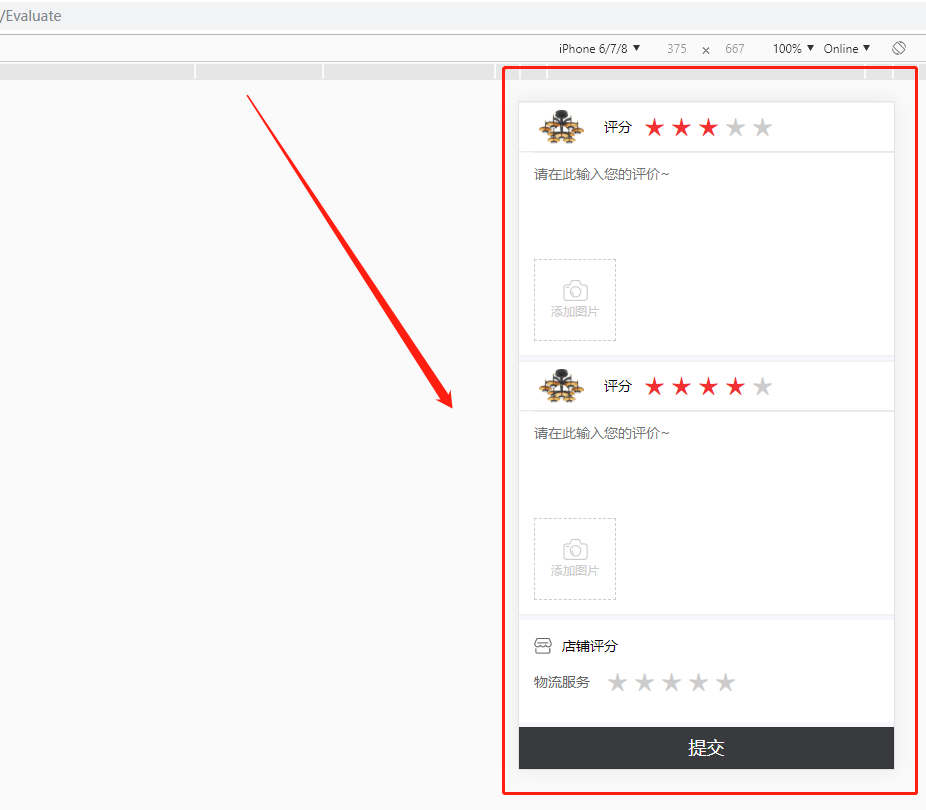
//Evaluate.vue
<template>
<div class="evaluate">
<div class="itemgoods" v-for="item in goodsList">
<group>
<div class="star-box vux-1px-b">
<img :src="item.img" /><span>评分</span>
<rater v-model="item.star" :max="5" active-color="#F23031"></rater>
</div>
<x-textarea placeholder="请在此输入您的评价~" @on-focus="onEvent('focus')" @on-blur="onEvent('blur')"></x-textarea>
<div class="uploadarea">
<div class="upload-box">
<img src="../../assets/icon-camera.png"/>
<span>添加图片</span>
</div>
</div>
</group>
</div>
<div class="store-star">
<div><img src="../../assets/icon-store.png"/>店铺评分</div>
<div><span>物流服务</span><rater v-model="storeStar" :max="5" active-color="#F23031"></rater></div>
</div>
<x-button type="primary" class="btn">提交</x-button>
</div>
</template>
<script>
import { Rater, Group, XTextarea, XButton } from 'vux'
export default {
name: 'evaluate',
data() {
return {
goodsList: [{
img: require('../../assets/demo.png'),
star: 3,
evaluate: '', //评价语
attachment: [], //上传的图片附件
},{
img: require('../../assets/demo.png'),
star: 4,
evaluate: '', //评价语
attachment: [], //上传的图片附件
}],
storeStar:0,//店铺评分
}
},
methods: {
onEvent(event) {
console.log('on', event)
}
},
components: {
Rater,
Group,
XTextarea,
XButton
},
}
</script>
<style scoped lang="less">
.itemgoods {
100%;
margin-bottom: 5px;
.star-box {
height: 50px;
100%;
display: flex;
align-items: center;
img {
45px;
height: 34px;
margin: 0px 20px;
vertical-align: middle;
}
span {
margin-right: 10px;
font-size: 14px;
}
}
}
.uploadarea{
padding: 15px;
.upload-box{
80px;
height: 80px;
border: 1px dashed #CCCCCC;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
font-size: 12px;
color: #CCCCCC;
img{
25px;
height: 21px;
}
}
}
.store-star{
background-color: #FFFFFF;
padding: 15px;
font-size: 14px;
margin-bottom: 42px;
img{
18px;
height: 16px;
vertical-align: middle;
margin-top: -3px;
margin-right: 10px;
}
>div:last-of-type{
height: 50px;
display: flex;
align-items: center;
span{
margin-right: 15px;
color: #666666;
}
}
}
.btn{
position: fixed;
bottom: 0px;
left: 0px;
}
</style>
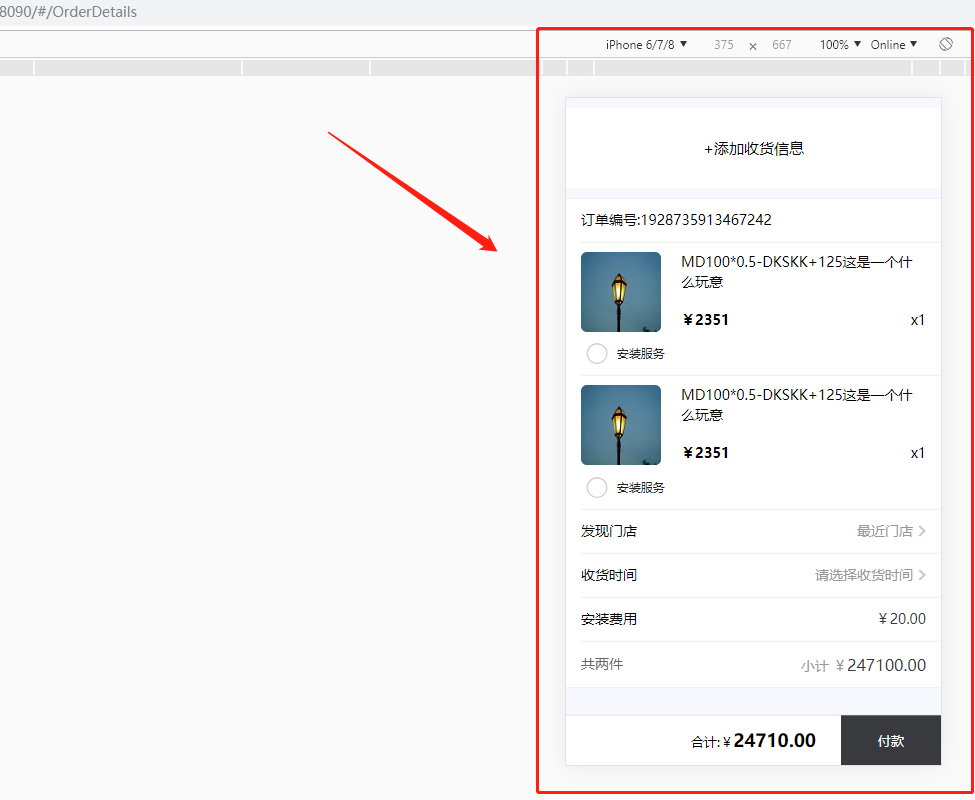
//serviceChecked
<template>
<div class="orderDetails">
<div class="receipt-info" @click="toChooseAddress">
<span v-show="!receiptAddress.show">+添加收货信息</span>
<div v-show="receiptAddress.show">
<p>{{receiptAddress.receipter}} {{receiptAddress.mobile}}</p>
<p>{{receiptAddress.address}}</p>
<x-icon class="icon-right" type="ios-arrow-forward" size="18"></x-icon>
</div>
</div>
<group style="margin-bottom: 50px;">
<cell :title="'订单编号:' + orderNumber" ></cell>
<cell-box v-for="item in goodsList" key="item.id">
<div class="goods-item">
<div class="goods-img">
<img src="../images/8888.jpg" height="88" width="88"/>
</div>
<div class="goods-info">
<p>{{item.name}}</p>
<div><strong>¥{{item.price}}</strong><span>x{{item.count}}</span></div>
</div>
<div class="goods-service">
<check-icon :value.sync="item.serviceChecked" @click.native="handleCheckedItem">安装服务</check-icon>
<span v-show="item.serviceChecked == true">服务费:¥{{item.servicePrice}}</span>
<div v-show="item.serviceChecked == true">
数量
<CalcNumber :count.sync="item.serviceCount"></CalcNumber>
</div>
</div>
</div>
</cell-box>
<cell title="发现门店" value="最近门店" is-link v-show="!storeInfo.show"></cell>
<cell-box is-link v-show="storeInfo.show">
<div class="store">
<p>发货门店</p>
<p><span>门店名称:</span>上海欧冰蓝贸易有限公司</p>
<p><span>门店地址:</span>上海市宝山区昌邑路15号简配路B馆</p>
<p><span>门店电话:</span>021-12374125</p>
</div>
</cell-box>
<datetime v-model="receiptTime" @on-change="change" title="收货时间" placeholder="请选择收货时间"></datetime>
<cell title="安装费用" >
<div>
<span style="color: #393a3e;">¥20.00</span>
</div>
</cell>
<cell inline-desc="共两件" >
<div>
小计 ¥<span style="color:#393a3e;font-size: 16px;">247100.00</span>
</div>
</cell>
</group>
<div class="pay-box vux-1px-t">
<button>付款</button>
<span>合计:¥<strong>24710.00</strong></span>
</div>
</div>
</template>
<script>
import { Group, Cell, CellBox, Datetime, CheckIcon } from 'vux'
import CalcNumber from '../../components/CalcNumber.vue'
export default {
name: 'orderDetails',
data() {
return {
receiptAddress:{//收货地址信息
show:false,
receipter:'张三',
mobile:'18321976666',
address:'上海上海市浦东新区东靖路558号'
},
storeInfo:{//发货门店信息
show:false,
name:'上海欧冰蓝贸易有限公司',
mobile:'021-12374125',
address:'上海市宝山区昌邑路15号简配路B馆'
},
receiptTime:'',//收货时间
orderNumber:'1928735913467242',//订单编号
goodsList:[{
id:'132412352',
name:'MD100*0.5-DKSKK+125这是一个什么玩意',
price:2351,
count:1,
serviceChecked:false,
servicePrice:'20.00',
serviceCount:1
},{
id:'13247232',
name:'MD100*0.5-DKSKK+125这是一个什么玩意',
price:2351,
count:1,
serviceChecked:false,
servicePrice:'20.00',
serviceCount:1
}]
}
},
methods:{
change (value) {
console.log('change', value)
},
handleCheckedItem(){
},
toChooseAddress(){
this.$router.push({
path:'/MyAddress'
})
}
},
components: {
Group,
Cell,
CellBox,
Datetime,
CheckIcon,
CalcNumber
}
}
</script>
<style scoped lang="less">
@white: #FFFFFF;
@black:#393a3e;
.vux-x-icon {
fill: #AAAAAA;
}
.receipt-info {
100%;
height: 80px;
background-color: @white;
margin-top: 10px;
margin-bottom: 10px;
display: flex;
flex-direction: column;
justify-content: center;
>span{
font-size: 15px;
display: block;
text-align: center;
}
>div{
text-indent: 15px;
position: relative;
line-height: 30px;
p:first-of-type{
font-size: 16px;
color: #000000;
}
p:last-of-type{
font-size: 13px;
color: #626262;
}
.icon-right{
position: absolute;
right: 10px;
top: 50%;
margin-top: -9px;
}
}
}
.goods-item{
100%;
overflow: hidden;
font-size: 14px;
.goods-img{
80px;
height: 80px;
margin-right: 20px;
margin-bottom: 10px;
overflow: hidden;
border-radius: 6px;
background-color: #eaeeef;
float: left;
img{
100%;
height: 100%;
}
}
.goods-info{
height: 80px;
float: left;
calc(~"100% - 100px");
display: flex;
flex-direction: column;
justify-content: space-between;
>p{
line-height: 20px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
>div span:last-of-type{
float: right;
}
}
.goods-service{
100%;
display: flex;
justify-content: space-between;
align-items: center;
font-size: 12px;
}
}
.store{
>p{
100%;
span{
color: #666666;
}
}
>p:first-of-type{
color: @black;
}
}
.pay-box{
100%;
height: 50px;
line-height: 50px;
background-color: @white;
position: fixed;
bottom: 0px;
left: 0px;
font-size: 13px;
button{
float: right;
7.5em;
height: 100%;
background-color: @black;
color: @white;
border:none;
}
>span{
float: right;
margin-right: 25px;
strong{
font-size: 18px;
}
}
}
</style>
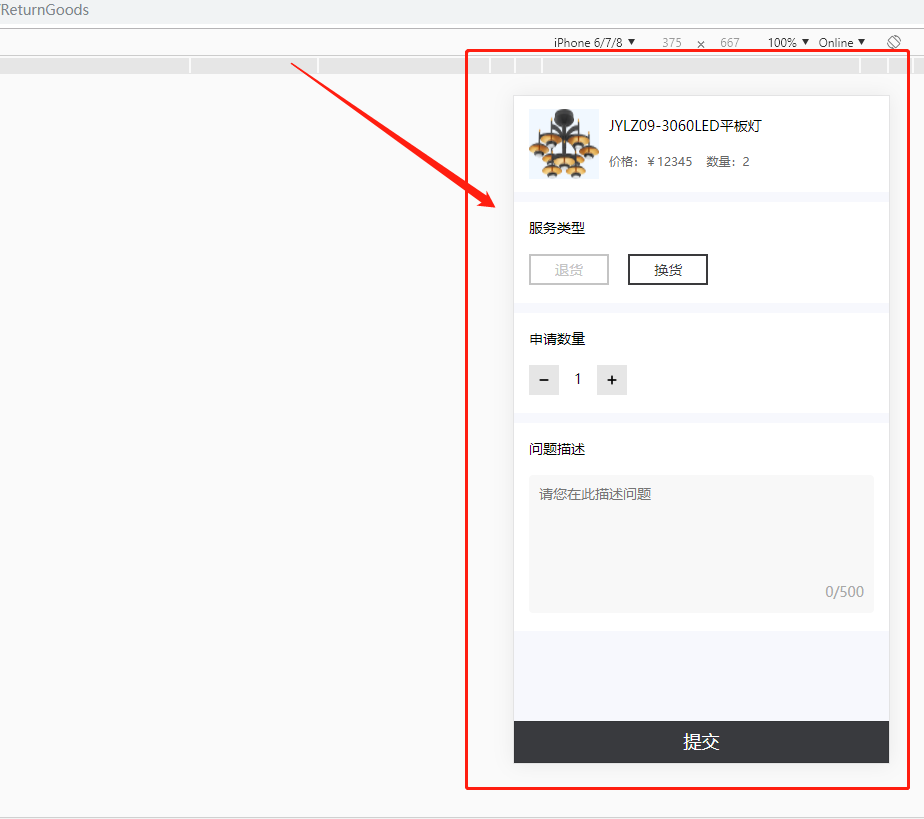
//ReturnGoods.vue
<template>
<div class="returnGoods">
<div class="goods-info">
<div class="img-box">
<img :src="goods.img" />
</div>
<div class="name-count">
<p>{{goods.name}}</p>
<p>价格:¥{{goods.price}} 数量:{{goods.count}}</p>
</div>
</div>
<div class="service-type">
<p>服务类型</p>
<checker v-model="type" default-item-class="type-item" selected-item-class="type-item-selected">
<checker-item value="return">退货</checker-item>
<checker-item value="exchange">换货</checker-item>
</checker>
</div>
<div v-show="type == 'return'" class="return-type">
<p>退款方式</p>
<span>原支付返还</span>
</div>
<div class="apply-number">
<p>申请数量</p>
<CalcNumber :count.sync="returnCount" size="30px"></CalcNumber>
</div>
<div class="description">
<p>问题描述</p>
<!--<x-textarea :max="500" name="description" placeholder="请您在此描述问题" :height="105"></x-textarea>-->
<div class="textarea-box">
<textarea placeholder="请您在此描述问题" v-model="description"></textarea>
<span>{{descripttionCount}}/500</span>
</div>
</div>
<x-button type="primary" class="btn">提交</x-button>
</div>
</template>
<script>
import { Checker, CheckerItem, InlineXNumber, XTextarea, XButton } from 'vux'
import CalcNumber from '../../components/CalcNumber.vue'
export default {
name: 'returnGoods',
data() {
return {
goods: {
img: require('../../assets/demo.png'),
name: 'JYLZ09-3060LED平板灯',
price: 12345,
count: 2
},
type: 'return', //服务类型
returnCount:1,
description:'',//问题描述
descripttionCount:0,//当前问题描述已经编写的文字长度
}
},
methods: {
calcDescription(v){
console.log(v)
if(this.description.length>=50){
this.description = this.description.substring(0,50);
}
this.descripttionCount = this.description.length;
}
},
watch:{
description:function(value,old){
console.log(value.length)
if(value.length>=500){
this.description = this.description.substring(0,500);
}
this.descripttionCount = value.length;
}
},
components: {
Checker,
CheckerItem,
CalcNumber,
InlineXNumber,
XTextarea,
XButton
},
}
</script>
<style scoped lang="less">
.goods-info {
height: 70px;
padding: 13px 15px;
background-color: #FFFFFF;
margin-bottom: 10px;
.img-box {
70px;
height: 100%;
float: left;
margin-right: 10px;
background-color: #f1f8ff;
overflow: hidden;
img {
100%;
height: 100%;
}
}
.name-count {
calc(~"100% - 80px");
height: 100%;
float: left;
font-size: 12px;
display: flex;
flex-direction: column;
justify-content: space-around;
position: relative;
p:first-of-type {
font-size: 14px;
line-height: 18px;
-webkit-line-clamp: 2;
display: -webkit-box;
-webkit-box-orient: vertical;
overflow: hidden;
}
p:last-of-type {
color: #666666;
}
}
}
.type-item {
77px;
height: 28px;
border: 1.5px solid #C4C4C4;
color: #C4C4C4;
text-align: center;
line-height: 28px;
font-size: 14px;
margin-right: 15px;
}
.type-item-selected, .return-type span {
77px;
height: 28px;
border: 1.5px solid #3A3A3C;
color: #3A3A3C;
text-align: center;
line-height: 28px;
font-size: 14px;
}
.service-type, .return-type, .apply-number, .description{
padding: 15px 15px 18px;
background-color: #FFFFFF;
font-size: 14px;
margin-bottom: 10px;
p{
margin-bottom: 15px;
}
}
.return-type span{
display: block;
88px;
}
.description{
margin-bottom: 42px;
}
.btn{
position: fixed;
bottom: 0px;
left: 0px;
}
.textarea-box{
padding: 10px;
background-color: #f8f8f8;
border-radius: 4px;
overflow: hidden;
textarea{
100%;
height: 90px;
resize:none;
line-height: 18px;
font-size: 14px;
border: none;
outline: none;
background-color: #f8f8f8;
}
span{
float: right;
color: #a8a8a8;
}
}
</style>
本文学习自这位靓仔