数塔
http://acm.hdu.edu.cn/showproblem.php?pid=2084
Problem Description
在讲述DP算法的时候,一个经典的例子就是数塔问题,它是这样描述的:
有如下所示的数塔,要求从顶层走到底层,若每一步只能走到相邻的结点,则经过的结点的数字之和最大是多少?
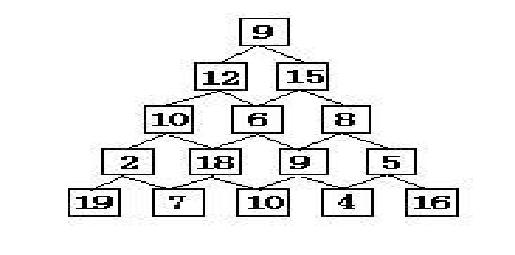
已经告诉你了,这是个DP的题目,你能AC吗?
有如下所示的数塔,要求从顶层走到底层,若每一步只能走到相邻的结点,则经过的结点的数字之和最大是多少?
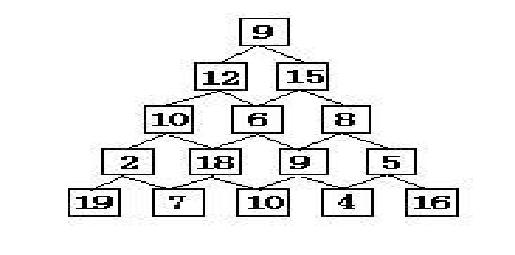
已经告诉你了,这是个DP的题目,你能AC吗?
Input
输入数据首先包括一个整数C,表示测试实例的个数,每个测试实例的第一行是一个整数N(1 <= N <= 100),表示数塔的高度,接下来用N行数字表示数塔,其中第i行有个i个整数,且所有的整数均在区间[0,99]内。
Output
对于每个测试实例,输出可能得到的最大和,每个实例的输出占一行。
Sample Input
1
5
7
3 8
8 1 0
2 7 4 4
4 5 2 6 5
Sample Output
30
动态方程:dp[i][j] = max(dp[i-1][j] + map[i][j], dp[i-1][j-1] + map[i][j]);
解题代码:

1 #include <stdio.h> 2 #include <math.h> 3 #include <string.h> 4 #include <iostream> 5 using namespace std; 6 7 const int MAX = 104; 8 9 int dp[MAX][MAX], map[MAX][MAX]; 10 11 int main () 12 { 13 int n, T; 14 scanf("%d", &T); 15 int max_sum; 16 while (T--) 17 { 18 scanf ("%d", &n); 19 memset(map, 0, sizeof (map)); 20 memset(dp, 0, sizeof (dp)); 21 for (int i = 1; i <= n; i ++) 22 { 23 for (int j = 1; j <= i; j ++) 24 scanf ("%d", &map[i][j]); 25 } 26 max_sum = 0; 27 for (int i = 1; i <= n; i ++) 28 { 29 for (int j = 1; j <= i; j ++) 30 { 31 dp[i][j] = max(dp[i-1][j] + map[i][j], dp[i-1][j-1] + map[i][j]); 32 if (dp[i][j] > max_sum) 33 max_sum = dp[i][j]; 34 } 35 } 36 printf ("%d ", max_sum); 37 } 38 return 0; 39 }