#include <iostream>
//迭代法
class Node{
public:
int value;
Node* next;
Node(int data){
this->value = data;
}
} ;
class Node{
public:
int value;
Node* next;
Node(int data){
this->value = data;
}
} ;
Node* reverseNode(Node* head)
{
Node* pre = head;
{
Node* pre = head;
Node* cur = NULL;
while(pre!=NULL)
{
{
Node* tem = pre->next;
pre->next = cur;
cur = pre;
pre = tem;
}
return cur;
}
//递归法
Node* d_reverseNode(Node* head)
{
Node* d_reverseNode(Node* head)
{
if(head==NULL||head->next==NULL)
{
return head;
}
{
return head;
}
Node* tem = d_reverseNode(head->next);
head->next->next = head;
head->next = NULL;
return tem;
head->next->next = head;
head->next = NULL;
return tem;
}
int main()
{
{
Node* node1 = new Node(1);
Node* node2 = new Node(2);
Node* node2 = new Node(2);
Node* node3 = new Node(3);
Node* node4 = new Node(4);
Node* node4 = new Node(4);
Node* node5 = new Node(5);
Node* head = node1;
head->next = node2;
node2->next = node3;
node3->next = node4;
node4->next = node5;
Node* head = node1;
head->next = node2;
node2->next = node3;
node3->next = node4;
node4->next = node5;
node5->next = NULL;
Node* cur = head;
while(cur!=nullptr){
Node* cur = head;
while(cur!=nullptr){
std::cout << cur->value << ' ';
cur = cur->next;
cur = cur->next;
}
Node* t = reverseNode(head);
Node* tem = t;
std::cout << ' ';
Node* tem = t;
std::cout << ' ';
while(t!=nullptr)
{
std::cout << t->value << ' ';
t = t->next;
}
std::cout << ' ';
Node* t_1 = d_reverseNode(tem);
{
std::cout << t->value << ' ';
t = t->next;
}
std::cout << ' ';
Node* t_1 = d_reverseNode(tem);
while(t_1!=nullptr)
{
std::cout << t_1->value << ' ';
t_1 = t_1->next;
}
}
{
std::cout << t_1->value << ' ';
t_1 = t_1->next;
}
}
测试结果:
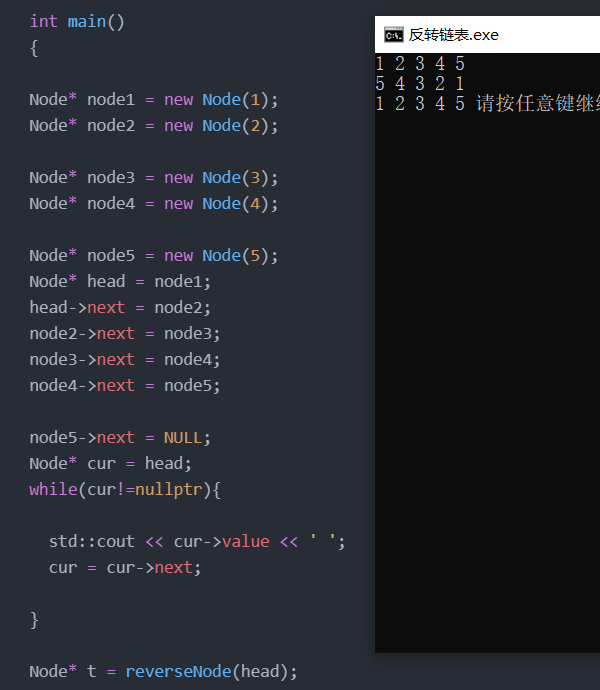