代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
#box {
width: 500px;
height: 400px;
margin: 50px auto;
text-align: center;
}
#banner {
width: 500px;
height: 375px;
position: relative;
}
#banner img {
width: 100%;
height: 100%;
}
p {
position: absolute;
left: 0;
height: 30px;
line-height: 30px;
background: rgba(0, 0, 0, 0.513);
color: #fff;
font-weight: bold;
width: 100%;
}
p:nth-child(1) {
top: 0px;
}
p:last-child {
bottom: 0px;
}
#banner button {
position: absolute;
width: 50px;
height: 40px;
}
#left {
left: 0;
top: 0;
bottom: 0;
margin: auto;
}
#right {
right: 0;
top: 0;
bottom: 0;
margin: auto;
}
.active{
background:orange;
color:#fff;
}
</style>
</head>
<body>
<div id="box">
<button class="active">顺序</button>
<button>循环</button>
<div id="banner">
<p>1/4</p>
<button id="left">左</button>
<button id="right">右</button>
<img src="images/onepiece.jpg" alt="">
<p>合影</p>
</div>
</div>
<script>
var btn = document.getElementsByTagName('button');
var ps = document.getElementsByTagName('p');
var img = document.getElementsByTagName('img')[0];
var arr = ['images/onepiece.jpg', 'images/onepiece2.jpg', 'images/onepiece3.jpg', 'images/onepiece4.jpg'];
var arr_title = ['合照','乔巴','路飞','索隆'];
var flag = true; // true-顺序【默认】 false-循环
var num = 0;
// 顺序按钮
btn[0].onclick = function(){
flag = true;
this.className = 'active';
btn[1].className = '';
}
// 循环按钮
btn[1].onclick = function(){
flag = false;
this.className = 'active';
btn[0].className = '';
}
// 右翻页
btn[3].onclick = function () {
console.log(flag)
num++; // 0 1 2 3
if (flag) { // 顺序播放时
if (num > arr.length - 1) {
num = 3;
alert('已经是最后一张啦!');
}
img.src = arr[num];
// 修改标题
ps[1].innerHTML = arr_title[num];
// 当前页
ps[0].innerHTML = (num+1) + '/' + arr.length;
}else{ // 循环播放
if(num > arr.length - 1){
num = 0;
}
img.src = arr[num];
// 修改标题
ps[1].innerHTML = arr_title[num];
// 当前页
ps[0].innerHTML = (num+1) + '/' + arr.length;
}
}
// 左翻页
btn[2].onclick = function(){
num--;
if(flag){ // 顺序
if(num < 0){
num = 0;
alert('已经是第一张啦!')
}
img.src = arr[num];
// 修改标题
ps[1].innerHTML = arr_title[num];
// 当前页
ps[0].innerHTML = (num+1) + '/' + arr.length;
}else{ // 循环
if(num < 0 ){
num = arr.length - 1;
}
img.src = arr[num];
// 修改标题
ps[1].innerHTML = arr_title[num];
// 当前页
ps[0].innerHTML = (num+1) + '/' + arr.length;
}
}
/*
1.设定顺序,循环 开关 true- false-
2.翻页,右 ,先判断开关状态,如果顺序, 4321 如果循环 43214321
3.翻页,左 ....
4.修改标题,当前页
*/
</script>
</body>
</html>
效果
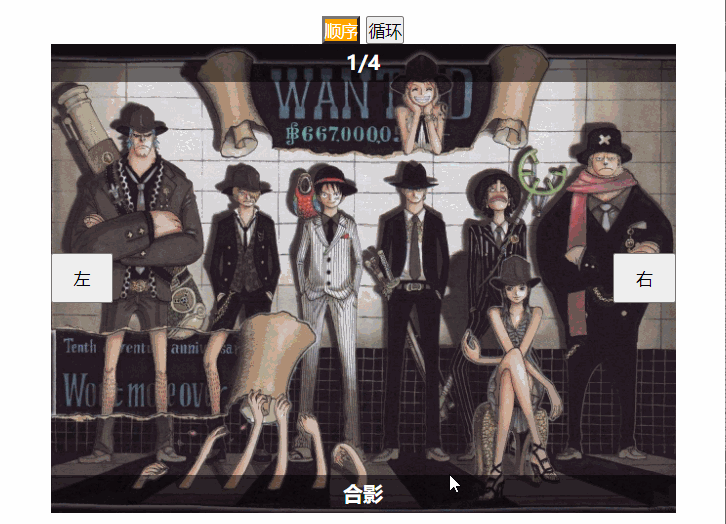