今天写程序,遇到了一个要实现string.split()这个的一个函数。python里面有,qt里面有,c++里面没有。照着网上抄了一个,放在这里。有需要的时候直接拽过去用,否则老是写了小例子就扔,用的时候没有,也是个麻烦事
例如 “aa*bb*cc” 会存储成vector<string> "aa" "bb" "cc"
1 // temp1.cpp : 定义控制台应用程序的入口点。 2 3 #include "stdafx.h" 4 #include <iostream> 5 #include <stdio.h> 6 #include <string> 7 #include <vector> 8 using namespace std; 9 10 11 int _tmain(int argc, _TCHAR* argv[]) 12 { 13 char a[] = "abc*123*xyz"; //目标是解析成 abc 123 xyz 然后存储在下面的变量 vector<string>中 14 string strArry = a; 15 vector<string> strArryList; 16 17 18 size_t last = 0; 19 size_t index = strArry.find_first_of("*",last); //找到last坐标后面的第一个* 20 while( index != std::string::npos )//找到一个推进vector一个,一直到找到了最后 21 { 22 strArryList.push_back( strArry.substr(last, index-last)); 23 last = index +1; 24 index = strArry.find_first_of("*",last); 25 } 26 if(index - last > 0) //记得把最后一个推进去.这里是"xyz" 27 { 28 strArryList.push_back( strArry.substr(last, index-last)); 29 } 30 31 32 for(int i = 0; i < strArryList.size(); i++) 33 std::cout<<strArryList[i]<<std::endl; 34 35 getchar(); 36 return 0; 37 }
下面是又写的一个split函数的代码
1 #include <iostream> 2 #include <string> 3 #include <vector> 4 5 using namespace std; 6 // spCharacter [IN] : 分隔符 7 // objString [IN] : 要分解的字符串 8 // stringVector [OUT] : 分解了的字符串 9 bool splitString(char spCharacter, const string& objString, vector<string>& stringVector) 10 { 11 if (objString.length() == 0) 12 { 13 return true; 14 } 15 16 size_t posBegin = 0; 17 size_t posEnd = 0; 18 bool lastObjStore = true; 19 20 while (posEnd != string::npos) 21 { 22 posBegin = posEnd; 23 posEnd = objString.find(spCharacter, posBegin); 24 25 if (posBegin == posEnd) 26 { 27 posEnd += 1; 28 continue; 29 } 30 31 if (posEnd == string::npos) 32 { 33 stringVector.push_back( objString.substr(posBegin, objString.length()-posBegin) ); 34 break; 35 } 36 37 stringVector.push_back( objString.substr( posBegin, posEnd - posBegin) ); 38 posEnd += 1; 39 } 40 return true; 41 } 42 int main(int argc, char** argv) 43 { 44 vector<string> paths; 45 paths.push_back("abcde"); 46 paths.push_back("aaar/"); 47 paths.push_back("aaar//"); 48 paths.push_back("aaar/bbb"); 49 paths.push_back("aaar/c/bbb"); 50 paths.push_back("aaar//bbb"); 51 paths.push_back("/aar"); 52 paths.push_back("/"); 53 paths.push_back(""); 54 55 for (auto iter = paths.begin(); iter != paths.end(); iter++) 56 { 57 vector<string> temp; 58 splitString('/', *iter, temp); 59 60 cout<<"--------begin------"<<endl; 61 cout<<"the source string : ["<<*iter<<"]"<<endl; 62 cout<<"-------------------"<<endl; 63 for (auto iterSplit = temp.begin(); iterSplit != temp.end(); iterSplit++) 64 { 65 cout<<*iterSplit<<endl; 66 } 67 cout<<"--------end------"<<endl<<endl;; 68 } 69 70 return 0; 71 }
编译命令: g++ -o test main.cpp -std=c++11
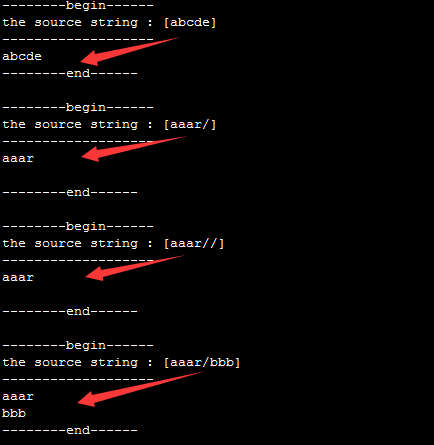
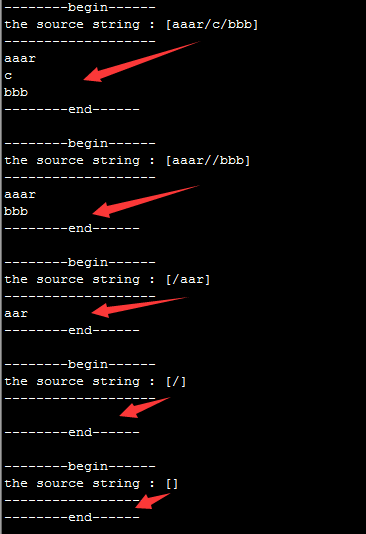