知识点:
后台:将上传的图片写入指定服务器路径,保存起来,返回上传后的图片路径(在springBoot中,参考博客:http://blog.csdn.net/change_on/article/details/59529034)
前端:在Vue.js前端框架中,使用Vue_Core_Image_Upload插件,上传图片 (github地址:https://github.com/Vanthink-UED/vue-core-image-upload)
后台:
1)在Controller中写一个方法,处理上传图片文件
package com.hand.hand.controller;
import com.hand.hand.util.FileUtil; //文件工具类
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.CrossOrigin;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
/**
* Created by nishuai on 2017/12/26.
*/
@CrossOrigin
@Controller
public class FileUploadController {
//处理文件上传
@RequestMapping(value="/uploadimg", method = RequestMethod.POST)
public @ResponseBody String uploadImg(@RequestParam("file") MultipartFile file,
HttpServletRequest request) {
String contentType = file.getContentType(); //图片文件类型
String fileName = file.getOriginalFilename(); //图片名字
//文件存放路径
String filePath = "C:\Users\Administrator\Desktop\vue-manage-system-master\static\uploadimg\";
//调用文件处理类FileUtil,处理文件,将文件写入指定位置
try {
FileUtil.uploadFile(file.getBytes(), filePath, fileName);
} catch (Exception e) {
// TODO: handle exception
}
// 返回图片的存放路径
return filePath;
}
}
2)FileUtil工具类,实现uploadFile方法
package com.hand.hand.util;
import java.io.File;
import java.io.FileOutputStream;
/**
* Created by nishuai on 2017/12/26.
*/
public class FileUtil{
//文件上传工具类服务方法
public static void uploadFile(byte[] file, String filePath, String fileName) throws Exception{
File targetFile = new File(filePath);
if(!targetFile.exists()){
targetFile.mkdirs();
}
FileOutputStream out = new FileOutputStream(filePath+fileName);
out.write(file);
out.flush();
out.close();
}
}
前台
3)Vue中使用Vue-core-images-upload插件实现图片的上传功能(可看考官方文档)
<div class="img">
<img class="pre-img" :src="src" alt="">
<vue-core-image-upload :class="['pure-button','pure-button-primary','js-btn-crop']"
:crop="false"
text="上传图片"
inputOfFile="file" //输出文件的名称
url="http://localhost:5050/uploadimg" //服务器api
extensions="png,gif,jpeg,jpg"
@imageuploaded="imageuploaded" //图片上传成功后调用此方法
@errorhandle="handleError"></vue-core-image-upload>
</div>
<script>
import VueCoreImageUpload from 'vue-core-image-upload'
export default {
components: {
'vue-core-image-upload': VueCoreImageUpload,
},
data() {
return {
src: './static/img/hospital1.jpg' //默认的图片路径
}
},
methods: {
imageuploaded(res) { //图片上传成功后调用此方法,res为返回值为服务器存放图片的路径,再将图片路径赋值给src
console.log("文件上传成功!");
this.src=res;
},
handleError(){ //图片上失败后调用此方法
console.log("文件上传失败!");
this.$notify.error({
title: '上传失败',
message: '图片上传接口上传失败,可更改为自己的服务器接口'
});
}
}
};
</script>
4)前端页面效果,点击上传图片可调用,http://localhost:5050/uploadimg后端接口
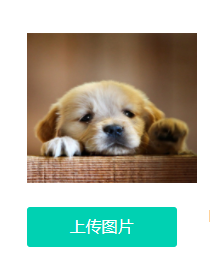